Methods and Its Types
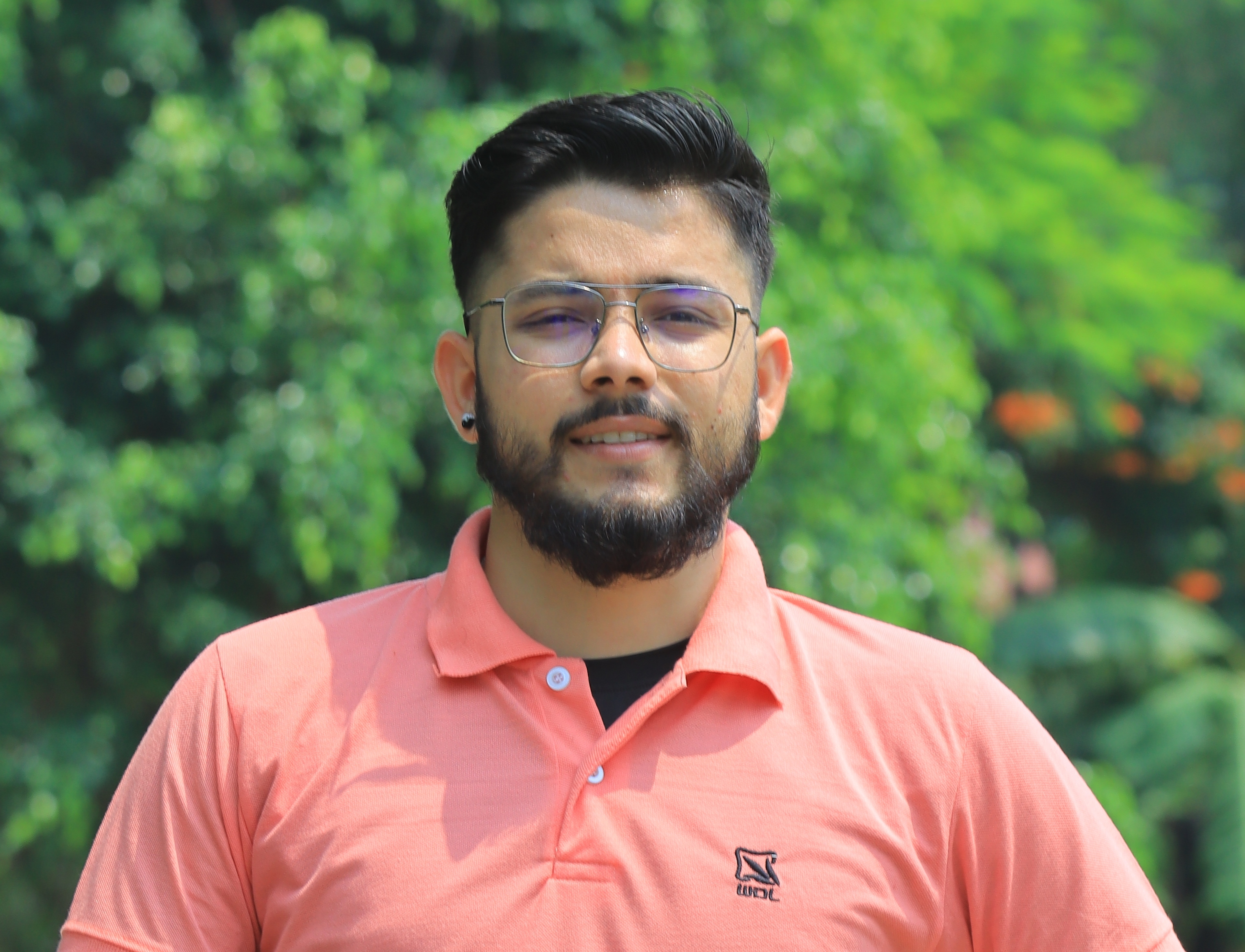
Table of contents
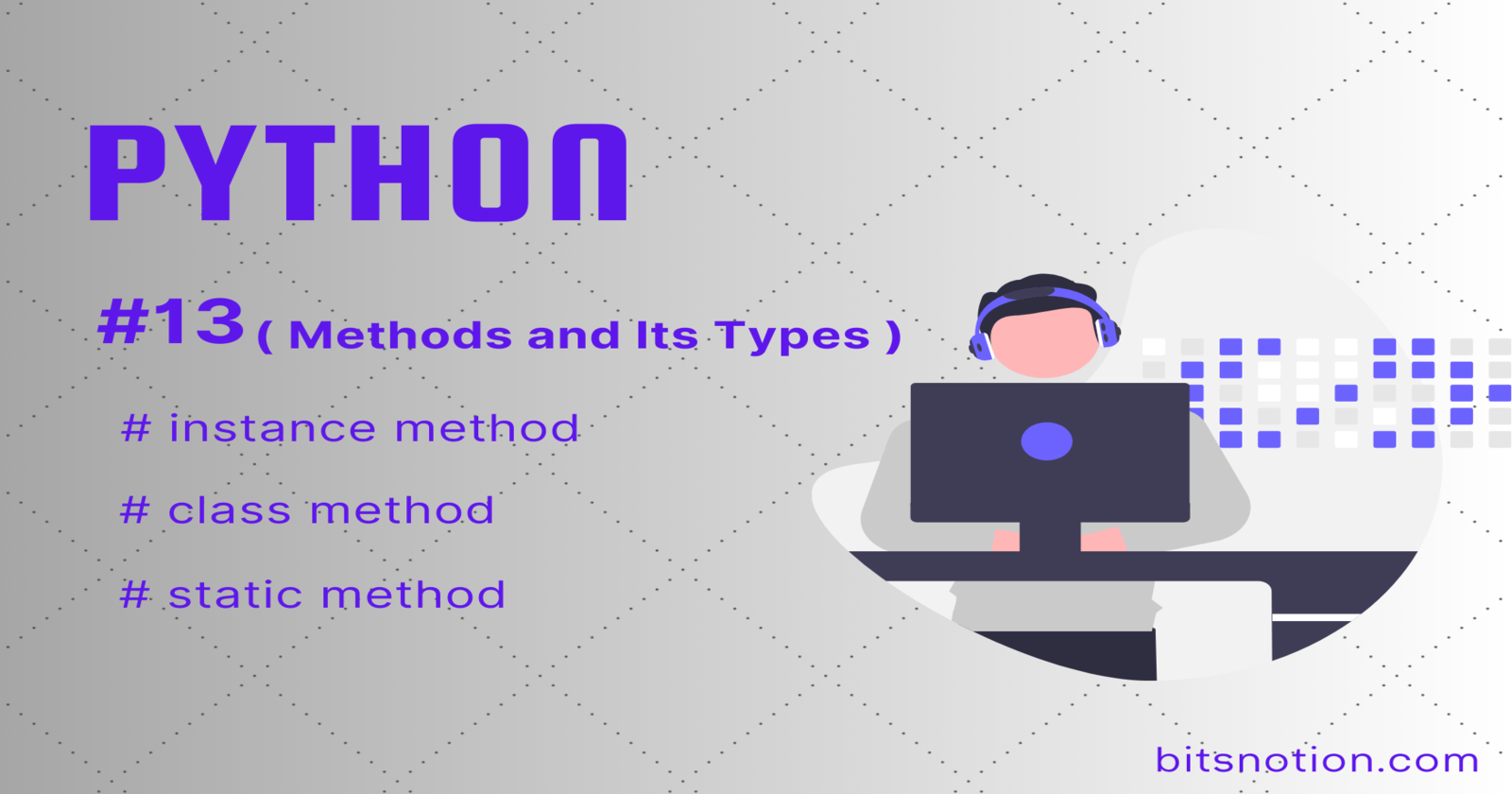
In object-oriented programming, Python provides different types of methods to define the behavior of classes. Three commonly used methods are instance methods, class methods, and static methods. Such methods help us to create more organized and efficient code structures in Python.
Instance Method
Instance methods are the most common type of method in Python classes.
They are associated with objects (instances) of a class and are defined within the class definition.
Instance methods take the
self
parameter, which represents the instance itself.They can access and modify instance variables and invoke other instance methods of the same class. To define an instance method, we use the
def
keyword.
class Person:
def instance_method(self, name, age):
# Access instance variables
self.name = name
self.age = age
# Creating an instance of MyClass
obj = Person()
# Calling the instance method
obj.instance_method("Nirmal","23")
print(obj.name, obj.age)# Output = Nirmal 23
In the example above, instance_method()
is an instance method that takes two arguments. It accesses the instance variables arg1
and arg2
using the self
parameter performs some operations and modifies the instance variables accordingly.
Class Method
They can be called on the class itself to perform operations rather than instances.
Class methods take the
cls
parameter, which represents the class itself.We use the
@classmethod
decorator to define a class method.Class methods are often used for alternative constructors.
class MyClass:
class_variable = "Hello, World!"
@classmethod
def class_method(cls):
print("This is a class method.")
print("Accessing class variable:", cls.class_variable)
# Calling class method
MyClass.class_method()
Static Method
If we have nothing to do with instance and class then we use static method.
They are defined within the class definition using the
@staticmethod
decorator.Static methods are not bound to the instance or the class, making them independent and self-contained.
class MyClass:
@staticmethod
def static_method():
print("This is a static method.")
# Calling the static method
MyClass.static_method()
Output:
This is a static method.
Subscribe to my newsletter
Read articles from Nirmal Pandey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
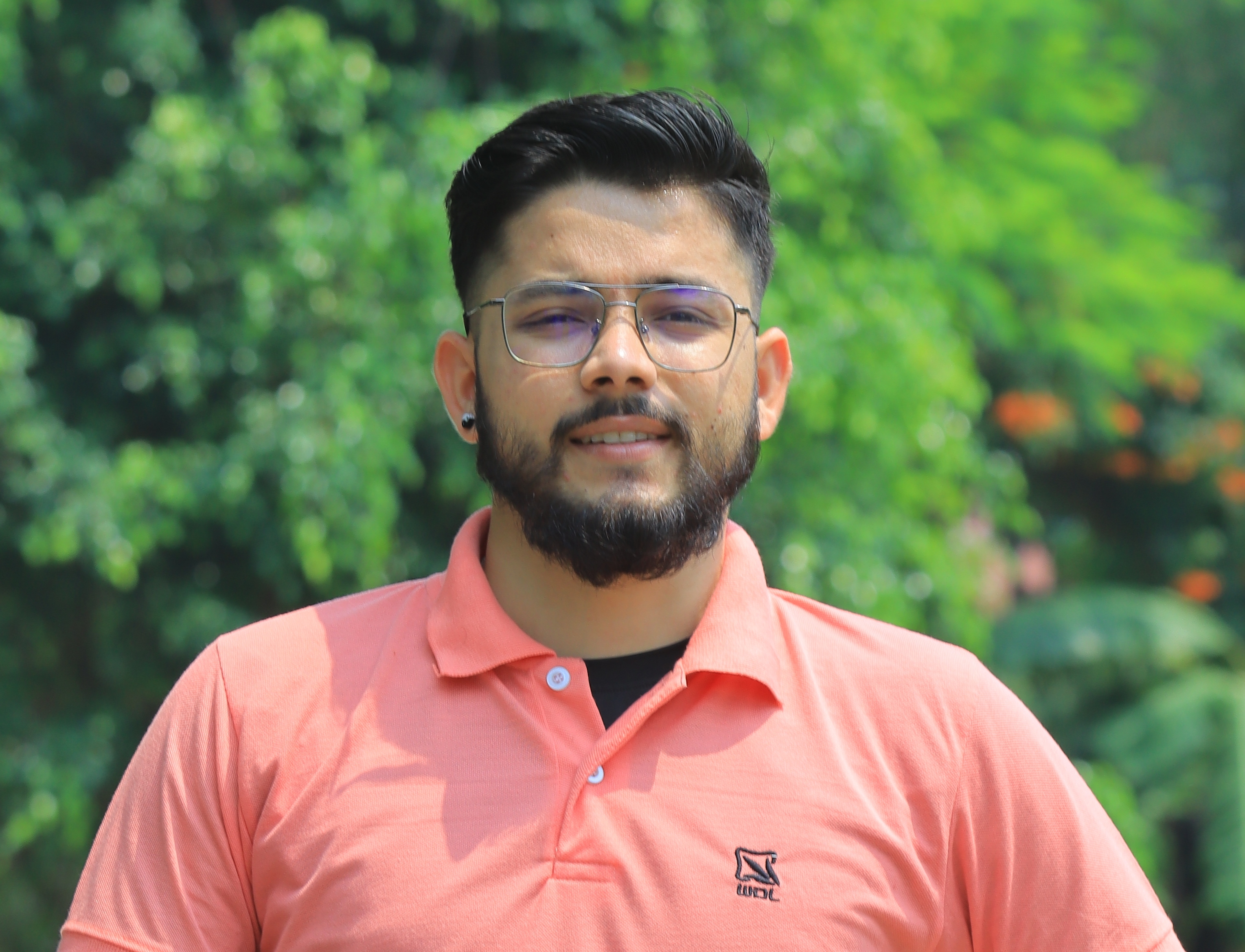
Nirmal Pandey
Nirmal Pandey
๐ Hi there, I am Nirmal. I am a former software engineer with a keen interest in data science and analytics domains. Besides, I love to contribute to open source and help others to understand various tech stuffs.