HTML one shot (revision)
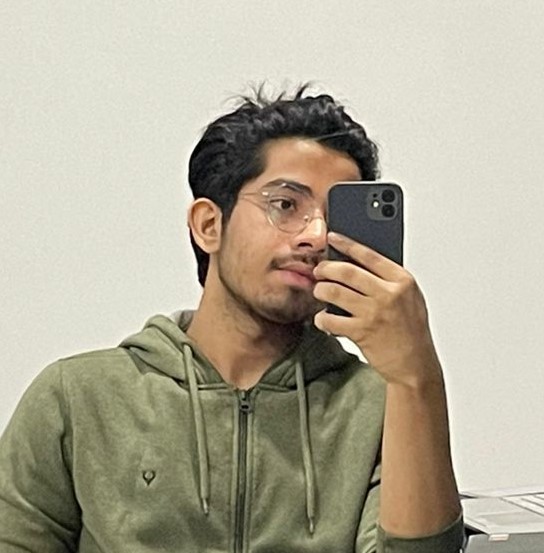
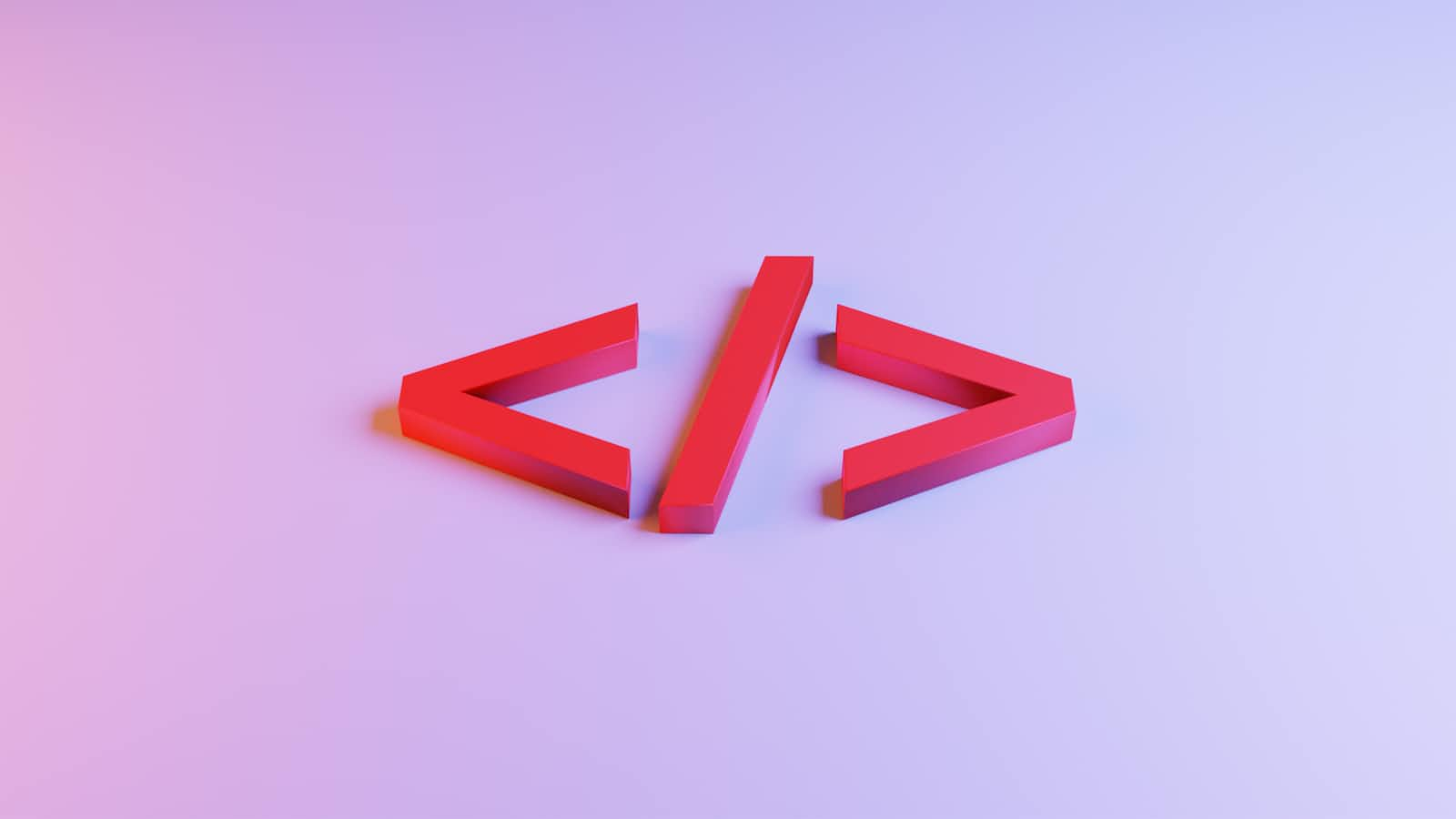
*Basics of HTML:
<!DOCTYPE html> :
The doctype tells the browser to use standards mode, if not it uses older methods(quirk).<html> : root, consists of <head> and <body>
<html lang = "ISO code"> : helps browser to know the content language.
*Head tag
<head>: takes all metadata.(a set of data that provides information about other data)
<title>
: browser's title bar or tab.<meta charset="...">
: such as UTF-8 or ISO-8859-1, which ensures proper text rendering and international character support.<meta name="description" content="...">
: provides a concise summary or description of the webpage's content. Search engines may use this description in search results to give users a preview of the page.<meta name="keywords" content="...">
: This element specifies a comma-separated list of keywords relevant to the webpage's content. While search engines may not give significant weight to this metadata anymore, it can still be used for reference.<meta name="viewport" content="...">
: This element defines the viewport properties for responsive web design.<link rel="stylesheet" href="...">
: links an external CSS file to the HTML<script src="..."></script>
: links an external JavaScript file to the HTML<base href="...">
: This element specifies the base URL for all relative URLs within the HTML document, which helps resolve relative links correctly.<meta name="robots" content="...">
: This element instructs search engine crawlers on how to index and handle the webpage. Values like "index" or "noindex" determine if the page should be included in search engine indexes.
*MetaData
HTTP-Equiv Metadata:
<meta http-equiv="refresh" content="5;URL=
http://example.com/
">
: This tag instructs the browser to refresh or redirect the webpage after a specified time interval (in seconds) defined by the "content" attribute.<meta http-equiv="X-UA-Compatible" content="IE=edge">
: This tag is used to specify the version of Internet Explorer (IE) compatibility mode.
Open Graph Metadata: Open Graph (OG) is a metadata protocol developed by Facebook to enhance the display of shared links on social media platforms. It allows website owners to control how their content is presented when shared on platforms like Facebook, Twitter, and LinkedIn. Open Graph metadata is added to the HTML
<head>
section using the following format:<meta property="og:title" content="Your Page Title"> <meta property="og:description" content="Description of your page"> <meta property="og:image" content="URL to an image associated with the page"> <meta property="og:url" content="URL of the page">
By providing these Open Graph tags, you can control the title, description, image, and URL preview when someone shares your webpage on social media platforms.
Open Graph metadata helps improve the visual representation and click-through rates of shared links by ensuring a consistent and appealing presentation across different social media platforms. It provides an opportunity to optimize how your webpage appears when shared, attracting more engagement and traffic.
*Semantic Html
It involves using HTML elements that have inherent semantic value, making it easier for both humans and machines to understand the purpose and context of the content.
Here are some key aspects and benefits of using semantic HTML:
Clearer Structure: Semantic HTML helps organize the content in a structured manner. Instead of relying solely on divs and spans for layout purposes, semantic elements like
<header>
,<nav>
,<section>
,<article>
,<footer>
,<aside>
, etc., provide a clearer structure to the webpage. This improves the readability and maintainability of the code.Accessibility: By using semantic elements, such as
<h1>
to<h6>
for headings,<nav>
for navigation,<main>
for the main content, and<figure>
for images with captions, you provide meaningful cues to assistive technologies.Search Engine Optimization (SEO): Semantic elements help search engines identify important sections, such as the main content, headings, navigation, and footer, which can positively impact the page's ranking and visibility in search results.
Here are a few examples of semantic HTML elements:
<header>
: Represents the introductory content at the top of a webpage or a section.<nav>
: Defines a section containing navigation links.<main>
: Indicates the main content of a webpage. only one is used.<article>
: Represents a self-contained composition within a document, such as a blog post, news article, or forum post.<section>
: Defines a thematic grouping of content.<aside>
: Represents content that is tangentially related to the main content, such as sidebars or callout boxes.<footer>
: Represents the footer or end section of a webpage or a section.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Semantic HTML Example</title>
</head>
<body>
<header>
<h1>My Website</h1>
<nav>
<ul>
<li><a href="#">Home</a></li>
<li><a href="#">About</a></li>
<li><a href="#">Services</a></li>
<li><a href="#">Contact</a></li>
</ul>
</nav>
</header>
<main>
<section>
<h2>About Us</h2>
<p>Welcome to our website! We provide top-quality products and excellent services to our customers.</p>
</section>
<section>
<h2>Our Services</h2>
<ul>
<li>
<h3>Web Design</h3>
<p>We offer professional web design services tailored to your needs.</p>
</li>
<li>
<h3>Graphic Design</h3>
<p>We create visually appealing graphics and designs for various purposes.</p>
</li>
<li>
<h3>SEO Optimization</h3>
<p>We help optimize your website for better search engine visibility.</p>
</li>
</ul>
</section>
</main>
<footer>
<p>© 2023 My Website. All rights reserved.</p>
</footer>
</body>
</html>
In this example, you can observe the use of semantic elements such as <header>
, <nav>
, <main>
, <section>
, <h1>
to <h3>
, <ul>
, <li>
, and <footer>
. These elements provide a clear structure and convey the meaning and purpose of the content within the webpage.
Role Attribute
The role
attribute is typically combined with appropriate semantic HTML elements to provide additional context and clarity.
<button role="switch" aria-checked="false">Toggle</button>
Accessibility Object Model (AOM)
The AOM is like a semantic version of the DOM.
The Accessibility Object Model (AOM) is a JavaScript API that provides developers with programmatic access to accessibility information and functionality of web content. It is designed to enhance the capabilities of web developers in creating more accessible websites and applications. The AOM allows developers to interact with and manipulate the accessibility tree, which represents the structure and properties of accessible elements on a webpage.
The AOM tree is dynamically generated and maintained by the browser, ensuring that it accurately reflects the current state of the webpage. This enables assistive technologies, such as screen readers, to traverse the AOM tree and convey the content and functionality to users with disabilities.
If you're planning to learn React, it's important to note that React itself provides several accessibility features and patterns out of the box. React has its own way of managing the accessibility tree and handles updates and events to ensure accessible interactions. React uses concepts like virtual DOM diffing and synthetic events to provide accessible interfaces by default.
In React, you'll typically work with accessible components, use ARIA attributes, and apply best practices for accessibility in your JSX code. React also has a strong ecosystem of accessibility libraries and tools, such as
react-aria
,react-router-dom
, andreact-modal
, which further simplify the implementation of accessible components and patterns.
Semantic Structure
DOM
Attributes:
Boolean Attributes:
only two values either true or false
Examples of boolean attributes include
disabled
,readonly
,checked
,required
, andautoplay
. For instance,<input type="checkbox" checked>
Enumerated Attributes:
Enumerated attributes are attributes with a predefined set of allowed values.
Examples of enumerated attributes include
type
(e.g.,<input type="text">
),
Global Attributes:
Global attributes are attributes that can be used on any HTML element.
Examples of global attributes include
id
,class
,style
,tabindex
,role
, andcontenteditable
.
id Attribute:
The
id
attribute is used to uniquely identify an element within a document. It must be unique within the document.The
id
attribute is often used for scripting or styling purposes.
class Attribute:
The
class
attribute is used to assign one or more class names to an element. Class names can be shared among multiple elements.used to apply CSS styles or to select elements using JavaScript or CSS selectors.
style Attribute:
The
style
attribute is used to apply inline CSS styles to an element.For example,
<div style="color: blue; font-size: 16px;">Hello, world!</div>
Some HTML tags:
Text Tags:
<h1>
to<h6>
: Heading tags for defining different levels of headings.<p>
: Paragraph tag for defining a paragraph of text.<span>
: Inline container for grouping and styling text.<strong>
: Specifies strong importance or emphasis.<em>
: Specifies emphasis.<i>
: Specifies italicized text.<b>
: Specifies bold text.<u>
: Specifies underlined text.<s>
: Specifies strikethrough text.<sub>
: Specifies subscript text.<sup>
: Specifies superscript text.
Link Tags:
<a>
: Anchor tag for creating hyperlinks.<link>
: Specifies external stylesheets, icon files, etc.
List Tags:
<ul>
: Unordered list tag for creating a bullet-point list.<ol>
: Ordered list tag for creating a numbered list.<li>
: List item tag used within<ul>
or<ol>
.<dl>
: Definition list tag for creating a list of terms and their definitions.<dt>
: Definition term tag used within<dl>
.<dd>
: Definition description tag used within<dl>
.
Table tags:
<table>
: Represents the entire table.<caption>
: Provides a title or caption for the table.<thead>
: Groups the header content of the table.<tbody>
: Groups the body content of the table.<tfoot>
: Groups the footer content of the table.<tr>
: Represents a table row.<th>
: Represents a table header cell.<td>
: Represents a table data cell.<colgroup>
: Groups a set of<col>
elements to define column properties.<col>
: Specifies the properties of one or more table columns.<thead>
,<tbody>
, and<tfoot>
are optional, but they provide semantic structure to the table.
Here's an example of a simple table with three columns (Name, Age, and Email) and two rows of data:
<table>
<caption>Employee Information</caption>
<thead>
<tr>
<th>Name</th>
<th>Age</th>
<th>Email</th>
</tr>
</thead>
<tbody>
<tr>
<td>John Doe</td>
<td>30</td>
<td>john.doe@example.com</td>
</tr>
<tr>
<td>Jane Smith</td>
<td>35</td>
<td>jane.smith@example.com</td>
</tr>
</tbody>
</table>
In this code snippet, the <table>
element represents the entire table. The <caption>
element provides a title or caption for the table. The table header is defined using the <thead>
element and contains a single row (<tr>
) with three header cells (<th>
). The table body is defined using the <tbody>
element and contains two rows of data, each with three data cells (<td>
).
Image Tags:
<img>
: Embeds an image into an HTML document.
Video Tags:
<video>
: Embeds a video or video stream into an HTML document.<source>
: Specifies video sources for the<video>
element.<track>
: Specifies text tracks for the<video>
element (e.g., subtitles, captions).
Audio Tags:
<audio>
: Embeds an audio file or audio stream into an HTML document.<source>
: Specifies audio sources for the<audio>
element.<track>
: Specifies text tracks for the<audio>
element (e.g., subtitles, captions).
Here's an example of how these tags can be used:
<!-- Image -->
<img src="path/to/image.jpg" alt="Description of the image">
<!-- Video -->
<video controls>
<source src="path/to/video.mp4" type="video/mp4">
<source src="path/to/video.webm" type="video/webm">
<track kind="subtitles" src="path/to/subtitles.vtt" srclang="en" label="English">
Your browser does not support the video tag.
</video>
<!-- Audio -->
<audio controls>
<source src="path/to/audio.mp3" type="audio/mpeg">
<source src="path/to/audio.ogg" type="audio/ogg">
<track kind="subtitles" src="path/to/subtitles.vtt" srclang="en" label="English">
Your browser does not support the audio tag.
</audio>
Form Tags:
<form>
: Defines an HTML form for user input.<input>
: Represents an input control within a form (e.g., text input, checkbox, radio button).<textarea>
: Represents a multiline text input control.<select>
: Represents a dropdown list or a list box control.<option>
: Defines an option within a<select>
element.<button>
: Represents a clickable button within a form.<label>
: Associates a label with a form control.<fieldset>
: Groups related form elements together.<legend>
: Defines a caption or title for a<fieldset>
.
Explanation of the method
attribute: The method
attribute is used in the <form>
tag to specify the HTTP method to be used when submitting the form data to the server. It determines how the form data is transmitted.
There are two commonly used values for the method
attribute:
GET
method:The
GET
method is the default method used by HTML forms if themethod
attribute is not specified.When the form is submitted with the
GET
method, the form data is appended to the URL as query parameters.This method is typically used for retrieving data from the server and for submitting small amounts of non-sensitive data.
Example:
<form action="/submit-form" method="get">
POST
method:The
POST
method is used when you want to send the form data to the server in the body of the HTTP request, rather than as part of the URL.This method is often used when submitting sensitive or large amounts of data, such as login forms or file uploads.
Example:
<form action="/submit-form" method="post">
Here's an example of an HTML form that demonstrates the usage of the method
attribute:
Security: Avoid sending sensitive data via GET
requests. Since form data is appended to the URL as query parameters, it becomes visible in the browser's address bar, browser history, server logs, and can be bookmarked. Therefore, it is not suitable for passwords or other sensitive information.
<form action="/submit-form" method="post">
<label for="name">Name:</label>
<input type="text" id="name" name="name" required>
<label for="email">Email:</label>
<input type="email" id="email" name="email" required>
<button type="submit">Submit</button>
</form>
HTML BEST PRACTICES (LINK)
<iframe>
An <iframe>
(short for inline frame) is an HTML element that allows you to embed another HTML document within the current document. It provides a way to display content from a different source or website within a specific section of a web page. The content inside the <iframe>
is displayed as a separate browsing context, independent of the surrounding page.
Here are some key aspects and considerations regarding the use of <iframe>
in HTML:
Syntax:
htmlCopy code<iframe src="URL"></iframe>
Attributes:
src
: Specifies the URL of the document to be displayed within the<iframe>
.width
andheight
: Define the dimensions of the<iframe>
. You can set these attributes with pixel values (width="500px"
) or percentages (width="100%"
).sandbox
: Optional attribute that provides security restrictions for the content within the<iframe>
. It allows you to control permissions such as restricting scripts or preventing form submission.frameborder
: Deprecated attribute that specifies whether to display a border around the<iframe>
. Instead, CSS can be used to style the border.allowfullscreen
: Optional attribute that enables the<iframe>
content to be displayed in full-screen mode.
Cross-Origin Considerations: When using <iframe>
to embed content from a different domain, you need to consider cross-origin restrictions. The Same Origin Policy prevents scripts within the <iframe>
from directly accessing or modifying the contents of the parent document, unless both documents share the same origin (protocol, domain, and port).
Responsive <iframe>
: To make the <iframe>
responsive and adapt to different screen sizes, you can use CSS techniques. For example, setting the CSS property width: 100%
on the <iframe>
ensures that it scales to fit the container's width. You can also use CSS frameworks, such as Bootstrap, that provide responsive classes to handle <iframe>
sizing.
SEO Considerations: Search engines treat the content within an <iframe>
as a separate document. This means that the content within the <iframe>
might not be indexed or considered as part of the parent document's SEO. If SEO is a concern, consider alternative methods to integrate external content into your page, such as server-side includes or APIs.
Security: When embedding content from external sources, be cautious about the trustworthiness of the content provider. Malicious or poorly secured content can pose security risks to your website and users. Ensure that you trust the source and validate the content before embedding it within an <iframe>
.
Accessibility: Consider the accessibility implications of using <iframe>
. Provide alternative content or a textual description of the <iframe>
content for users who may not have access to it, such as screen reader users.
It's important to use <iframe>
judiciously and consider the impact on user experience, security, and accessibility. Evaluate the need for embedding external content and explore alternative approaches when appropriate.
Subscribe to my newsletter
Read articles from Ayush Gupta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
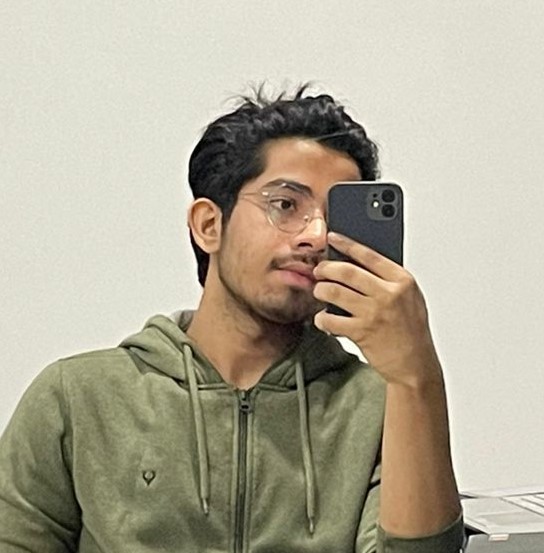
Ayush Gupta
Ayush Gupta
Pursuing Computer Science.