JavaScript 2D Array – Two Dimensional Arrays in JS

Table of contents
- Why Use a 2D Array in JavaScript?
- How to Access Elements in a JavaScript 2D Array
- How to access the first and last elements of a 2D array
- How to add all elements of a 2D array
- How to Manipulate 2D Arrays in JavaScript
- How to insert an element into a 2D array
- How to remove an element from a 2D array
- How to Create 2D Arrays in JavaScript
- How to create a 2D array using an array literal
- How to create a 2D array using a nested for loop
- How to Update Elements in 2D Arrays in JavaScript
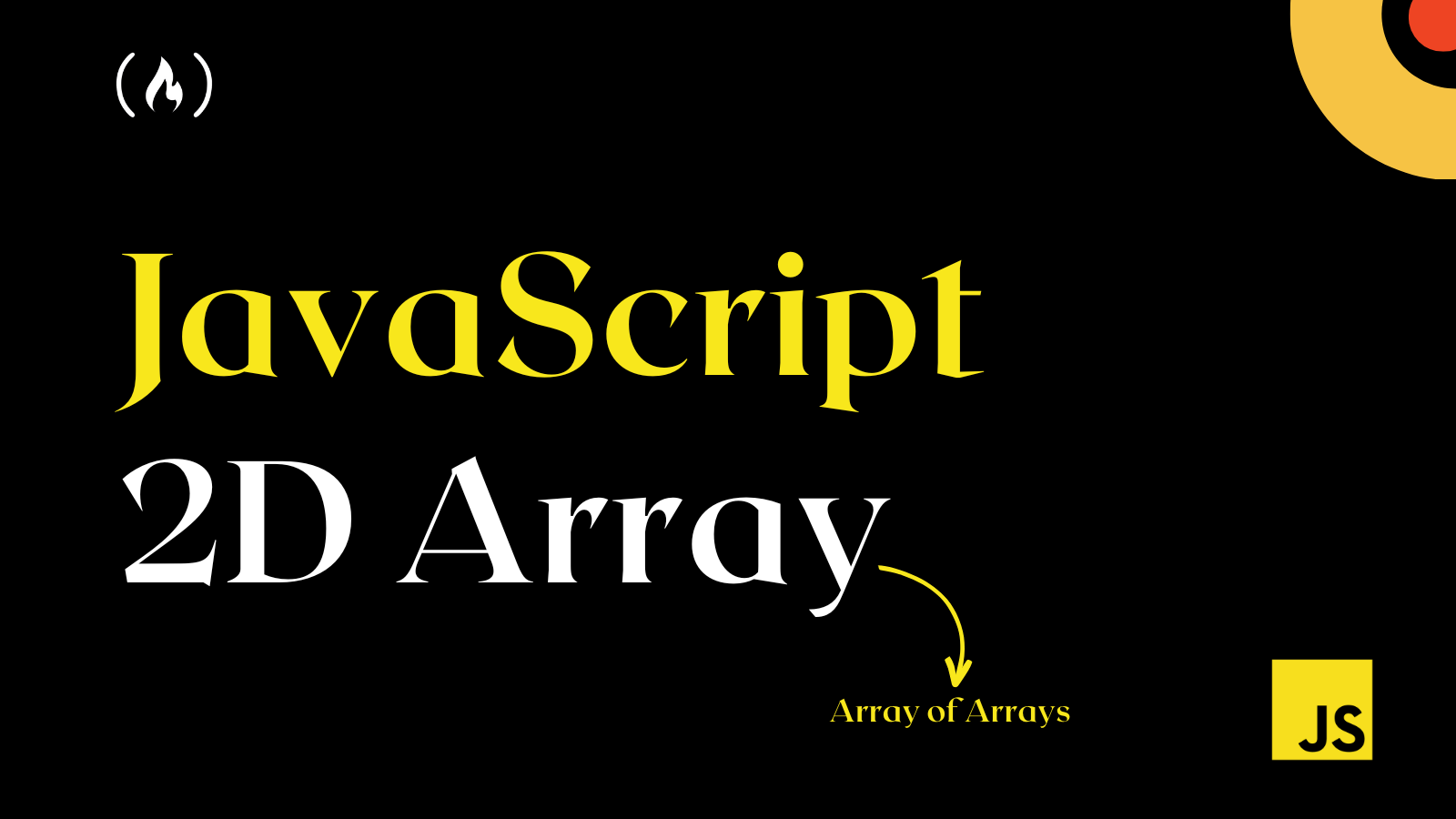
In JavaScript programming, we are all used to creating and working with one-dimensional arrays. ).
In this article, you will learn what two-dimensional arrays are and how they work in JavaScript.
What is a Two-Dimensional Array?
In JavaScript, a two-dimensional array, also known as a 2D array, is an array of arrays. It allows you to store data in a grid-like structure with rows and columns. Each element in a 2D array is accessed using two indices: one for the row and another for the column.
// Create a 2D array with 3 rows and 4 columns
var grid = [
[1, 2, 3, 4],
[5, 6, 7, 8],
[9, 10, 11, 12]
];
Conceptually, you can think of a 2D array as a table with rows and columns, where each cell holds a value. This structure is commonly used in various programming scenarios, such as representing game boards, storing spreadsheet-like data, working with images and pixels, or solving mathematical problems involving matrices.
In programming languages that support two-dimensional arrays, such as JavaScript, you can create, access, and manipulate elements within the array using the two indices. This allows for efficient data storage and retrieval, as well as performing operations on the grid-like structure.
Overall, a two-dimensional array provides a powerful and flexible way to organize and work with structured data that exhibits a grid-like pattern, making it a fundamental data structure in many programming tasks.
Why Use a 2D Array in JavaScript?
In JavaScript, we use two-dimensional arrays, also known as matrices, to store and manipulate data in a structured and organized manner.
There are several reasons to use a 2D array in JavaScript:
Tabular Data: If you have data that naturally fits into a grid-like structure, such as spreadsheet data or game boards, a 2D array provides a convenient way to represent and manipulate that data.
Matrix Operations: Matrices are commonly used in mathematical computations, scientific simulations, and image processing. A 2D array allows you to store and perform operations on matrices efficiently.
Game Development: Many games require a grid-based layout, where each cell represents a specific element or state. A 2D array provides a simple and intuitive way to represent game boards and track the state of game elements.
Image Processing: When working with images, pixels are often arranged in a grid-like structure. A 2D array can be used to store pixel values, apply filters or transformations, and manipulate image data.
Algorithms and Problem Solving: Certain algorithms and problem-solving techniques involve working with grids or matrices. A 2D array allows you to implement and operate on these data structures effectively.
How to Access Elements in a JavaScript 2D Array
You can access the elements of a two-dimensional array using two indices, one for the row and one for the column. Suppose you have the following two-dimensional array:
var grid = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
];
To access an element in the array, you need to specify the row index followed by the column index inside square brackets []
. For example:
var element = grid[1][2];
console.log(element); // Output: 6
In the above code, grid[1]
accesses the second row (index 1), and then [2]
accesses the third column (index 2) within that row. The resulting value is 6
.
You can also assign a new value to an element using the same access pattern:
grid[0][1] = 10;
console.log(grid[0][1]); // Output: 10
In this case, we update the value of the element at the first row and second column to 10
.
Remember that array indices start from 0, so the first row is accessed using index 0, the second row with index 1, and so on. Similarly, the first column within a row is accessed using index 0, the second column with index 1, and so on.
How to access the first and last elements of a 2D array
Sometimes you might need to find the first and last elements of a two-dimensional array. You can do this using the first and last index of both the rows and columns.
The first element will always have the row and column index of 0, meaning you will use arrayName[0][0],
.
The index of the last element can be tricky, however. For example, in the example above, the first element is ‘John Doe’ while the
console.log(MathScore[0][0]); // returns 'John Doe'
console.log(MathScore[MathScore.length-1][(MathScore[MathScore.length -1]).length - 1]); // returns 'B'
How to add all elements of a 2D array
In some situations, all the elements of your 2D array can be numbers, so you may need to add them together and arrive at just one digit.To add all elements of a 2D array in JavaScript, you can use nested loops to iterate over the array and accumulate the values.
var grid = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
];
var sum = 0;
for (var i = 0; i < grid.length; i++) {
for (var j = 0; j < grid[i].length; j++) {
sum += grid[i][j];
}
}
console.log(sum); // Output: 45
How to Manipulate 2D Arrays in JavaScript
You can manipulate 2D arrays just like one-dimensional arrays using general array methods like pop, push, splice and lots more.
How to insert an element into a 2D array
You can add an element or many elements to a 2D array with the push() and unshift() methods.
The push() method adds elements(s) to the end of the 2D array, while the unshift() method adds element(s) to the beginning of the 2D array.
let MathScore = [
["John Doe", 20, 60, "A"],
["Jane Doe", 10, 52, "B"],
["Petr Chess", 5, 24, "F"],
["Ling Jess", 28, 43, "A"],
["Ben Liard", 16, 51, "B"]
];
MathScore.push(["Tom Right", 30, 32, "B"]);
MathScore.unshift(["Alice George", 28, 62, "A"]);
this will return:
[
["John Doe", 20, 60, "A", 80],
["Jane Doe", 10, 52, "B", 62],
["Petr Chess", 5, 24, "F", 29],
["Ling Jess", 28, 43, "A", 71],
["Ben Liard", 16, 51, "B", 67]
]
You can also use the unshift()
method to insert the element at the beginning and the splice()
method to insert at the middle of the array:
let MathScore = [
["John Doe", 20, 60, "A"],
["Jane Doe", 10, 52, "B"],
["Petr Chess", 5, 24, "F"],
["Ling Jess", 28, 43, "A"],
["Ben Liard", 16, 51, "B"]
];
MathScore.splice(2, 0, ["Alice George", 28, 62, "A"]);
this is output:
[
["John Doe", 20, 60, "A"],
["Jane Doe", 10, 52, "B"],
["Alice George", 28, 62, "A"],
["Petr Chess", 5, 24, "F"],
["Ling Jess", 28, 43, "A"],
["Ben Liard", 16, 51, "B"]
]
How to remove an element from a 2D array
You can also remove element(s) from the beginning and end of a 2D array with the pop()
and shift()
methods.
let MathScore = [
["John Doe", 20, 60, "A"],
["Jane Doe", 10, 52, "B"],
["Petr Chess", 5, 24, "F"],
["Ling Jess", 28, 43, "A"],
["Ben Liard", 16, 51, "B"]
];
MathScore.pop();
MathScore.shift();
this is the output:
[
["Jane Doe", 10, 52, "B"],
["Petr Chess", 5, 24, "F"],
["Ling Jess", 28, 43, "A"],
]
You can also remove elements from each array element:
let MathScore = [
["John Doe", 20, 60, "A"],
["Jane Doe", 10, 52, "B"],
["Petr Chess", 5, 24, "F"],
["Ling Jess", 28, 43, "A"],
["Ben Liard", 16, 51, "B"]
];
MathScore.forEach((score) => {
score.pop();
});
console.log(MathScore)
output return:
[
["John Doe", 20, 60],
["Jane Doe", 10, 52],
["Petr Chess", 5, 24],
["Ling Jess", 28, 43],
["Ben Liard", 16, 51]
]
You can also use the shift()
method to remove the element at the beginning and the splice()
method to remove array elements from specific positions:
let MathScore = [
["John Doe", 20, 60, "A"],
["Jane Doe", 10, 52, "B"],
["Petr Chess", 5, 24, "F"],
["Ling Jess", 28, 43, "A"],
["Ben Liard", 16, 51, "B"]
];
MathScore.splice(2, 1);
output:
[
["John Doe", 20, 60, "A"],
["Jane Doe", 10, 52, "B"],
["Ling Jess", 28, 43, "A"],
["Ben Liard", 16, 51, "B"]
]
How to Create 2D Arrays in JavaScript
There are two options for creating a multi-dimensional array. You can create the array manually with the array literal notation, which uses square brackets []
to wrap a list of elements separated by commas. You can also use a nested loop.
How to create a 2D array using an array literal
This is just like the examples we have been considering using the following syntax:
let MathScore = [
["John Doe", 20, 60, "A"],
["Jane Doe", 10, 52, "B"],
["Petr Chess", 5, 24, "F"],
["Ling Jess", 28, 43, "A"],
["Ben Liard", 16, 51, "B"]
];
How to create a 2D array using a nested for loop
There are many approaches to doing this. But generally, you create a nested loop where the first loop will loop through the rows while the second loop will loop through the elements in the inner array (columns):
let arr = [];
let rows = 4;
let columns = 3;
// creating two-dimensional array
for (let i = 0; i < rows; i++) {
arr[i] = [];
for (let j = 0; j < columns; j++) {
arr[i][j] = j;
}
}
console.log(arr);
You can also decide to create a function that accepts these values as arguments:
const create2Darr = (rows, columns) => {
let arr = [];
let value = 0;
// creating two-dimensional array
for (let i = 0; i < rows; i++) {
arr[i] = [];
for (let j = 0; j < columns; j++) {
arr[i][j] = value++;
}
}
console.log(arr);
};
let rows = 4;
let columns = 3;
create2Darr(rows, columns
How to Update Elements in 2D Arrays in JavaScript
This is very similar to how you do it with one-dimensional arrays, where you can update an array’s value by using the index to assign another value.You can use the same approach to change an entire row or even individual elements:
let MathScore = [
["John Doe", 20, 60, "A"],
["Jane Doe", 10, 52, "B"],
["Petr Chess", 5, 24, "F"],
["Ling Jess", 28, 43, "A"],
["Ben Liard", 16, 51, "B"]
];
MathScore[1] = ["Alice George", 28, 62, "A"];
console.log(MathScore);
Subscribe to my newsletter
Read articles from MOHAMMAD AYMON directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
