Map, Filter, Reduce functions in JavaScript
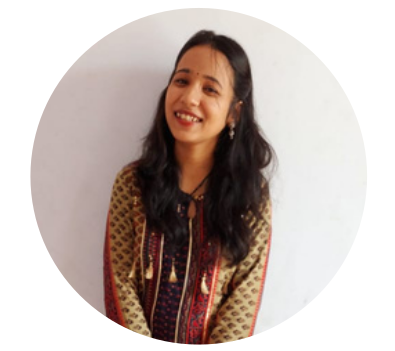
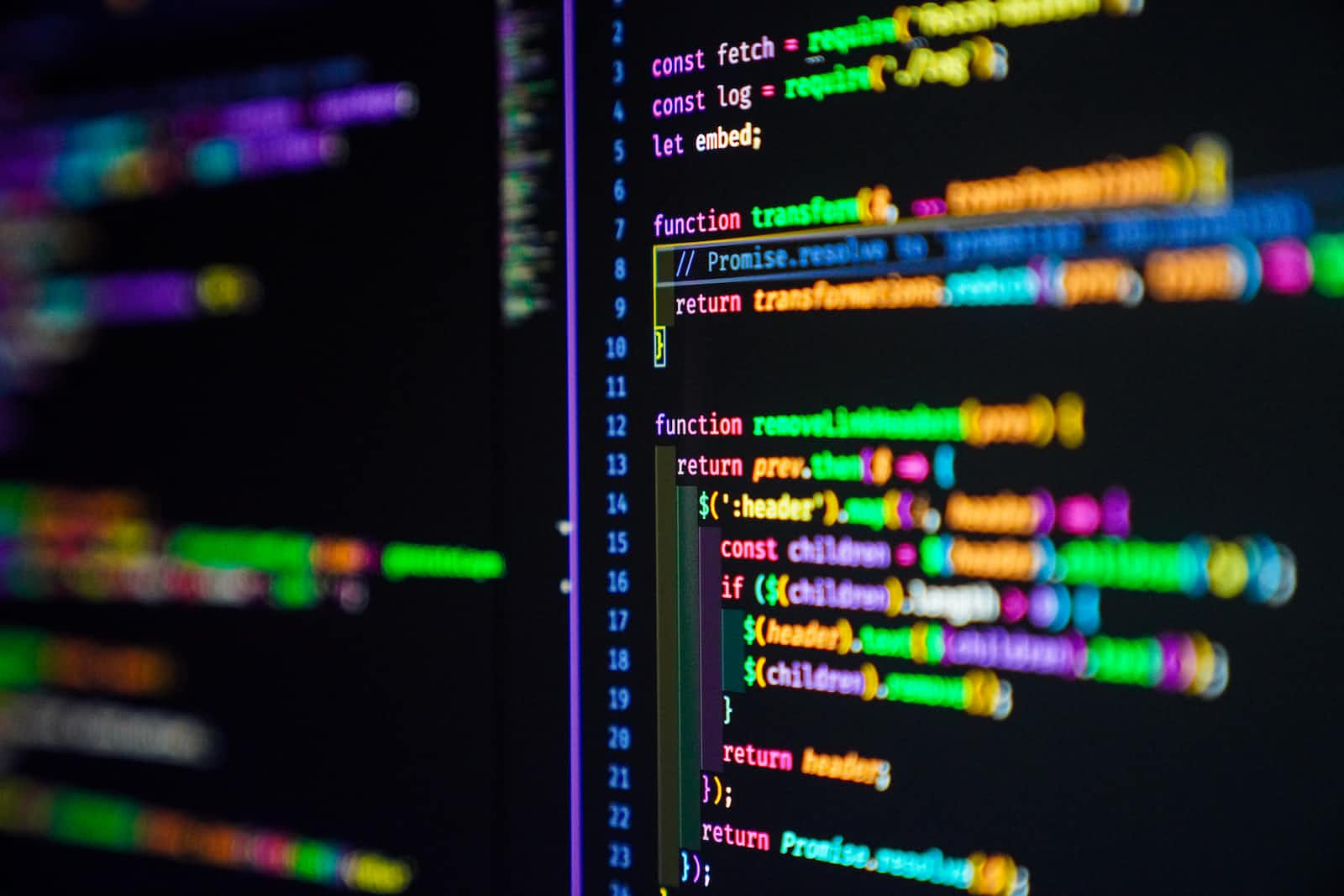
Overview:
JavaScript is a powerful programming language that provides developers with many built-in functions that can help the developer to manipulate data in many different ways. Three of these functions are map()
, filter()
, and reduce()
. In this blog, we will explore what these functions are, and how to use them to transform and manipulate data.
Map Function:
The map
function is used to transform each element of an array into a new value based on a given function. The original array is not modified, instead, a new array is created with the transformed values. The new array will have the same length as the original array. It allows us to iterate over an array and modify each element in it, returning a new array with the modified elements.
Syntax :
// Arrow function
array.map((element) => { /* … */ })
array.map((element, index) => { /* … */ })
array.map((element, index, array) => { /* … */ })
// Callback function
array.map(callbackFn)
array.map(callbackFn, thisValue)
The parameters of map
function are:
callbackFn
: A function for each element in the array. Its return value is added as a single element in the new array.element
: The current element being processed in the array.index
: The index of the current element.array
: The array map was called upon.thisValue
: The value to be passed as this when executing the function.
Return value:
A new array with each element being the result of the callback function.
Example:
Let's say, we have an array of numbers and we want to double each number in the array.
// Arrow Function :
const numbers = [1,2,3,4,5];
const doubled = numbers.map((num) => num * 2);
console.log(doubled);
// output: [2,4,6,8,10]
console.log(numbers);
// output: [1,2,3,4,5]
// Callback function:
const numbers = [1,2,3,4,5];
const doubleTheValue = num => num * 2;
const doubled = numbers.map(doubleTheValue);
console.log(doubled);
// output: [2,4,6,8,10]
console.log(numbers);
//output: [1,2,3,4,5]
The above code takes an array of numbers and creates a new array containing the double value of the numbers in the first array.
Filter Function:
The filter
function is used to create a new array that contains only elements that pass a certain condition. The function takes in an argument that represents the current element being processed and returns a boolean
value. If the value is true
, the element will be included in the new array. If the value is false
, the element will be excluded from the new array.
Syntax:
// Arrow function
array.filter((element) => { /* … */ })
array.filter((element, index) => { /* … */ })
array.filter((element, index, array) => { /* … */ })
// Callback function
array.filter(callbackFn)
array.filter(callbackFn, thisValue)
The parameters of filter
function are:
callbackFn
: A function for each element in the array. Its return value is added as a single element in the new array.element
: The current element being processed in the array.index
: The index of the current element.array
: The array map was called upon.thisValue
: The value to be passed as this when executing the function.
Return value:
A copy of a portion of the given array, filtered down to just the elements from the given array that pass the test implemented by the provided function. If no elements pass the test, an empty array will be returned.
Example:
We have an array of strings and we want to create a new array that contains only those strings having a length greater than 8.
//Arrow function
const words = ["freecodecamp", "hackerrank", "exercism", "neogCamp"];
const newWords = words.filter((word) => word.length > 8);
console.log(newWords);
//output: ["freecodecamp", "hackerrank"]
const words = ["freecodecamp", "hackerrank", "exercism", "neogCamp"];
const newWords = words.filter((word) => word.length > 12);
console.log(newWords);
//output: [];
//Callback function
const words = ["freecodecamp", "hackerrank", "exercism", "neogCamp"];
const wordsHavingLengthGreaterThanEight = word => word.length > 8;
const newWords = words.filter(wordsHAvingLengthGreaterThanEight);
console.log(newWords);
//output: ["freecodecamp", "hackerrank"]
Another example, let's say we have an array of numbers and we want to create an array that contains only even numbers.
const numbers = [2,3,4,5,6];
const evenNumberArr = numbers.filter((num) => num % 2 === 0);
console.log(evenNumberArr);
//output: [2,4,6];
Reduce Function:
The reduce
function is used to aggregate elements of an array into a single value based on a given function. It reduces an array to a single value. The function takes two arguments: an accumulator
and the currentValue
being processed. The accumulator
is a value that is passed from one iteration to the next and accumulates the result of the previous iterations. The currentValue
is the current value being processed.
Syntax:
array.reduce((accumulator, currentValue, currentIndex, array) => { /* … */ }, initialValue)
The parameters of reduce
function are:
accumulator
: The returned value of the previous iteration. On first call,initialValue
if specified, otherwise the value ofarray[0]
.currentValue
: The value of the current element. On first call, the value ofarray[0]
if aninitialValue
was specified, otherwise the value ofarray[1]
.currentIndex
: The index position ofcurrentValue
in the array. On first call,0
ifinitialValue
was specified, otherwise1
.array
: The original array on which reduce was called.initialValue
: The initial value of the accumulator.
Return value:
The value that results from running the "reducer" callback function to completion over the entire array.
If
initialValue
is specified,callbackFn
starts executing with the first value in the array ascurrentValue
. IfinitialValue
is not specified,accumulator
is initialized to the first value in the array, andcallbackFn
starts executing with the second value in the array ascurrentValue
.
Example:
Here's an example of using the reduce function to calculate the sum of an array of numbers.
const numbers = [1,2,3,4,5]
const sumOfArr = numbers.reduce((accumulator, currentvalue) => accumulator + currentValue, 0);
console.log(sumOfArr);
//output: 15
Conclusion:
In this blog post, we discussed the map
, filter
and reduce
functions in javascript. These functions are powerful tools that allow developers to manipulate data in many different ways. The map
function is used to transform each element in an array, the filter
function is used to create a new array that contains only elements that pass a certain condition, and the reduce
function is used to reduce an array to a single value.
Subscribe to my newsletter
Read articles from sweta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
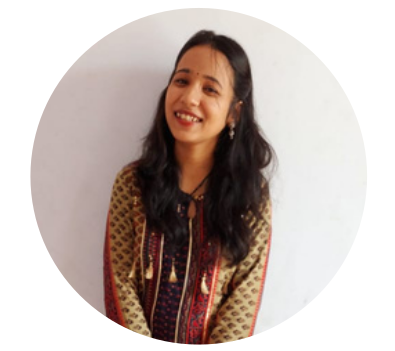