Understanding Reinforcement Learning (RL)

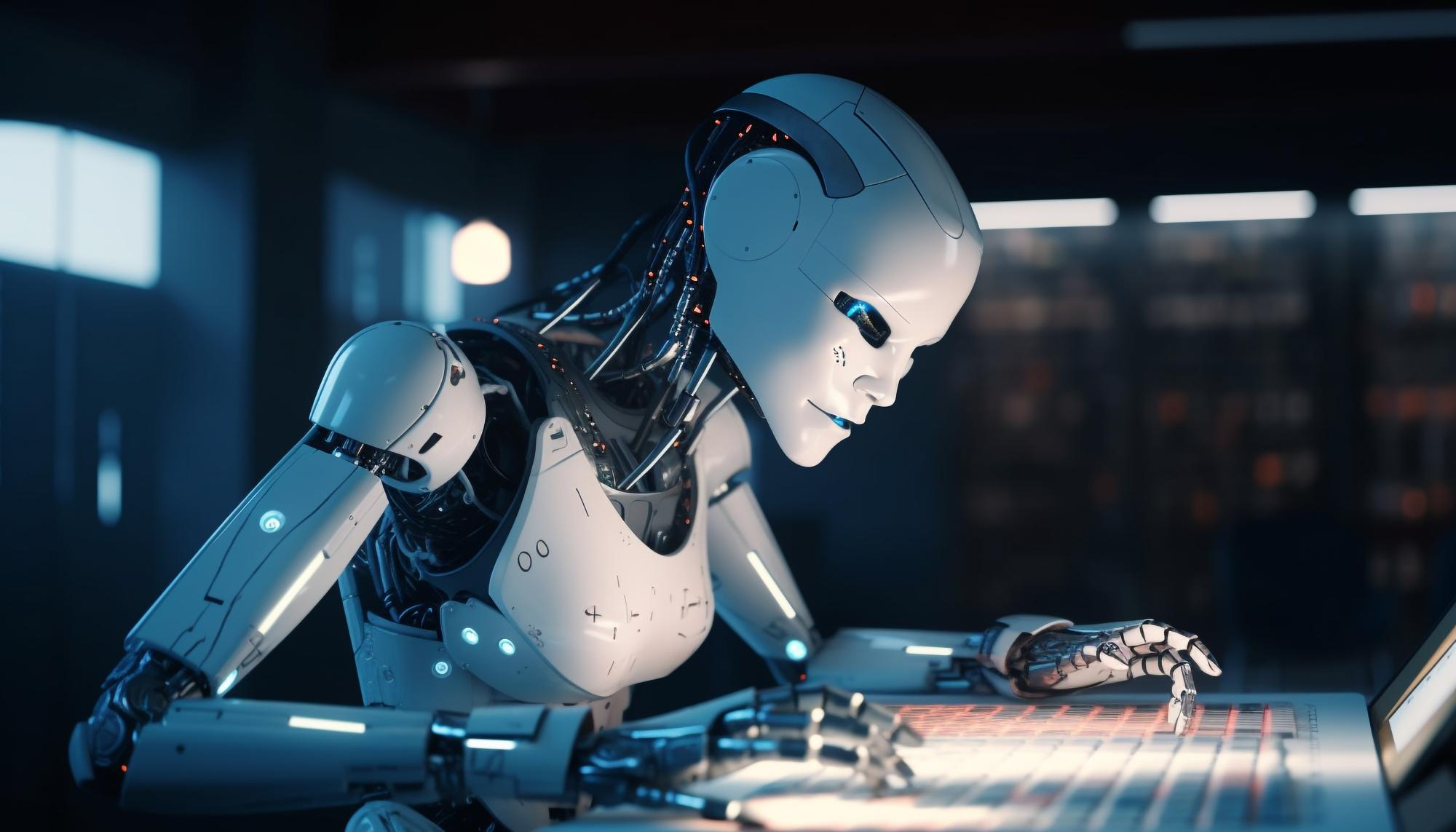
Introduction
Reinforcement Learning (RL) is a subfield of machine learning that focuses on how agents can learn to make sequential decisions by interacting with an environment. It has gained significant attention in recent years due to its potential applications in various domains, such as robotics, game playing, autonomous driving, and recommendation systems. In this blog, we will delve into the fundamentals of Reinforcement Learning and explore Reinforcement Learning from Human Feedback (RLHF), a technique that leverages human expertise to improve the learning process. Additionally, we will provide Python code samples and present a case study to illustrate these concepts.
Reinforcement Learning Fundamentals
To understand Reinforcement Learning, we need to grasp its fundamental concepts.
Markov Decision Process (MDP): In RL, we model the interaction between an agent and an environment as a Markov Decision Process (MDP). An MDP consists of states, actions, rewards, and transition probabilities. The agent takes actions in specific states, receives rewards from the environment, and transitions to new states based on the action taken.
Agent, Environment, and State: The agent interacts with the environment by observing its current state and taking actions. The environment responds to the agent's actions by transitioning to a new state. The interaction continues until the agent achieves its goal or reaches a termination condition.
Actions, Rewards, and Policies: Actions are the decisions taken by the agent in each state. Rewards are numerical values that provide feedback on the agent's actions. The agent's goal is to learn a policy, which is a mapping from states to actions, that maximizes the cumulative rewards obtained over time.
Value Functions and Q-Learning: Value functions estimate the expected cumulative reward an agent can achieve from a particular state or state-action pair. Q-Learning is a popular RL algorithm that learns the optimal Q-values (state-action values) using an iterative update process. By updating the Q-values based on the rewards received and the estimated future rewards, the agent gradually improves its decision-making abilities.
Exploration vs. Exploitation Trade-off: In RL, the agent faces a trade-off between exploration and exploitation. Exploration involves trying out new actions to gather more information about the environment and potentially discover better strategies. Exploitation involves leveraging the current knowledge to choose actions that maximize rewards. Striking the right balance between exploration and exploitation is crucial for effective learning.
Reinforcement Learning from Human Feedback (RLHF)
In some scenarios, learning solely through trial and error can be challenging or time-consuming. RLHF provides a way to leverage human expertise to guide the learning process, reducing exploration time and accelerating the agent's progress.
Need for Human Feedback in RL: RLHF addresses situations where it is impractical or too costly to rely solely on exploration to learn optimal policies. Human feedback can provide valuable guidance to the agent, enabling faster convergence and better performance.
Types of Human Feedback: Human feedback can come in different forms, including demonstrations, rankings, and comparisons.
Demonstrations: Demonstrations involve providing example trajectories or sequences of states and actions that represent good behavior. By learning from expert demonstrations, the agent can mimic the desired behavior and reduce the exploration required to discover it.
Rankings: Rankings involve comparing different trajectories or actions based on their quality. For example, an expert might rank different gameplay sequences in terms of performance or reward obtained. Rankings provide a relative comparison that can guide the agent's learning process.
Comparisons: Comparisons involve directly comparing pairs of trajectories or actions to indicate which one is better. This form of feedback helps the agent understand the relative merits of different choices and refine its decision-making.
Dataset Aggregation (DAgger): DAgger is an RLHF algorithm that combines expert demonstrations with agent experience to create a training dataset. It starts with initial training using expert demonstrations and then uses the agent to collect new data. The expert's policy is used to label the agent's new data, which is then added to the training set. This iterative process continues, creating a dataset that includes both expert and agent trajectories.
Comparison with Traditional RL: RLHF approaches, such as DAgger, offer several advantages over traditional RL methods:
Faster Convergence: By leveraging expert knowledge, RLHF algorithms can converge more quickly compared to pure RL, where the agent learns solely through exploration.
Improved Performance: Human feedback provides valuable guidance to the learning process, leading to higher performance and more effective decision-making.
Code Implementation
Let's explore some Python code examples to understand the implementation of RL and RLHF algorithms. Setting up the RL Environment: We can use OpenAI Gym, a popular RL library, to set up a simple environment. For example, we'll use the "CartPole-v1" environment, where the agent balances a pole on a cart.
import gym
env = gym.make('CartPole-v1')
Implementing Q-Learning Algorithm
Q-Learning is a well-known RL algorithm for environments with discrete action spaces. Here's a simplified implementation:
import numpy as np
# Initialize Q-values arbitrarily
Q = np.zeros((env.observation_space.n, env.action_space.n))
# Q-Learning parameters
alpha = 0.5 # Learning rate
gamma = 0.9 # Discount factor
epsilon = 0.1 # Exploration rate
# Q-Learning algorithm
for episode in range(num_episodes):
state = env.reset()
done = False
while not done:
if np.random.rand() < epsilon:
action = env.action_space.sample() # Exploration
else:
action = np.argmax(Q[state, :]) # Exploitation
next_state, reward, done, _ = env.step(action)
Q[state, action] += alpha * (reward + gamma * np.max(Q[next_state, :]) - Q[state, action])
state = next_state
Integrating RLHF into Q-Learning
To incorporate RLHF into Q-Learning, we can use the DAgger algorithm. Here's an example:
# Expert demonstrations
expert_trajectories = [expert_agent.play_episode() for _ in range(num_expert_episodes)]
# Initialize training dataset
training_data = expert_trajectories
# DAgger algorithm
for iteration in range(num_iterations):
# Train agent on current dataset
agent.train(training_data)
# Generate new trajectories from the agent
agent_trajectories = [agent.play_episode() for _ in range(num_agent_episodes)]
# Aggregate the expert and agent trajectories
training_data = expert_trajectories + agent_trajectories
Training an AI to Play a Game
Let's consider a case study where we train an AI agent to play a maze game. The agent needs to navigate through the maze, collect rewards, and avoid obstacles.
Problem Statement: Game Environment: We have a maze environment represented as a grid, with the agent starting at a specific position. The agent can move in four directions (up, down, left, right) and receives rewards for collecting items in the maze. The goal is to train the agent to navigate the maze efficiently and maximize the cumulative rewards.
RL Approach with Q-Learning: We can apply Q-Learning to train the agent to learn an optimal policy to navigate the maze. The agent iteratively updates Q-values based on the observed rewards and the expected future rewards.
In corporating RLHF for Improved Performance: To improve the agent's learning process, we can incorporate RLHF techniques. For example, we can start with expert demonstrations showing how to navigate the maze efficiently. The agent can then learn from these demonstrations and gradually explore and improve its strategy.
Evaluation and Results: To evaluate the performance of the RL agent trained with RLHF, we can measure metrics such as the average reward obtained, the success rate in reaching the goal, and the convergence time compared to the agent trained solely through Q-Learning. Through analysis and comparison, we can assess the effectiveness of RLHF in accelerating learning and achieving better performance.
Conclusion
Reinforcement Learning offers a powerful framework for agents to learn optimal decision-making policies through interaction with an environment. RLHF, such as DAgger, provides a means to incorporate human expertise and guidance, resulting in faster convergence and improved performance. Through Python code examples and a case study, we have explored the fundamentals of RL and demonstrated the integration of RLHF techniques. However, this blog only scratches the surface of RL and RLHF, and further exploration and experimentation are essential to deepening your understanding and expertise in this exciting field.
Refer to the below resources to explore further:
Subscribe to my newsletter
Read articles from Nitin Agarwal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Nitin Agarwal
Nitin Agarwal
Data Scientist with 12 years of industry experience.