Mastering JavaScript Array Methods: A Comprehensive Guide
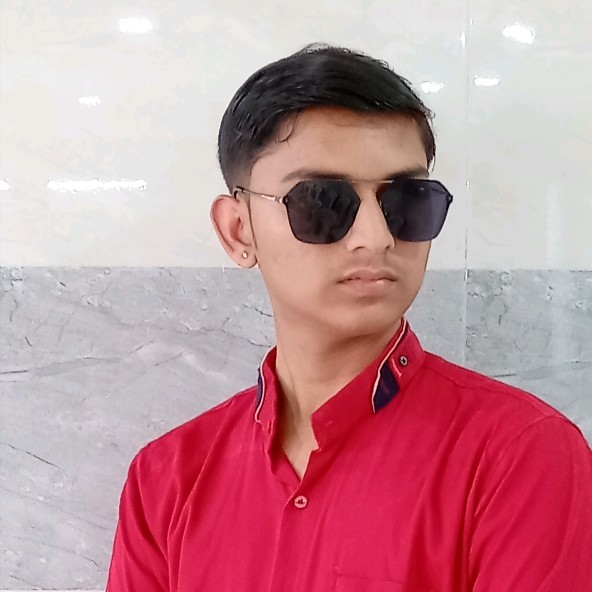
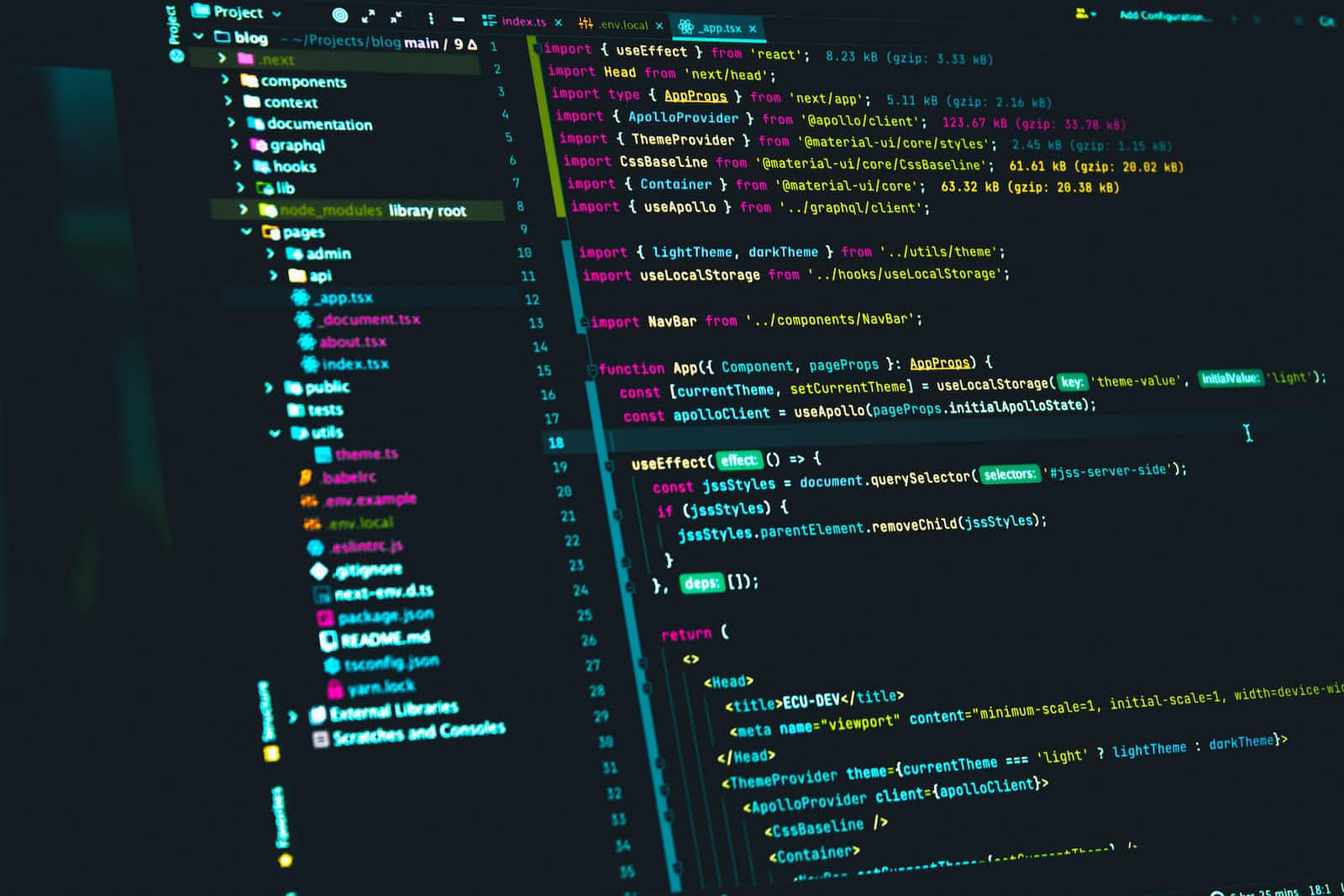
Introduction: Arrays are an essential part of JavaScript programming, and being proficient in working with arrays can greatly enhance your coding skills. In this blog post, we will dive into the world of JavaScript array methods. We will explore the most commonly used array methods and provide detailed explanations and examples for each method. By the end of this guide, you will have a solid understanding of how to manipulate, modify, and iterate over arrays with ease.
Sure! Here's a list of commonly used array methods along with their corresponding short forms to help you remember them:
push() - Add elements to the end of the array.
pop() - Remove the last element from the array.
concat() - Merge two or more arrays.
join() - Join array elements into a string.
slice() - Extract a section of the array.
splice() - Change array contents by adding, removing, or replacing elements.
shift() - Remove the first element from the array.
unshift() - Add elements to the beginning of the array.
reverse() - Reverse the order of the array elements.
sort() - Sort the elements of the array.
map() - Create a new array by performing a function on each element.
filter() - Create a new array with elements that pass a certain condition.
forEach() - Execute a function on each array element.
Table of Contents:
Manipulating Arrays:
- 1.1. push()
push(): The push()
method adds one or more elements to the end of an array and returns the new length of the array. Imagine we have an array of icons representing fruits, and we want to add a new fruit to the array:
const icons = ['๐', '๐'];
icons.push('๐');
console.log(icons); // Output: ['๐', '๐', '๐']
1.2. pop()
pop(): The
pop()
method removes the last element from an array and returns that element. Let's say we want to remove the last fruit icon from the array:
const icons = ['๐', '๐', '๐'];
const removedIcon = icons.pop();
console.log(removedIcon); // Output: '๐'
console.log(icons); // Output: ['๐', '๐']
- 1.3. concat()
concat(): The concat()
method is used to merge two or more arrays and returns a new array. Suppose we have two arrays of fruit and vegetable icons, and we want to combine them into a single array:
const fruitIcons = ['๐', '๐'];
const vegetableIcons = ['๐ฅ', '๐ฅฆ'];
const combinedIcons = fruitIcons.concat(vegetableIcons);
console.log(combinedIcons); // Output: ['๐', '๐', '๐ฅ', '๐ฅฆ']
- 1.4. join()
Join(): The join()
method joins all elements of an array into a string, using a specified separator. Let's say we have an array of icons representing different weather conditions, and we want to create a string with the icons separated by a comma:
const weatherIcons = ['โ๏ธ', '๐ง๏ธ', 'โ
', '๐ฆ๏ธ'];
const joinedString = weatherIcons.join(', ');
console.log(joinedString); // Output: 'โ๏ธ, ๐ง๏ธ, โ
, ๐ฆ๏ธ'
- 1.5. slice()
slice(): The slice()
method extracts a section of an array and returns a new array without modifying the original array. Suppose we have an array of icons representing various activities, and we want to extract a subset of icons:
const activityIcons = ['โฝ', '๐ฎ', '๐', '๐จ', '๐ด'];
const slicedArray = activityIcons.slice(1, 4);
console.log(slicedArray); // Output: ['๐ฎ', '๐', '๐จ']
1.6. splice()
splice(): The
splice()
method changes the contents of an array by removing, replacing, or adding elements in place. Let's say we have an array of icons representing fruits, and we want to replace a specific fruit with a new one:const fruitIcons = ['๐', '๐', '๐', '๐ฅญ']; const removedIcons = fruitIcons.splice(2, 1, '๐', '๐ฅฅ'); console.log(removedIcons); // Output: ['๐'] console.log(fruitIcons); // Output: ['๐', '๐', '๐', '๐ฅฅ', '๐ฅญ']
Modifying Arrays:
2.1. shift()
shift(): The
shift()
method removes the first element from an array and shifts all other elements down by one position. Let's use fruit icons to illustrate this method:const fruitIcons = ['๐', '๐', '๐', '๐ฅญ']; const removedIcon = fruitIcons.shift(); console.log(removedIcon); // Output: '๐' console.log(fruitIcons); // Output: ['๐', '๐', '๐ฅญ']
2.2. unshift()
unshift(): The
unshift()
method adds one or more elements to the beginning of an array and shifts existing elements up. Let's add fruit icons to the beginning of an array usingunshift()
:const fruitIcons = ['๐', '๐', '๐ฅญ']; fruitIcons.unshift('๐', '๐'); console.log(fruitIcons); // Output: ['๐', '๐', '๐', '๐', '๐ฅญ']
2.3. reverse()
reverse(): The
reverse()
method reverses the order of the elements in an array. Let's reverse an array of fruit icons:const fruitIcons = ['๐', '๐', '๐', '๐ฅญ']; fruitIcons.reverse(); console.log(fruitIcons); // Output: ['๐ฅญ', '๐', '๐', '๐']
2.4. sort()
sort(): The
sort()
method arranges the elements of an array in lexicographic (alphabetical) order by default. Let's sort an array of fruit icons:const fruitIcons = ['๐', '๐', '๐', '๐ฅญ']; fruitIcons.sort(); console.log(fruitIcons); // Output: ['๐', '๐', '๐', '๐ฅญ']
Iterating over Arrays:
3.1. forEach()
forEach(): The
forEach()
method allows you to execute a provided function once for each element in an array. Let's iterate over an array of fruit icons and log each fruit to the console:const fruitIcons = ['๐', '๐', '๐', '๐ฅญ']; fruitIcons.forEach(function(fruit) { console.log(fruit); });
3.2. map()
map(): The
map()
method creates a new array by calling a provided function on every element in the original array. Let's create a new array with uppercase fruit names using an array of fruit icons:const fruitIcons = ['๐', '๐', '๐', '๐ฅญ']; const fruitNames = fruitIcons.map(function(fruit) { return fruit + ' Fruit'; }); console.log(fruitNames);
3.3. filter()
filter(): The
filter()
method creates a new array with all elements that pass a given condition. Let's filter out fruit icons that start with '๐' from an array of fruit icons:const fruitIcons = ['๐', '๐', '๐', '๐ฅญ']; const filteredFruits = fruitIcons.filter(function(fruit) { return fruit.startsWith('๐'); }); console.log(filteredFruits);
Section 1: Manipulating Arrays In this section, we will explore array methods that allow us to add, remove, and combine elements within an array. Each method will be explained step by step, with code examples to demonstrate their usage.
Section 2: Modifying Arrays In this section, we will focus on array methods that modify the content and order of array elements. You will learn how to add or remove elements from the beginning or end of an array, reverse the order of elements, and sort the array based on certain criteria.
Section 3: Iterating over Arrays Iterating over arrays is a common task in JavaScript. In this section, we will cover array methods that simplify the process of iterating over array elements. We will discuss how to perform actions on each element, create new arrays based on existing ones, and filter out specific elements based on conditions.
Conclusion: Understanding and mastering array methods in JavaScript is crucial for efficient and effective coding. In this blog post, we have covered the most commonly used array methods, providing detailed explanations and practical examples for each method. By familiarizing yourself with these array methods, you can manipulate, modify, and iterate over arrays with confidence, enabling you to write more powerful and concise code.
Remember, practice is key to mastering array methods. So, don't hesitate to experiment with these methods and explore their various use cases. Happy coding!
Subscribe to my newsletter
Read articles from Divyesh Godhani directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
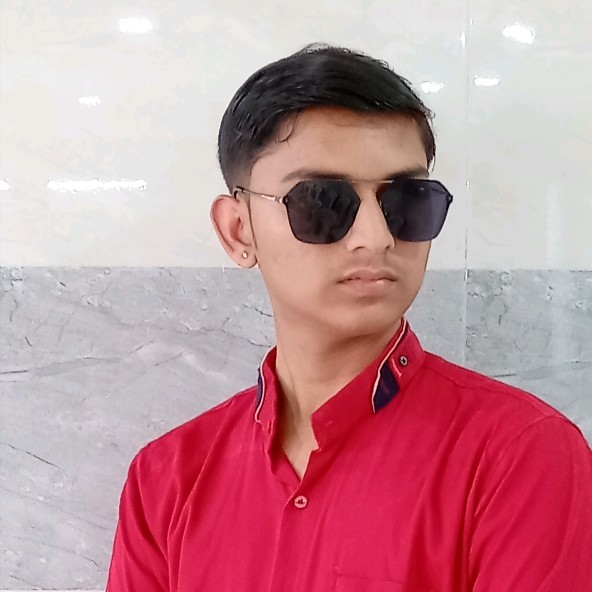
Divyesh Godhani
Divyesh Godhani
I am a MERN Stack and WordPress/PHP Developer at heart and create features that are best suited for the job at hand.