API Handling In Frontend.
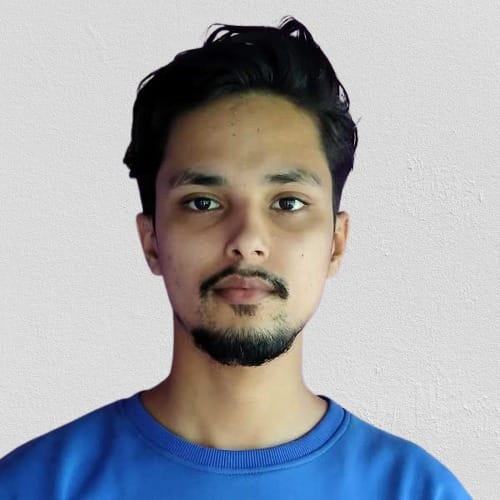
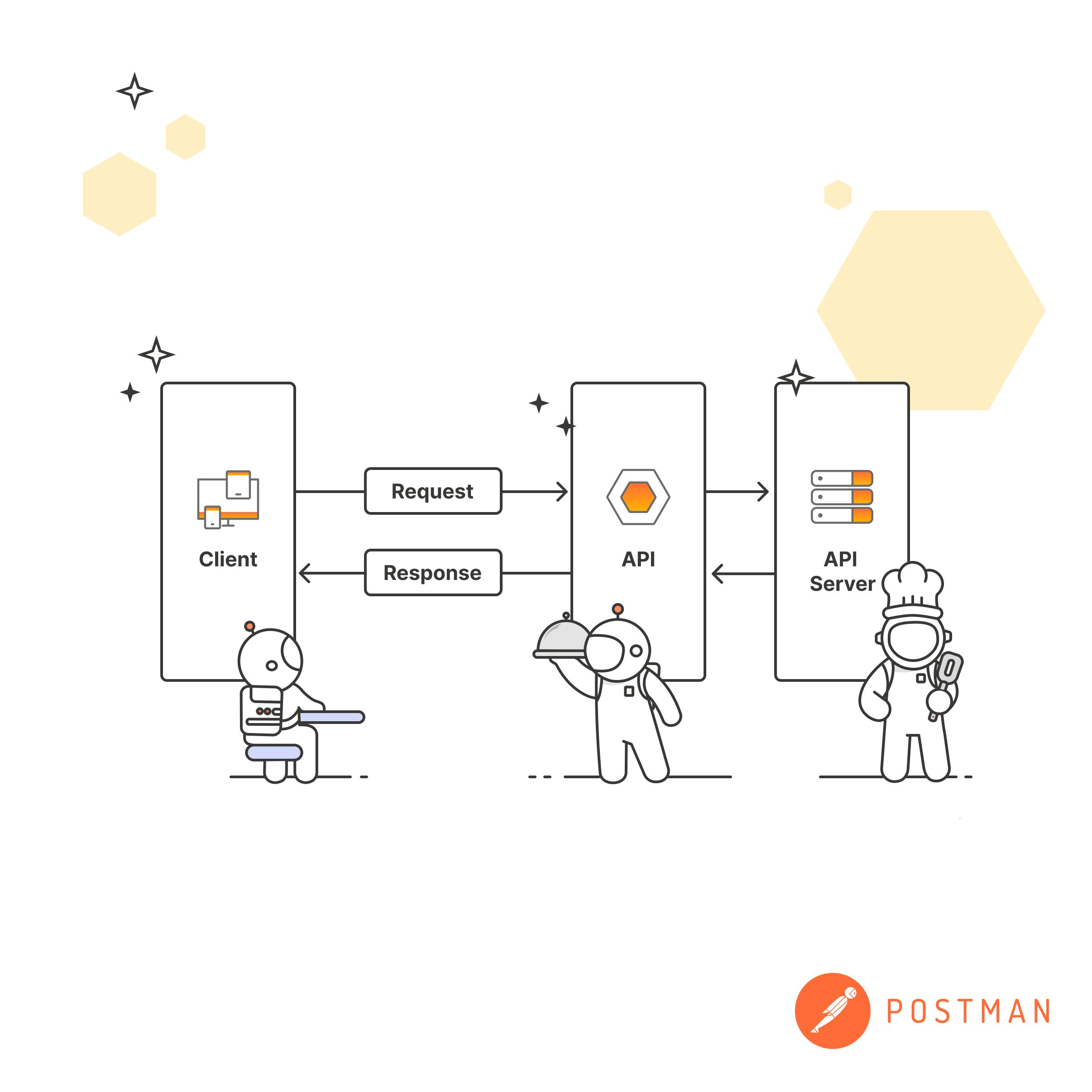
API stands for Application Programming Interface. It is a set of rules and protocols that allows different software applications to communicate with each other. APIs enable developers to access certain functionalities or data from external systems or services.
APIs are commonly used to connect software applications with web services, databases, operating systems, and other software components. They establish a consistent method for applications to ask for and share data. APIs simplify the interaction process by providing ready-made methods and structures, allowing developers to work with the underlying system or service without having to worry about its complex inner workings.
Imagine you have an app that needs weather information. Instead of building your own weather system from scratch, you can use an API provided by a weather service. The API acts as a bridge between your app and the weather service, allowing your app to send requests for weather data and receive the response in a format it can understand.
Types of API
APIs can be categorized into different types, such as:
Web APIs: These APIs are designed to be used over the web using HTTP protocols. They allow applications to access data or perform actions on remote servers. Web APIs often return data in formats like JSON or XML.
RESTful APIs: Representational State Transfer (REST) is an architectural style for designing networked applications. RESTful APIs adhere to the principles of REST and use standard HTTP methods (GET, POST, PUT, DELETE) to perform operations on resources.
SOAP APIs: Simple Object Access Protocol (SOAP) is a messaging protocol that allows programs running on different operating systems to communicate with each other. SOAP APIs use XML to structure the data and typically require a dedicated client library for integration.
Third-Party APIs: These APIs are developed and provided by external service providers, allowing developers to access specific features or data of their services. Examples include social media APIs (e.g., Twitter API, Facebook Graph API) or payment gateway APIs (e.g., PayPal API, Stripe API).
Fetching Data.
- Using fetch api
The Fetch API in JavaScript simplifies sending requests to servers and handling responses. With Fetch, you use the fetch()
function to send a request to a server and receive a response. It's like sending a letter and getting a reply. You can then use the response data by chaining .then()
to process it. If any errors occur, you can handle them using .catch()
. Fetch is a modern and beginner-friendly way to make HTTP requests in JavaScript, enabling you to retrieve data from a server or send data to it. It provides a streamlined approach for communicating with servers and handling data in your web applications.
Get
The GET method is an HTTP request method used to retrieve data from a specified resource on a server. It is commonly used in web development to fetch web pages, access APIs, or retrieve static file.
Daily life examples: watching videos, searching video getting like count in youtube.
// Make a GET request to the API endpoint
fetch('https://https://randomuser.me/api')
// Convert the response to JSON format
.then(response => response.json())
.then(data => {
// Process the data returned from the API
console.log(data);
})
.catch(error => {
// Handle any errors that occur during the fetch
console.error('Error:', error);
});
//Note: It will load the api in the console
Post request
The POST method is an HTTP request method used to submit or send data to be processed by a specified resource on a server. It is commonly used in web development to create new resources, update existing ones, or perform actions that modify the server's state based on the provided data.
Daily life example: adding comment, like, etc.
fetch("https://randomuser.me/api", { // Send a POST request to the specified URL
method: "POST", // Specify the HTTP method as POST
headers: { // Set the request headers
"Content-Type": "application/json" // Specify the content type as JSON
},
body: JSON.stringify({ key: "value" }) // Convert the request payload to JSON and provide it as the body of the request
})
.then(response => response.json()) // Convert the response to JSON format
.then(data => {
// Process the fetched data
console.log(data); // Log the data to the console
})
.catch(error => {
// Handle any errors that occur during the fetch
console.error('Error:', error); // Log the error message to the console
});
Examples
This code is for exploring the different HTTP requests like get and post. I used the above mechanism exactly.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title></title>
</head>
<body>
Api handling in javascript
<h2></h2>
<script>
// Make a GET request to the API endpoint
fetch("https://randomuser.me/api")
// Convert the response to JSON format
.then((response) => response.json())
.then((data) => {
// Process the data returned from the API
console.log(data.results[0].cell);
document.body.querySelector("h2").innerText = data.results[0].cell;
})
.catch((error) => {
// Handle any errors that occur during the fetch
console.log("Error:", error);
});
// post request
fetch("https://jsonplaceholder.typicode.com/posts", {
// Send a POST request to the specified URL
method: "POST", // Specify the HTTP method as POST
headers: {
// Set the request headers
"Content-Type": "application/json", // Specify the content type as JSON
},
body: JSON.stringify({ Name: "Roshan" }), // Convert the request payload to JSON and provide it as the body of the request
})
.then((response) => response.json()) // Convert the response to JSON format
.then((data) => {
// Process the fetched data
console.log(data); // Log the data to the console
})
.catch((error) => {
// Handle any errors that occur during the fetch
console.error("Error:", error); // Log the error message to the console
});
</script>
</body>
</html>
Note: Apply the above code after you understand the concept in the first two code for get and post.
- Using Axios
Axios is a popular JavaScript library used for making HTTP requests from the browser or Node.js. It provides a simple and intuitive API for performing asynchronous HTTP operations and has built-in support for handling request and response interceptors, handling request cancellation, and transforming request and response data.
// GET request
axios.get('https://randomuser.me/api')
.then(response => {
// Process the response from the GET request
console.log(response.data);
})
.catch(error => {
// Handle any errors that occur during the request
console.error('Error:', error);
});
// POST request
axios.post('https://randomuser.me/api', options)
.then(response => {
// Process the response from the POST request
console.log(response.data);
})
.catch(error => {
// Handle any errors that occur during the request
console.error('Error:', error);
});
I know you may be thinking why other than a promise is not used for handling API. It is because for beginners it's overwhelming. First, try to catch the concept of handling API for different requests. After it, you can use different ways like using async await.
Okay an example using async/await.
async function fetchData() {
try {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
console.log(data);
} catch (error) {
console.error('Error:', error);
}
}
fetchData();
The only way to succeed in anything is the ultimate practice.
Subscribe to my newsletter
Read articles from Roshan Guragain directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
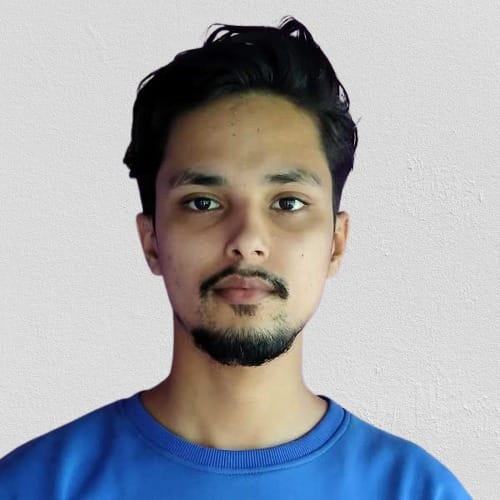
Roshan Guragain
Roshan Guragain
I am a Software developer who is passionate about making web app using the technologies like Html, Css, Javascript, React, Mongodb, Mongoose, Express, Node.