Java Code Examples Part 1
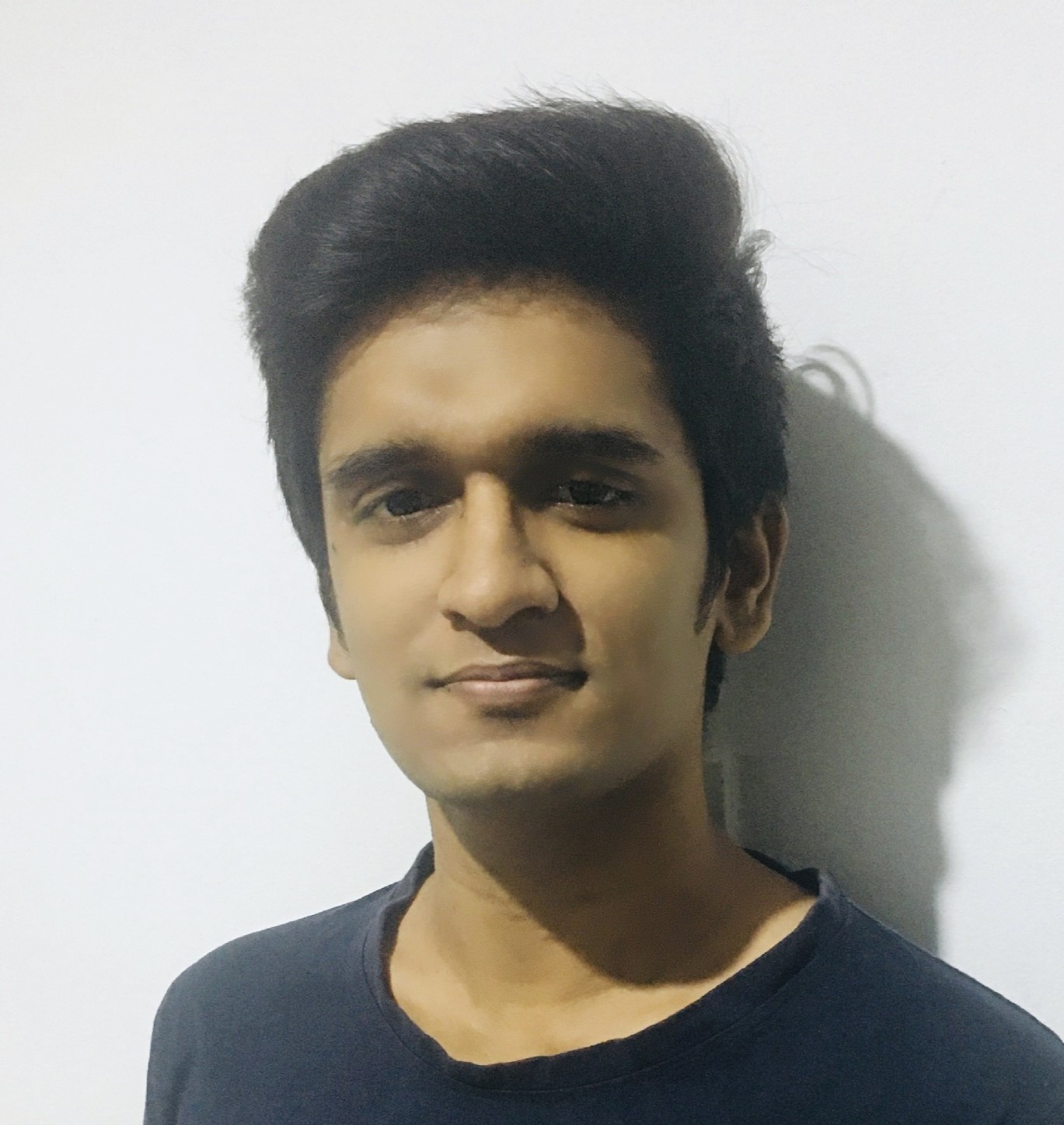
Table of contents
- Example 1: How to swap the values of two variables without using a temporary variable in Java?
- Example 2: How to check if a given string is a palindrome in Java?
- Example 3: How would you find the maximum element in an array using Java?
- Example 4: How to sort an array of integers in ascending order using the bubble sort algorithm in Java?
- Example 5: How to remove duplicates from an ArrayList in Java?
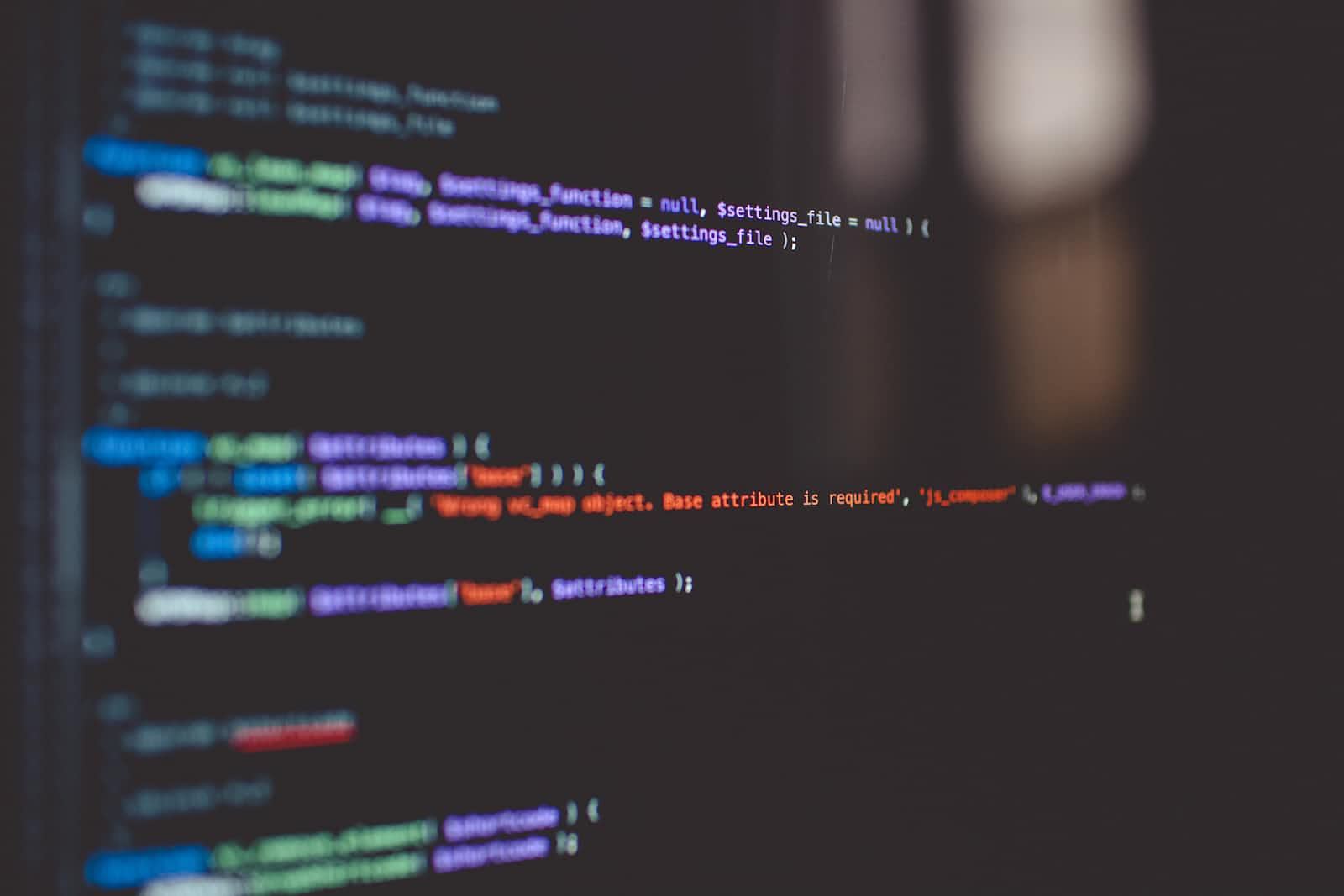
Example 1: How to swap the values of two variables without using a temporary variable in Java?
To swap the values of two variables without using a temporary variable in Java, you can utilize the XOR (^) operator. Here's an example code snippet:
int a = 5;
int b = 10;
System.out.println("Before swapping:");
System.out.println("a = " + a);
System.out.println("b = " + b);
a = a ^ b;
b = a ^ b;
a = a ^ b;
System.out.println("After swapping:");
System.out.println("a = " + a);
System.out.println("b = " + b);
By performing XOR operations between the two variables, the original values are effectively swapped without requiring an additional temporary variable.
Example 2: How to check if a given string is a palindrome in Java?
To check if a given string is a palindrome in Java, you can use the following code:
public class PalindromeChecker {
public static boolean isPalindrome(String str) {
String reversed = new StringBuilder(str).reverse().toString();
return str.equals(reversed);
}
public static void main(String[] args) {
String word1 = "level";
String word2 = "hello";
System.out.println(word1 + " is a palindrome: " + isPalindrome(word1));
System.out.println(word2 + " is a palindrome: " + isPalindrome(word2));
}
}
In the isPalindrome
method, we create a new StringBuilder
object from the given string and use its reverse()
method to obtain the reversed version of the string. Then, we compare the original string with the reversed string using the equals()
method. If they are equal, the string is a palindrome.
In the main
method, we demonstrate the usage of the isPalindrome
method by checking two example words: "level" (which is a palindrome) and "hello" (which is not a palindrome).
Example 3: How would you find the maximum element in an array using Java?
To find the maximum element in an array using Java, you can iterate through the array and keep track of the maximum value encountered. Here's an example code snippet:
public class MaximumElementFinder {
public static int findMax(int[] array) {
if (array == null || array.length == 0) {
throw new IllegalArgumentException("Array is empty or null.");
}
int max = array[0];
for (int i = 1; i < array.length; i++) {
if (array[i] > max) {
max = array[i];
}
}
return max;
}
public static void main(String[] args) {
int[] numbers = { 5, 2, 9, 1, 7 };
int maxNumber = findMax(numbers);
System.out.println("The maximum number in the array is: " + maxNumber);
}
}
In the findMax method, we initialize the max variable with the first element of the array. Then, we iterate through the remaining elements of the array. If we encounter a number greater than the current max, we update the max variable with the new value. Finally, we return the maximum value found.
In the main method, we demonstrate the usage of the findMax method by passing an array of numbers. The program then prints the maximum number found in the array.
Example 4: How to sort an array of integers in ascending order using the bubble sort algorithm in Java?
To sort an array of integers in ascending order using the bubble sort algorithm in Java, you can use the following code:
public class BubbleSort {
public static void bubbleSort(int[] array) {
int n = array.length;
boolean swapped;
for (int i = 0; i < n - 1; i++) {
swapped = false;
for (int j = 0; j < n - i - 1; j++) {
if (array[j] > array[j + 1]) {
// Swap array[j] and array[j+1]
int temp = array[j];
array[j] = array[j + 1];
array[j + 1] = temp;
swapped = true;
}
}
// If no two elements were swapped in the inner loop, the array is already sorted
if (!swapped) {
break;
}
}
}
public static void main(String[] args) {
int[] numbers = { 5, 2, 9, 1, 7 };
System.out.println("Before sorting:");
for (int number : numbers) {
System.out.print(number + " ");
}
bubbleSort(numbers);
System.out.println("\nAfter sorting:");
for (int number : numbers) {
System.out.print(number + " ");
}
}
}
In the 'bubbleSort' method, we use nested loops to compare adjacent elements in the array and swap them if they are in the wrong order. The outer loop iterates n-1 times, where n is the length of the array. The inner loop compares elements and performs swaps.
In the main method, we demonstrate the usage of the bubbleSort method by passing an array of numbers. The program prints the array before sorting, performs the bubble sort algorithm, and then prints the sorted array.
Example 5: How to remove duplicates from an ArrayList in Java?
To remove duplicates from an ArrayList in Java, you can use the following code:
import java.util.ArrayList;
import java.util.HashSet;
public class RemoveDuplicatesFromArrayList {
public static void main(String[] args) {
ArrayList<Integer> numbers = new ArrayList<>();
numbers.add(1);
numbers.add(2);
numbers.add(3);
numbers.add(2);
numbers.add(4);
numbers.add(3);
numbers.add(5);
System.out.println("ArrayList with duplicates: " + numbers);
HashSet<Integer> uniqueSet = new HashSet<>(numbers);
ArrayList<Integer> uniqueList = new ArrayList<>(uniqueSet);
System.out.println("ArrayList without duplicates: " + uniqueList);
}
}
In the code above, we start with an ArrayList called numbers that contains duplicate elements. We create a new HashSet called uniqueSet and pass the numbers list to its constructor, which automatically eliminates duplicates.
Next, we create a new ArrayList called uniqueList and pass the uniqueSet to its constructor. This creates a new list with unique elements only.
Finally, we print the original list with duplicates and the new list without duplicates to demonstrate the removal of duplicates.
Subscribe to my newsletter
Read articles from Nipun Shihara directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
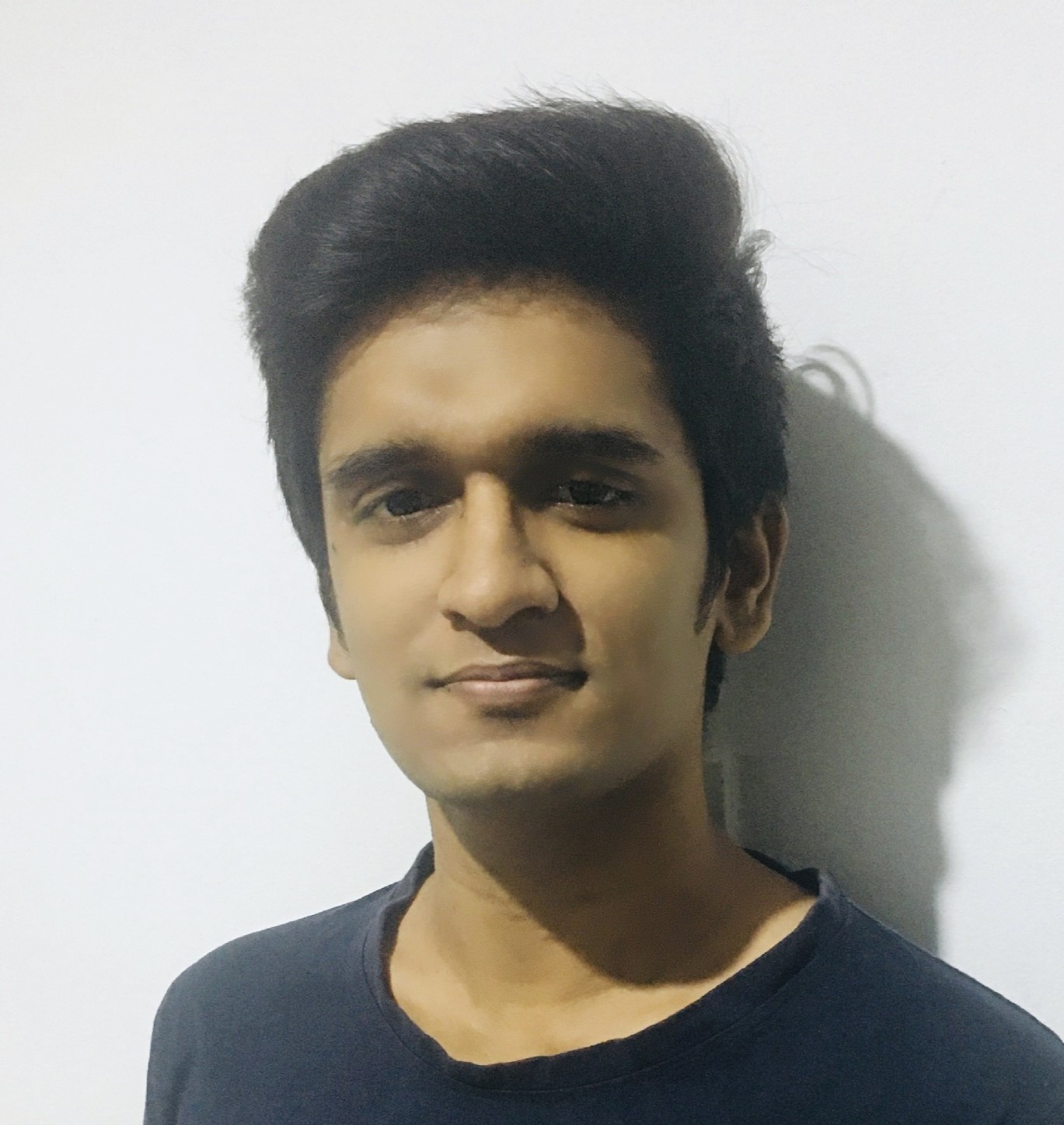
Nipun Shihara
Nipun Shihara
I am a dedicated individual with exceptional interpersonal skills, analytical skills and an outstanding team player with an enthusiasm to explore new things. My aspiration is to reach heights by joining a company that offers a stable and positive atmosphere and encourages me to enhance my professional skills further.