The Underrated React Hooks: Unleashing the Power of useImperativeHandle (Part 1)
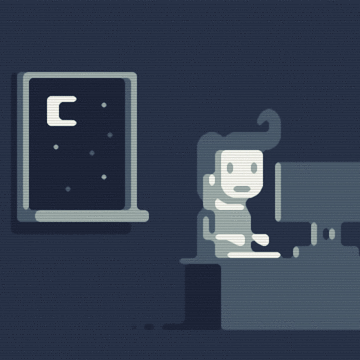
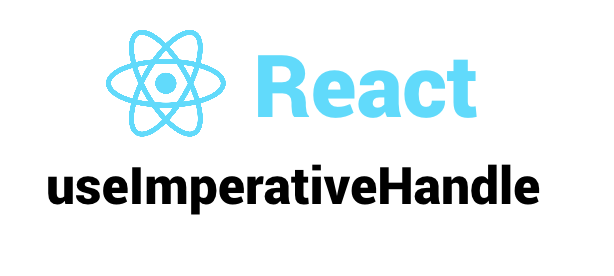
React hooks have revolutionized the way we handle state and side effects in React applications. While popular hooks like useState and useEffect receive well-deserved attention, there are hidden gems that often go unnoticed. In this article, we'll shine a spotlight on one such hook: useImperativeHandle. Prepare to unlock a new level of control and flexibility in your React components.
What is useImperativeHandle? useImperativeHandle is a lesser-known but powerful hook provided by React. It allows you to customize the instance value that is exposed to parent components when using the ref attribute.
Controlling Parent-Child Communication: In some cases, you may encounter scenarios where you need to communicate with a child component from its parent. Traditionally, this can be achieved using callbacks or prop drilling. However, with useImperativeHandle, you can establish a direct and more explicit communication channel by exposing only the necessary functionality to the parent component.
Example:
import React, { useRef, useImperativeHandle, forwardRef } from 'react';
const ChildComponent = forwardRef((props, ref) => {
const internalRef = useRef();
// Expose specific functions to the parent component
useImperativeHandle(ref, () => ({
// Example function to focus an input element
focusInput: () => {
internalRef.current.focus();
},
}));
return <input ref={internalRef} type="text" />;
});
// parent component
const ParentComponent = () => {
const childRef = useRef();
const handleButtonClick = () => {
// Access the exposed function from the child component
childRef.current.focusInput();
};
return (
<div>
<ChildComponent ref={childRef} />
<button onClick={handleButtonClick}>Focus Input</button>
</div>
);
};
By using useImperativeHandle, you can encapsulate complex internal logic and expose only the necessary interface to the parent component. This promotes cleaner and more maintainable code, as the parent component doesn't need to be aware of all the internal implementation details. It also allows for better abstraction, making the child component's functionality more self-contained and reusable.
Another advantage of useImperativeHandle is the ability to optimize performance by avoiding unnecessary re-renders. Since you have control over what is exposed to the parent component, you can choose to update the exposed value only when it's absolutely necessary. This level of granularity helps minimize unnecessary re-rendering of components and enhances overall application performance.
Don't overlook the power of useImperativeHandle when working with React components. It provides a clean and controlled way to establish parent-child communication, encapsulate functionality, and optimize performance. By leveraging this underrated hook, you can take your React applications to new heights of flexibility and maintainability.
Stay tuned for Part 2 of our series on underrated React hooks, where we'll explore another hidden gem that can elevate your development experience.
Subscribe to my newsletter
Read articles from Shadrack Opoku directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
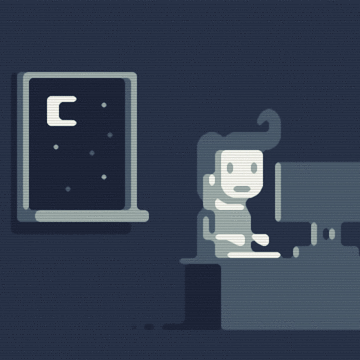
Shadrack Opoku
Shadrack Opoku
Shadrack is an exceptional developer with a strong affinity for Linux and a passion for building impressive applications using JavaScript and Python. His expertise in Linux is overwhelming, and he enjoys exploring its endless possibilities. With a talent for crafting elegant code and a focus on innovation, Shadrack excels in web development, functional programming, system designing and automation. Beyond technical skills, Shadracks's commitment to meaningful projects sets him apart. He thrives on solving complex problems and creating impactful solutions. With a continuous thirst for knowledge.