Today I learnt: Lists and Loops in Python
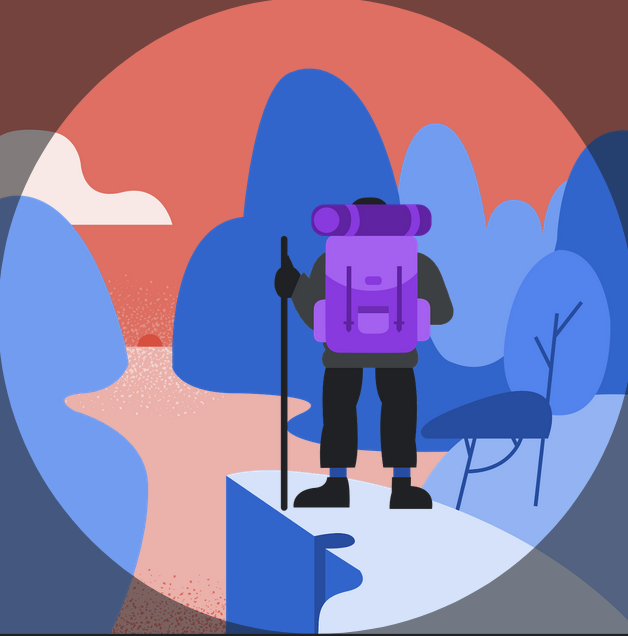
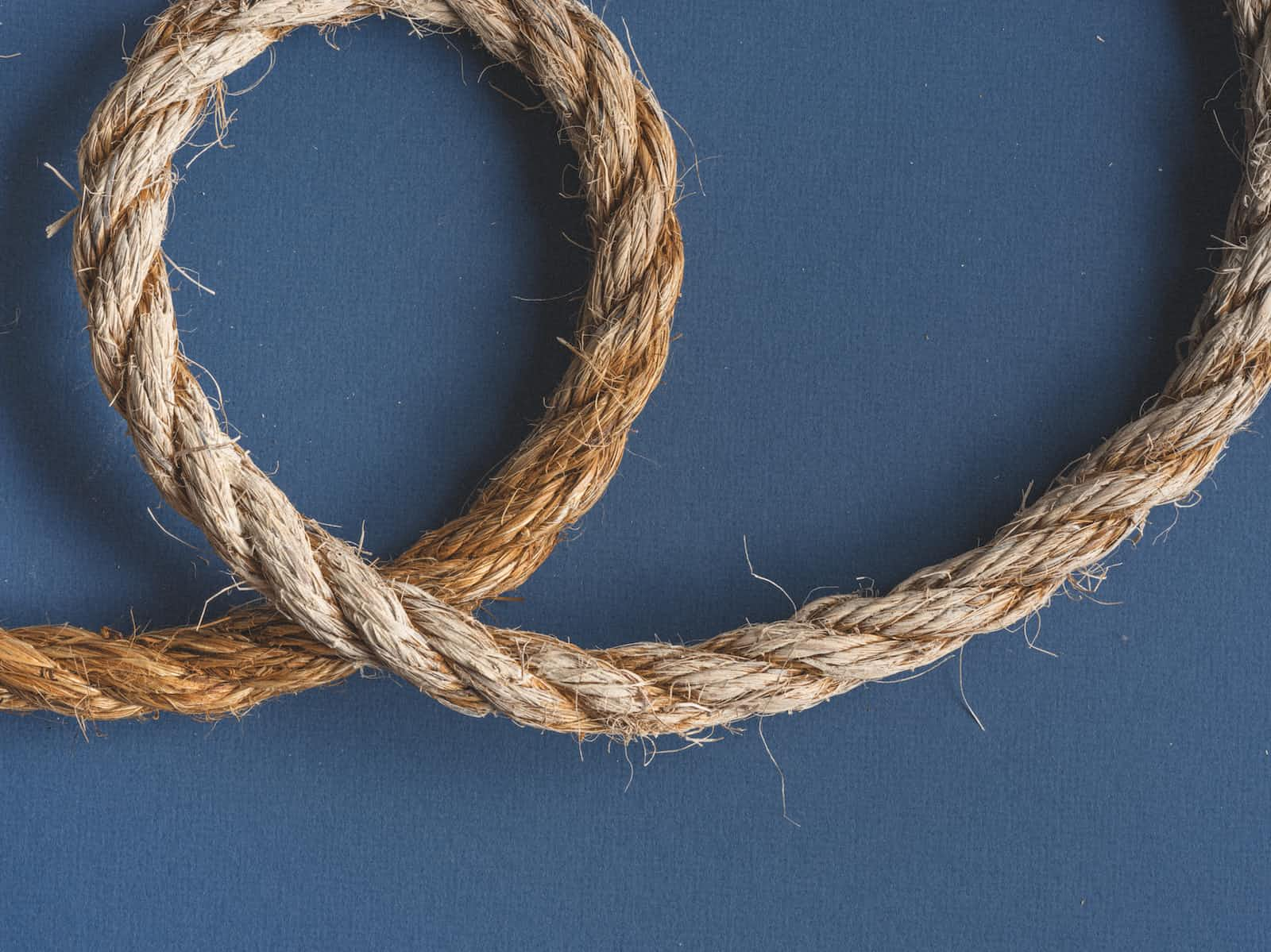
Hello reader! I am an aspiring data scientist and this is my first post on hashnode. The intention behind this blog is to document my learning journey as I learn data science from scratch without a background in coding. These posts will be a way for me to reflect on whatever I am learning and share my progress with others. If you are also just starting out in data science, hop along and let's get started!
Lists
Lists are a form of data structure. Data structures are ways of holding data. If we think of a piece of data as a file, data structures are the file cabinet. Their purpose is to hold files. Similarly, lists hold several pieces of data.
list_a = [a,b,c,d]
# This list holds the four letters a,b,c and d.
The elements of a list are identified through their index. The first item has the index of "0". The second item has an index of "1" and so on. Lists also have a negative index. Index of "-1" denotes the last item of the list, "-2" denotes the second last item of the list and so on.
list_a = [a,b,c,d]
variable_a = list_a[2]
# This assigns the third element of the list to variable_a (letter c).
Loops
The way I understand is that programming consists of two: tasks that we tell the computer to perform and order the tasks that we tell it to follow. Using a function like "print()" tells the computer to perform a certain task. With control flow statements, we tell the computer what order to follow when performing such tasks.
There are two main types of control flow statements: loops and conditional statements. Loops are a form of control flow statements that are used to tell the computer to repeat a certain task a certain number of times.
Now, we can either specify the number of times we want the computer to perform the task, or we can tell it to perform it over each item of a collection of data (such as a list). We use "For loops" for these cases.
list_a = [1,2,3,4]
for item in list_a:
print(item)
# This will print all the items in the list one by one
We can also tell the computer to keep repeating the task till the point a certain condition is met. We use "While" loops for such cases.
list_a = [1,2,3,4]
i = 0
while i < len(list_a):
print(list_a[i])
i += 1
# This will do the same thing, this time using the While loop.
# len(list_a) is a function which will return the length of list_a.
# list_a[i] denotes the items of the list using their index.
Loops are one of two main types of control flow statements in Python, the other being conditional statements (e.g. if, else), which I will explore in another article.
Subscribe to my newsletter
Read articles from Sourabh Bhati directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
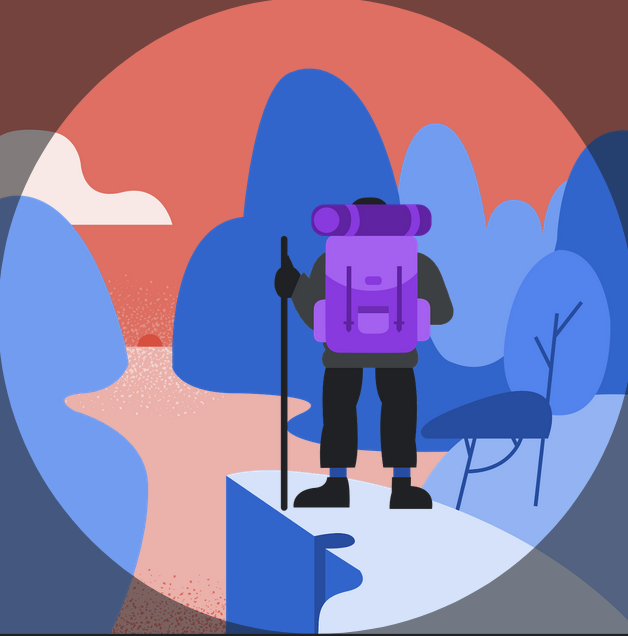