Libraries in solidity
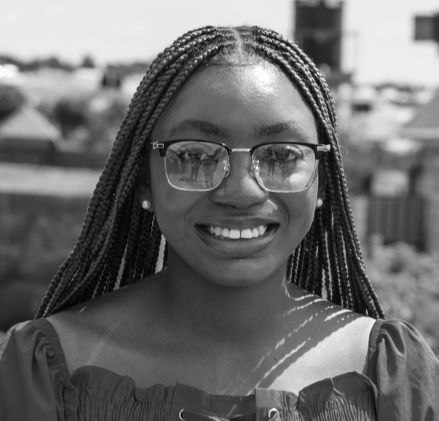
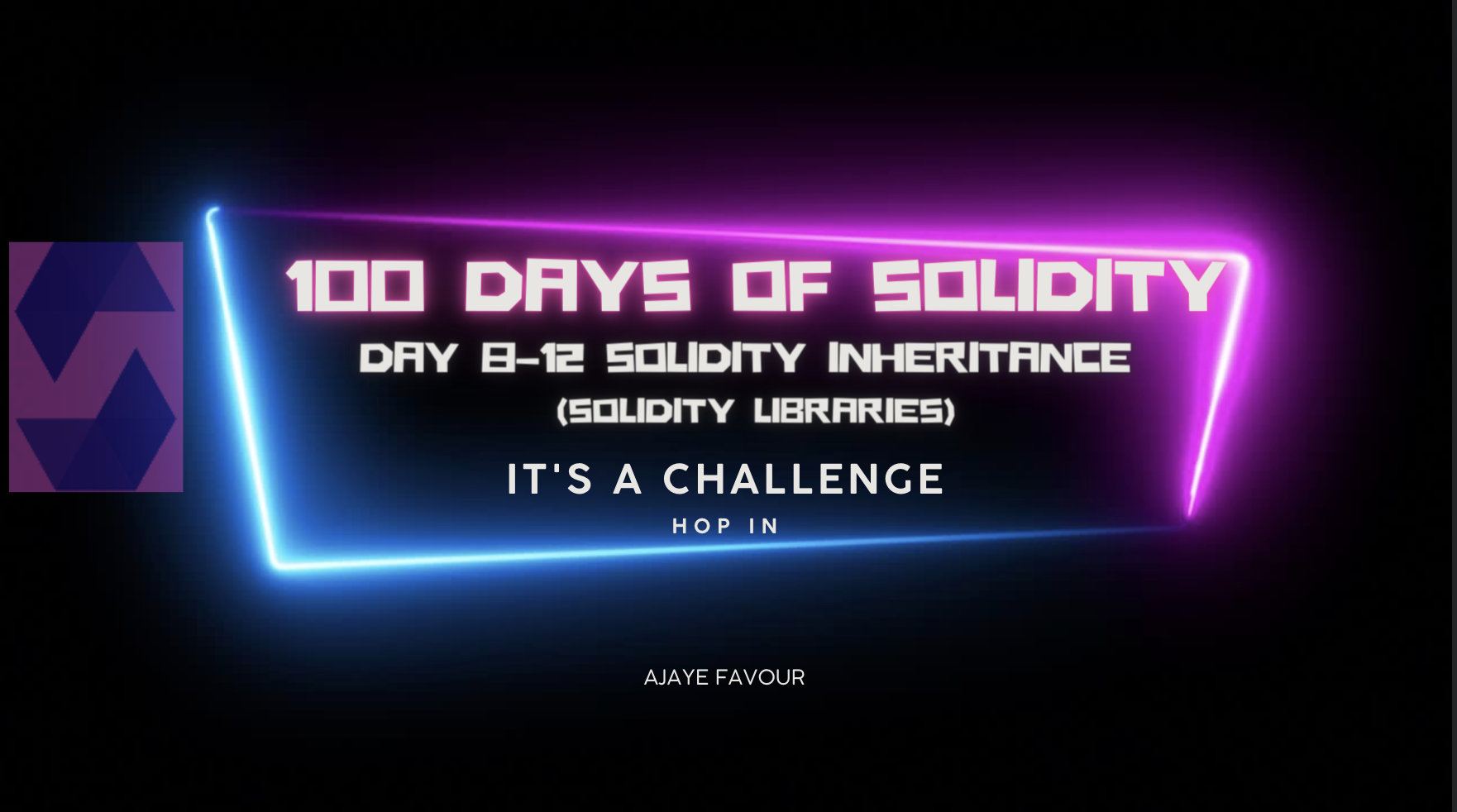
In Solidity, a library is a reusable collection of functions and code that can be deployed and used by other contracts. It allows you to separate code and enhance data types.
Understanding Libraries in a simpler way
Let's explain libraries in a simple way:
Think of a library as a toolbox filled with useful tools (functions) that you can borrow to build something. In the world of Solidity and smart contracts, a library is a collection of functions that can be reused by different contracts.
Instead of writing the same code over and over again in multiple contracts, you can create a library with those functions. Then, other contracts can use that library to access and use those functions without having to rewrite them.
Libraries help make your code shorter, more organized, and easier to maintain. They allow you to avoid repeating the same code and instead focus on building the unique parts of your contracts.
Just like you borrow tools from a toolbox when you need them, contracts borrow functions from a library when they need specific functionality. Libraries are like a helpful resource that saves you time and effort when writing and managing code.
Key points about libraries in solidity
Here are some key points to understand about libraries in Solidity:
Code Reusability: Libraries enable you to write modular code that can be used by multiple contracts. By separating commonly used functionality into a library, you can avoid duplicating code and make your contracts more concise and maintainable.
Deployed Once: Unlike regular contracts, libraries are not deployed independently. Instead, they are deployed once, and their bytecode is reused by contracts that utilize them. This helps reduce deployment costs and allows for efficient code sharing.
Stateless: Libraries do not have storage or persistent states. They can only operate on the data passed to them as function arguments. This means that libraries cannot store or modify contract-specific data.
Gas Efficiency: Libraries can enhance gas efficiency by reducing redundant code and allowing for optimized bytecode. Since the library's code is reused, it reduces the overall size of the compiled contract and can result in cost savings during deployment and execution.
Use cases for libraries in Solidity
These are just a few examples of how libraries can be used in Solidity.
Math Library: A common use case for libraries is mathematical operations. You can create a library that includes functions for calculations such as addition, subtraction, multiplication, and division. This library can be reused across multiple contracts, avoiding code duplication and promoting efficiency.
String Utilities: Solidity doesn't have built-in string manipulation functions, so you can create a library to handle string operations like concatenation, length calculation, or substring extraction. This library can be used by contracts that require string manipulation functionality.
Sorting Algorithms: Libraries can also be used to implement sorting algorithms such as bubble sort, quicksort, or mergesort. By encapsulating the sorting logic in a library, you can reuse it in various contracts that require sorting functionality for different data sets.
Access Control: Libraries can provide functions for access control mechanisms, such as role-based permissions or access modifiers. By creating a library for access control, you can easily incorporate secure permission systems into multiple contracts, ensuring consistent and controlled access to specific functions or data.
Data Structures: Libraries can define and implement common data structures like arrays, linked lists, or binary trees. These data structure libraries can be utilized by contracts that need to organize and manage data in specific ways.
Crypto Operations: Libraries can be employed for cryptographic operations like hashing, encryption, or digital signatures. By encapsulating these operations in a library, you can reuse them in contracts that require cryptographic functionality for secure operations.
The idea is to create reusable code components that can be shared across multiple contracts, promoting code efficiency, reducing redundancy, and facilitating code maintenance.
Code examples demonstrating the usage of libraries in Solidity
Example 1:
Example 2:
In the first example, a MathUtils library is created to provide mathematical operations. The library is then used within the MyContract contract to perform calculations.
In the second example, a StringUtils library is defined to handle string manipulation functions. The library is utilized within the MyContract contract to concatenate strings and retrieve their lengths.
By declaring the functions as internal, you encapsulate them within the library. This means that the functions can only be accessed and used within the library or by contracts that use the library. It helps maintain the integrity of the library's functionality and prevents direct external access or misuse. Internal functions provide a level of access control. They cannot be accessed by external contracts or accounts directly. This restricts the usage of the functions to the library and the contracts that have imported and utilized the library. It helps ensure that only authorized contracts can utilize the functions.
Summary
Libraries allow you to encapsulate commonly used functionality, promote code reuse, and enhance the efficiency of your smart contracts. They provide a way to organize and share commonly used functionality across multiple contracts, promoting code reusability and reducing development time; making your code more efficient and reusable.
Common use cases for libraries in Solidity include mathematical operations, data manipulation, sorting algorithms, and more.
Libraries should be stateless and not modify contract states directly. They should operate solely on the provided input parameters and return results without altering any contract-specific data.
Libraries are not designed for storing large amounts of data. Avoid using libraries as a substitute for contract storage. Instead, focus on using libraries for reusable functions and separate storage concerns appropriately.
Libraries should not rely on external states or access variables outside their scope. They should be self-contained and independent, making them easier to use and reducing dependence on external factors.
In case you missed solidity data types, click here.
Read about Solidity’s fallback function and function overloading here.
Also, click here to learn more about variables and control structures in solidity and here for solidity functions.
Learn a lot more about inheritances here.
Learn about abstract contracts and interfaces here
Click here to see the Github repo for this 100 days of solidity challenge.
Click here for guidelines for becoming a Solidity developer.
To learn more about blockchain, click here.
To learn more about Web3, click here.
Follow me for more knowledge on Solidity, Ethereum, and blockchain.
Subscribe to my newsletter
Read articles from Favour Ajaye directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
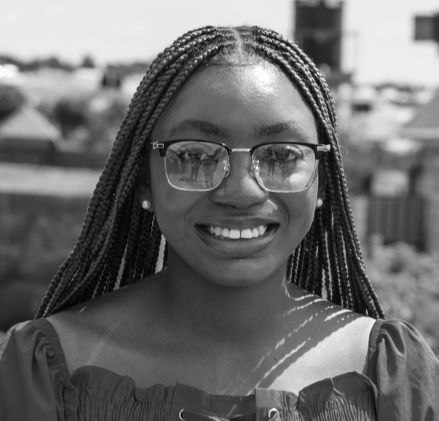
Favour Ajaye
Favour Ajaye
smart contract developer