Automating My Workflow: Streamlining Task Management with Linear and TickTick
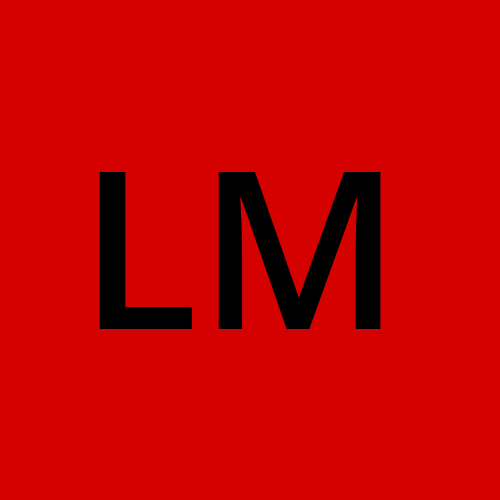
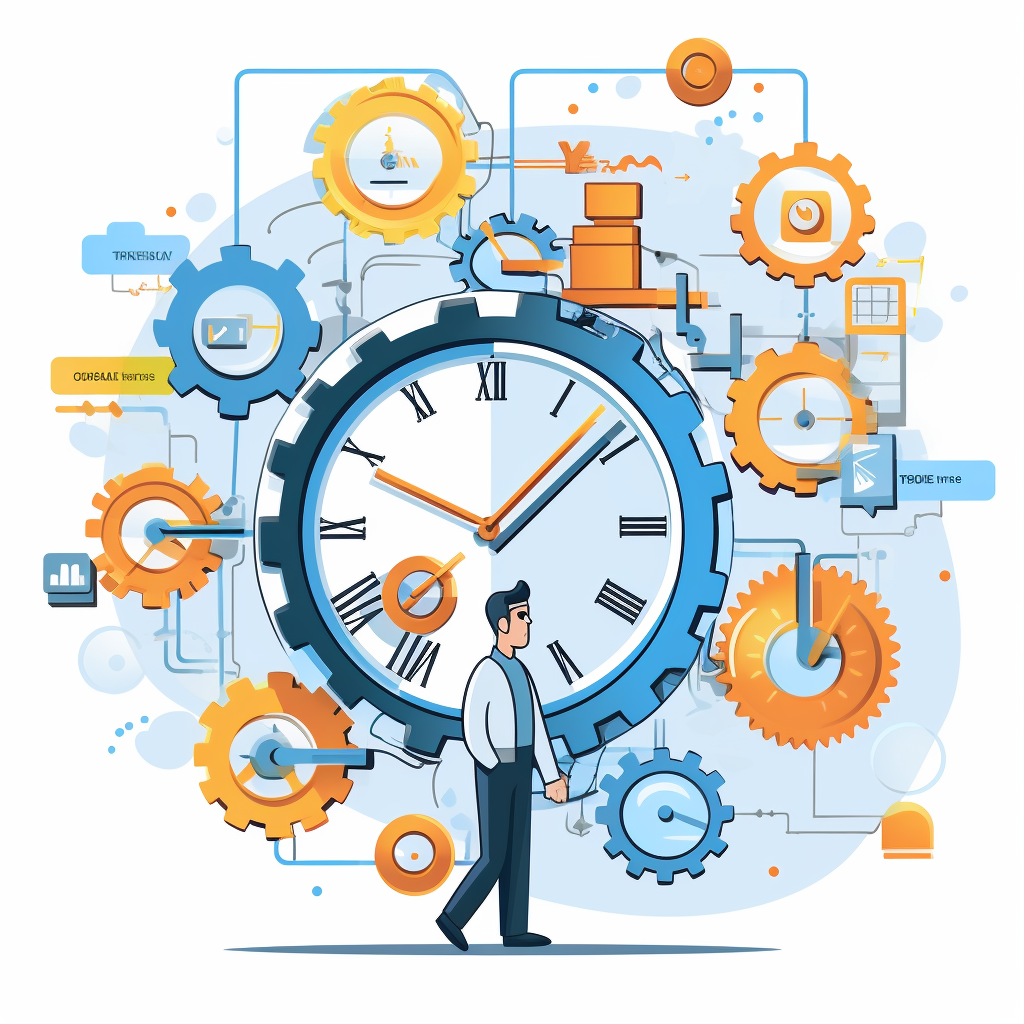
Have you ever found yourself overwhelmed by tasks and struggled to manage your time during crunch periods, or even just on a regular workday? If you're nodding in agreement right now, you're not alone. I've grappled with the same issue, hopping from one 'solution' to another, seeking a productivity system that actually works. If you are interested in software solutions like I am, I'm sure you've had a similar experience at some point.
During a high-pressure period at Juicebox, I found myself confronted with a mountain of tasks that needed to be handled swiftly to roll out our new project page. The sheer volume was daunting.
I have experimented with a multitude of methods, most of which eventually fell apart due to being too burdensome or just plain annoying to maintain. That was until I stumbled upon time blocking in Cal Newport's book 'Deep Work'.
Normally, I would take work assigned to me in Linear, open up TickTick, create a new task, assign it to a schedule, and so on. But when you're faced with 40+ issues to re-create in Linear, this process quickly turns into an arduous chore.
I decided to automate the process. Instead of manually creating tasks in TickTick from Linear, I set up a service that automatically creates a new task in TickTick whenever a new task is assigned to me in Linear. This simple automation step was a game-changer!
Prerequisites
Before we dive in, let's go over the prerequisites you'll need:
An account with Pipedream (This is the one I used, but feel free to experiment with other similar services and let me know how they work for you!)
Access to your Linear API Settings
An account with TickTick
A basic understanding of JavaScript, while not necessary, could be beneficial.
Once you have these set, we're good to go!
The Process
Firstly, set up a new workflow in Pipedream by clicking 'New +' to generate a new Workflow.
To connect Linear, search for 'Linear' and select 'New Updated Issue (Instant)'.
You'll be presented with a few options:
First, enter your Linear API key.
Second, select the Team ID that you want to use for generating the issues.
Optional: You can also choose a project filter to only create issues from a specific project.
Once the Trigger is configured, you'll have the option to select an event. This isn't crucial; it's more for testing the filters we'll be applying next. I recommend choosing an event that involves an issue being assigned to you.
Now it's time to add a NodeJS step. Select 'Node' and then 'Run Node code'. This will generate a code template.
The code we're going to write will check if the event we're monitoring is relevant (i.e., is it assigned to you?), and format the output data correctly if it is. Here's what the completed code block looks like:
export default defineComponent({
async run({ steps, $ }) {
// This function determines the TickTick priority
// from the Linear Priority.
const determinePriority = (prior) => {
// Linear Priority
// - 0 = No priority, 1 = Urgent, 2 = High, 3 = Normal, 4 = Low
//
// TickTick Priority
// - 0 = No priority, 5 = High, 3 = Medium, 1 = Low
switch (prior) {
case 1:
case 2:
return 5
case 3:
return 3
case 4:
return 1
default:
return 0
}
};
const previousAssigneeId = steps.trigger.event.updatedFrom.assigneeId;
const assigneeId = steps.trigger.event.data.assigneeId;
const userId = '<YOUR_ASSIGNEE_ID>';
if (previousAssigneeId === undefined) {
$.flow.exit("Event is not assigning a user")
}
if (assigneeId === userId) {
return {
id: steps.trigger.event.data.number,
issueUrl: steps.trigger.event.url,
title: steps.trigger.event.data.title,
description: steps.trigger.event.data.description,
priority: determinePriority(steps.trigger.event.data.priority)
};
} else {
$.flow.exit("Event data is not assigned to user");
}
},
});
You'll need to replace <YOUR_ASSIGNEE_ID>
with your Linear user id, which you can easily find using a Linear issue assigned to you in the Pipedream event explorer.
After you've tested and verified that this step works, we can move on to the next step.
For this next step, search for TickTick and select 'Use any TickTick API in Node.js'. Follow the instructions to connect to a new account. Once done, it's time to add the following code:
import { axios } from "@pipedream/platform"
export default defineComponent({
props: {
ticktick: {
type: "app",
app: "ticktick",
}
},
async run({steps, $}) {
const title = `<PROJECT_TAG>-${steps.code.$return_value.id}: ${steps.code.$return_value.title}`
const content = `${steps.code.$return_value.issueUrl}\n${steps.code.$return_value.description ?? ''}`
const priority = steps.code.$return_value.priority
const data = {
title,
content,
priority,
// projectId: '<YOUR_PROJECT_ID>',
tags: ["๐ค"]
}
return await axios($, {
method: "post",
url: `https://api.ticktick.com/open/v1/task`,
headers: {
Authorization: `Bearer ${this.ticktick.$auth.oauth_access_token}`,
},
data,
})
},
})
Note the <PROJECT_TAG>
placeholder in the const title = ...
line; replace it with your project tag. For instance, at Juicebox, we use JB
, so my line reads const title = 'JB-...'
You'll also see a commented line, projectId: <YOUR_PROJECT_ID>
. This is for specifying a TickTick project for task organization. It's slightly more complex to set up, but I'll cover it in a future blog post. Meanwhile, if you're eager to explore it now, feel free to check out my GitHub Repo for clues. Without a projectId
, all issues will be sent to your Inbox by default.
When you run a successful test, you should see a task created directly in TickTick.
And That's It!
Now all updated issues assigned to you will be automatically created in TickTick, making it easier for you to manage your time. This is my first-ever blog post, so if you have any questions or feedback, please feel free to leave a comment below or connect with me on Twitter at @lachmcculloch. I look forward to hearing from you!
Subscribe to my newsletter
Read articles from Lachlan McCulloch directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
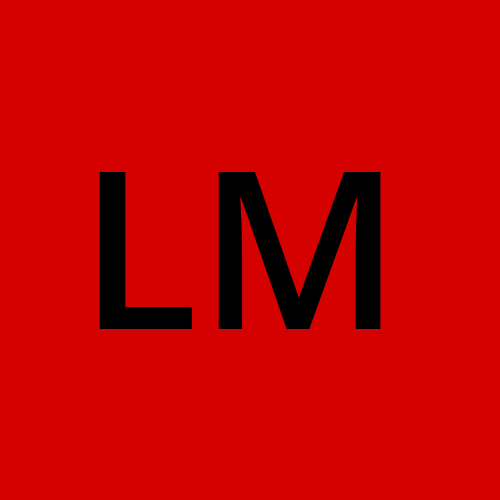