🔥Exploring the Power of Express.js: Simplifying Web Development
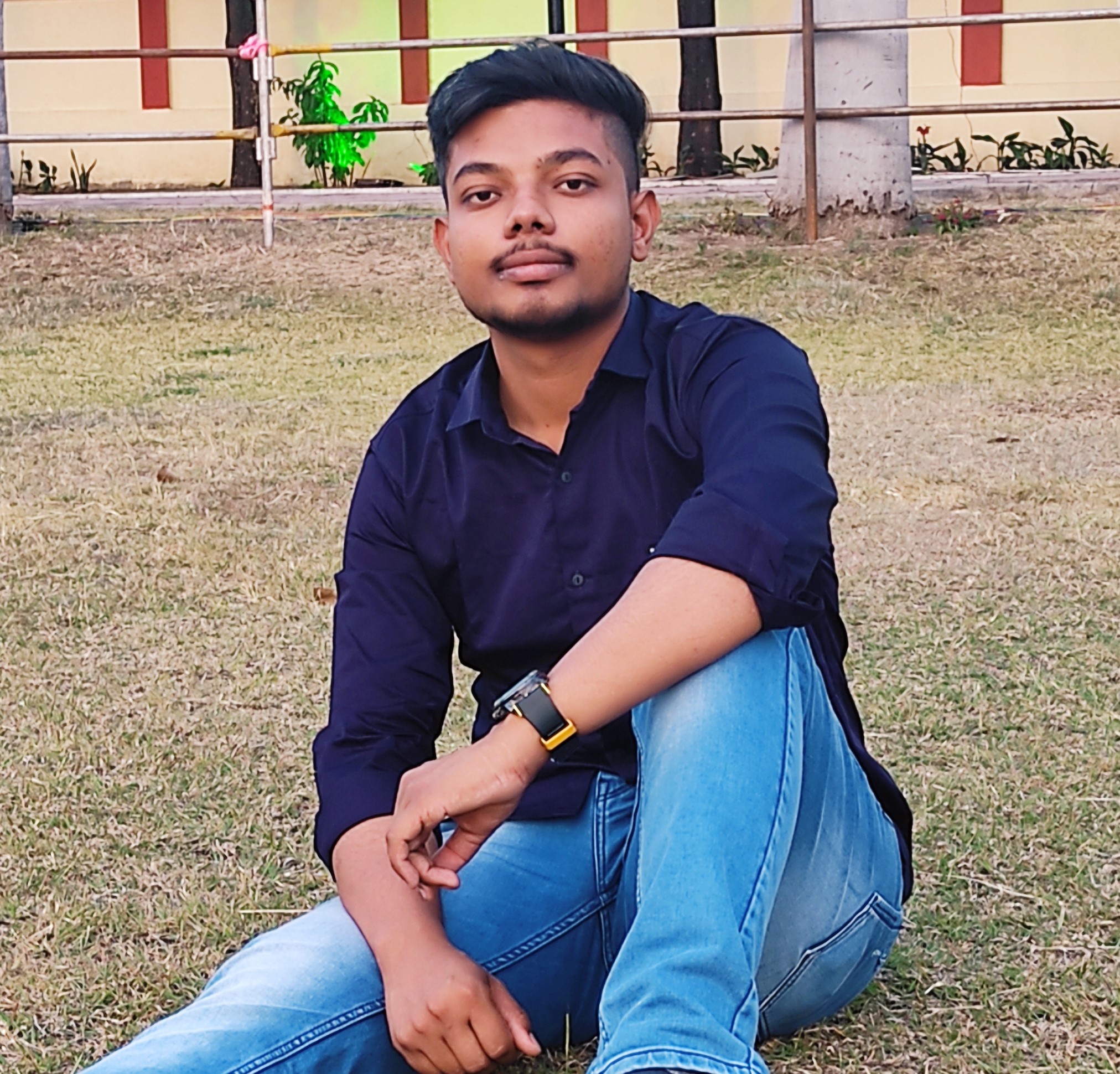
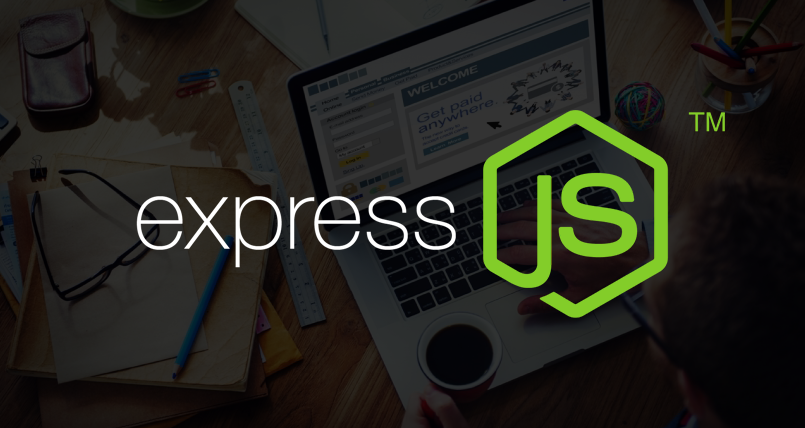
👉Introduction:
Express.js is a popular web application framework for Node.js that simplifies the development of web applications and APIs. we will explore the key features of Express.js and demonstrate how to build a web application using code examples.
🔗Getting Started with Express.js:
To begin, let's set up a basic Express.js project. Start by installing Express.js using npm and create a new project directory. Then, create a package.json
file and install Express.js as a dependency. Next, create an index.js
file and import the Express module. We can now define a simple route and start the server.
// Step 1: Install Express.js
$ npm install express
// Step 2: Create a new project directory and package.json file
// Step 3: Import Express.js in index.js
const express = require('express');
const app = express();
// Step 4: Define a route
app.get('/', (req, res) => {
res.send('Hello, Express!');
});
// Step 5: Start the server
app.listen(3000, () => {
console.log('Server started on port 3000');
});
🔗Routing in Express.js:
Routing is a fundamental aspect of web applications. Express.js provides an intuitive way to define routes for handling different HTTP methods and request paths. Let's create a more complex application with multiple routes.
app.get('/about', (req, res) => {
res.send('About page');
});
app.post('/login', (req, res) => {
// Handle login request
});
app.put('/users/:id', (req, res) => {
const userId = req.params.id;
// Update user with the given ID
});
app.delete('/users/:id', (req, res) => {
const userId = req.params.id;
// Delete user with the given ID
});
🔗Middleware in Express.js:
Express.js utilizes middleware functions to enhance the functionality of your web application. Middleware functions can perform tasks such as request parsing, authentication, logging, and error handling. Let's implement a middleware function to log requests.
const logger = (req, res, next) => {
console.log(`${req.method} ${req.url}`);
next();
};
app.use(logger);
// Example of a route with multiple middleware functions
app.get('/user/:id', authenticate, (req, res) => {
// Handle user request
});
function authenticate(req, res, next) {
// Perform authentication logic
next();
}
🔗Template Engines in Express.js:
Express.js supports various template engines like EJS, Pug, and Handlebars. Template engines allow you to dynamically generate HTML or other content based on data. Let's use the EJS template engine to render a user view.
// Set the template engine
app.set('view engine', 'ejs');
// Render a view using EJS template engine
app.get('/user/:id', (req, res) => {
const userId = req.params.id;
// Fetch user details from a database
const user = { name: 'John Doe', age: 30 };
res.render('user', { user });
});
🔗Error Handling in Express.js:
Error handling is crucial in any application. Express.js provides error-handling middleware functions to handle and respond to errors. Let's create a simple error-handling middleware function.
app.use((err, req, res, next) => {
// Handle the error
res.status(500).send('Internal Server Error');
});
✔Conclusion:
Express.js is a powerful web application framework that simplifies the development of web applications and APIs in Node.js. In this blog post, we covered the essential features of Express.js, including routing, middleware, template engines, and error handling. Armed with this knowledge and the provided code examples, you are well-equipped to start building your own web applications using Express.js. Happy coding!
Note: The code examples provided in this blog post are simplified for demonstration purposes. It's important to follow best practices and consider security measures when building real-world applications with Express.js.
🤘Feel free to leave a comment if you found this information informative and valuable. Additionally, I would greatly appreciate hearing your thoughts and feedback on the content. Thank you!💖
Subscribe to my newsletter
Read articles from Sujeet Vishvkarma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
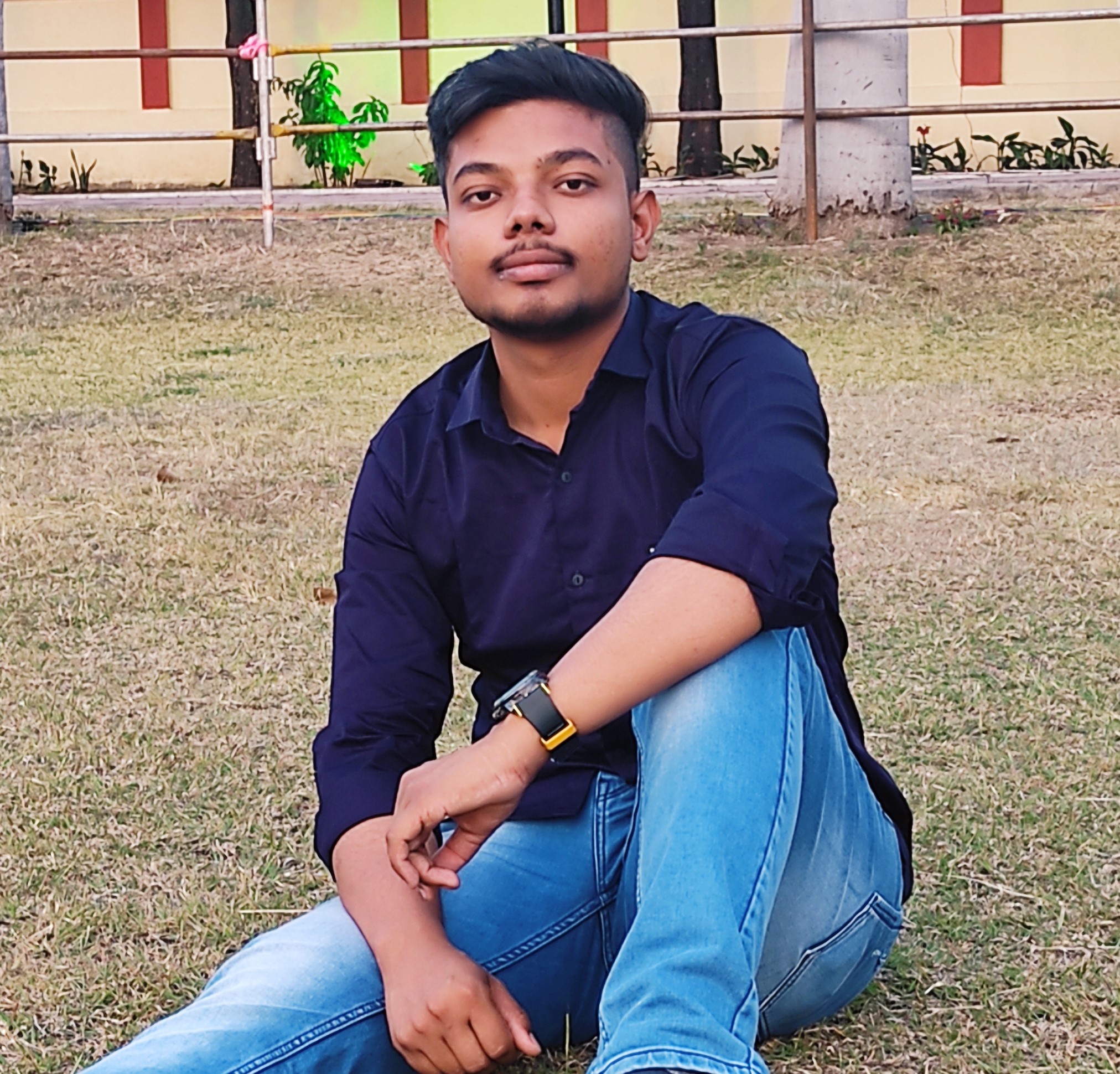
Sujeet Vishvkarma
Sujeet Vishvkarma
SIH finalist 2K22 🚀 | 💙Full Stack Developer💙,📢Learn in Public🌎,💖Open Source, Technical Writing ✍ 🟠 Hungry😋 for More📚 Knowledge 🤠