Functions Junction
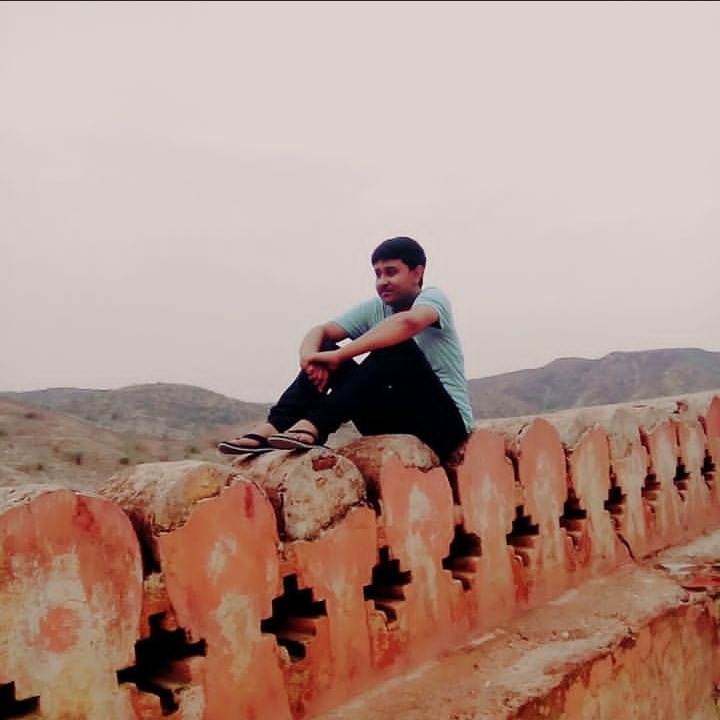
The functions are the heart of JavaScript. Then what are functions? Functions are the way of writing code that can be used anywhere in the code. In javascript, there are many different ways of writing functions. Let's discuss them
Function Declaration
a function declaration is a way of defining a function by a special keyword of function
function simple(){
console.log("I am the simplest type of function")
}
simple()
Arrow Functions
Arrow Functions are new to javascript and they are introduced in the ES6 version of javascript. They are assigned with a variable. Let's see an example
const arrow = () => {
console.log("I am the youngest one to the family")
}
arrow()
Function Expression
This type of syntax is very unique in a programming language in which a function is assigned with a variable.
const express = function(){
console.log("I am the unique one")
}
express()
Anonymus Function
The which are declared without a name is called anonymous function, but there is a catch with declaring an anonymous function. When anonymous functions are declared as function expression then it is a correct format but when declared only the function
keyword it gives a syntax error of function name not defined
. Let's understand this with code
const anonymous = function(){
console.log("I will not give error, i am good")
}
anonymous()//output => will not give any error
function(){
console.log("you thought functions are not complicated")
}
//output => syntax error "name of function not defined
Immediately Invoked Function Expression(IIFE)
IIFE is the most different type of function having the most different syntax. As most of the functions require explicit function calls, while an IIFE is defined and immediately executed without the need for explicit function calls. Also, one of the most important things is that IIFEs are not hoisted, because they are not statements.
(function() {
// Code inside the IIFE
console.log("This is an IIFE!");
})();
Higher Order Function
A function that takes another function as an argument or returns a function from it is called as Higher Order Function
and the function passed into the higher order function are called as a callback function
function multiplier(factor) {
return function(number) {
return number * factor;
};
}
const double = multiplier(2);
console.log(double(5)); // Output: 10
Constructor Functions
This type of function serves as the blueprint of multiple objects with similar properties and behavior. This function returns an object
function Person(name) {
this.name = name;
}
const person = new Person('John');
console.log(person); // Output: Person { name: 'John' }
First Class Functions
The ability to use functions as value is called a first-class function. These functions can be passed as arguments, parameters and also returns functions
function sayHello() {
console.log("Hello, world!");
}
// Assigning the function to a variable
const greet = sayHello;
What is the difference between all the types?
The main difference between all the types of function in javascript are hoisting. Only the function declaration is hoisted while all the other types are not. It is because in all the other types of functions, the functions are treated as a variable.
There is a difference between the behavior of the this
keyword in function declarations and arrow functions in JavaScript. In a regular function declaration, the value of this
is determined by the execution context in which the function is called. It refers to the object that is the subject of the current execution context. The value of this
can change dynamically based on how the function is invoked.
On the other hand, arrow functions do not have their own this
value. Instead, they lexically inherit the this
value from the enclosing (parent) scope. This means that the this
value inside an arrow function is determined by the context in which the arrow function is defined, rather than how it is called.
Subscribe to my newsletter
Read articles from akshit bhati directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
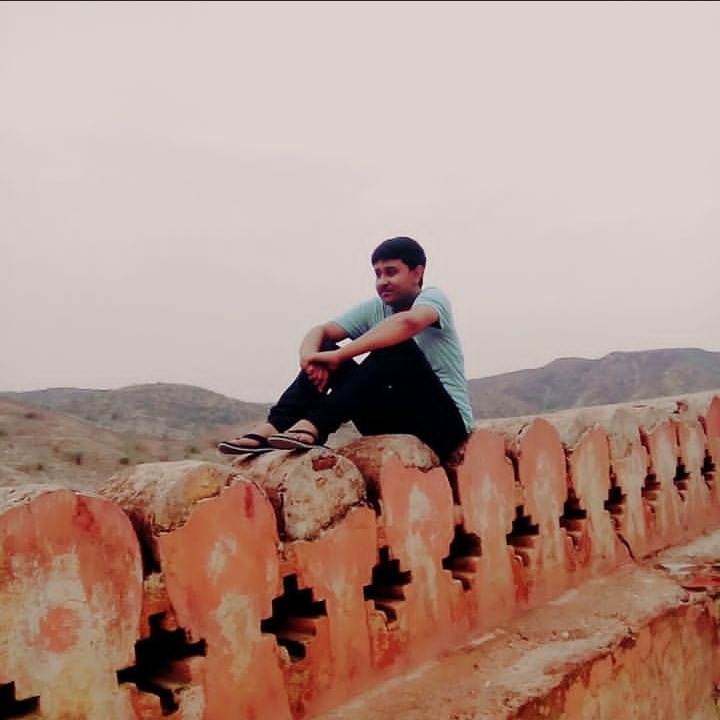