JavaScript Objects: Real Life Objects, Properties, and Methods
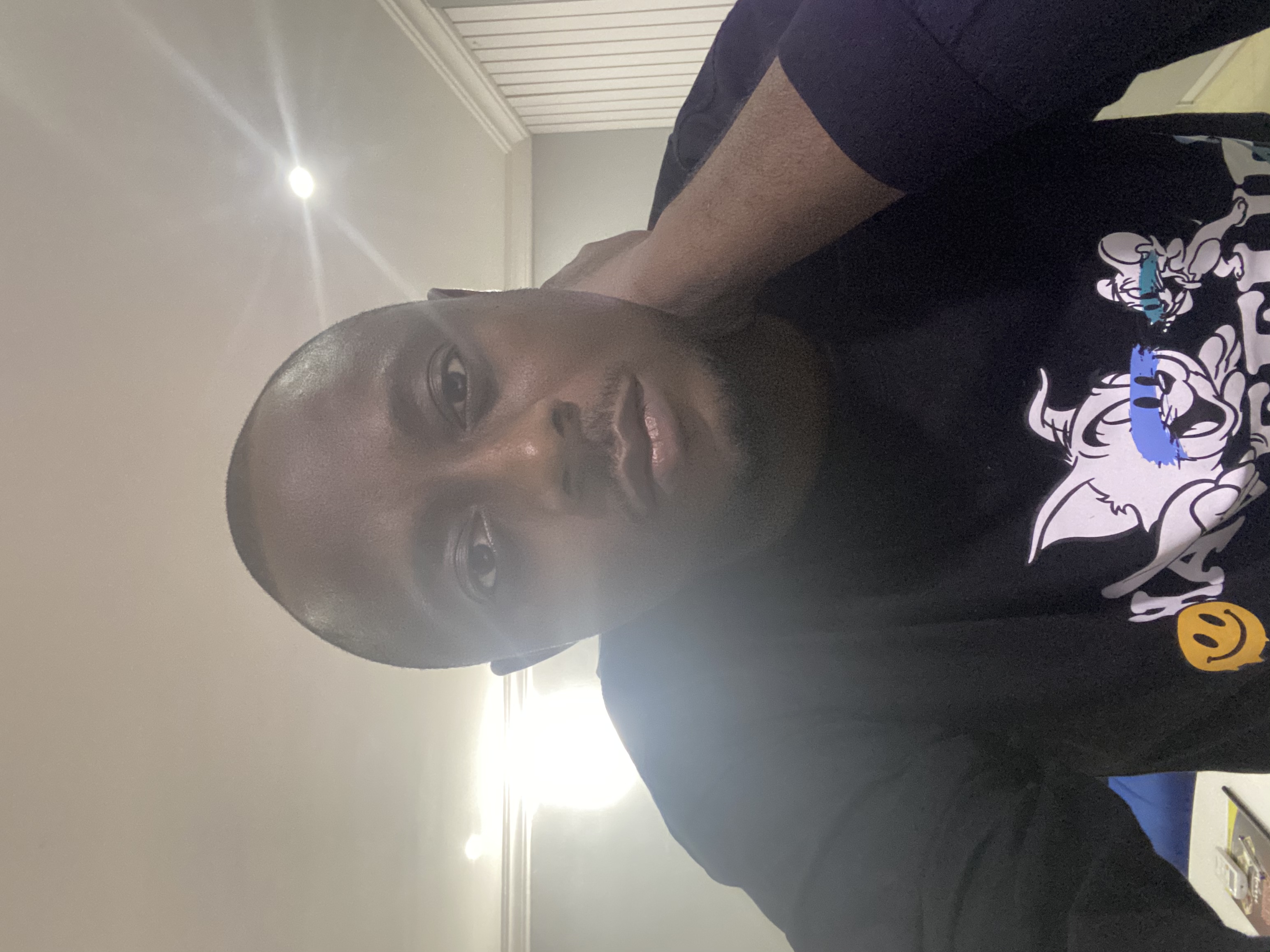
Table of contents
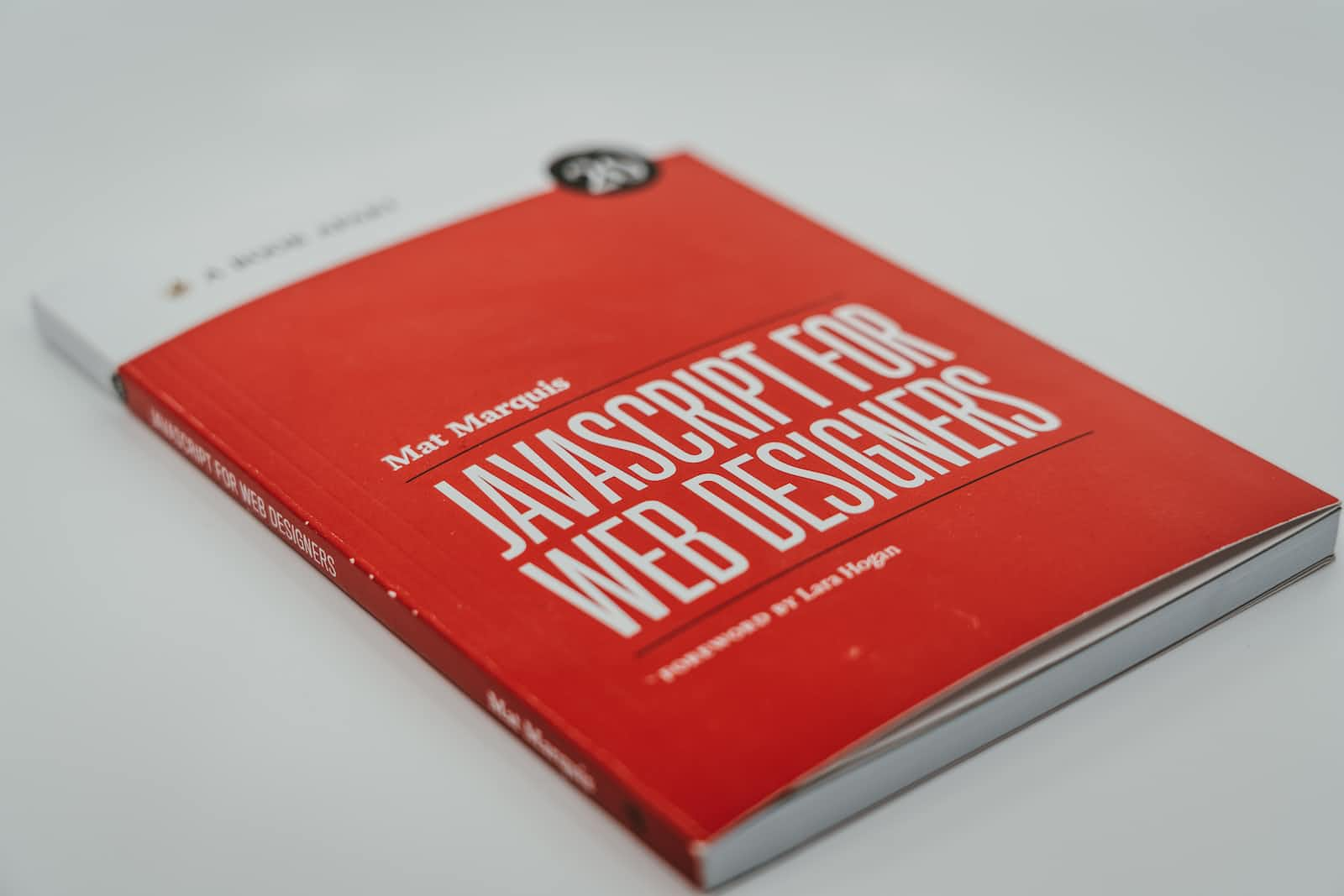
In JavaScript, objects are a fundamental concept used to represent real-life entities, their properties, and the actions they can perform. Let's explore how objects are defined, how properties are accessed, and how methods can be utilized.
Object Definition
Objects in JavaScript can be defined using object literals, which consist of name-value pairs enclosed in curly braces.
const car = {
name: "Fiat",
model: "500",
weight: "850kg",
color: "white",
};
In this example, car
is an object with properties such as name
, model
, weight
, and color
.
Accessing Object Properties
Object properties can be accessed using dot notation or bracket notation.
// Using dot notation
console.log(car.name); // Output: Fiat
// Using bracket notation
console.log(car["model"]); // Output: 500
Both approaches yield the same result. Dot notation is commonly used when the property name is known, while bracket notation is useful when the property name is dynamic or needs to be evaluated.
Object Methods
Objects can also contain methods, which are essentially functions defined as properties. These methods can perform actions or calculations related to the object.
const car = {
name: "Fiat",
model: "500",
start: function() {
console.log("The car is started.");
},
drive: function() {
console.log("The car is being driven.");
},
stop: function() {
console.log("The car is stopped.");
},
};
In this example, car
has methods like start
, drive
, and stop
that can be invoked to perform corresponding actions.
Accessing Object Methods
Object methods can be accessed using the same dot notation or bracket notation as used for properties. The method is invoked by adding parentheses ()
after the method name.
car.start(); // Output: The car is started.
car["drive"](); // Output: The car is being driven.
Conclusion
JavaScript objects allow us to model real-life entities and encapsulate their properties and actions within a single construct. Properties represent the characteristics of an object, while methods define the actions or behaviors associated with it. By utilizing objects, properties, and methods, we can build dynamic and interactive applications that mimic real-world scenarios.
By understanding how objects work in JavaScript and how to access their properties and methods, you can leverage the full power of the language to create more complex and feature-rich applications.
Subscribe to my newsletter
Read articles from Ogunuyo Ogheneruemu B directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
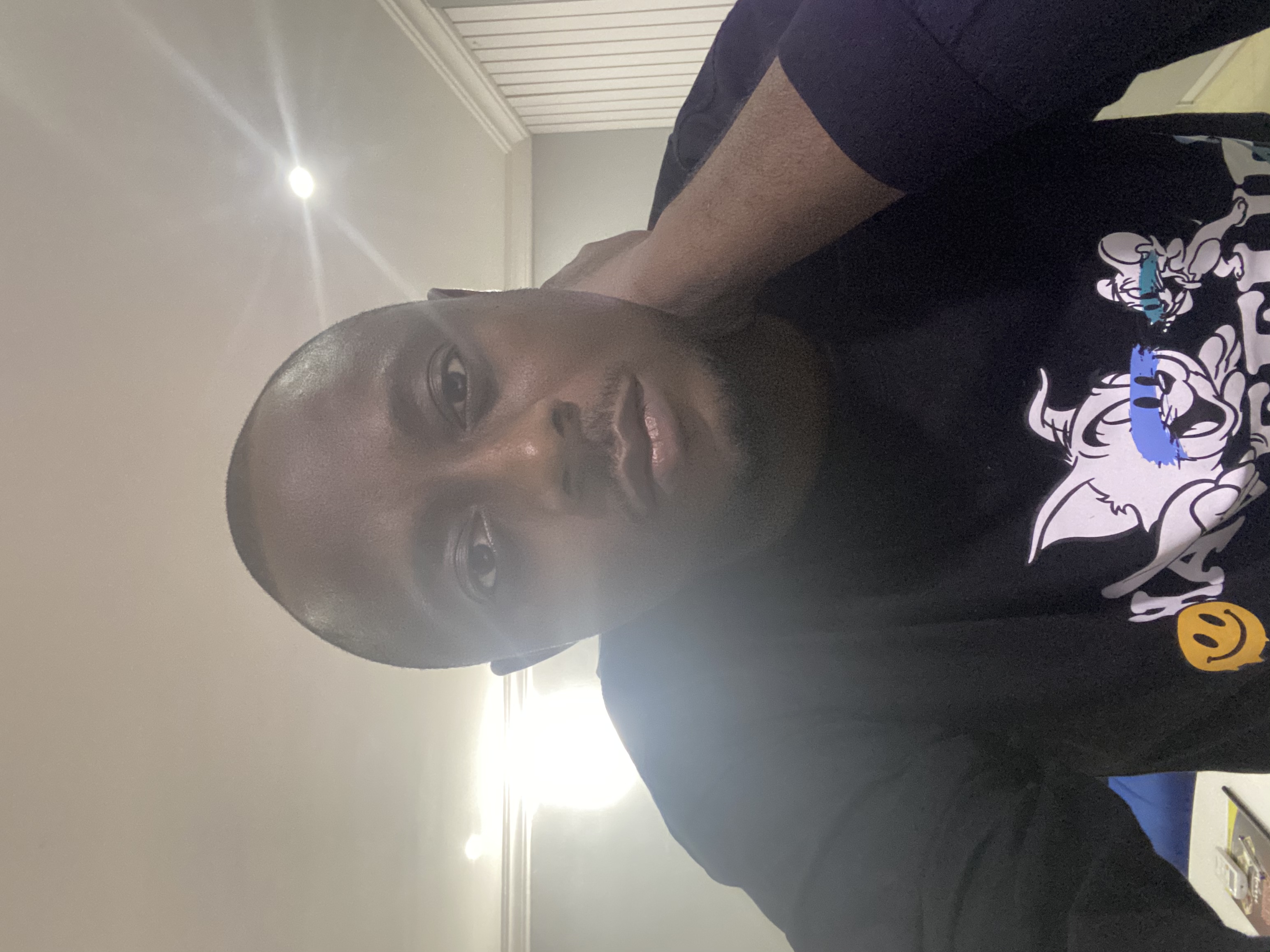
Ogunuyo Ogheneruemu B
Ogunuyo Ogheneruemu B
I'm Ogunuyo Ogheneruemu Brown, a senior software developer. I specialize in DApp apps, fintech solutions, nursing web apps, fitness platforms, and e-commerce systems. Throughout my career, I've delivered successful projects, showcasing strong technical skills and problem-solving abilities. I create secure and user-friendly fintech innovations. Outside work, I enjoy coding, swimming, and playing football. I'm an avid reader and fitness enthusiast. Music inspires me. I'm committed to continuous growth and creating impactful software solutions. Let's connect and collaborate to make a lasting impact in software development.