Building Secured Applications in .NET Core C#
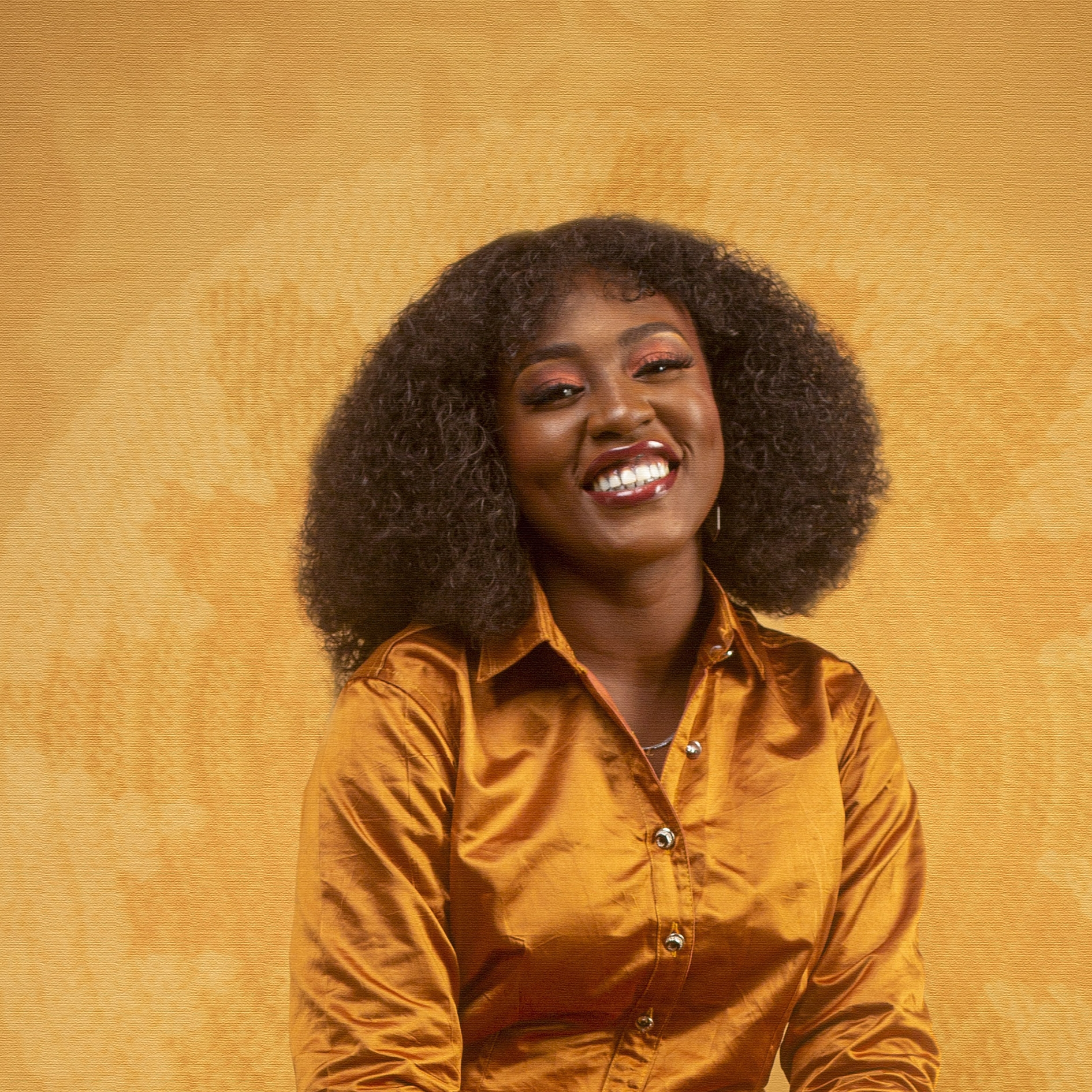
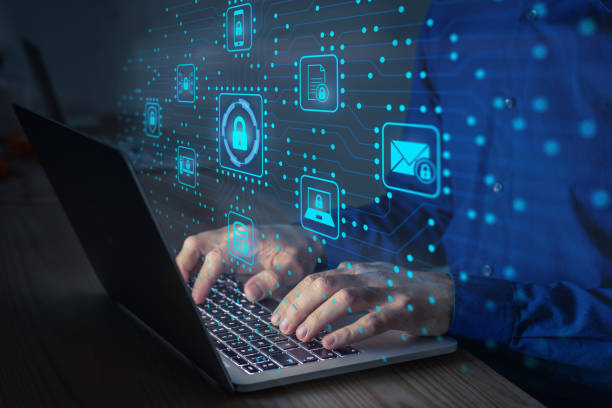
In the modern era of digitalization, ensuring security stands as a paramount concern when it comes to the development of any application. The construction of secure applications holds immense importance to safeguard sensitive user data, averting unauthorized access, and providing protection against potential cyber threats. Amidst the realm of developing secure applications, .NET Core, an all-encompassing framework devised by Microsoft, offers a sturdy foundation. This informative article will go into essential techniques and the best methods for building strong apps in .NET Core using the C# programming language.
Secure Application Development Best Practices
Input Validation
From protecting against malicious user attacks to mitigating widespread security flaws such as SQL injection and cross-site scripting (XSS) attacks, input validation plays an important role in developing secure applications. It is important to thoroughly verify all user data before processing or storing it, this way we ensure application integrity.
// Example of input validation using data annotations
public class UserModel
{
[Required]
public string Username { get; set; }
[Required]
[EmailAddress]
public string Email { get; set; }
// Add more validation attributes as per your requirements
}
Authentication and Authorization
It's essential for the security of your applications to provide strong authentication and authorization systems. Use industry-standard authentication mechanisms, such as OAuth or OpenID Connect. Make sure that user roles and permissions are used to restrict access to sensitive resources. To handle these aspects effectively, make use of the built-in authentication and authorisation middleware in .NET Core.
// Example of authentication and authorization middleware configuration
public void ConfigureServices(IServiceCollection services)
{
// Add authentication services
services.AddAuthentication(options =>
{
options.DefaultAuthenticateScheme = JwtBearerDefaults.AuthenticationScheme;
options.DefaultChallengeScheme = JwtBearerDefaults.AuthenticationScheme;
}).AddJwtBearer(options =>
{
options.TokenValidationParameters = new TokenValidationParameters
{
// Add token validation parameters
};
});
// Add authorization policy
services.AddAuthorization(options =>
{
options.AddPolicy("AdminOnly", policy =>
{
policy.RequireRole("Admin");
});
});
// Add other service configurations
}
Secure Data Storage
In order to ensure application security, sensitive data must be protected. To safeguard data while it is at rest and while it is being sent, use the right encryption techniques. .NET Core provides libraries and APIs for implementing different encryption methods like AES or RSA. Consistently use powerful cryptographic techniques to encrypt sensitive data, such as passwords or credit card numbers, before storing it.
// Example of data encryption using AES algorithm
public byte[] EncryptData(byte[] data, byte[] key, byte[] iv)
{
using Aes aes = Aes.Create();
aes.Key = key;
aes.IV = iv;
using MemoryStream memoryStream = new MemoryStream();
using CryptoStream cryptoStream = new CryptoStream(memoryStream, aes.CreateEncryptor(), CryptoStreamMode.Write);
cryptoStream.Write(data, 0, data.Length);
cryptoStream.FlushFinalBlock();
return memoryStream.ToArray();
}
// Add corresponding decryption method
Error Handling and Logging
Identifying and addressing security vulnerabilities or breaches requires effective error handling and tracking. Implement appropriate exception handling procedures and keep a log of any potential security-related mistakes or occurrences.Utilize .NET Core's logging framework to record important data for analysis and troubleshooting, such as error details and stack traces.
using Microsoft.Extensions.Logging;
// ...
class MyClass
{
private readonly ILogger<MyClass> logger;
public MyClass(ILogger<MyClass> logger)
{
this.logger = logger;
}
public void SomeMethod()
{
try
{
// Code that may throw exceptions
// ...
}
catch (Exception ex)
{
// Log the exception details
logger.LogError(ex, "An error occurred while processing the request.");
// Handle the exception or rethrow it
// ...
}
}
}
Conclusion
It's important to follow best practices and have a thorough grasp of potential security concerns while developing protected apps in .NET Core C#. You may strengthen your apps' security posture and defend them against various attacks by adhering to the recommendations given in this article. As the threat landscape continues to change, keep yourself up to date with the most recent security standards and procedures. Your applications will be resilient against new threats if you use secure coding techniques and thorough application security controls.
It's time to use these techniques in your .NET Core projects. Keep an eye out for threats, put security first, and create apps that can endure the dynamic cybersecurity environment.
Subscribe to my newsletter
Read articles from Gift Temitope directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
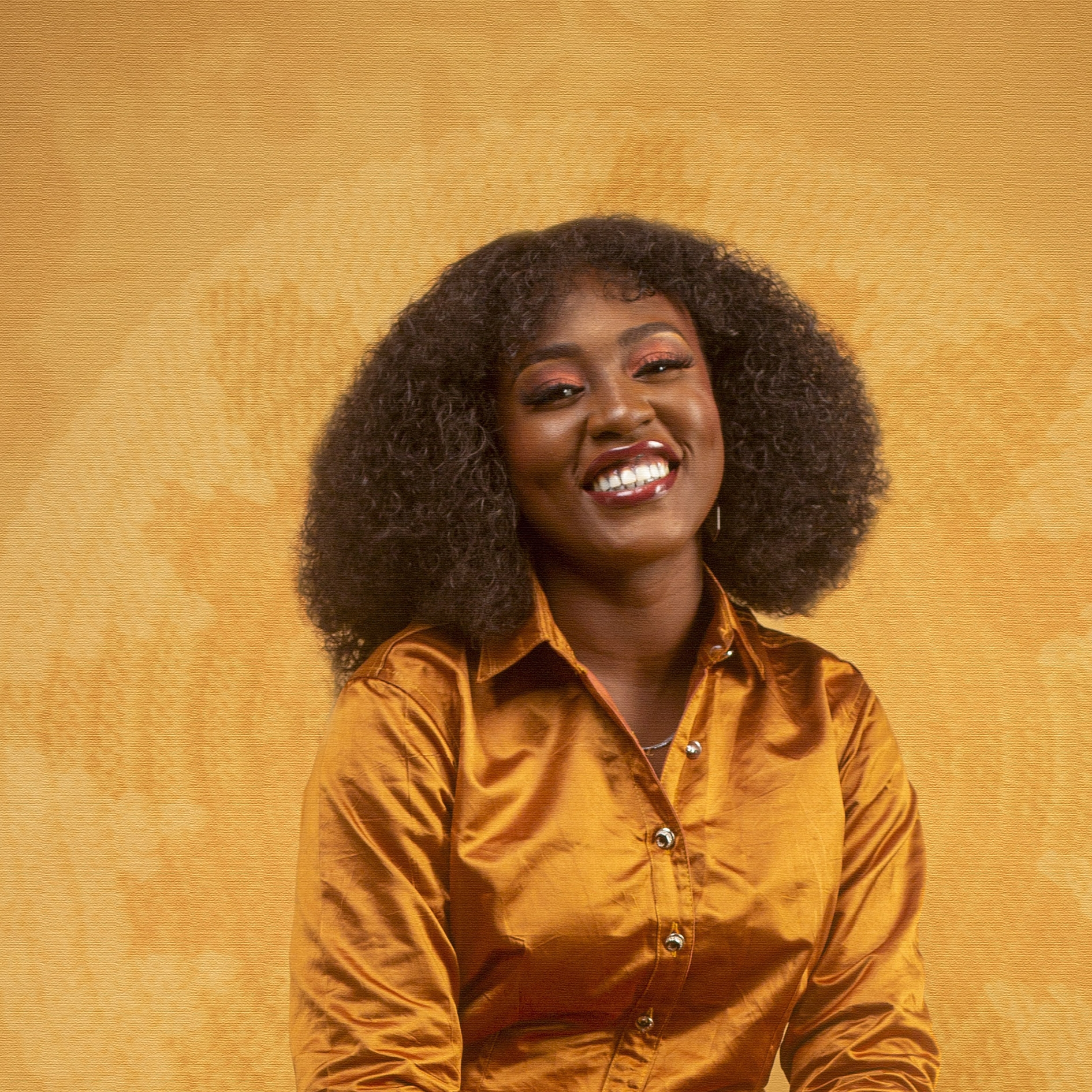
Gift Temitope
Gift Temitope
Passionate about technology and driven by innovation, I am constantly exploring the limitless possibilities of the digital world. As a tech enthusiast, I thrive on solving complex problems and creating impactful solutions. With a strong background in software development and a knack for staying up-to-date with the latest industry trends, I'm always ready to embark on new challenges and push the boundaries of what's possible. Let's connect and collaborate to shape the future of technology together. #TechEnthusiast #InnovationDriven