In-Depth Analysis: Post-Increment vs Pre-Increment Operators
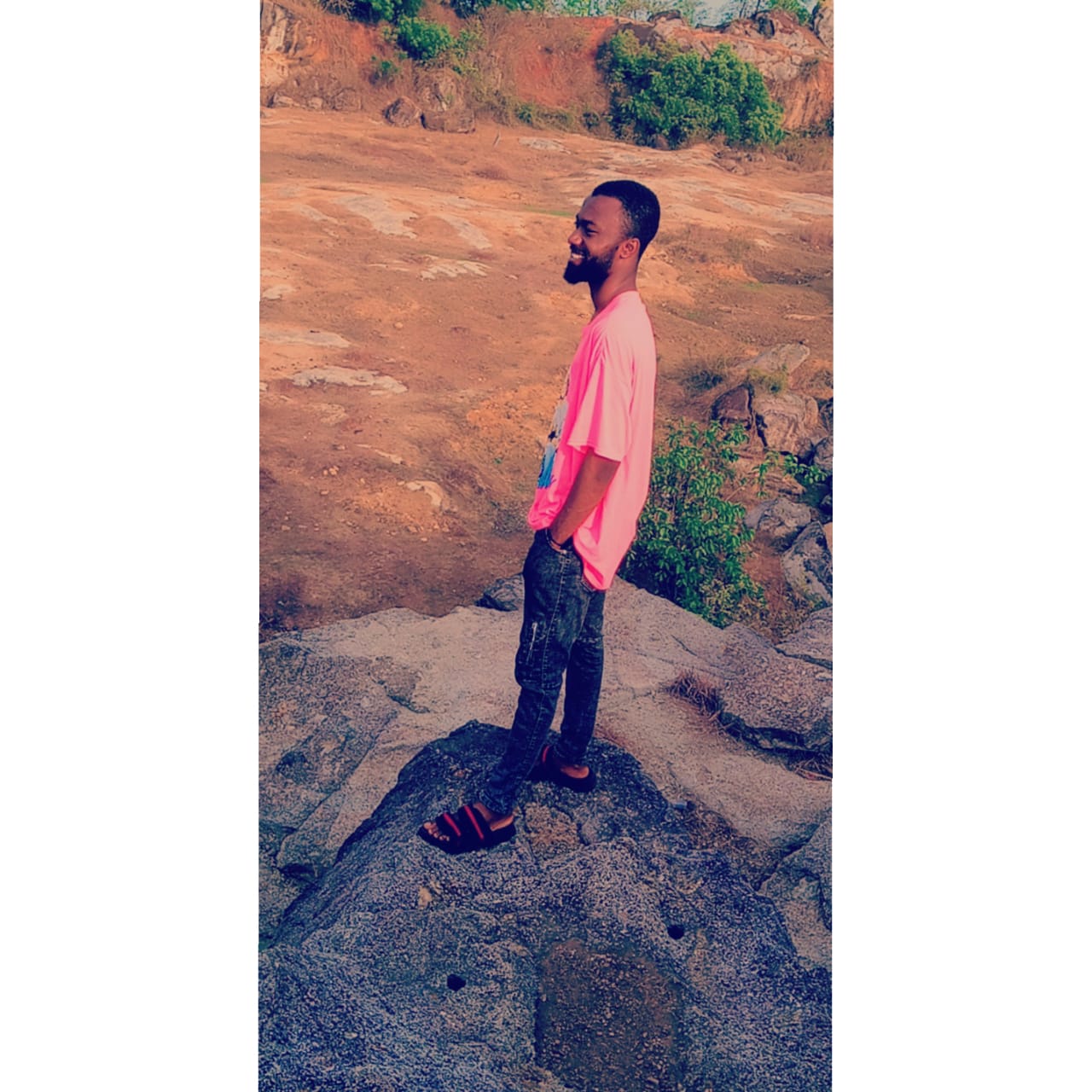
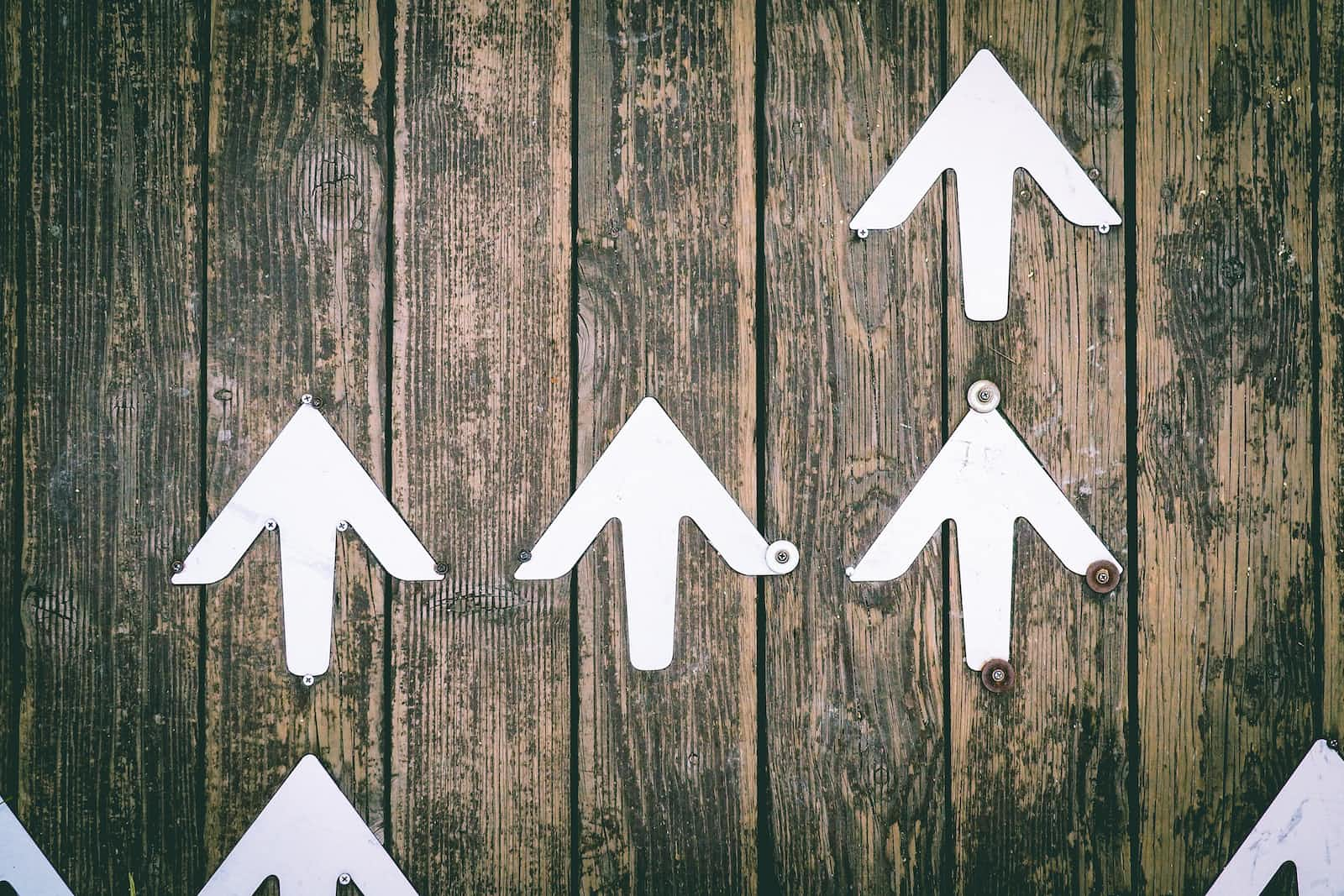
I wrote this blog post because I had a problem with my code and couldn't debug it. It was one of those days when you start questioning whether you have what it takes to be a "real" developer. Everything seemed fine, but the code wouldn't increment as expected.
In software development, it is common to look for new and shorter ways to solve old problems and improve existing solutions. An in-depth look at increment and decrement operations supports this trend. Over time, the three conventional methods of decrementing and incrementing have become shorter and more readable, leading to better overall code readability.
x = x + 1; // 1. increment x by 1
x += 1; // 2. increment x by 1
++x; // 3. increment x by 1
Suppose we have a variable x
assigned a value of 5. This statement x = x + 1
adds 1 to x. The new value of x becomes 6.
#include <stdio.h>
int main(void)
{
int x;
x = 5;
x = x + 1;
printf("%d", x); // output 6
return (0);
}
Likewise, a newer and shorter way to rewrite x = x + 1
is x += 1
. Given a variable x with a value of 5, x += 1
sets the new value of x to 5 + 1. Therefore, the value becomes 6.
#include <stdio.h>
int main(void)
{
int x;
x = 5;
x += 1;
printf("%d", x); // output 6
return (0);
}
INCREMENT AND DECREMENT OPERATOR
The more recent method of increment is by using the operators:
++
: Increment Operator--
: Decrement Operator
The increment operator, represented by two plus signs (++), adds 1 to the value of a variable. The decrement operator, represented by two minus signs (--), subtracts 1 from the value of a variable.
However, it can be confusing because there are two ways to perform increment and decrement operations:
Pre-Increment Operation
Post-Increment Operation
Pre-Decrement Operation
Post-Decrement Operation
PRE-INCREMENT OPERATOR
The pre-increment operator has the operator placed before
the variable. Such as ++x
#include <stdio.h>
int main()
{
int x, y;
x = 5;
y = ++x;
printf("x is equal to %d\n", x); // x is equal to 6
printf("y is equal to %d\n", y); // y is equal to 6
}
After execution, the x and y values were incremented to 6 as expected.
Here are the implicit workings of the operation.
y = ++x;
x = x + 1; // first step, x is incremented and it's value changed
y = x; // second step, y is assigned the new value of x
//new value of x is 6
//new value of y is 6
The code above shows how the expression is executed.
The post-increment operator
++
has higher precedence than the assignment operator=
. Therefore the value of x is incremented.The new value of x is assigned to y.
POST-INCREMENT OPERATION
An Increment can be post-increment
if the operator is placed after
the variable. Such as x++
.
#include <stdio.h>
int main()
{
int x, y;
x = 5;
y = x++;
printf("x is equal to %d\n", x); // x is equal to 6
printf("y is equal to %d\n", y); // y is equal to 5
}
In the code above, we declared two variables, x and y, and assigned a value of 5 to x. In the third statement, y is assigned the post-increment value of x. However, when the results were printed, the value of y was not incremented. This behaviour occurs because the post-increment operator increments the value of x only after it has been used in the assignment statement.
Here are the implicit workings involved in post-increment operation
y = x++;
y = x; // 1. first step, y is assigned the value of x
x = x + 1; // 2. second step, x is then incremented
//new value of x is 6
//new value of y is 5 (no changes)
First, the value of x is assigned to y.
Second, x is incremented.
This was the bug I encountered. Although I knew about these operators, I didn't learn there was a deviation in their operation.
The post-increment/decrement operator is helpful when you need to use a variable's value in an expression before updating it. It lets you perform calculations or assignments with the original value and then update the variable for future use.
The code below is a program that converts a decimal to hexadecimal.
#include <stdio.h>
void decToHex(int decimal, char hex[])
{
int quotient = decimal;
int remainder;
int index = 0;
while (quotient != 0)
{
remainder = quotient % 16;
if (remainder < 10)
hex[index++] = remainder + '0'; // Convert remainder to character
else
hex[index++] = remainder - 10 + 'A'; // Convert remainder to hexadecimal(A-F)
quotient = quotient / 16;
}
// Reverse the hexadecimal string
int i;
int length = index;
for (i = 0; i < length / 2; i++)
{
char temp = hex[i];
hex[i] = hex[length - 1 - i];
hex[length - 1 - i] = temp;
}
hex[length] = '\0'; // Add null-terminating character
}
int main()
{
int decimal = 27;
char hex[18];
decToHex(decimal, hex);
printf("Hexadecimal: %s\n", hex); // output: 1B
return 0;
}
index++
is a post-increment operator, which means that the value of index
is incremented after
the current expression has been evaluated. In this case, the current expression is remainder + '0'
or remainder - 10 + 'A'
, depending on the value of remainder
. Therefore, the first value of index
that is used in the expression is 0. After that, index
is incremented by 1.
Initially, I tried to convert the remainder to a character using the ++index operator, but I did not get the desired result. After carefully studying the operators' workings, I realized my mistake and had to fix it.
POST-DECREMENT OPERATION
The post-decrement operation is represented by the --
operator placed on the right side of an operand or variable. Similar to the post-increment operation, the value is decremented after the current expression has been evaluated. This means the original value of the operand or variable is used in the expression and then decreased by 1.
#include <stdio.h>
int main()
{
int x, y;
x = 5;
y = x--;
printf("x is equal to %d\n", x); // x is equal to 4
printf("y is equal to %d\n", y); // y is equal to 5
}
When the statement y = x--
is executed, the value of x is assigned to y before x is decremented. In other words, y gets the current value of x, and then x is decreased by 1.
PRE-DECREMENT OPERATION
The pre-decrement operation has the operation placed before the operand or variable --x
.
#include <stdio.h>
int main()
{
int x, y;
x = 5;
y = --x;
printf("x is equal to %d\n", x); // x is equal to 4
printf("y is equal to %d\n", y); // y is equal to 4
}
In the code, above the operator sign was placed before
the operand. After execution, the values of x and y were decremented to 4 as expected.
Here are the implicit workings of the operation.
y = --x;
x = x - 1; // 1. first step, decrements the value of x
y = x; // 2. second step, assigned the new value of x to y
//new value of x is 4
//new value of y is 4
The pre-decrement operator is executed on x
The new value of x is assigned to y
Further Examples
#include <stdio.h>
int main()
{
int a, b, x, y;
a = 22;
b = 23;
x = 9;
y = 10;
while (++x < y) // line x
printf("value of x becomes: %d\n ", x); // no output
while (a++ < b) // line y
printf("value of a becomes: %d ", a); //output = 23
return (0);
}
The while statement in line "x" has a condition that prints the value of x while the pre-increment of x is less than y. In this case, the pre-increment of x is 10, which does not satisfy the condition, so no value is printed.
The line "y" contains a while statement with a condition that prints the value of a while the post-increment of a is less than b. In this case, the post-increment of a is 23, which satisfies the condition, so the value is printed.
Summary
The statement
y = ++x
. The value of x is assigned to y after x is incremented. Likewise--x.
The statement
y = x++
. The assignment of x to y takes place first, and then x is incremented. Likewisex--
The pre-increment/decrement operator has higher precedence than the assignment operator. Therefore
++/--
is evaluated first.
Subscribe to my newsletter
Read articles from Patrick Okafor directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
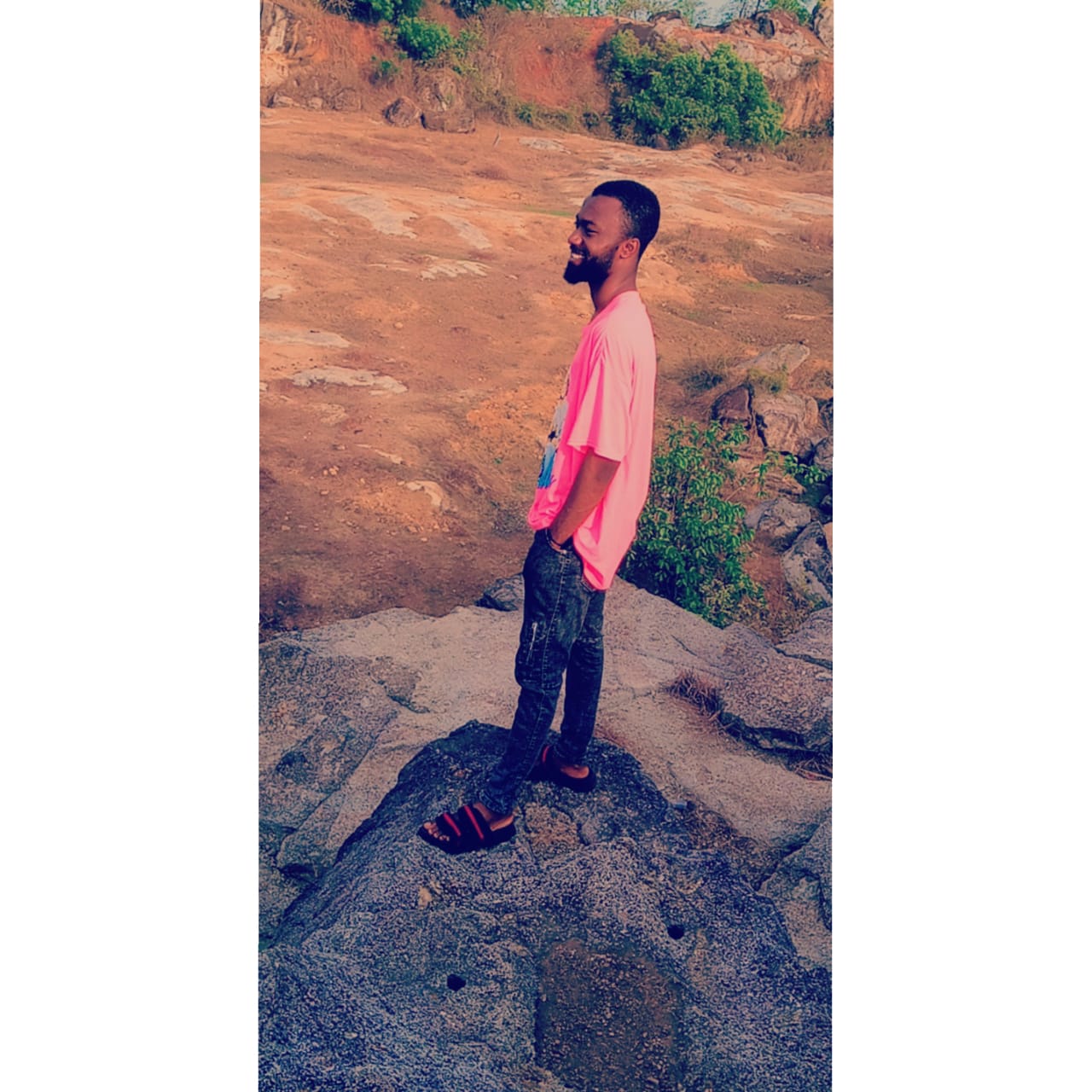
Patrick Okafor
Patrick Okafor
As a software developer and technical writer, I specialize in creating informative articles on software development, computer programming, and various other technology-related subjects. I focus on providing clear and concise explanations, particularly tailored for beginners in the field. I will make complex concepts accessible and enjoyable to learn for all readers.