Fetching Data from API in React!!
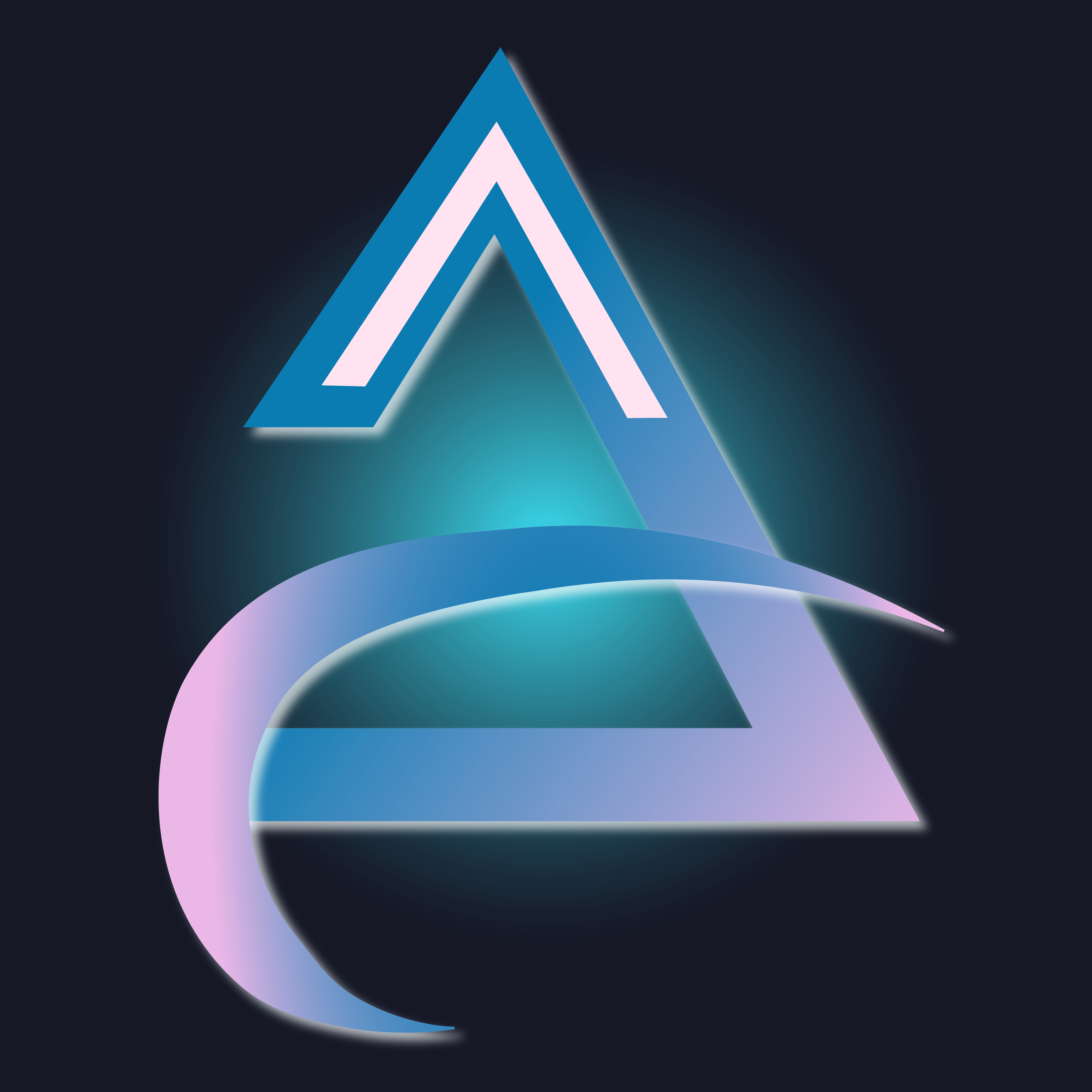
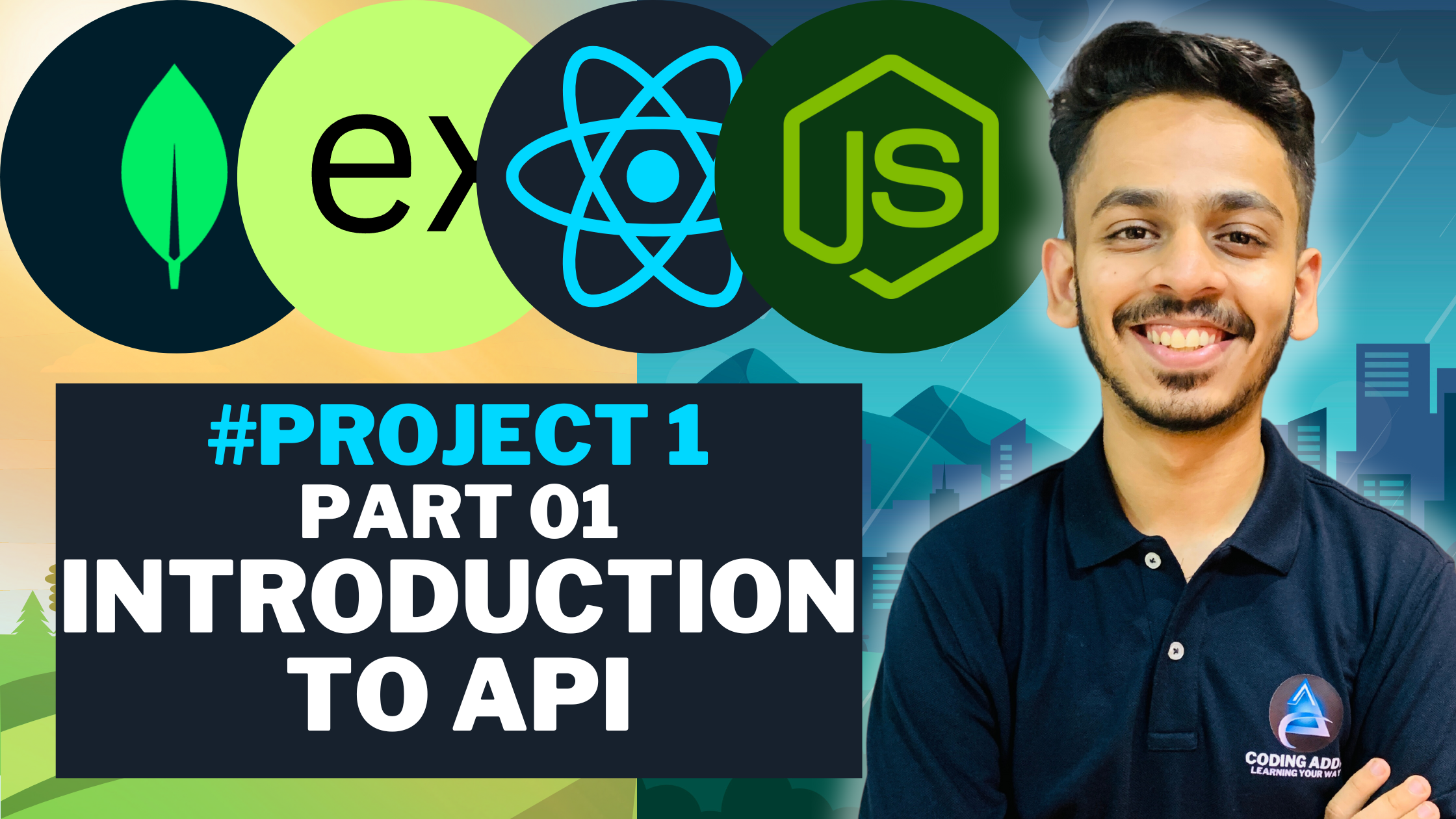
What is an API?
An API, short for Application Programming Interface, is a set of rules, protocols, and tools that allows different software applications to communicate with each other. It defines the methods and formats for data exchange between systems, enabling seamless interaction and integration. In simpler terms, an API acts as a bridge between two software applications, allowing them to interact and share information. It provides a standardized way for applications to request and exchange data or functionality without needing to understand the underlying implementation details. APIs are widely used in various contexts, including web development, mobile app development, cloud computing, IoT (Internet of Things), and more. They enable developers to leverage existing services, functionalities, or data sources provided by other applications or systems without having to build everything from scratch. This promotes reusability, efficiency, and collaboration among different software components or even between different organizations.
Types of API?
There are several types of APIs available, each catering to different needs and serving specific purposes. Here are some commonly encountered types of APIs:
RESTful APIs: REST (
Representational State Transfer
) APIs are based on a set of architectural principles that use HTTP methods (such asGET, POST, PUT, DELETE
) to perform operations on resources. They typically communicate using JSON or XML for data exchange and are widely used for web services and web APIs.SOAP APIs: SOAP (
Simple Object Access Protocol
) APIs are based on XML and use the SOAP protocol for communication. They follow a more rigid and structured approach compared to RESTful APIs and often rely on XML-based request and response messages. SOAP APIs are commonly used in enterprise-level systems and integrations.GraphQL APIs: GraphQL is a
query language
and runtime for APIs. It allows clients to define the structure of the data they need, and the server responds with exactly that data. GraphQL APIs provide a flexible and efficient way to retrieve data and have gained popularity, especially in scenarios where fine-grained control over data retrieval is required.Web APIs: Web APIs, also known as
HTTP APIs
, are designed specifically for interaction with web applications. They provide a set of URLs (endpoints) that allow developers to access specific functionalities or retrieve data from a web-based service or application. Web APIs can be RESTful APIs, SOAP APIs, or other API types accessible over HTTP.Database APIs: Database APIs provide an interface to interact with databases, allowing developers to perform operations such as
querying, inserting, updating, or deleting data
. They provide a layer of abstraction and simplify the interaction with databases by providing methods or functions to access and manipulate data stored within the database system.Library or SDK APIs: Libraries and Software Development Kits (SDKs) often come with their own APIs that provide pre-defined
functions, methods, and classes
to simplify the integration and use of the library or SDK within an application. These APIs are specific to the library or SDK and typically serve a specific purpose, such as image processing, machine learning, or payment integration.External Service APIs: Many third-party services, such as
social media platforms
(e.g., Facebook, Twitter),payment gateways
(e.g., PayPal, Stripe), mapping services (e.g., Google Maps), orweather services
, provide APIs that allow developers to access their functionalities and data within their own applications.Operating System APIs: Operating system APIs provide developers with access to various functionalities and services offered by the underlying operating system. These APIs allow developers to interact with the file
system, network protocols, hardware devices, user interface components
, and other system-level features.
Importance of Data Retrieval from APIs
Data retrieval from APIs holds significant importance in various domains due to the following reasons:
Access to Real-Time and Updated Data: APIs provide a means to retrieve real-time data directly from the source. This ensures that the information obtained is up to date and reflects the most recent changes or additions. Industries such as finance, e-commerce, weather forecasting, and social media heavily rely on real-time data for accurate decision-making and providing timely services.
Data Aggregation and Integration: APIs allow businesses to aggregate data from multiple sources by fetching information from various APIs. This enables organizations to consolidate diverse data sets into a unified format, facilitating comprehensive analysis and insights. Integration of data from different APIs enables a holistic view of information, enhancing operational efficiency and decision-making capabilities.
Seamless Collaboration and Interoperability: APIs enable seamless communication and data exchange between different software applications, systems, or platforms. By retrieving data through APIs, organizations can integrate external services, leverage third-party functionalities, and establish interoperability between disparate systems. This promotes collaboration, enhances productivity, and facilitates the creation of innovative applications and services.
Scalability and Flexibility: APIs offer scalability and flexibility in accessing data. With well-designed APIs, businesses can easily scale their data retrieval operations as their requirements grow. APIs provide the flexibility to customize the data retrieved based on specific needs, allowing organizations to fetch only the relevant information instead of retrieving complete datasets. This optimized data retrieval approach improves efficiency and reduces unnecessary bandwidth and processing costs.
Enabling Mobile and Web Applications: APIs play a vital role in enabling the functionality of mobile apps and web applications. By fetching data from APIs, developers can integrate external services, retrieve dynamic content, and create interactive and feature-rich applications. APIs provide ready-made functionality, saving time and effort in developing complex features from scratch. This accelerates application development and enhances the overall user experience.
Integration with IoT and Emerging Technologies: As the Internet of Things (IoT) and emerging technologies like artificial intelligence (AI) and machine learning (ML) gain prominence, data retrieval from APIs becomes crucial. IoT devices can fetch data from APIs to facilitate real-time monitoring, control, and automation. AI and ML algorithms often rely on vast amounts of data, and APIs serve as a convenient source to access the necessary information for training and inference purposes.
Methods to Fetch Data from API in React?
In React, there are multiple methods and approaches to fetch data from an API. Here are three commonly used methods:
Using the Fetch API:
The Fetch API is a built-in browser API that provides a simple and straightforward way to make HTTP requests, including fetching data from APIs.
Here's an example of how to use the Fetch API to fetch data in React:import React, { useEffect, useState } from 'react'; function MyComponent() { const [data, setData] = useState([]); useEffect(() => { fetch('https://api.example.com/data') .then(response => response.json()) .then(data => setData(data)) .catch(error => console.error(error)); }, []); // Render the fetched data return ( <div> {data.map(item => ( <p key={item.id}>{item.name}</p> ))} </div> ); }
In this example, the
useEffect
hook is used to fetch the data from the API when the component mounts. Thefetch
function is called with the API URL, and the response is converted to JSON using the.json()
method. The fetched data is then stored in the component's state using thesetData
function.Using third-party libraries like Axios:
Axios is a popular HTTP client library that simplifies making HTTP requests. It offers a more feature-rich and convenient API compared to the Fetch API.
To use Axios in React, you'll first need to install it:npm install axios
Here's an example of how to use Axios to fetch data in React:
import React, { useEffect, useState } from 'react'; import axios from 'axios'; function MyComponent() { const [data, setData] = useState([]); useEffect(() => { axios.get('https://api.example.com/data') .then(response => setData(response.data)) .catch(error => console.error(error)); }, []); // Render the fetched data return ( <div> {data.map(item => ( <p key={item.id}>{item.name}</p> ))} </div> ); }
In this example, the
axios.get
method is used to fetch data from the API. The response is accessed through theresponse.data
property, and the fetched data is stored in the component's state using thesetData
function.Using async/await syntax:
If you prefer working with async/await syntax for handling asynchronous operations, you can modify the previous examples as follows:
Using Fetch API:import React, { useEffect, useState } from 'react'; function MyComponent() { const [data, setData] = useState([]); useEffect(() => { async function fetchData() { try { const response = await fetch('https://api.example.com/data'); const data = await response.json(); setData(data); } catch (error) { console.error(error); } } fetchData(); }, []); // Render the fetched data return ( <div> {data.map(item => ( <p key={item.id}>{item.name}</p> ))} </div> ); }
Using Axios:
import React, { useEffect, useState } from 'react'; import axios from 'axios'; function MyComponent() { const [data, setData] = useState([]); useEffect(() => { async function fetchData() { try { const response = await axios.get('https://api.example.com/data'); setData(response.data); } catch (error) { console.error(error); } } fetchData(); }, []); // Render the fetched data return ( <div> {data.map(item => ( <p key={item.id}>{item.name}</p> ))} </div> ); }
In both examples, the
fetchData
function is defined as an async function, allowing the use of theawait
keyword to wait for the asynchronous operations to complete.
According to me for beginners using Fetch API is better for learning purposes but in the further part we will be seeing how to implement Axios and async and await.
Implementation of Data Retrieval using Axios!
Step 1 :-
Open your react project and go to App.jsx flie and clear all the unnecessary code needed.Step 2:-
Choose an API to fetch data from, in this implementation, we will be usingOpenWeatherAPI
.Step 3:-
Right***-***click onCurrent Weather
and clickopen in new tab
, you will be redirected to the page where you will get the EndPoint Link to fetch data.Step 4:-
Click onGet API Key
on the page which is shown inStep 2
.Step 6:-
ClickAPI Key
and copy yourAPI Key
and save it somewhere.Step 7:-
Go to the page which is shown onStep 3
and scroll down theExample of API Call
and save that linkStep 8:-
Now go to your code and create a div where you will print the response from the API. And for styling we are usingtailwindcss
you can refer theDocumentation
for installation oftailwindcss
in yourreact project
. Now runnpm run dev
andnpm run react-json-pretty
to install Axios and react-json-pretty on yourreact project
. Thenimport
both of the inApp.jsx
.Step 9:-
Create a stateweatherData
to store the response from the API and import useState.Step 10:-
Create Async Arrow Function namelyfetchData
so that we can use this function to fetch data response from the API.Step 11:-
Create two variable namelyapiKey
andurl
to save your API Key and the Endpoint Url.Step 12:-
Create variable namelyres
where we will store the response of the API. Here we will beawaiting
for response which we will be fetching fromaxios.get()
.Step 13:-
Afterawaiting
the response then we will be setting the value response variable toweatherData
.Step 14:-
Now we have to check weather the API response is coming on or not so we will useuseEffect
to run the function every time when the page is rendered. And we willconsole.log
the data inres
variable to check the response.Step 15:-
To see the output on page then we will useJSONPretty
to Pretty Print the the response.Step 16:-
Now start the server using the commandnpm start
in Normal React ornpm run dev
in ViteJs.
So, this is how we fetch response from a API service using Axios and async-await in React. Now using the .
operator you can specifically use any value from the response which we obtained.
So, in this blog, we have learned about What is API, What are the different types of API, Importance of Data Retrieval from APIs, How to implement fetching data from API and Implementation of data retrieval from APIs . There is much more to learn about the use of CSS in ReactJS, explore this topic and its used cases and elevate your skills.
In the next blog, we will be completing the weather App Project in ReactJS. To know more, make sure to watch the video on our Youtube channel. Happy Coding!!
Adios Amigos ๐๐
Subscribe to my newsletter
Read articles from Coding Adda directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
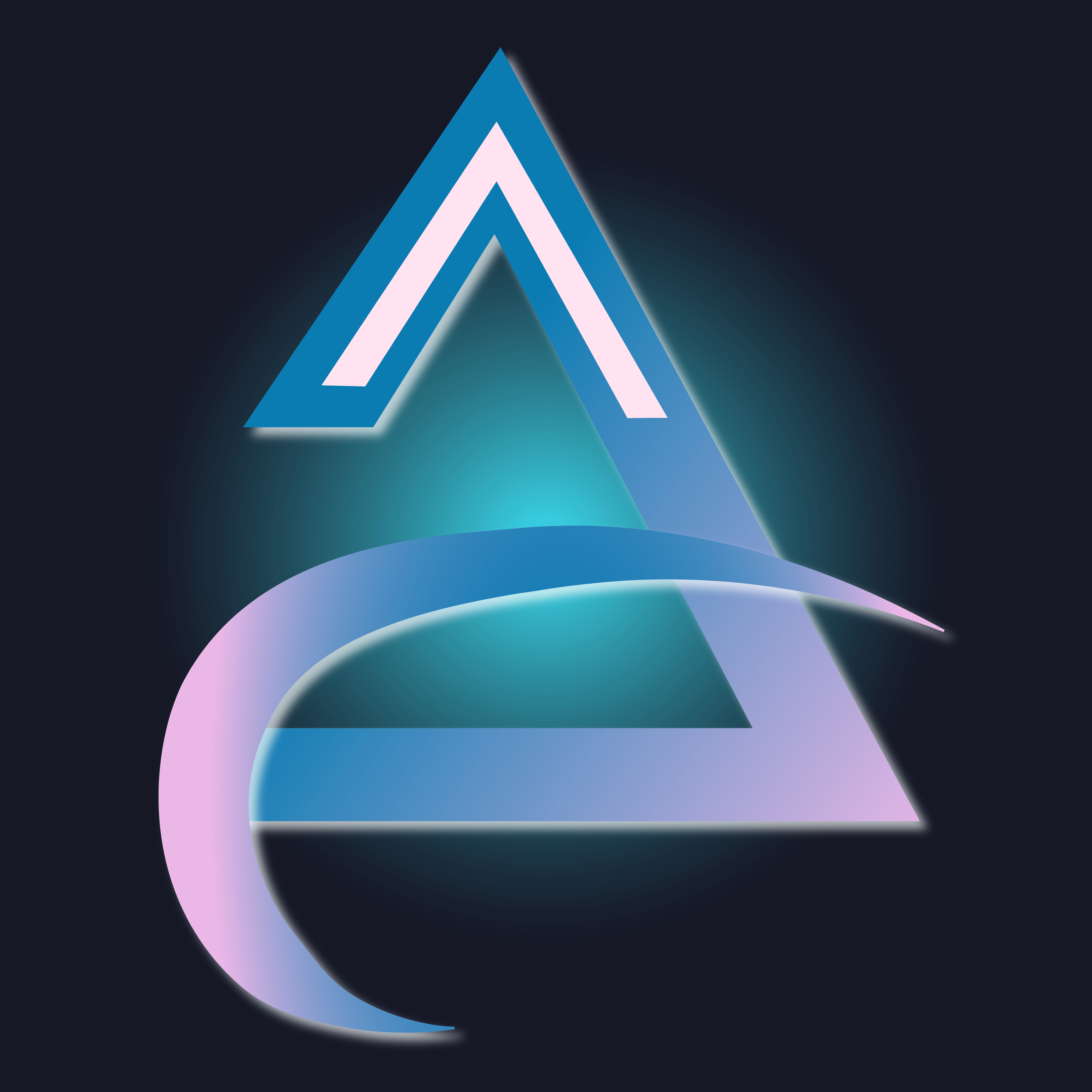
Coding Adda
Coding Adda
Hello Everyone, Presenting you CODING ADDA, We at Coding Adda will help you solve problems in Coding. We at Coding Adda try to clarify the concepts of Coding through short videos and real-life examples. Do support us in our journey. Like, Share and Subscribe to the Channel.