Understanding REACTJS SIMPLIFIED for a complete Beginner
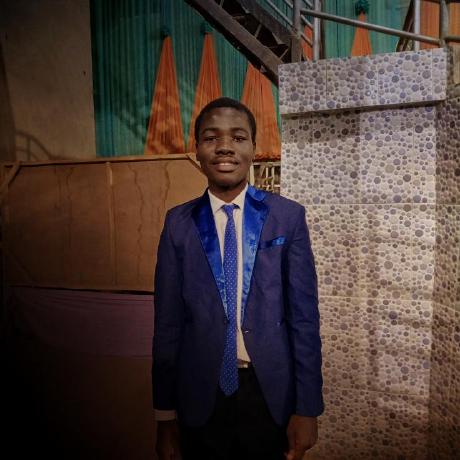
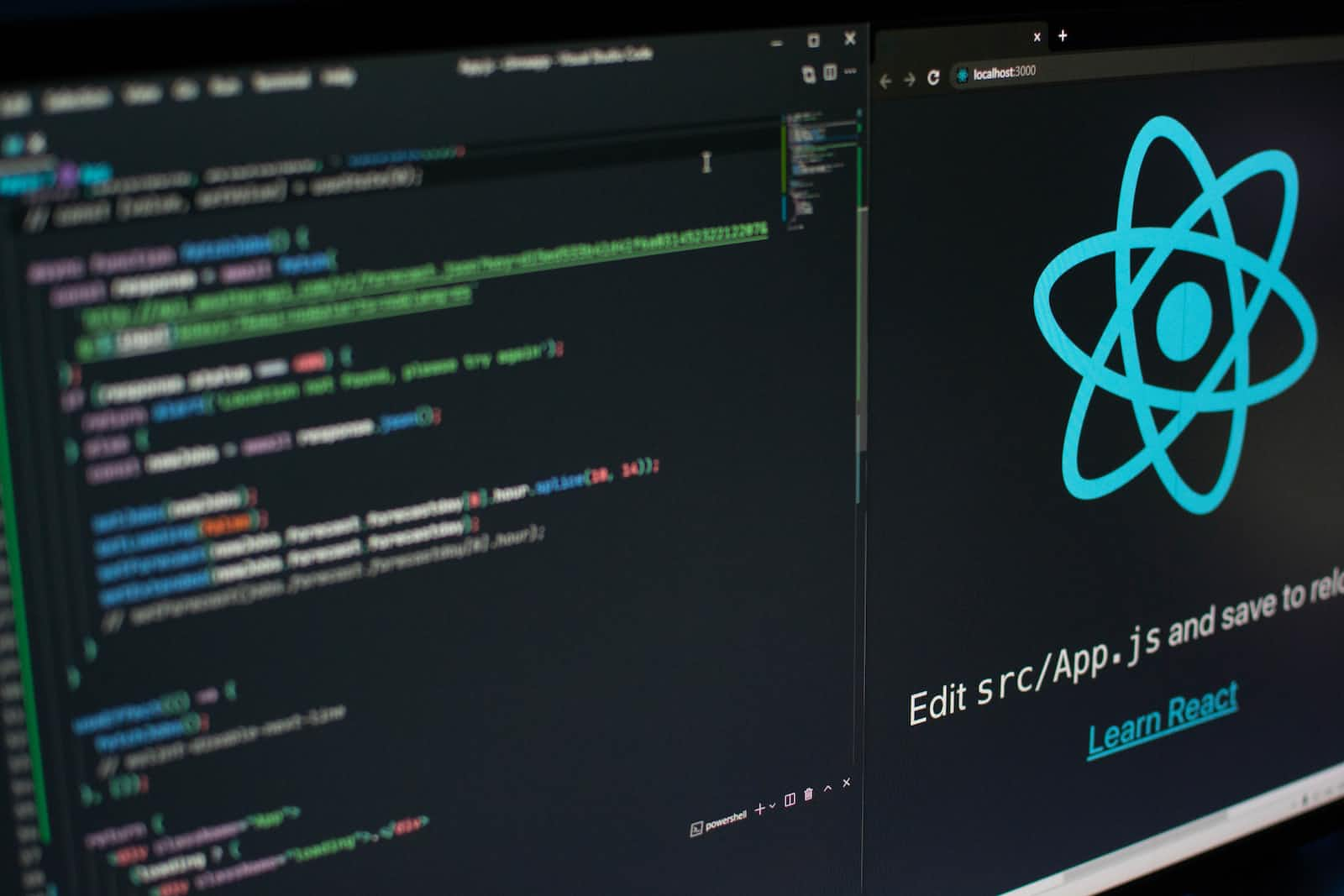
Been surfing the internet hard just to have a comprehension of REACTJS? Well, here is your stop and the final one! Let's get right to business...
What is ReactJS?
ReactJS (also known as React.js or simply React) is a flexible and powerful open-source JavaScript Library for developing rich client-side applications.
React creates a means and method that makes building rich web applications easier and faster.
ReactJS is a JavaScript library for building user interfaces that encourage the creation of reusable UI (User Interfaces) components that present data that are mutable (or change over time). ReactJS can help reduce the use of boilerplate codes (sections of codes that are used in multiple with little or no variation).
ReactJS has some advantages which include being capable of being used on the client and server side as well with other frameworks or technologies. The components and data patterns in React help to improve readability and make it easy to maintain larger web applications.
Any Prerequisite to understanding ReactJS?
Yes of course, before starting with ReactJS, it is recommended to know, HTML (Hypertext Markup Language), CSS (Cascading Style Sheet) and the fundamentals of Javascript with some advanced vanilla JavaScript (pure JavaScript without the addition of external libraries) codes defined in the ES6 templates.
Who Created ReactJS?
React was created by Jordan Walke, a software engineer at Facebook. He was inspired by the functional programming language Lisp and sought to create a library that would allow developers to create reusable components that could be easily combined to build complex user interfaces.
React Features
JSX
Components
Virtual DOM
Simplicity
Performance
JSX
JSX stands for JavaScript XML. It is a JavaScript syntax extension. It is also an XML or HTML-like syntax used by ReactJS.
Just as Blade is to PHP, just as Django Templates is to Django; so is JSX to ReactJS.
This essentially means you can write JavaScript logic codes within an HTML template, it allows you to bring the dynamics of JavaScript into a static markup language. Hence, it extends the ES6 so that HTML-like text can co-exist with JavaScript react code. It is not compulsory to use JSX in React though, but It's simply recommended.
import React from 'react';
import ReactDOM from 'react-dom';
const imageUrl = 'https://example.com/image.jpg';
const element = <img src={imageUrl} alt="Example" />;
ReactDOM.render(element, document.getElementById('root'));
In this example, we use JSX to create an <img>
element and provide attributes such as src
and alt
. The src
attribute is set using a JavaScript expression {imageUrl}
.
These snippets demonstrate how JSX allows us to write HTML-like syntax within JavaScript code. It enables us to create and compose React components in a more declarative and intuitive way.
Components
ReactJS deals essentially with components. ReactJS application is made up of multiple components, and each of these components has its logic and controls and can exist on its own. These components can be reusable which helps the developer to maintain the code when working on larger-scale projects
import React from 'react';
// Functional component
const Greeting = ({ name }) => {
return <h1>Hello, {name}!</h1>;
};
// Class component
class Counter extends React.Component {
constructor(props) {
super(props);
this.state = {
count: 0,
};
}
incrementCount() {
this.setState((prevState) => ({ count: prevState.count + 1 }));
}
render() {
return (
<div>
<h2>Counter: {this.state.count}</h2>
<button onClick={() => this.incrementCount()}>Increment</button>
</div>
);
}
}
// Parent component
class App extends React.Component {
render() {
return (
<div>
<Greeting name="Alice" />
<Greeting name="Bob" />
<Counter />
</div>
);
}
}
export default App;
In this example, we have three components: Greeting
, Counter
, and App
.
The The greeting
component is a functional component that receives a name
prop and displays a greeting message with the provided name.
The The counter
component is a class component that manages a count
state. It has a method incrementCount()
to update the state when the button is clicked, and it renders the current count along with a button to increment it.
The The app
component is the parent component that renders both the Greeting
component twice with different names and the Counter
component.
This code demonstrates the basic structure of React components, and how they can receive and use props, manage state, and render JSX elements.
Virtual DOM
React Virtual DOM is a concept used in React.js to optimize the rendering process. It is a lightweight copy of the actual DOM (Document Object Model) that React creates and manipulates. The Virtual DOM acts as an intermediary between the application's state changes and the actual browser DOM.
When a component's state changes, React creates a new Virtual DOM tree and compares it with the previous one. It determines the differences and updates only the necessary parts in the actual DOM, avoiding unnecessary re-renders
Manipulating the Virtual DOM is faster than directly interacting with the browser's DOM. React batches multiple updates and performs them in an optimized manner, minimizing the performance impact
Declarative updates: React allows developers to express how the UI should look for a given state. Instead of manually manipulating the DOM, developers define components using JSX, which is then translated into the Virtual DOM representation.
Portable: The Virtual DOM is independent of the specific browser environment. It allows developers to write React applications that can run on various platforms and environments, making them versatile and adaptable.
Simplicity
ReactJS uses JSX file which makes the application simple and to code as well as understand
Performance
The React Virtual DOM is a cross-platform and programming API that deals with HTML, XML, or XHTML. The DOM exists entirely in the memory. Due to this, when we create a component, we are writing virtual components that will turn into the DOM and this leads to smoother and faster performance.
Pros and Cons of React.JS
PROS
ReactJS is easy to learn :
Any developer who comes particularly from a JavaScript background can easily get a good hang of ReactJS and start building stuff in a matter of time.
ReactJS makes it easy to create dynamic web applications :
ReactJS solved the issue of creating dynamic web applications with HTML and eliminates unnecessary complex coding
ReactJS has reusable components
ReactJS is made up of components with unique logic and controls. These components can be easily nested with other components to allow building more complex applications easily.
ReactJS has a set of rich developer tools
The React developer tools have been designed as Chrome and Firefox dev extensions and allow the inspection of React component hierarchies in the virtual DOM
Scope for testing the codes
ReactJS applications are quite easy to test. It offers a scope where the developer can test and debug their codes with the help of native tools.
React Cons?
Well, nothing much, but if you would think about it, you could consider that
- ReactJS mainly covers the UI layers of your web application, hence you need other technologies to get a complete tooling set for the development of your web project.
Setting up a new React Project
To create a fully functional React Project you need to install Node and create-react-app
After the installation, you can use the code below to create a new React Application. NB: In the code below my-react-app is the name of the new react application (so you can use anything)
//use this to create a new react app
npx create-react-app my-react-app
//Use the code below enter the directory of the app created
cd my-react-project
//use the code below to start the application
npm start
Once the initial project compilation is done, a new browser window will open and display the URL eg http://localhost:3000/. It also displays the placeholder content.
NB
ReactJS Project Structure
The React project structure can vary based on individual preferences and the specific requirements of the project. However, there's a common project structure that highlights the purpose of each directory or file:
src/: This directory contains the main source code of your React application.
index.js: The entry point of the application where the React components are rendered into the DOM.
App.js: The main component that serves as the root of the application.
components/: This directory holds reusable components that can be used throughout the application.
pages/: This directory contains components that represent individual pages or views of your application.
styles/: CSS or styling-related files specific to your components or pages.
services/: Utility functions, API clients, or service modules used by the application.
assets/: Static files such as images, fonts, or other resources used in the application.
public/: This directory contains static files that will be served by the web server.
index.html: The main HTML file where the React application is injected.
favicon.ico: The icon that appears in the browser's tab for your application.
node_modules/: This directory contains the installed dependencies managed by npm or Yarn.
package.json: This file defines the project configuration, dependencies, scripts, and other metadata.
.gitignore: This file specifies the files and directories that should be ignored by Git version control.
README.md: A file containing important information and instructions about the project.
Other configuration files: Various configuration files may be present based on project needs, such as webpack.config.js, babel.config.js, .eslintrc, etc. These files handle build configurations, transpilation, linting rules, and more.
It's important to note that this is a simplified structure, and the organization may vary depending on project complexity and individual preferences. The main goal is to keep the codebase organized and modular, separating concerns such as components, styles, services, and assets for better maintainability and scalability.
Commonly used npm commands in ReactJS
Sure! Here are some commonly used npm commands in React that are useful for beginners:
npm init: Initializes a new npm package in your project directory. It creates a
package.json
file that holds project metadata and manages dependencies.npm install: Installs all the dependencies listed in the
package.json
file. Runningnpm install
without any package name will install all dependencies.npm install: Installs a specific package and adds it as a dependency to your project. For example,
npm install react
installs the React package.npm uninstall: Removes a specific package from your project's dependencies. For example,
npm uninstall react
removes the React package.npm start: Starts the development server and runs your React application. This command is typically used to launch the application during development. It looks for a script called
"start"
in thescripts
section of yourpackage.json
file.npm run build: Builds your React application for production. It generates optimized and minified files ready for deployment. This command looks for a script called
"build"
in thescripts
section of yourpackage.json
file.npm run: Runs a custom script defined in the
scripts
section of yourpackage.json
file. You can create custom scripts for tasks like running tests, linting, formatting, etc.npm update: Updates the installed packages to their latest versions based on the version range specified in the
package.json
file.npm search: Searches the npm registry for packages related to the specified name.
npm info: Displays information about a specific package, including the latest version, description, author, and more.
npm list: Lists all the installed packages and their versions in a tree-like structure.
These are just a few examples of npm commands that are commonly used in React development. As you progress and explore more tools and libraries, you'll come across additional npm commands specific to those packages.
Essential aspects of ReactJS in conclusion
React Components: Imagine React components as building blocks of a website or app. Each component represents a specific part of the user interface, like a button, a header, or a form. Components can be reused throughout the project, making development faster and more organized.
JSX: JSX is a way to write code that combines JavaScript and HTML. It's like writing HTML tags inside your JavaScript code. JSX makes it easier to describe how your components should look, making your code more readable and expressive.
State and Props: Think of "state" as a component's memory. It holds data that can change, like the number of likes on a post. "Props" are like messages passed between components. They allow you to send data from a parent component to its children. For example, a parent component can pass the username to a child component using props.
Rendering: Rendering means showing your React components on the screen. You use the
ReactDOM.render()
function to tell React which component to render and where to put it in the web page.Event Handling: Events are actions that happen on your website, like a button click or a form submission. You can write event handlers, which are functions that run when an event occurs. Event handlers allow your React components to respond to user actions.
Component Lifecycle: Components have a lifecycle, just like a living thing. They are born (mounted), they can change (update), and they can die (unmount). React provides special methods that you can use at different stages of a component's life. These methods allow you to perform actions like fetching data when a component is first shown or cleaning up resources when a component is removed.
State Management: As your app grows, managing the state becomes important. When a component's state changes, React automatically updates the user interface. For more complex state management, you can use tools like Redux or React Context to keep track of data across different components.
Component Communication: Components often need to share data or communicate with each other. This is done by passing data from a parent component to its children using props. If multiple components at different levels need to share data, you can lift the state up to a common parent component.
Styling: Styling in React can be done using CSS classes, inline styles, or CSS-in-JS libraries. CSS classes allow you to apply pre-defined styles, while inline styles let you write CSS directly in your JSX code. CSS-in-JS libraries provide more advanced styling techniques, where you write CSS using JavaScript.
React Developer Tools: React Developer Tools are browser extensions that help you inspect and debug your React components. They provide a helpful interface to understand the component hierarchy, inspect props and state, and track component updates.
Project Setup and Tooling: To start a React project, you need to set up the development environment. This involves installing dependencies, configuring build tools like Babel and Webpack, and using a development server to preview your app locally.
Resources and Community: There are many resources available to learn React, including official documentation, tutorials, and online communities. It's important to explore these resources, ask questions, and engage with the React community for support and learning opportunities.
By understanding these essential aspects of React, you'll be able to create interactive and dynamic user interfaces for your web applications. Remember, practice and experimentation are key to becoming proficient in React.JS
Enjoy the journey of learning and building with React!
Feel free to meet me at My Online Portfolio and I am open to your questions as well collaborations.
Subscribe to my newsletter
Read articles from Olumide Adeola directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
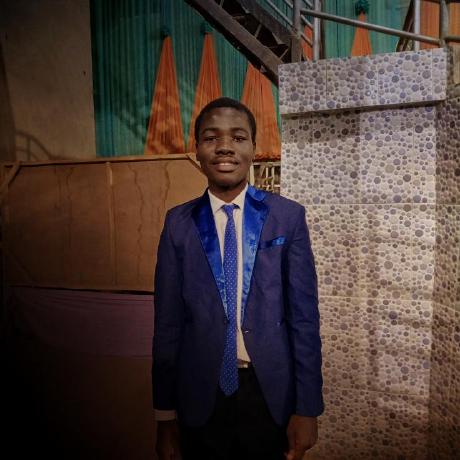
Olumide Adeola
Olumide Adeola
Classical | Health | Academics | Services et Solutions | Finance | Agriculture | Technology This is what my passion revolves round....