Simplifying 300 Python Programs on the list- Questions 81-90.
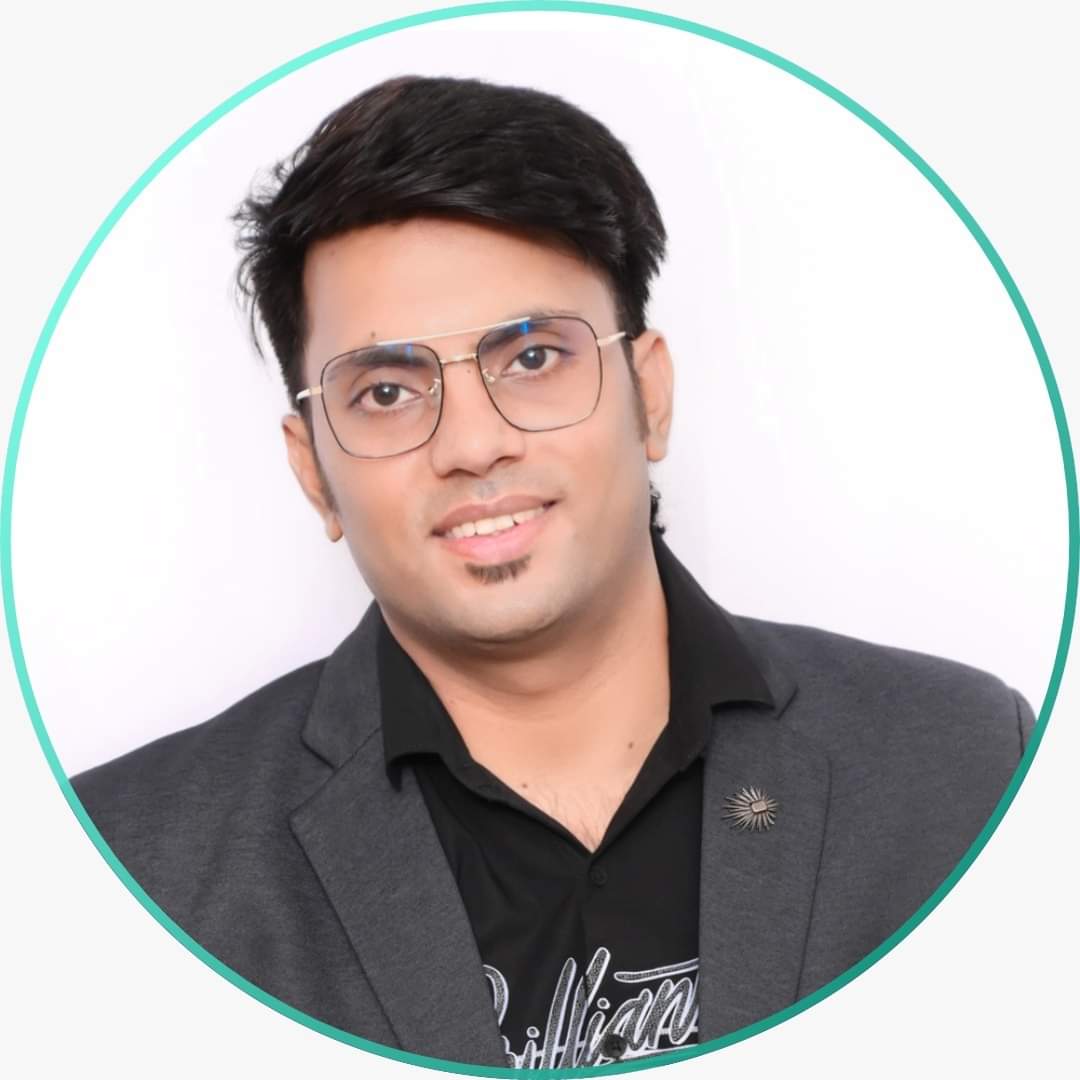
Table of contents
- Q81-Write a Python program to check if a nested list is a subset of another nested list.
- Q82- Write a Python program to count the number of sublists that contain a particular element.
- Q83- Write a Python program to count the number of unique sublists within a given list.
- Q84-Write a Python program to sort each sublist of strings in a given list of lists.
- Q85-Write a Python program to scramble the letters of a string in a given list.
- Q86- Write a Python program to find the maximum and minimum values in a given heterogeneous list.
- Q87- Write a Python program to extract common index elements from more than one given list.
- Q88- Write a Python program to sort a given matrix in ascending order according to the sum of its rows?
- Q89-Write a Python program to extract specified size of strings from a give list of string values?
- Q90-Write a Python program to extract specified number of elements from a given list, which follows each other continuously.
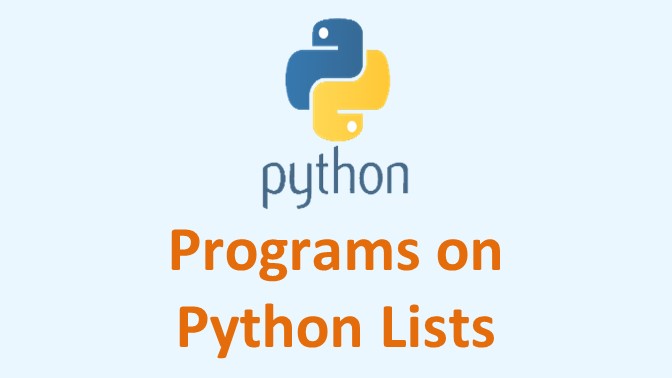
"Embark on a transformative journey as I dive deep into the realm of Python lists. Over the next 90 days, join me as I unravel the secrets of the list.
Q81-Write a Python program to check if a nested list is a subset of another nested list.
for example, consider the situation below.
Original list:
[[1, 3], [5, 7], [9, 11], [13, 15, 17]]
[[1, 3], [13, 15, 17]]
If one of the said lists is a subset of another.:
True
Original list:
[[[1, 2], [2, 3]], [[3, 4], [5, 6]]]
[[[3, 4], [5, 6]]]
If one of the said lists is a subset of another.:
True
Original list:
[[[1, 2], [2, 3]], [[3, 4], [5, 7]]]
[[[3, 4], [5, 6]]]
If one of the said lists is a subset of another.:
False
to do this task, we need to take the list and iterate it over the list and check if it is part of that or not, if yes set variable is_sublist==True.
ls1=[[1, 3], [5, 7], [9, 11], [13, 15, 17]]
ls2=[[1, 3], [13, 15, 17]]
is_sublist=False
for i in ls2:
if i in ls1:
is_sublist=True
break
if is_sublist==True:
print("True")
else:
print("False")
Q82- Write a Python program to count the number of sublists that contain a particular element.
for example, consider below:
Original list:
[[1, 3], [5, 7], [1, 11], [1, 15, 7]]
Count 1 in the said list:
3
Count 7 in the said list:
2
Original list:
[['A', 'B'], ['A', 'C'], ['A', 'D', 'E'], ['B', 'C', 'D']]
Count 'A' in the said list:
3
Count 'E' in the said list:
1
for doing this task, we can use the count method available in the list, to count occurrences of any element in the list.
but first, we can transform the lists of lists into a single list, for this, we can run a loop and append all the items of the list into an empty list.
ls=[['A', 'B'], ['A', 'C'], ['A', 'D', 'E'], ['B', 'C', 'D']]
new_ls=[]
for i in ls:
if isinstance(i,list):
for j in i:
new_ls.append(j)
else:
new_ls.append(i)
new_ls.count('E')
Result- 1
Q83- Write a Python program to count the number of unique sublists within a given list.
for example:
Original list:
[[1, 3], [5, 7], [1, 3], [13, 15, 17], [5, 7], [9, 11]]
A number of unique lists of the said list:
{(1, 3): 2, (5, 7): 2, (13, 15, 17): 1, (9, 11): 1}
Original list:
[['green', 'orange'], ['black'], ['green', 'orange'], ['white']]
A number of unique lists of the said list:
{('green', 'orange'): 2, ('black',): 1, ('white',): 1}
that means we need to convert the given list into a dictionary and count the sublists.
this one we have already done in the earlier examples, let's see below.
take an empty dictionary and append all the items as keys with their count as value.
ls=[[1, 3], [5, 7], [1, 3], [13, 15, 17], [5, 7], [9, 11]]
new_ls=[]
for i in ls:
i=tuple(i)
new_ls.append(i)
d={}
k=d.keys()
for i in new_ls:
if i in k:
d[i]=d[i]+1
else:
d[i]=1
print(d)
NOTE: we had to convert all the sublists to tuples, because in the dictionary when we will insert lists into the dictionary, it will not accept them, in the dictionary the key can be only immutable(a tuple is an immutable data structure)
Result- {(1, 3): 2, (5, 7): 2, (13, 15, 17): 1, (9, 11): 1}
Q84-Write a Python program to sort each sublist of strings in a given list of lists.
for example-
Original list:
[[2], [0], [1, 3], [0, 7], [9, 11], [13, 15, 17]]
Sort the list of lists by length and value:
[[0], [2], [0, 7], [1, 3], [9, 11], [13, 15, 17]]
Q85-Write a Python program to scramble the letters of a string in a given list.
for example:
Original list:
['Python', 'list', 'exercises', 'practice', 'solution']
After scrambling the letters of the strings of the said list:
['tnPhyo', 'tlis', 'ecrsseiex', 'ccpitear', 'noiltuos']
in this example, we can interchange the position of the letters within the string for doing that we can use the shuffle function from a random module.
or we can reverse the string as well to interchange the positions of the string.
ls=['Python', 'list', 'exercises', 'practice', 'solution']
new_ls=[]
for i in ls:
i=i[::-1]
new_ls.append(i)
print(new_ls)
Result: ['oPhynt', 'ltis', 'esecseixr', 'itceacrp', 'isotnluo']
OR use the shuffle function as below.
from random import shuffle
ls=['Python', 'list', 'exercises', 'practice', 'solution']
new_ls=[]
for i in ls:
i=list(i)
shuffle(i)
st="".join(i)
new_ls.append(st)
print(new_ls)
Result: ['oPhynt', 'ltis', 'esecseixr', 'itceacrp', 'isotnluo']
Q86- Write a Python program to find the maximum and minimum values in a given heterogeneous list.
for example:
Original list:
['Python', 3, 2, 4, 5, 'version']
Maximum and Minimum values in the said list:
(5, 2)
in this question, we can iterate a loop over given list and check if the item is a integer append it to a another empty list and then finding max and min value.
ls=['Python', 3, 2, 4, 5, 'version']
new_ls=[]
for i in ls:
if isinstance(i,int):
new_ls.append(i)
maximum=max(new_ls)
minimum=min(new_ls)
print(maximum)
print(minimum)
OR : we can use the list comprehension method to do this.
#OR
ls=['Python', 3, 2, 4, 5, 'version']
maximum=max([i for i in ls if isinstance(i,int)])
minimum=min([i for i in ls if isinstance(i,int)])
print(minimum)
print(maximum)
Q87- Write a Python program to extract common index elements from more than one given list.
for example:
Original lists:
[1, 1, 3, 4, 5, 6, 7]
[0, 1, 2, 3, 4, 5, 7]
[0, 1, 2, 3, 4, 5, 7]
Common index elements of the said lists:
[1, 7]
for finding the common index elements, we need to take each item from each list and check them if they are same.
if they are same then appeding them in to list.
as we already know that for selecting each item from the each list we can use zip function, that can provide each item from the each list within the each iteration.
ls1=[1, 1, 3, 4, 5, 6, 7]
ls2=[0, 1, 2, 3, 4, 5, 7]
ls3=[0, 1, 2, 3, 4, 5, 7]
new_ls=[]
for i,j,k in zip(ls1,ls2,ls3):
if i==j==k:
new_ls.append(i)
print(new_ls)
Q88- Write a Python program to sort a given matrix in ascending order according to the sum of its rows?
for example:
Original Matrix:
[[1, 2, 3], [2, 4, 5], [1, 1, 1]]
Sort the said matrix in ascending order according to the sum of its rows
[[1, 1, 1], [1, 2, 3], [2, 4, 5]]
Original Matrix:
[[1, 2, 3], [-2, 4, -5], [1, -1, 1]]
Sort the said matrix in ascending order according to the sum of its rows
[[-2, 4, -5], [1, -1, 1], [1, 2, 3]]
for doing this, we can use sorted function and by using sorted function we can use key=sum.
ls=[[1, 2, 3], [-2, 4, -5], [1, -1, 1]]
new_ls=sorted(ls,key=sum)
print(new_ls)
Result: [[-2, 4, -5], [1, -1, 1], [1, 2, 3]]
Q89-Write a Python program to extract specified size of strings from a give list of string values?
for example:
Original list:
['Python', 'list', 'exercises', 'practice', 'solution']
length of the string to extract:
8
After extracting strings of specified length from the said list:
['practice', 'solution']
this one we can do it by calculating the length of the string by iterating over a list.
ls=['Python', 'list', 'exercises', 'practice', 'solution']
new_ls=[ i for i in ls if len(i)==8]
print(new_ls)
Reulst=['practice', 'solution']
Q90-Write a Python program to extract specified number of elements from a given list, which follows each other continuously.
here, we need to identify the number and it's occurances.
for example in a given list- [1, 1, 3, 4, 4, 5, 6, 7]
find 2 such numbers which are occured two times- [1,4]
for doing this we can use groupby function thatfrom itertools that we have already used for grouping the numbers from a list if it has proceding duplicates.
groupby function basically returns the item from the list as a key along with its frequency of duplications in the proceding index.
for example: [1, 1, 3, 4, 4, 5, 6, 7], 1 is repeated and 4 is repeated.
ls=[1, 1, 3, 4, 4, 5, 6, 7]
for key,value in groupby(ls):
print("key:",key,end=" ")
print("value:",list(value))
#here the value contain the multiple items, hence it will have to
#be converted in the list to represent it.
Result:
key: 1 value: [1, 1]
key: 3 value: [3]
key: 4 value: [4, 4]
key: 5 value: [5]
key: 6 value: [6]
key: 7 value: [7]
now we can use the groupby function to seperate duplicates in the new list and then
from itertools import groupby
ls=[1, 1, 3, 4, 4, 5, 6, 7]
new_ls=[]
new_ls1=[]
for item,group in groupby(ls):
group=list(group)
if len(group)==2:
new_ls.append(group)
for i in new_ls:
new_ls1.append(i[0])
print(new_ls1)
Result=[1,4]
take another example, to extract a number which is repeated 4 times in the list- [0, 1, 2, 3, 4, 4, 4, 4, 5, 7]
from itertools import groupby
ls=[0, 1, 2, 3, 4, 4, 4, 4, 5, 7]
new_ls=[]
new_ls1=[]
for item,group in groupby(ls):
group=list(group)
if len(group)==4:
new_ls.append(group)
for i in new_ls:
new_ls1.append(i[0])
print(new_ls1)
Result- [4]
Subscribe to my newsletter
Read articles from Kapil Manwani directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
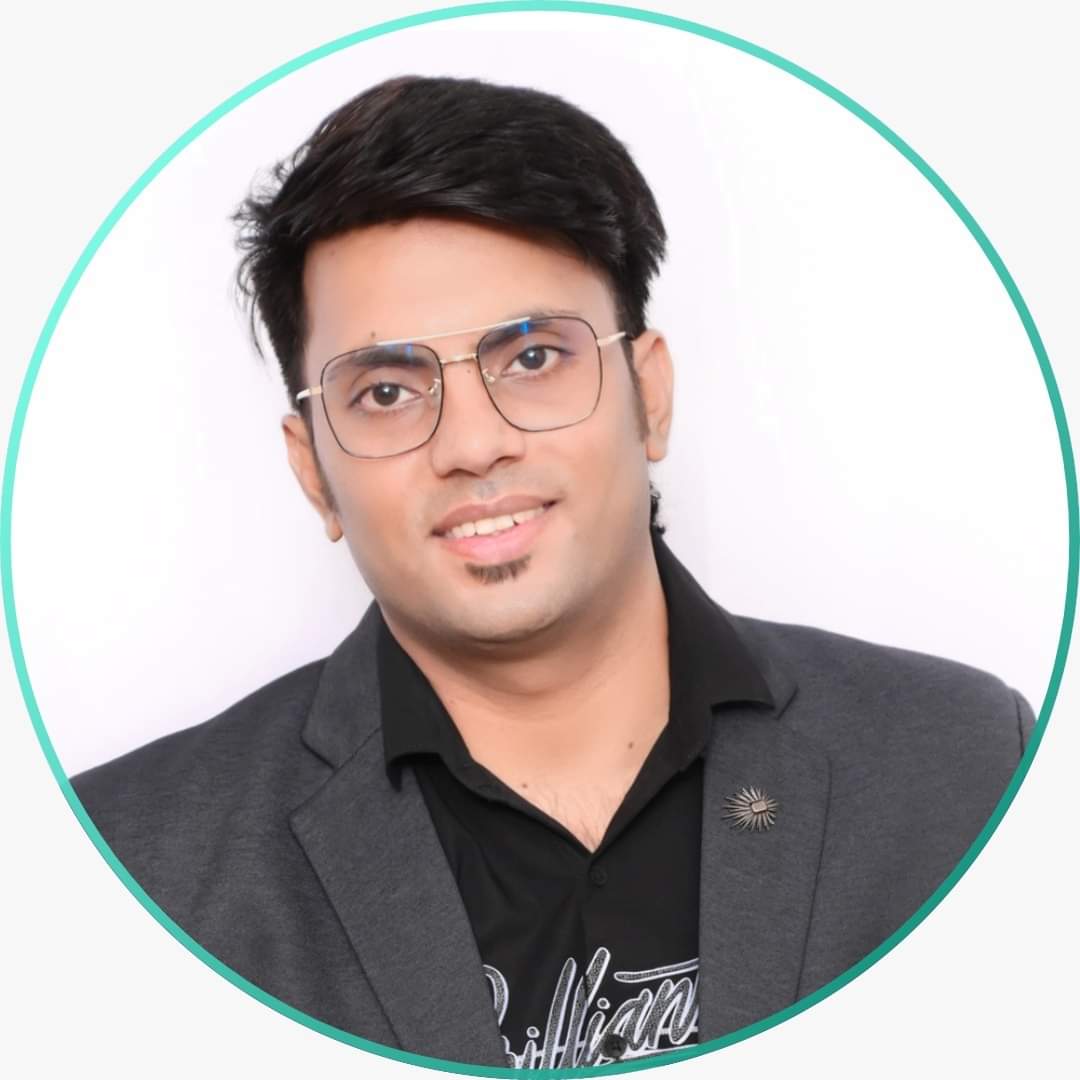
Kapil Manwani
Kapil Manwani
Proficient in a variety of DevOps technologies, including AWS, Linux, Python, Shell Scripting, Docker, Terraform, and Computer Networking. I have a strong ability to troubleshoot issues.