Solidity Arrays
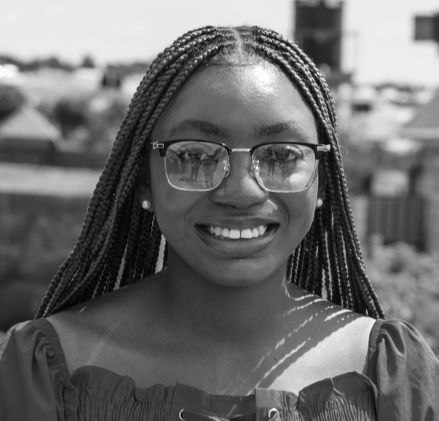
Table of contents
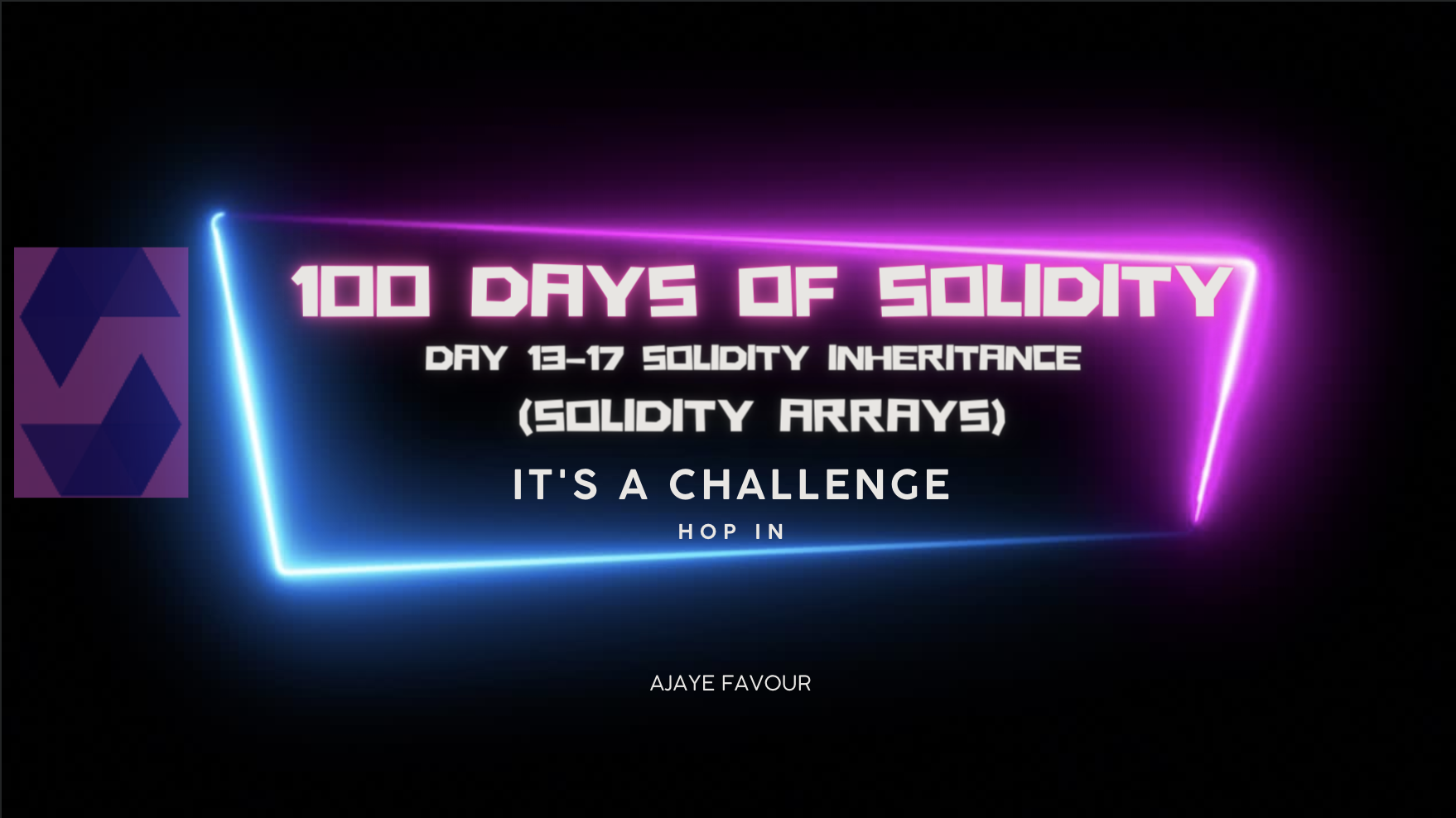
What is an array in solidity?
In Solidity, arrays are used to store collections of data of the same type. You can think of an array as a list or a container that can hold multiple values.
Here's a simple explanation of arrays in Solidity
Declaration: To use an array, you first need to declare it, specifying the data type of the elements it will hold. For example, you can declare an array of integers as
uint[]
, an array of addresses asaddress[]
, or an array of strings asstring[]
.Initialization: After declaring an array, you can initialize it by assigning values to its elements. You can do this either during the declaration or later in your code. For example,
uint[] numbers = [1, 2, 3];
initializes an array of integers with three elements: 1, 2, and 3.Accessing Elements: You can access individual elements of an array using their index. The index starts from 0 for the first element and increments by 1 for each subsequent element. For example,
numbers[0]
would give you the value 1 from thenumbers
array.Modifying Elements: You can modify elements in an array by assigning new values to specific indices. For example,
numbers[1] = 5;
would change the second element of thenumbers
array to 5.Array Length: Solidity provides a built-in property called
length
that allows you to get the number of elements in an array. For example,numbers.length
would give you the length of thenumbers
array.Dynamic and Fixed-Size Arrays: In Solidity, you can have both dynamic and fixed-size arrays. Dynamic arrays can change in size during runtime, while fixed-size arrays have a predetermined length that cannot be changed. For example,
uint[3] fixedArray;
creates a fixed-size array with three elements, and you cannot add or remove elements from it.Array Functions: Solidity provides various array functions and utilities to work with arrays, such as
push
to add elements at the end of a dynamic array,pop
to remove and return the last element of a dynamic array, andlength
to get the length of an array.
Dynamically-sized arrays can only be resized in storage. In memory, such arrays can be of arbitrary size but the size cannot be changed once an array is allocated.
Increasing the length of a storage array by calling push() has constant gas costs because storage is zero- initialised, while decreasing the length by calling pop() has a cost that depends on the “size” of the element being removed. If that element is an array, it can be very costly, because it includes explicitly clearing the removed elements similar to calling delete on them.
Example of an array in solidity
In this example, we have a smart contract called ArrayExample
that demonstrates different array operations.
The contract has a dynamic size array
dynamicArray
and a fixed size arrayfixedArray
.The
initializeArrays()
function initializes both arrays with initial values.The
pushToDynamicArray()
function allows you to add an element to the end of the dynamic array.The
getElementFromDynamicArray()
function retrieves the element at a specified index from the dynamic array.The
updateElementInDynamicArray()
function updates the value of an element in the dynamic array.The
deleteElementInDynamicArray()
function deletes an element from the dynamic array.The
popFromDynamicArray()
function removes and returns the last element of the dynamic array.The
getDynamicArrayLength()
function returns the length of the dynamic array.The
createArrayInMemory()
function demonstrates creating an array in memory and initializes it with values.
You can deploy and interact with this contract to observe how the different array operations work.
Arrays are useful for storing and managing collections of data in Solidity. They allow you to group related data together and perform operations on the entire collection or individual elements.
Check out my other articles on Solidity Basics (Solidity Data Types and Operators)**, [solidity inheritance](https://favourajaye.hashnode.dev/solidity-inheritance), Solidity fallback function and function overloading, Variables and control structures in solidity, Solidity Functions, Libraries in solidity, Abstract contracts and Interfaces in solidity, [Guidelines on becoming a Blockchain Developer in 2023 (Solidity)](https://medium.com/coinsbench/guidelines-on-becoming-a-blockchain-developer-in-2023-solidity-2922150f2f84), [What is blockchain?](https://medium.com/web3-magazine/what-is-blockchain-b42a508ad4da), [All you need to know about web 3.0](https://medium.com/web3-magazine/all-you-need-to-know-about-web-3-0-5d756af5b13), [Solidity: Floating points and precision](https://medium.com/@favoriteblockchain/solidity-floating-points-and-precision-ecdc4c8afb84) and others.**
Click here to see the Github repo for this 100 days of solidity challenge.
Don’t forget to follow me, put your thoughts in the comment section, and turn on your notifications.
SEE YOU NEXT TIME.
Subscribe to my newsletter
Read articles from Favour Ajaye directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
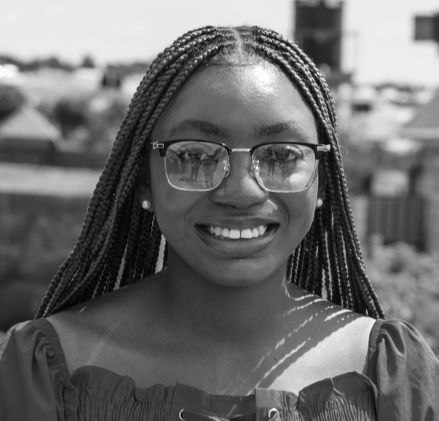
Favour Ajaye
Favour Ajaye
smart contract developer