Simplifying 300 Python Programs on the list- Questions 61 to 70.
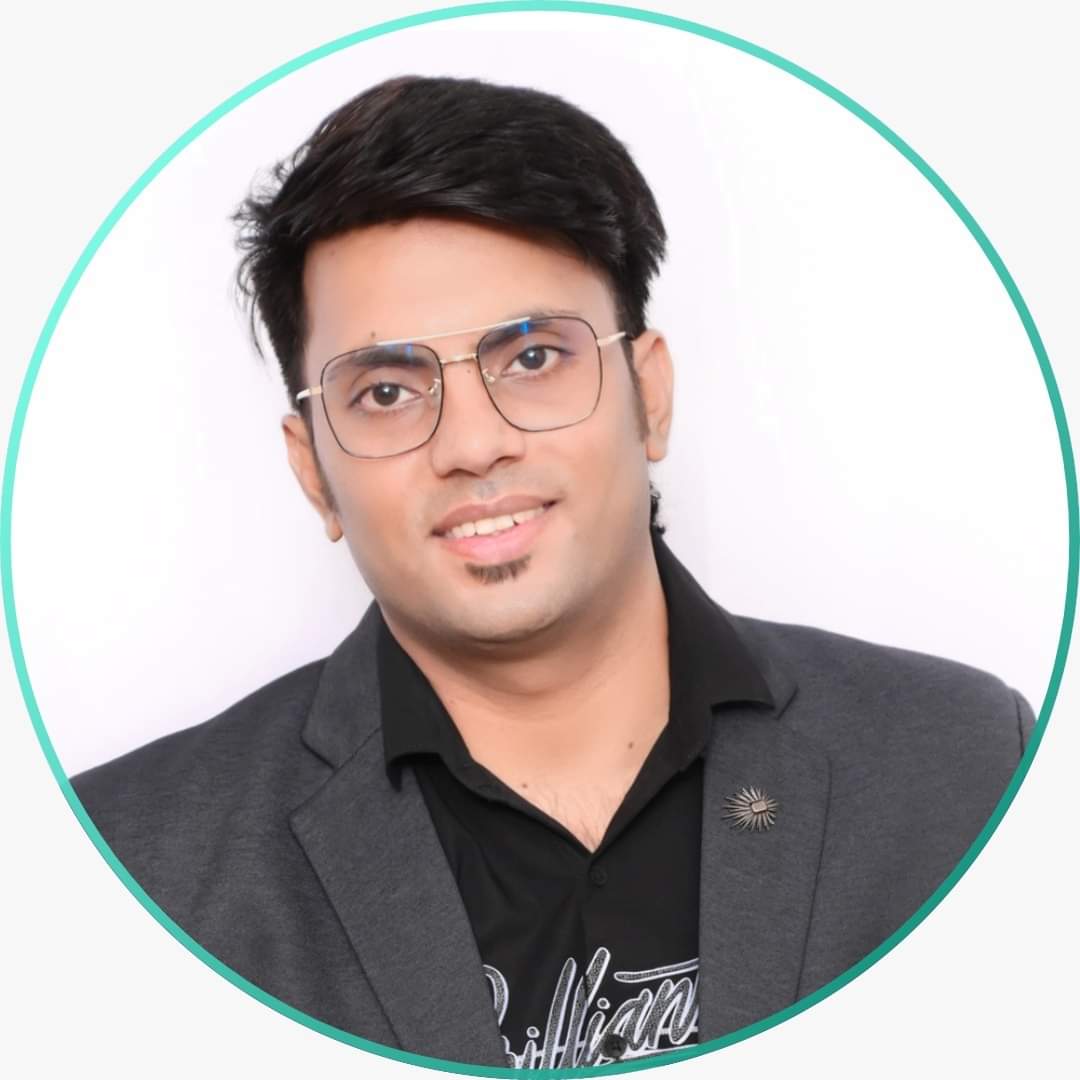
Table of contents
- Q61-Write a Python program to find all the values in a list that are greater than a specified number.
- Q62- Write a Python program to extend a list without appending.
- Q63- Write a Python program to remove duplicates from a list of lists.
- Q64- Write a Python program to find items starting with a specific character from a list.
- Q65- Write a Python program to check whether all dictionaries in a list are empty or not.
- Q66- Write a Python program to flatten a given nested list structure.
- Q67- Write a Python program to remove consecutive (following each other continuously) duplicates (elements) from a given list.
- Q68-Write a Python program to pack consecutive duplicates of a given list of elements into sublists.
- Q69- Write a Python program to split a given list into two parts where the length of the first part of the list is given.
- Q70- Write a Python program to remove the K'th element from a given list, and print the updated list.
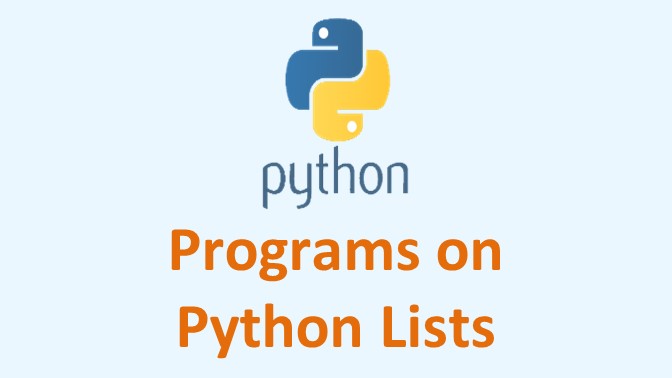
Q61-Write a Python program to find all the values in a list that are greater than a specified number.
to work on this, we need to take a number from the user and compare that number with all the numbers in the list.
n=int(input("please provide a value: "))
ls=[1,2,3,4,5,6,7,8,9,10,11,12]
new_ls=[]
for i in ls:
if i>n:
new_ls.append(i)
else:
pass
print(new_ls)
Result= [6, 7, 8, 9, 10, 11, 12]
Q62- Write a Python program to extend a list without appending.
for example, take sample data as below
ls1 = [10, 20, 30]
ls2= [40, 50, 60]
Expected output : [40, 50, 60, 10, 20, 30]
this one we have already done, we can use two methods to do this.
one is we can use extend method.
the second one is we can use directly replace the 0th index of the element with the list.
ls1=[10, 20, 30]
ls2=[40, 50, 60]
ls2.extend(ls1)
print(ls2)
Result= [40, 50, 60, 10, 20, 30]
#OR
ls1=[10, 20, 30]
ls2=[40, 50, 60]
ls1[:0]=ls2 #replace the 0th index items with the ls2.
print(ls1)
Q63- Write a Python program to remove duplicates from a list of lists.
for example, the given list of lists is:
ls=[[10, 20], [40], [30, 56, 25], [10, 20], [33], [40]]
Result shoud be= [[10, 20], [30, 56, 25], [33], [40]]
we need to remove the duplicate lists.
for this, we need to iterate a loop on the list and take an empty list.
append the items in the empty list one by one with the condition that if that item exists then do not append else append.
ls=[[10, 20], [40], [30, 56, 25], [10, 20], [33], [40]]
ls1=[]
for i in ls:
if i not in ls1:
ls1.append(i)
else:
pass
print(ls1)
Result: [[10, 20], [40], [30, 56, 25], [33]]
Q64- Write a Python program to find items starting with a specific character from a list.
Expected in this question is:
Expected Output:
Original list:
['abcd', 'abc', 'bcd', 'bkie', 'cder', 'cdsw', 'sdfsd', 'dagfa', 'acjd']
Items start with a from the said list:
['abcd', 'abc', 'acjd']
Items start with d from the said list:
['dagfa']
Items start with w from the said list:
[]
for doing that above task, we can use the start method of the string.
iterate a loop over the list every single item in the list is a string.
check if that string starts with the particular character or not.
if yes, then append that word in the empty list, else not.
ls=['abcd', 'abc', 'bcd', 'bkie', 'cder', 'cdsw', 'sdfsd', 'dagfa', 'acjd']
new_ls=[]
for i in ls:
if i.startswith('a'):
new_ls.append(i)
else:
pass
print(new_ls)
Result: ['abcd', 'abc', 'acjd']
ls=['abcd', 'abc', 'bcd', 'bkie', 'cder', 'cdsw', 'sdfsd', 'dagfa', 'acjd']
new_ls=[]
for i in ls:
if i.startswith('d'):
new_ls.append(i)
else:
pass
print(new_ls)
Result: ['dagfa']
Q65- Write a Python program to check whether all dictionaries in a list are empty or not.
Sample list : [{},{},{}]
Return value : True
Sample list : [{1,2},{},{}]
Return value : False
to do this program:
just let it be that every item in the list is an empty dictionary.
iterate a loop over the list, and check whether every dictionary is empty or not.
if not empty change the variable value to False. and break the loop immediately.
ls=[{},{},{}]
dict_empty=True
for i in ls:
if len(i)!=0:
dict_empty=False
break
print(dict_empty)
Result: True
ls=[{},{},{},{1:1}]
dict_empty=True
for i in ls:
if len(i)!=0:
dict_empty=False
break
print(dict_empty)
Result: False
OR
ls=[{},{},{}]
dict_empty=True
for i in ls:
if i:
dict_empty=False
break
print(dict_empty)
Q66- Write a Python program to flatten a given nested list structure.
Expected Results:
Original list: [0, 10, [20, 30], 40, 50, [60, 70, 80], [90, 100, 110, 120]]
Flatten list:
[0, 10, 20, 30, 40, 50, 60, 70, 80, 90, 100, 110, 120]
to do that we can iterate a loop over the list, and check if that item received from the iteration is an integer value append in the empty list directly.
else if that item is a list, iterate a loop in that list and append each item in the new list.
we can use isinstance() function directly to check whether the item is str, int, list, tuple, etc...
any object in python is also called an instance.
ls=[0, 10, [20, 30], 40, 50, [60, 70, 80], [90, 100, 110, 120]]
new_ls=[]
for i in ls:
if isinstance(i,int):
new_ls.append(i)
elif isinstance(i,list):
for j in i:
new_ls.append(j)
else:
pass
print(new_ls)
Result: [0, 10, 20, 30, 40, 50, 60, 70, 80, 90, 100, 110, 120]
Q67- Write a Python program to remove consecutive (following each other continuously) duplicates (elements) from a given list.
Expected the Result is:
[0, 0, 1, 2, 3, 4, 4, 5, 6, 6, 6, 7, 8, 9, 4, 4]
After removing consecutive duplicates:
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 4]
this one we have already covered, you can check the below blog.
Q68-Write a Python program to pack consecutive duplicates of a given list of elements into sublists.
for example:
[0, 0, 1, 2, 3, 4, 4, 5, 6, 6, 6, 7, 8, 9, 4, 4]
After packing consecutive duplicates of the said list elements into sublists:
[[0, 0], [1], [2], [3], [4, 4], [5], [6, 6, 6], [7], [8], [9], [4, 4]]
for doing this, we need to take two empty lists, ls1=[], ls2=[]
First Method:
we will iterate a loop over the given list.
check if the item received from each iteration is the last item in the ls1, if yes append that item in the ls1, also to start the program we can check if the len(ls) ==0.
in the else part, if the next item is not the last item of the ls1, then append the whole ls1 to the ls2, this is how it packs the whole list and appends to the new list.
n_list = [0, 0, 1, 2, 3, 4, 4, 5, 6, 6, 6, 7, 8, 9, 4, 4]
result = []
current_group = []
for num in n_list:
if len(current_group) == 0 or num == current_group[-1]:
current_group.append(num)
else:
result.append(current_group)
current_group = [num]
if len(current_group) > 0:
result.append(current_group)
print("Original list:")
print(n_list)
print("\nAfter packing consecutive duplicates of the said list elements into sublists:")
print(result)
Second Method: we can use group by function from itertools.
group by: we can get the key and value, for example, key =0, and its value is 0,0.
so group by forms a group like, 0:0,0, 1:1, 2:2, 3:3, 4:4,4 ...
so we get duplicates as a group and we can append them to the new list as below.
from itertools import groupby
ls = [0, 0, 1, 2, 3, 4, 4, 5, 6, 6, 6, 7, 8, 9, 4, 4]
result=[]
for key, group in groupby(ls):
new_ls=[]
for n in group:
new_ls.append(n)
result.append(new_ls)
print(result)
Result: [[0, 0], [1], [2], [3], [4, 4], [5], [6, 6, 6], [7], [8], [9], [4, 4]]
Q69- Write a Python program to split a given list into two parts where the length of the first part of the list is given.
for example:
Original list:
[1, 1, 2, 3, 4, 4, 5, 1]
Length of the first part of the list: 3
Splited the said list into two parts:
([1, 1, 2], [3, 4, 4, 5, 1])
so for doing this divide the list into two parts, where the length of the first part of the list will be given by the user.
now append both lists into the empty list.
n=int(input("please provide the lenth of the first list: "))
ls=[1, 1, 2, 3, 4, 4, 5, 1]
new_ls=[]
ls1=ls[0:n]
ls2=ls[n:]
new_ls.append(ls1)
new_ls.append(ls2)
print(new_ls)
Result:
please provide the lenth of the first list: 3
[[1, 1, 2], [3, 4, 4, 5, 1]]
Q70- Write a Python program to remove the K'th element from a given list, and print the updated list.
for example:
Original list:
[1, 1, 2, 3, 4, 4, 5, 1]
After removing an element at the 2nd position of the said list:
[1, 1, 3, 4, 4, 5, 1]
we will ask index from the user of that element.
then we will check the element on that index number.
remove the element using the remove method of the list.
ls=[1, 1, 2, 3, 4, 4, 5, 1]
n=int(input("please provide the index of the element to be removed from the list: "))
element=ls[n]
ls.remove(element)
print(ls)
Result:
please provide the index of the element to be removed from the list: 3
[1, 1, 2, 4, 4, 5, 1]
Subscribe to my newsletter
Read articles from Kapil Manwani directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
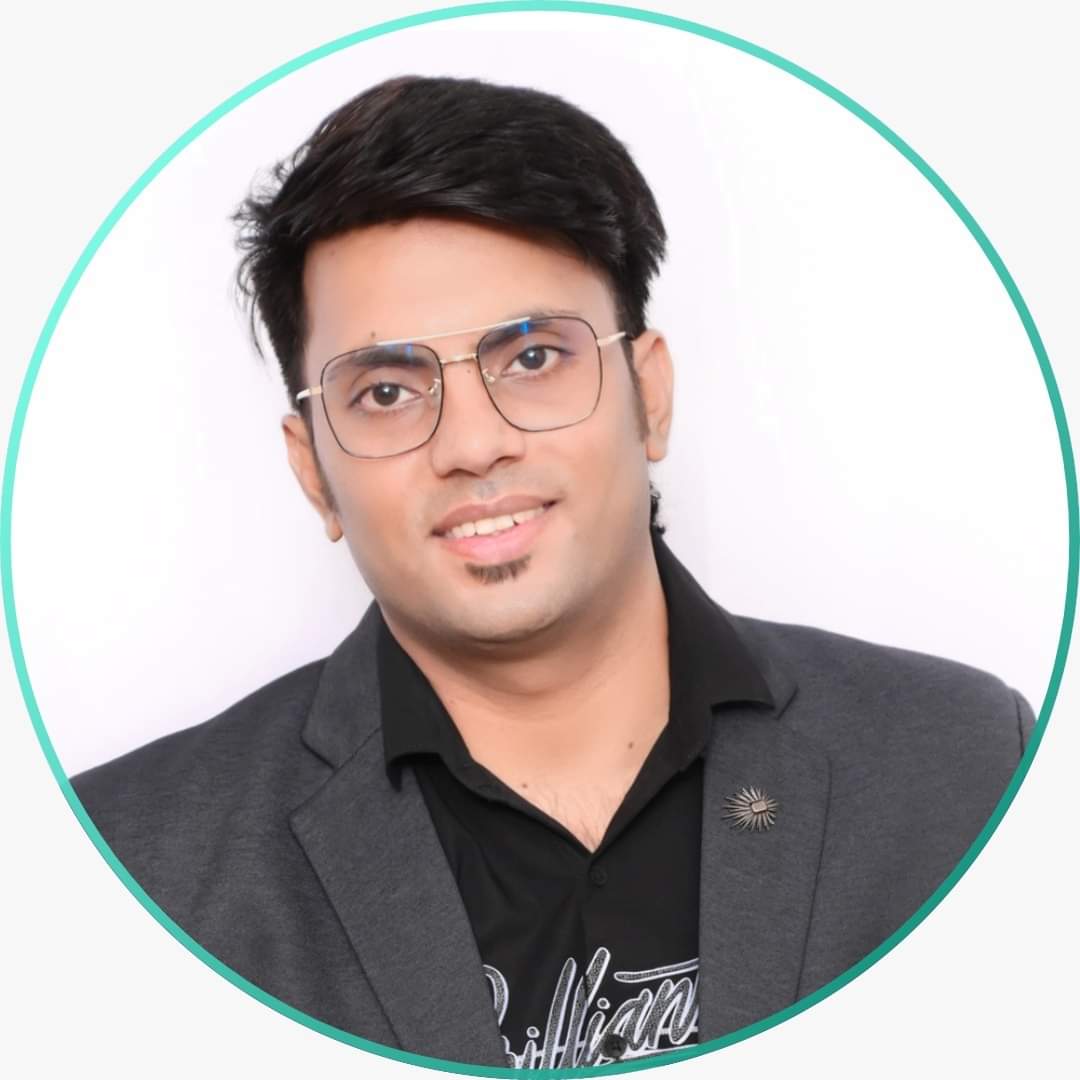
Kapil Manwani
Kapil Manwani
Proficient in a variety of DevOps technologies, including AWS, Linux, Python, Shell Scripting, Docker, Terraform, and Computer Networking. I have a strong ability to troubleshoot issues.