Building Custom Gutenberg Blocks: A Step-by-Step Guide with Examples
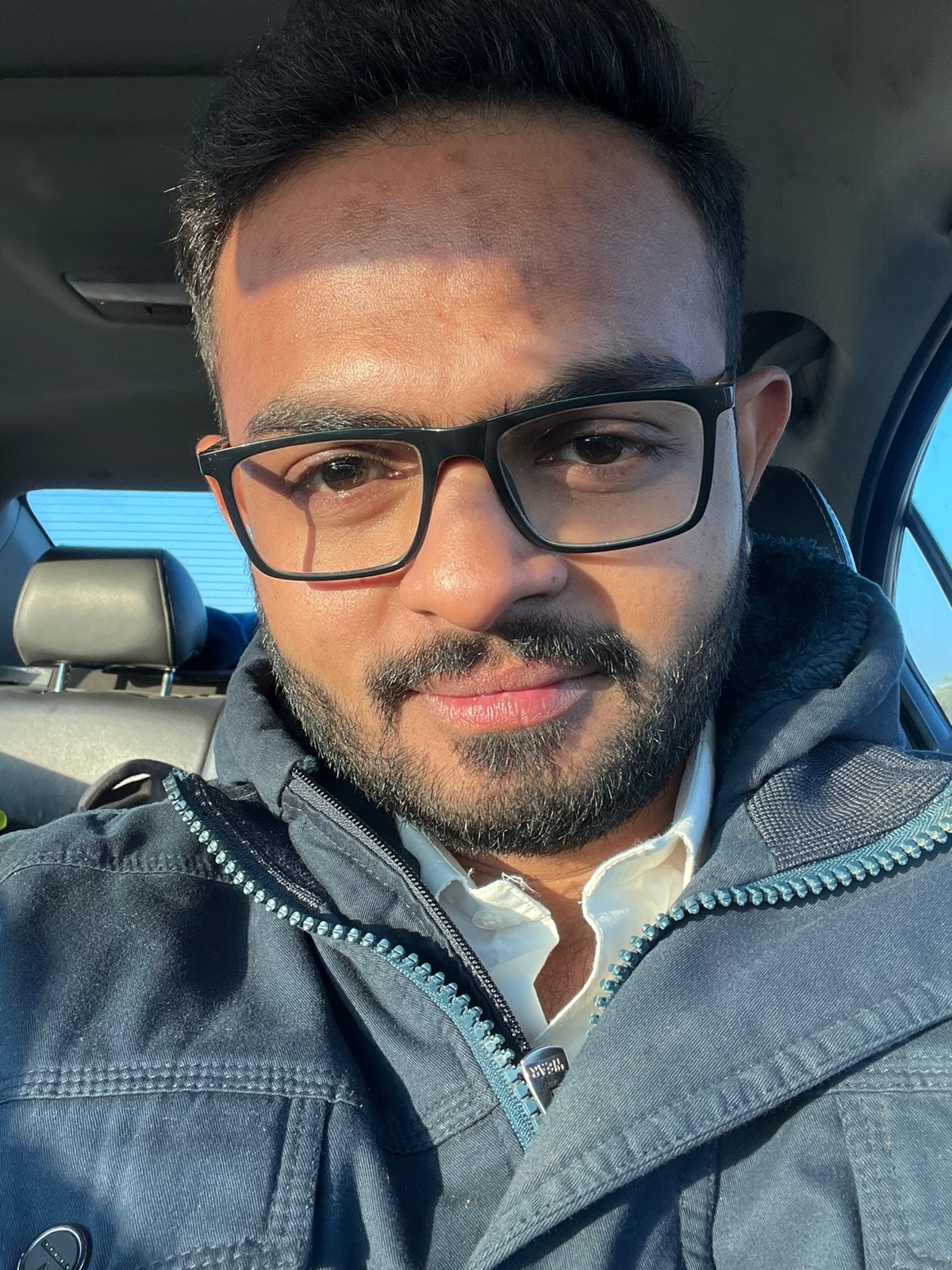
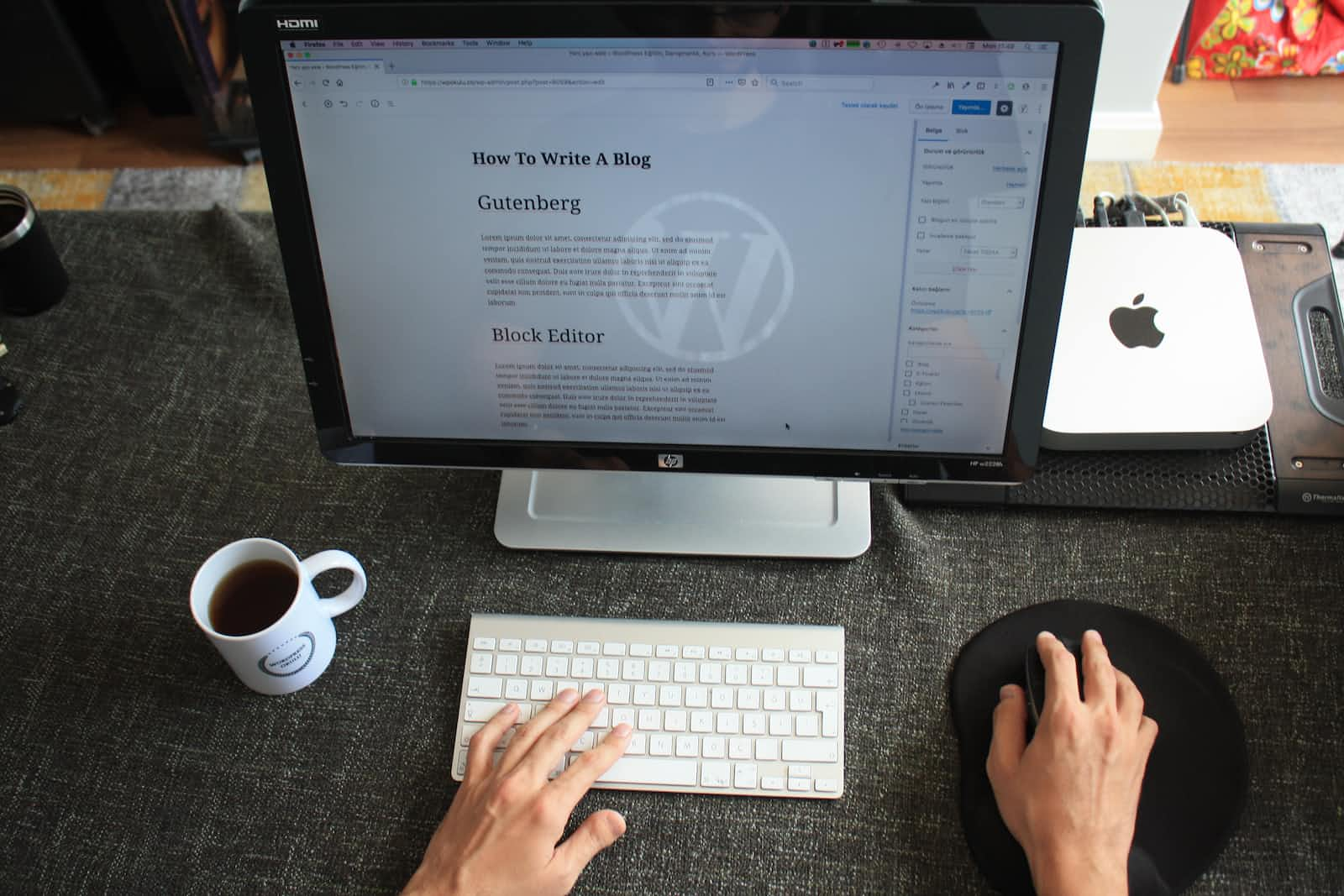
Introduction:
The Gutenberg block editor has revolutionized content creation in WordPress, allowing users to create rich, dynamic, and engaging layouts with ease. One of the most exciting aspects of Gutenberg is the ability to build custom blocks tailored to specific needs. In this article, we will guide you through the process of building your own Gutenberg block, from concept to implementation, with practical examples along the way.
Understanding Gutenberg Blocks:
Before diving into building custom blocks, it's crucial to understand the fundamentals of Gutenberg blocks. We'll cover the basics, explaining what blocks are, how they work, and the various types available (such as text, image, and media blocks).
Planning Your Custom Block:
A well-thought-out plan is essential for creating a successful custom block. We'll discuss the importance of defining the block's purpose, functionality, and user interface. We'll also explore how to sketch out the block structure and determine the attributes and settings required.
Setting Up the Development Environment:
To build custom Gutenberg blocks, you'll need a development environment that includes WordPress and the necessary tools. We'll walk you through the process of setting up a local development environment or using tools like CodePen or Glitch for a quick start.
Creating a Simple Custom Block:
We'll begin by building a simple custom block, starting with the block registration process. We'll cover the essential components, such as registering the block type, defining its attributes, and rendering the block's content. By the end of this section, you'll have a functioning custom block that you can use in your Gutenberg editor.
Advanced Custom Block Features:
In this section, we'll explore advanced features to enhance your custom block. Topics covered will include block styles, block variations, adding additional controls (such as color pickers or dropdowns), integrating block transformations, and handling dynamic data within the block.
Extending Custom Blocks with JavaScript:
To add interactivity and dynamic behavior to your custom block, JavaScript is your ally. We'll delve into JavaScript-based enhancements, covering event handling, AJAX requests, and other techniques to take your custom block to the next level.
Testing and Deploying Your Custom Block:
Once your custom block is built, it's crucial to test its functionality thoroughly. We'll discuss testing methodologies and tools, ensuring compatibility across browsers and devices. Additionally, we'll explore deployment options, including packaging your block as a plugin or sharing it on the WordPress plugin repository.
Certainly! Here's an example of a simple custom Gutenberg block:
First, create a new directory for your custom block plugin in the WordPress plugins directory.
Inside the plugin directory, create a file called
my-custom-block.php
and add the following code:<?php /* Plugin Name: My Custom Block Description: A simple custom Gutenberg block. Version: 1.0 Author: Your Name Author URI: Your Website */ function my_custom_block_init() { wp_register_script( 'my-custom-block-script', plugins_url('block.js', __FILE__), array('wp-blocks', 'wp-editor') ); register_block_type('my-custom-block/my-block', array( 'editor_script' => 'my-custom-block-script', )); } add_action('init', 'my_custom_block_init');
Next, create a JavaScript file called
block.js
in the same directory and add the following code:(function() { var el = wp.element.createElement; var registerBlockType = wp.blocks.registerBlockType; var RichText = wp.editor.RichText; registerBlockType('my-custom-block/my-block', { title: 'My Custom Block', icon: 'smiley', category: 'common', attributes: { content: { type: 'array', source: 'children', selector: 'p', }, }, edit: function(props) { var content = props.attributes.content; var onChangeContent = function(newContent) { props.setAttributes({ content: newContent }); }; return el( RichText, { tagName: 'p', className: props.className, onChange: onChangeContent, value: content, } ); }, save: function(props) { var content = props.attributes.content; return el('p', { className: props.className }, content); }, }); })();
- Save the files and activate the plugin in the WordPress admin panel.
Once activated, you'll be able to add the "My Custom Block" to your Gutenberg editor. It will display a simple paragraph block where you can enter and save text.
Here's another example of creating the same custom Gutenberg block using Advanced Custom Fields (ACF):
Install and activate the Advanced Custom Fields plugin from the WordPress.org plugin repository.
Go to Custom Fields in the WordPress admin menu and create a new field group.
Add a new field to the field group with the following details:
Label: Content
Name: content
Type: WYSIWYG Editor
Save the field group.
Create a new directory for your custom block plugin in the WordPress plugins directory.
Inside the plugin directory, create a file called
my-acf-block.php
and add the following code:<?php /* Plugin Name: My ACF Block Description: A simple custom Gutenberg block using ACF. Version: 1.0 Author: Your Name Author URI: Your Website */ function my_acf_block_init() { if (function_exists('acf_register_block_type')) { acf_register_block_type(array( 'name' => 'my-acf-block', 'title' => 'My ACF Block', 'description' => 'A simple custom Gutenberg block using ACF.', 'render_callback' => 'my_acf_block_render_callback', 'category' => 'common', 'icon' => 'smiley', 'keywords' => array('custom', 'block'), )); } } add_action('acf/init', 'my_acf_block_init'); function my_acf_block_render_callback($block, $content = '', $is_preview = false) { $custom_class = 'my-acf-block'; if ($is_preview) { $custom_class .= ' preview'; } $content = get_field('content'); if (empty($content)) { $content = 'Enter your custom block content here.'; } echo '<div class="' . $custom_class . '">' . $content . '</div>'; }
- Save the file and activate the plugin in the WordPress admin panel.
Once activated, you'll be able to add the "My ACF Block" to your Gutenberg editor. It will display a block that renders the content entered in the ACF field.
Note: Remember to adjust the plugin name, description, author information, and other details according to your preferences. Additionally, you can customize the block's appearance and behavior by modifying the render callback function.
Ensure that you have the Advanced Custom Fields plugin installed and configured correctly before using this custom ACF block.
We're glad you found the above article helpful! If it provided value and insights, we kindly request you to show your appreciation by liking, commenting, and sharing it with your colleagues. Sharing knowledge is a wonderful way to empower others and help them discover new ideas. By spreading the word, you contribute to creating a community of learners who can benefit from these WordPress tricks and tips. Together, let's build a network of knowledge and inspire others to explore the possibilities of WordPress. Thank you for your support!
Do you still need assistance/help?
Some helpful links :
https://developer.wordpress.org/block-editor/
https://www.advancedcustomfields.com/resources/blocks/
Need Assistance?
Need assistance with upgrading to GA4 and integrating it with your WordPress website? Hire a skilled developer with expertise in Google Analytics and WordPress to ensure a smooth transition and maximize the benefits of advanced analytics for your business.
Subscribe to my newsletter
Read articles from Ramiz Theba directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
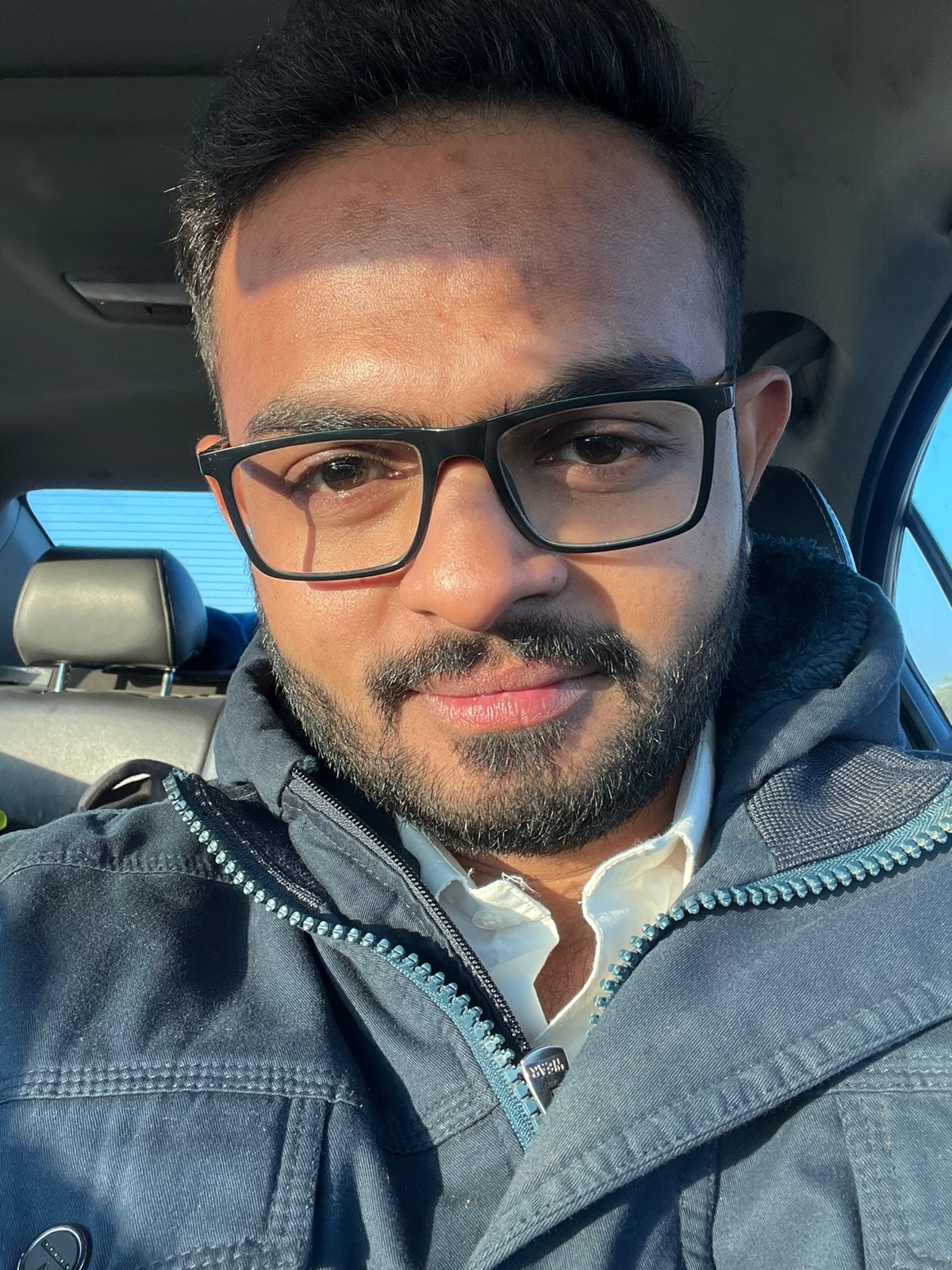
Ramiz Theba
Ramiz Theba
As a web developer, I have a passion for creating visually stunning and user-friendly websites. With several years of experience in both front-end and back-end development, I am well-versed in a variety of programming languages and frameworks. I am a problem solver at heart, and I enjoy the challenge of finding solutions to complex issues. I am always learning and staying current with the latest web technologies to ensure that my clients' websites are cutting-edge. My ultimate goal is to create websites that not only look great, but also provide a seamless and enjoyable user experience.