Understanding the Virtual DOM and Browser DOM in React.js: A Comprehensive Comparison

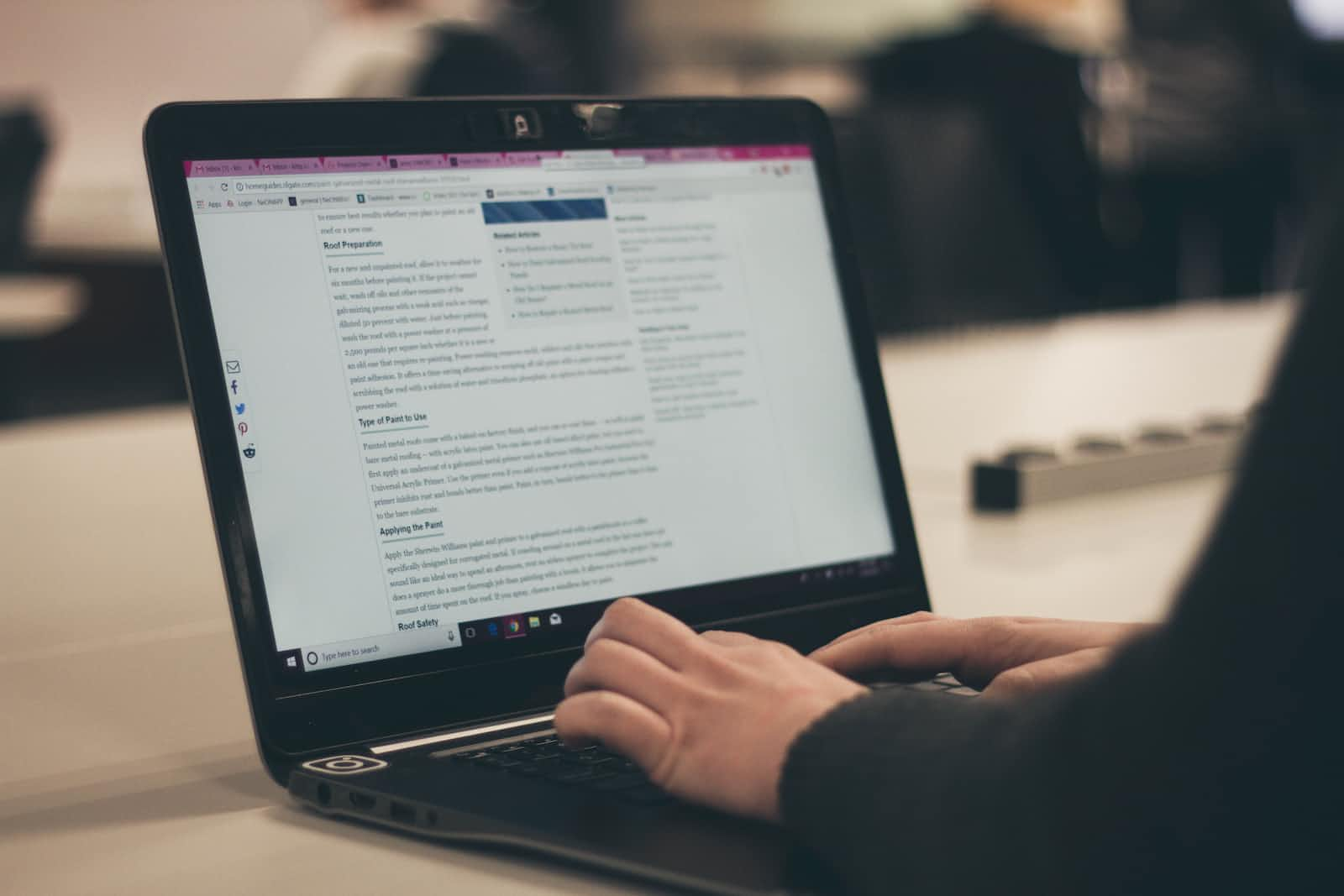
In the world of modern web development, React.js has emerged as one of the most popular JavaScript libraries for building user interfaces. Central to React's performance and efficiency is its innovative use of the Virtual DOM. The Virtual DOM is a key concept that sets React apart from traditional approaches to manipulating the Browser DOM. In this article, we will dive deep into the Virtual DOM and Browser DOM, exploring their differences, benefits, and how React leverages them. By understanding these concepts, developers can make informed decisions when building robust and scalable React applications.
What is DOM?
DOM stands for Document Object Model. It is a programming interface provided by web browsers that represent the structure and content of an HTML or XML document as a tree-like structure. The DOM allows programs, typically written in JavaScript, to access and manipulate the elements, attributes, and text within a web page.
What is Browser DOM?
Browser DOM, also known as the Document Object Model, is a programming interface provided by web browsers that represent the structure and content of an HTML or XML document. It allows JavaScript to interact with and manipulate the elements, attributes, and text within a web page.
Here are some key aspects of the Browser DOM:
Document Structure: The DOM represents an HTML or XML document as a hierarchical tree structure. The top-level node is the document object, which contains child nodes representing elements such as
<html>
,<head>
, and<body>
. These elements, in turn, can have child nodes representing other elements or text nodes.Nodes and Elements: Each element in the DOM is represented by a node object. There are different types of nodes, such as element nodes, text nodes, attribute nodes, and more. Element nodes represent HTML elements, such as
<div>
,<p>
, or<img>
. Text nodes contain the actual text content within an element.Accessing Elements: JavaScript can access and manipulate elements in the DOM using various methods and properties. Common methods include
getElementById()
,getElementsByTagName()
, andquerySelector()
. These methods allow developers to select specific elements based on their IDs, tags, or CSS selectors.Manipulating Content: Once an element is selected, JavaScript can modify its attributes, content, and styling. Properties like
innerHTML
,textContent
, andstyle
provides ways to change the element's content and appearance dynamically. Additionally, attributes likesetAttribute()
andremoveAttribute()
allow for modifying element attributes.Event Handling: The Browser DOM enables the registration of event handlers to respond to user interactions, such as clicks, keyboard input, or form submissions. JavaScript can attach event listeners to elements using methods like
addEventListener()
, allowing developers to execute code when specific events occur.Dynamic Updates: With the Browser DOM, JavaScript can dynamically update the content and structure of a web page. Elements can be created, modified, or removed from the DOM tree, enabling the creation of interactive and responsive web applications.
Cross-Browser Compatibility: While the Browser DOM is standardized, there may be slight differences in how different browsers implement it. Developers need to consider browser compatibility and may use libraries or frameworks, like jQuery or React, to abstract away some of these differences.
The Browser DOM provides a powerful interface for JavaScript to interact with web pages, allowing for dynamic content manipulation, event handling, and overall interactivity. It forms the foundation for many web development tasks and enables the creation of rich, interactive user experiences on the web.
What is React DOM?
React DOM is a specific package in the React library that provides methods and components for rendering React elements into the browser's Document Object Model (DOM). It serves as the bridge between React components and the actual HTML elements in a web page.
When using React to build web applications, the goal is to create reusable UI components that can efficiently update and render changes in response to data updates or user interactions. React DOM handles the rendering of these components into the browser DOM, ensuring that the user interface stays in sync with the application's state.
React DOM offers several key features and functionalities:
Component Rendering: React components are rendered into the DOM using the
ReactDOM.render()
method. This method takes a React element or component and a DOM element as arguments and attaches the component to the specified DOM element, making it visible on the webpage.Virtual DOM Diffing: React DOM utilizes a virtual representation of the DOM, known as the Virtual DOM, to optimize rendering performance. When a component's state or props change, React performs a diffing algorithm to determine the minimal set of changes needed to update the actual DOM. This approach avoids costly re-renders of the entire DOM tree and improves performance.
Event Handling: React DOM manages event handling for components, allowing developers to define event listeners and handlers in a declarative manner. React's synthetic event system normalizes event handling across different browsers, providing a consistent and reliable way to handle user interactions.
Server-side Rendering: React DOM supports server-side rendering (SSR), which allows components to be rendered on the server and sent to the client as HTML. This enables faster initial page loads and improved search engine optimization (SEO).
Portal Support: React DOM provides a feature called portals, which allows rendering a component's content into a different DOM node, even if it's outside the current component's hierarchy. Portals are useful for scenarios such as modals, tooltips, or rendering components outside the root DOM node.
React DOM is part of the React library responsible for rendering React components into the browser DOM. It facilitates efficient updates, handles event handling, supports server-side rendering, and provides additional features like portals.
Comparison Chart: Real DOM vs. Virtual DOM
Feature | Browser DOM | Virtual DOM |
Performance | Can be slower due to direct manipulation | Faster due to efficient diffing algorithm |
Rendering Efficiency | May require full re-rendering of the entire tree on every change | Performs partial updates, minimizing the number of DOM manipulations |
Direct Manipulation | Allows direct manipulation of the DOM tree, resulting in immediate changes | Manipulations are done on a virtual representation before updating the DOM |
Memory Usage | May consume more memory due to the need to store the entire DOM tree | Requires additional memory to maintain a virtual representation of the DOM |
Complexity | Can lead to complex code when managing dynamic UI updates | Provides a simpler and more declarative approach to managing UI updates |
Dependency on Libraries | May require additional libraries for efficient updates and optimizations | Often integrated as part of React.js, reducing external dependencies |
Browser Compatibility | Works consistently across browsers | Works consistently across browsers |
Development Workflow | Requires manual DOM manipulation and event handling | Offers a more streamlined development workflow with component-based rendering |
Ecosystem Support | Has a mature ecosystem of libraries and frameworks | Virtual DOM is specific to React.js, with a focused ecosystem of support |
Conclusion
Virtual DOM and Browser DOM are two fundamental concepts in React.js that play crucial roles in rendering and updating user interfaces. The Virtual DOM offers significant performance improvements by minimizing direct interactions with the Browser DOM and optimizing the update process. It allows React to efficiently determine and apply the necessary changes, resulting in faster rendering and a smoother user experience.
Subscribe to my newsletter
Read articles from Darshana Mallick directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Darshana Mallick
Darshana Mallick
I am a Web Developer and SEO Specialist.