Basics of Python
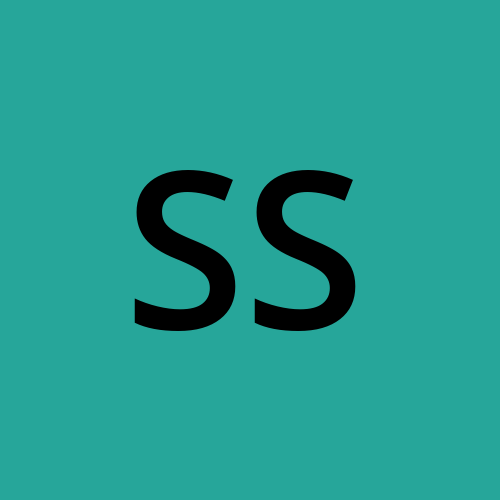
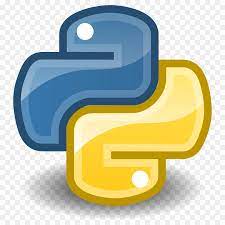
Day13 of #90daysofdevops Challenge
What is Python?
Python is an interpreted, object-oriented, high-level programming language with dynamic semantics. Its high-level built-in data structures, combined with dynamic typing and dynamic binding, make it very attractive for Rapid Application Development, as well as for use as a scripting or glue language to connect existing components.
Python's simple, easy-to-learn syntax emphasizes readability and therefore reduces the cost of program maintenance. Python supports modules and packages, which encourages program modularity and code reuse.
Task:
Install Python in your respective OS, and check the version.
To install Python on Linux, We need to run the below commands
sudo apt-get update
sudo apt-get install python3
To check Python version.
puthon3 --version
Read about different Data Types in Python.
Primitive Datatypes:
Numeric Types:
Integer: Represents integer values, such as 9,6000,-20.
Float: Represents floating-point numbers with decimal places, such as 22.7,-5.6
Complex: Represents complex numbers in the form a_bj, where a and b are floats and j is the imaginary unit.
Sequence Types:
the sequence data type in Python is used to store (in an organized fashion) the sequential data. The sequence data type in Python can be considered as a container that can store different data.
There are mainly three types of sequence data type in Python programming language, they are as follows:
string: A string is an immutable data type that is used to store the sequence of Unicode characters. In Python, there is no character data type so even a single character is treated as a string having a length of 1.
Strings are one of the most widely used data types of any programming language. A string in Python can be easily created using quotes (either single quotes, double quotes, or triple quotes). The quotes mark the beginning and end of the string
List: The list is a built-in data structure in Python that stores the data in sequential form. Lists can be treated as dynamic arrays. List data structures in Python can contain duplicate elements as each element is identified by a unique number/ position known as an index.
The list in Python provides a wide variety of methods and functions. We can put heterogeneous type values inside the lists. The most common associated method of lists are list(), append(), insert(), extend(), sort(), reverse(), remove(), pop(), clear() etc.
Tuple: The tuple is also a built-in data structure in Python which are somewhat similar to lists data structures in Python. A tuple is an immutable object that stores the elements in an ordered manner. We cannot change the elements of the tuple or the tuple itself, once it's assigned whereas we can change the elements of the list as they are mutable.
The tuple in Python provides a wide variety of methods and functions. We can put heterogeneous type values inside the tuples. The most common associated method of tuples is tuple(), count(), count(), index() etc.
Mapping Type:
dict(): Dictionaries are used to store data values in key: value pairs.
A dictionary is a collection that is ordered, changeable and does not allow duplicates.
Dictionaries are written with curly brackets, and have keys and values:
When we say that dictionaries are ordered, it means that the items have a defined order, and that order will not change.
Unordered means that the items do not have a defined order, you cannot refer to an item by using an index.
Set Types:
Set: Set items are unordered, unchangeable, and do not allow duplicate values.
Unordered means that the items in a set do not have a defined order.
Set items can appear in a different order every time you use them, and cannot be referred to by index or key.
Set items are unchangeable, meaning that we cannot change the items after the set has been created.
Boolean Type:
Booleans represent one of two values: True
or False
.
In programming, you often need to know if an expression is True
or False
.
You can evaluate any expression in Python, and get one of two answers, True
or False
.
When you compare two values, the expression is evaluated and Python returns the Boolean answer:
None Type:
The None
keyword is used to define a null value, or no value at all.
None is not the same as 0, False, or an empty string. None is a data type of its own (NoneType) and only None can be None.
Thanks for reading!!!!!
Subscribe to my newsletter
Read articles from sangeeta shinde directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
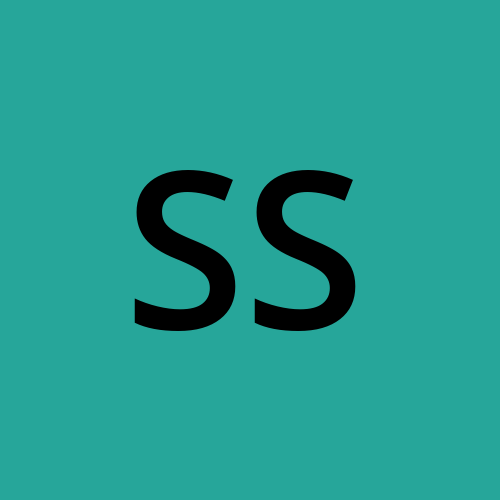