Array and it's methods in JavaScript
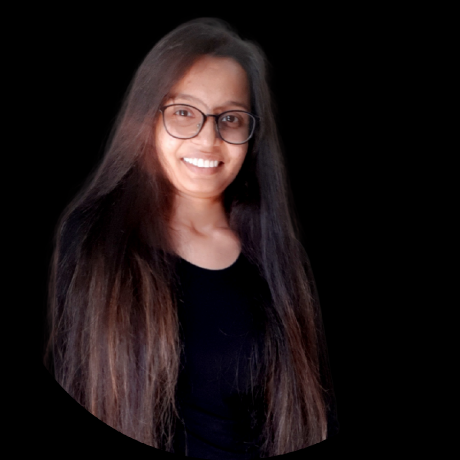
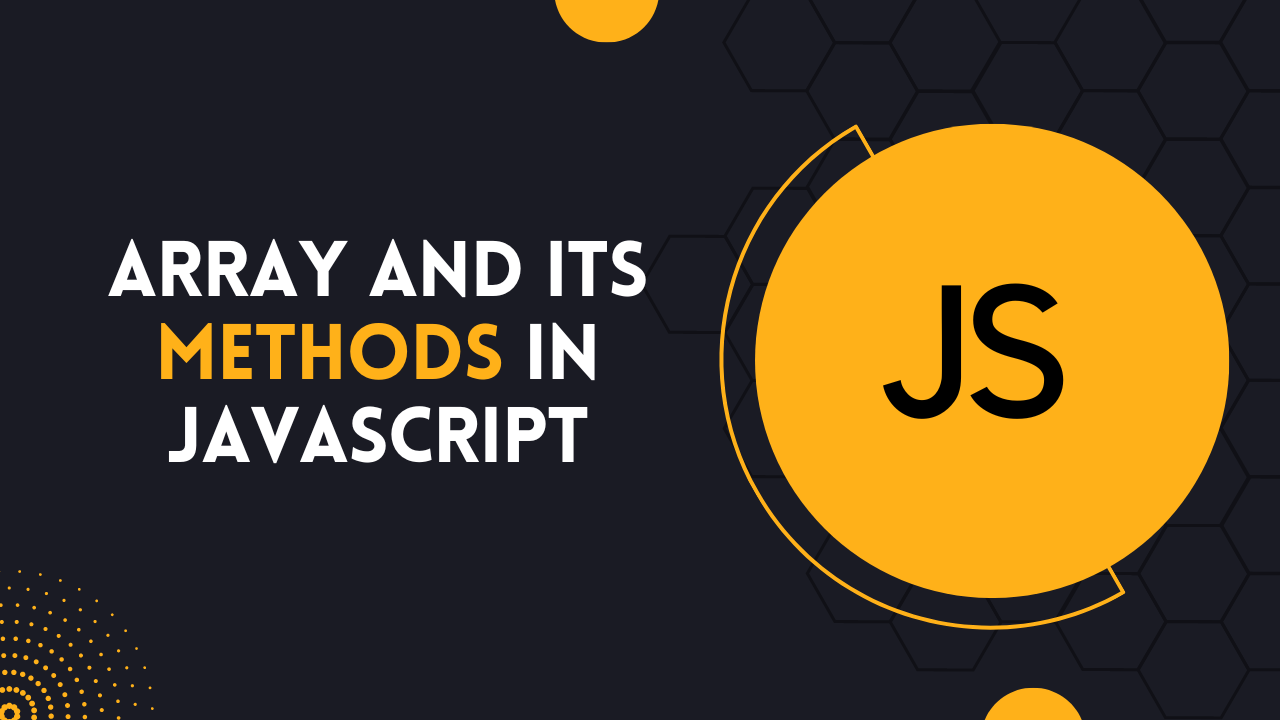
Introduction to Arrays in JS
An array is a data structure in JavaScript that enables you to store and manage a set of values. It is a technique for combining related data under a single variable name.
Arrays in JavaScript are dynamic, which means you can add or remove elements from them at runtime.
Arrays in JavaScript can be defined in several ways:
Using
square
brackets:const myArray = [1, 2, 3, 4, 5];
Using the
new
keyword:const myArray = new Array(1, 2, 3, 4, 5);
Using the
Array.from()
method:const myArray = Array.from([1, 2, 3, 4, 5]);
Arrays in JavaScript can contain any type of data, including other arrays, objects, and functions. The elements in an array are indexed starting from 0, so the first element in the array can be accessed with myArray[0].
Array Method
Length
The length property determines or returns the array's element count.
Syntax: array.length
names = ["loe", "joe", "zoe", "tom"]
console.log(names.length); // Length of Array
// OP: 4
Push
Adds new items to the end of an array.
Changes the length of the array.
Syntax: array.push("Values")
names = ["loe", "joe", "zoe", "tom"]
names.push("bob")
console.log(names);
// OP: ["loe", "joe", "zoe", "tom", "bob"]
Slice
Returns selected elements in an array, as a new array.
Selects from start to end(not inclusive)
Did not change the original array.
Syntax: array.slice(start, end)
names = ["loe", "joe", "zoe", "tom"]
array.slice(1, 3)
console.log(names);
// OP: ["joe", "zoe"]
Splice
Adds and/or removes array elements.
Overwrites the original array.
syntax: array.splice(index, howmany, item1, ....., itemX)
index: from ehere to start
howmany: how many to del
items: list of items
names = ["loe", "joe", "zoe", "tom"]
names.splice(2, 0, "lilo")
console.log(names);
// OP: ["loe", "joe", "lilo", "zoe", "tom", "bob"]
Concatenation
Join two or more arrays.
Returns a new array, containing the joined arrays.
Do not change the existing arrays.
Syntax: array1.concat(array2, array3, ..., arrayX)
name1 = ["loe", "joe"]
name2 = ["zoe", "tom"]
console.log(name1.concat(name2));
// OP: ["loe", "joe", "zoe", "tom"]
Fill
Fills specified elements in an array with a value.
Overwrites the original array.
Syntax: array.fill(value, start, end)
let arr = [1, 2, 3, 4, 5, 6, 7]
arr.fill('nandini', 2, 5)
console.log(arr)
// OP: [1, 2, 'nandini', 'nandini', 'nandini', 6, 7]
Includes
Returns
true
if an array contains a specified value.Returns
false
if the value is not found.Includes method is case sensitive.
array.includes(element, start)
element: The value to search for
start: Start position, default is 0.
let num = [1, 2, 3, 4, 5, 6, 7, 8];
console.log(num.includes(7, 7));
// OP: False
IndexOf
Returns the first index of a specified value.
Returns -1 if the value is not found.
Starts at a specified index and searches from left to right.
By default, the search starts at the first element and ends at the last.
Negative start values count from the last element (but still search from left to right).
Syntax: array.indexOf(item, start)
let num = [1, 2, 3, 'Nandini', 4, 5, 6, 7, 8, 'Nandini', 'Nandini'];
console.log(num.indexOf('Nandini'));
// OP: 3
lastindexOf
Returns the last index (position) of a specified value.
Returns -1 if the value is not found.
Starts at a specified index and searches from left to right.
By default, the search starts at the last element and ends at the first.
Negative start values count from the last element (but still search from left to right).
Syntax: array.lastIndexOf(item, start)
let num = [1, 2, 3, 'Nandini', 4, 5, 6, 7, 8, 'Nandini', 'Nandini'];
console.log(num.lastIndexOf('Nandini'));
// OP: 10
isArray
Returns
true
if an object is an array, otherwisefalse
.Syntax: Array.isArray(obj)
let num = [1, 2, 3, 'Nandini', 4, 5, 6, 7, 8, 'Nandini', 'Nandini'];
let num1 = 'Nandini';
console.log(Array.isArray(num1));
console.log(Array.isArray(num));
// OP
// false
// true
Map
Whatever is written inside the map will be linked with all the elements of an array
Creates a new array by calling a function for every array element.
Does not execute the function for empty elements.
Does not change the original array.
Syntax: array.map(function(currentValue, index, arr), thisValue)
function(): Required, A function to be run for each array element.
currentValue: Required, The value of the current element.
index: Optional, The index of the current element.
arr: Optional, The array of the current element.
thisValue: Optional, Default value is undefined.
A value passed to the function is to be used as this value.
let maths = [1, 4, 9, 16, 25];
console.log(maths.map(Math.sqrt));
// OP: [1, 2, 3, 4, 5]
Pop
12.Removes the last element of an array.
Changes the original array.
Returns the removed element.
Syntax: array.pop()
let maths = [1, 4, 9, 16, 25];
console.log(maths.pop());
console.log(maths);
// OP
// 25
// [1, 4, 9, 16]
Reverse
Reverses the order of the elements in an array.
Overwrites the original array.
Syntax: array.reverse()
let maths = [1, 4, 9, 16, 25];
console.log(maths.reverse());
// OP: [25, 16, 9, 4, 1]
Shift
Removes the first item of an array.
Changes the original array.
Returns the shifted element.
Syntax: array.shift()
let maths = ['Nandini', 1, 4, 9, 16, 25];
console.log(maths.shift());
console.log(maths);
// OP
// Nandini
// [1, 4, 9, 16, 25]
unShift
Adds new elements to the beginning of an array.
Overwrites the original array.
Syntax: array.unshift(item1, item2, ..., itemX)
let fruit = ['Apple', 'Bada Apple', 'Chota Apple', 'Double Apple'];
fruit.unshift('Apple 1', 'Grapes 2');
console.log(fruit);
// OP: ['Apple 1', 'Grapes 2', 'Apple', 'Bada Apple', 'Chota Apple', 'Double Apple']
Join
Returns an array as a string.
Does not change the original array.
Syntax: array.join(separator)
Any separator can be specified. The default is comma (,)
let Arr1 = [1, 2, 3, 4, 5, 6, 7];
let var1 = Arr1.join(' | ');
console.log(var1);
console.log(typeof var1);
// OP
// 1 | 2 | 3 | 4 | 5 | 6 | 7
// string
Sort
Sorts the elements of an array.
Overwrites the original array.
Sorts the elements as strings in alphabetical and ascending order.
Syntax: array.sort()
let names = ['Hitesh Sir', 'Anurag', 'Surya', 'Anirudh', 'Bipul'];
console.log(names.sort());
// OP: ['Anirudh', 'Anurag', 'Bipul', 'Hitesh Sir', 'Surya']
At
Returns an indexed element from an array.
Does not change the original array.
Syntax: array.at(index)
index: Optional, position of the array element to be returned, default is 0.
-1 returns the last element.
names = ["loe", "joe", "zoe", "tom"]
console.log(names.at(2));
// OP: zoe
With these powerful array methods at your disposal, you can unlock the full potential of JavaScript and take your coding skills to the next level.
Subscribe to my newsletter
Read articles from Nandini Chhajed directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
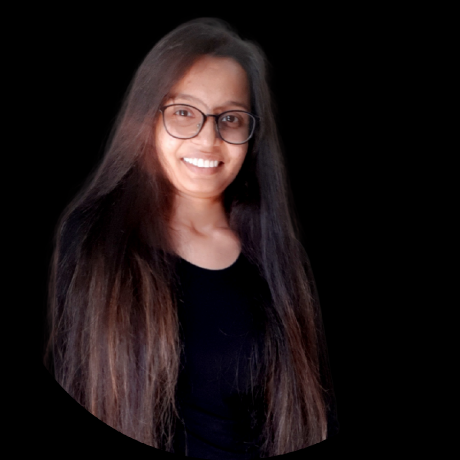
Nandini Chhajed
Nandini Chhajed
I am a final-year computer science student specializing in IoT. I am working on my web developer profile and aspire to become a Backend Developer. With a history of working at the Indian Space Research Organization (ISRO) as a mobile backend developer, During the course of this internship, I played a key role in developing a project to benefit the farmer’s community. This mobile application is a revolutionary way to help farmers ensure greater profitability through direct farmer-to-farmer, farmer-to-customer, and farmer-to-tractor owner communication. Working knowledge of C/C++, Python, Django, PostgreSQL, SQL databases, REST APIs, Kubernetes, Docker, Automation Testing, Version Control, and Microservice architecture, AdobeXD, and Figma. Dedicated to implementing object-oriented solutions, I am a Ui/Ux Designer proficient in personal interaction and communication to increase productivity. who bakes well and enjoys music. Dedicated to the responsibilities assigned to me.