Commonly used features and commands of Firebase in Angular
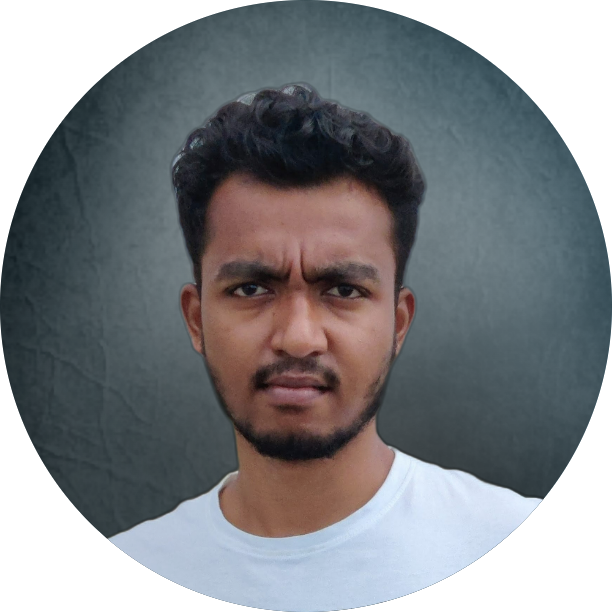
3 min read
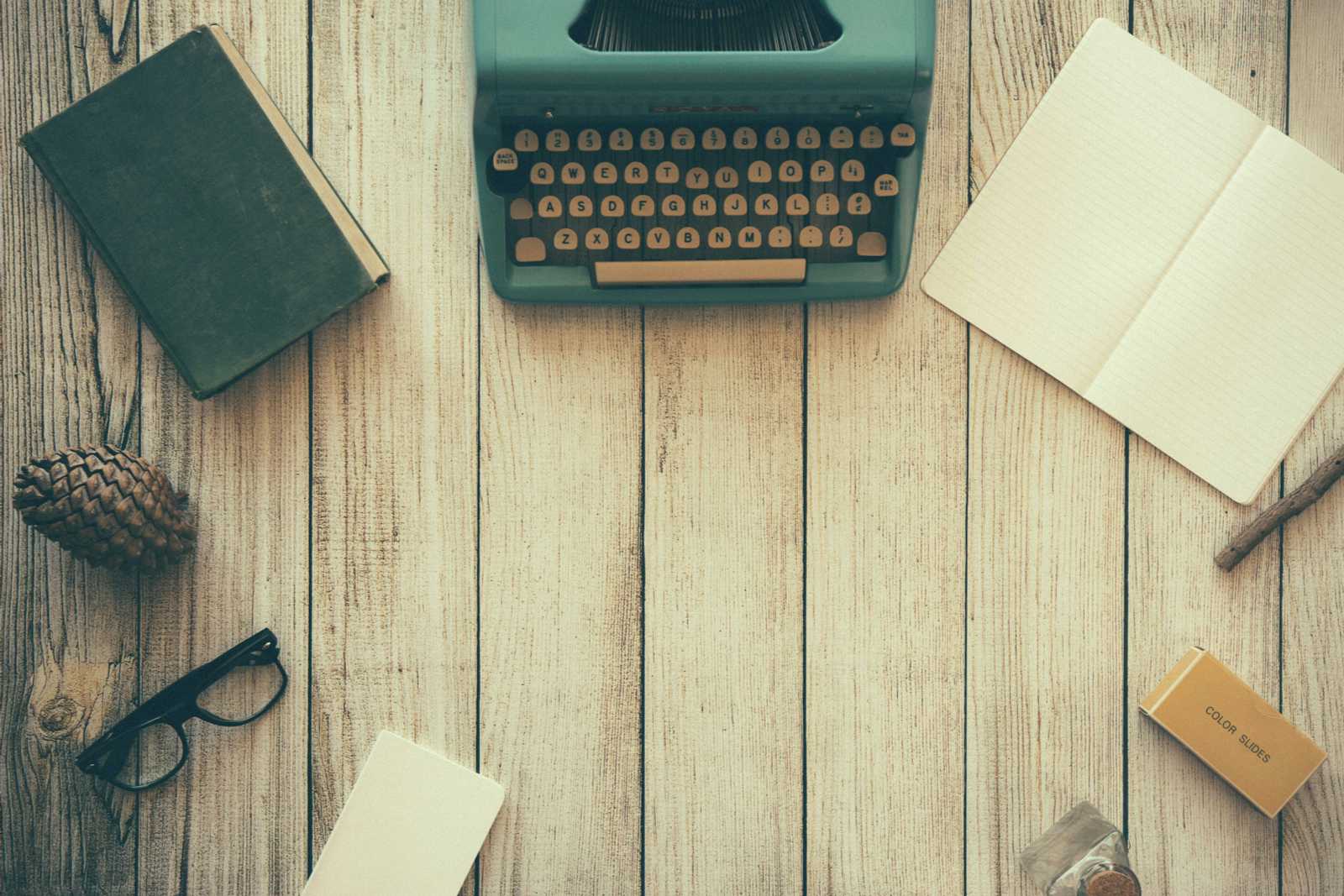
Here's a Firebase cheatsheet with commonly used features and commands in an Angular application:
Firebase Initialization in Angular
- Install Firebase packages:
npm install firebase @angular/fire
- Import Firebase and initialize it in your Angular app:
import { AngularFireModule } from '@angular/fire';
import { AngularFireAuthModule } from '@angular/fire/auth';
import { AngularFirestoreModule } from '@angular/fire/firestore';
import { AngularFireStorageModule } from '@angular/fire/storage';
const firebaseConfig = {
apiKey: 'YOUR_API_KEY',
authDomain: 'YOUR_AUTH_DOMAIN',
projectId: 'YOUR_PROJECT_ID',
storageBucket: 'YOUR_STORAGE_BUCKET',
appId: 'YOUR_APP_ID',
};
@NgModule({
imports: [
AngularFireModule.initializeApp(firebaseConfig),
AngularFireAuthModule,
AngularFirestoreModule,
AngularFireStorageModule,
// other imports
],
// other configurations
})
export class AppModule { }
Authentication in Angular
import { AngularFireAuth } from '@angular/fire/auth';
constructor(private afAuth: AngularFireAuth) {}
// Sign up with email and password
this.afAuth.createUserWithEmailAndPassword(email, password)
.then((userCredential) => {
// User creation successful
const user = userCredential.user;
})
.catch((error) => {
// An error occurred
const errorCode = error.code;
const errorMessage = error.message;
});
// Sign in with email and password
this.afAuth.signInWithEmailAndPassword(email, password)
.then((userCredential) => {
// User authentication successful
const user = userCredential.user;
})
.catch((error) => {
// An error occurred
const errorCode = error.code;
const errorMessage = error.message;
});
// Sign out
this.afAuth.signOut()
.then(() => {
// User signed out successfully
})
.catch((error) => {
// An error occurred
});
// Get the currently signed-in user
this.afAuth.user.subscribe((user) => {
if (user) {
// User is signed in
const uid = user.uid;
} else {
// User is signed out
}
});
Firestore (Database) in Angular
import { AngularFirestore } from '@angular/fire/firestore';
constructor(private firestore: AngularFirestore) {}
// Get a reference to a collection
const collectionRef = this.firestore.collection('collectionName');
// Add a document to a collection
collectionRef.add({ key: 'value' })
.then((docRef) => {
// Document added successfully
const documentId = docRef.id;
})
.catch((error) => {
// An error occurred
});
// Update a document
collectionRef.doc('documentId').update({ key: 'value' })
.then(() => {
// Document updated successfully
})
.catch((error) => {
// An error occurred
});
// Delete a document
collectionRef.doc('documentId').delete()
.then(() => {
// Document deleted successfully
})
.catch((error) => {
// An error occurred
});
// Get a document
collectionRef.doc('documentId').get()
.subscribe((doc) => {
if (doc.exists) {
// Document data exists
const documentData = doc.data();
} else {
// Document doesn't exist
}
}, (error) => {
// An error occurred
});
// Query documents in a collection
collectionRef.where('field', '==', 'value').get()
.subscribe((querySnapshot) => {
querySnapshot.forEach((doc) => {
// Access each document
const documentData = doc.data();
});
}, (error) => {
// An error occurred
});
Storage in Angular
import { AngularFireStorage } from '@angular/fire/storage';
constructor(private storage: AngularFireStorage) {}
// Get a reference to a file in storage
const fileRef = this.storage.ref('path/to/file.jpg');
// Upload a file
fileRef.put(file)
.then((snapshot) => {
// File uploaded successfully
const downloadUrl = snapshot.ref.getDownloadURL();
})
.catch((error) => {
// An error occurred
});
// Download a file
fileRef.getDownloadURL()
.subscribe((downloadUrl) => {
// File download URL obtained successfully
}, (error) => {
// An error occurred
});
// Delete a file
fileRef.delete()
.then(() => {
// File deleted successfully
})
.catch((error) => {
// An error occurred
});
This cheatsheet provides an overview of using Firebase features in an Angular application. Remember to install the necessary packages and import the required modules in your Angular app.
1
Subscribe to my newsletter
Read articles from Manthan Ankolekar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
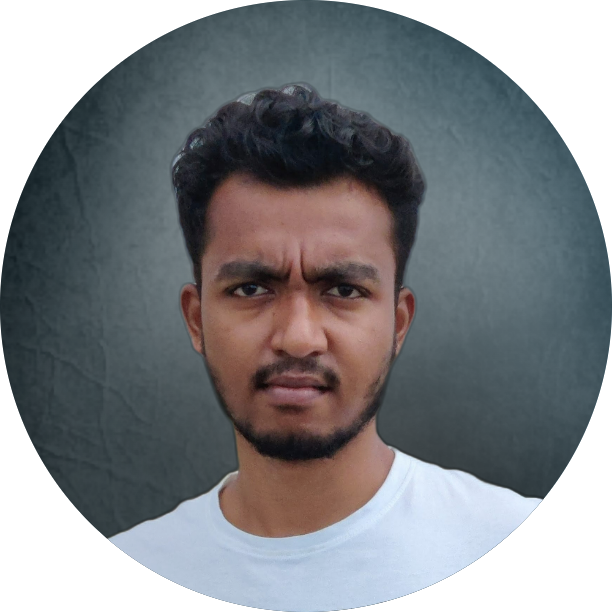
Manthan Ankolekar
Manthan Ankolekar
I am an intermediate learner, full-stack developer, and blogger.......