2D Array (Matrix)
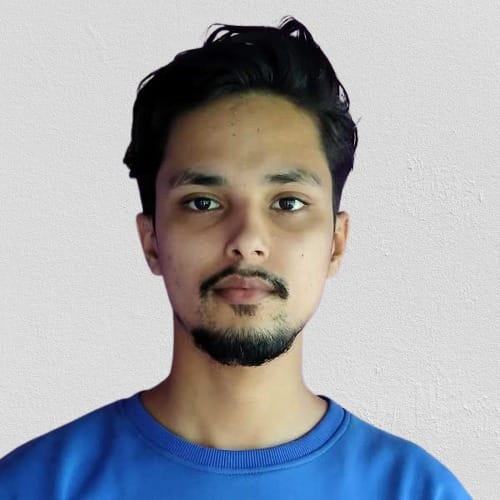

2D arrays, also called matrices, are an essential data structure in programming for storing and manipulating multi-dimensional data. Unlike 1D arrays that hold elements in a linear sequence, 2D arrays organize data in a grid-like structure with rows and columns. In this article, we will explore the concept of 2D arrays, covering their definition, declaration, initialization, accessing elements, and investigating the various operations that can be performed on them.
Declaration of 2D Array.
The declaration of the 2D array is different in each language.
For c++
// Syntax: data_type array_name[row_size][column_size];
int myArray[3][4]; // Declares a 2D array with 3 rows and 4 columns
For java
// Syntax: data_type[][] array_name = new data_type[row_size][column_size];
int[][] myArray = new int[3][4]; // Declares a 2D array with 3 rows and 4 columns
For Python
myArray = [
[0, 1, 2, 3],
[4, 5, 6, 7],
[8, 9, 10, 11]
]
For Javascript
let myArray = [
[0, 1, 2, 3],
[4, 5, 6, 7],
[8, 9, 10, 11]
];
For Ruby
myArray = [
[element1, element2, element3],
[element4, element5, element6],
[element7, element8, element9]
]
Why 2D Array?
2D array is important due to the following reason.
Tabular Data: 2D arrays can represent rows and columns of tabular data, making them suitable for storing and manipulating datasets or tables.
Matrices and Linear Algebra: 2D arrays are crucial for mathematical operations involving matrices, such as computer graphics, machine learning, and solving systems of linear equations.\
Game Development: 2D arrays are helpful for representing grid-based game worlds, tracking object positions, and managing interactions and collisions.
Representing Grids or Matrices: 2D arrays are ideal for organizing data in a grid-like structure, commonly used for game boards, mazes, spreadsheets, or images.
Image Processing: Images can be represented as 2D arrays of pixel values, enabling various image manipulation operations.
We will be solving the question in 2d array that link will be provided later.
Subscribe to my newsletter
Read articles from Roshan Guragain directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
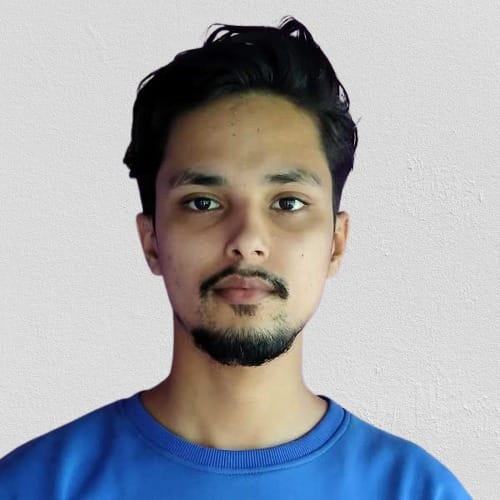
Roshan Guragain
Roshan Guragain
I am a Software developer who is passionate about making web app using the technologies like Html, Css, Javascript, React, Mongodb, Mongoose, Express, Node.