How to integrate stripe to Django
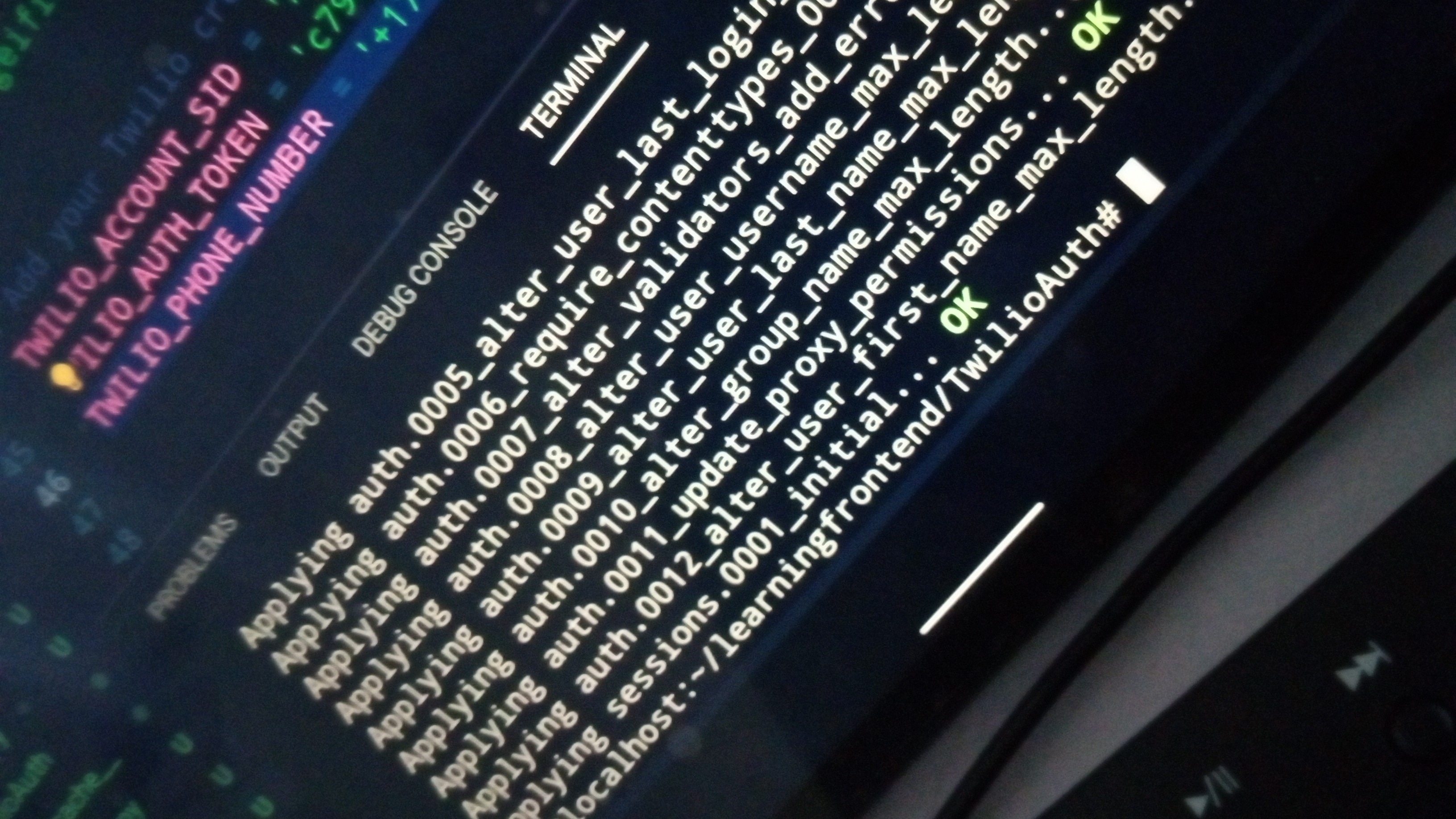
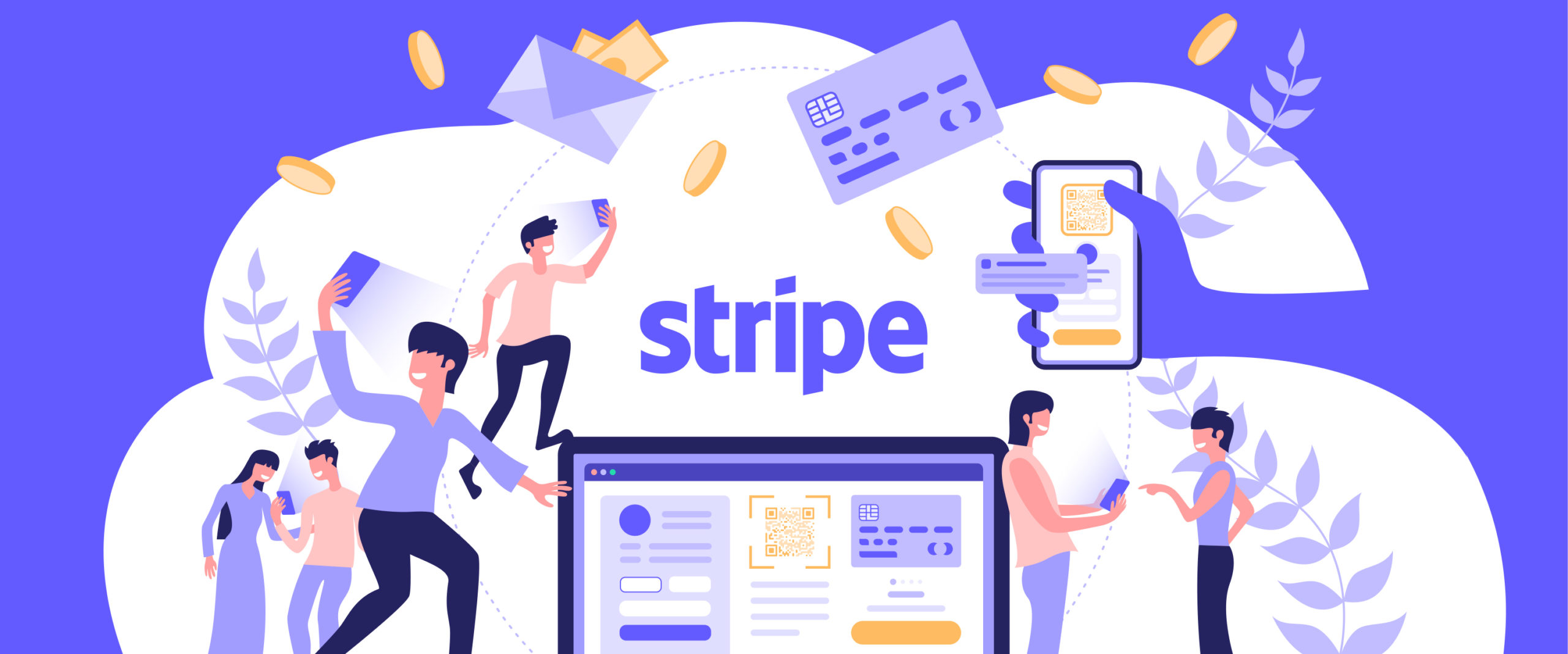
Step 1: Install the required packages
Ensure that you have the necessary packages installed. You can use pip to install them:
pip install stripe
pip install djstripe
Step 2: Configure Django settings
Open your Django project's settings file (settings.py
) and add the following configurations:
# urls.py
from your_app.webhooks import stripe_webhook
urlpatterns = [
# Other URL patterns...
path('stripe/webhook/', stripe_webhook),
]
Make sure to replace 'your-stripe-secret-key'
with your actual Stripe secret key and 'your-stripe-webhook-secret'
with your webhook secret.
Step 3: Run migrations
After configuring the settings, run the migrations to create the necessary database tables for djstripe:
python manage.py migrate djstripe
Step 4: Collect static files
If you haven't already, collect the static files for djstripe:
python manage.py collectstatic
Step 5: Create a Stripe webhook endpoint
To handle Stripe webhooks, you need to create an endpoint in your Django app. Create a new file called webhooks.py
(or any other name you prefer) inside your app directory and add the following code:
# webhooks.py
from django.http import HttpResponse
from django.views.decorators.csrf import csrf_exempt
import stripe
stripe.api_key = 'your-stripe-secret-key'
@csrf_exempt
def stripe_webhook(request):
payload = request.body
sig_header = request.headers['Stripe-Signature']
event = None
try:
event = stripe.Webhook.construct_event(
payload, sig_header, DJSTRIPE_WEBHOOK_SECRET
)
except ValueError as e:
return HttpResponse(status=400)
except stripe.error.SignatureVerificationError as e:
return HttpResponse(status=400)
# Handle the event
# Add your code here to process the event based on its type
return HttpResponse(status=200)
Make sure to replace 'your-stripe-secret-key'
with your actual Stripe secret key.
Step 6: Configure the Stripe webhook endpoint URL
Add the following URL pattern to your project's urls.py
file to map the webhook endpoint:
# urls.py
from your_app.webhooks import stripe_webhook
urlpatterns = [
# Other URL patterns...
path('stripe/webhook/', stripe_webhook),
]
Step 7: Use Stripe in your views
Now you can use Stripe in your Django views. Here's an example of creating a payment intent:
from django.conf import settings
import stripe
stripe.api_key = settings.DJSTRIPE_SECRET_KEY
def create_payment_intent(request):
intent = stripe.PaymentIntent.create(
amount=1000, # amount in cents
currency='usd',
payment_method_types=['card'],
)
# Add your code to handle the payment intent response
return render(request, 'payment.html', {'client_secret': intent.client_secret})
Make sure to replace settings.DJSTRIPE_SECRET_KEY
with your actual Stripe secret key.
That's it! You've now integrated Stripe with Django using the djstripe package. You can explore the djstripe documentation for more advanced features and functionalities.
Subscribe to my newsletter
Read articles from Ifiok Ambrose directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
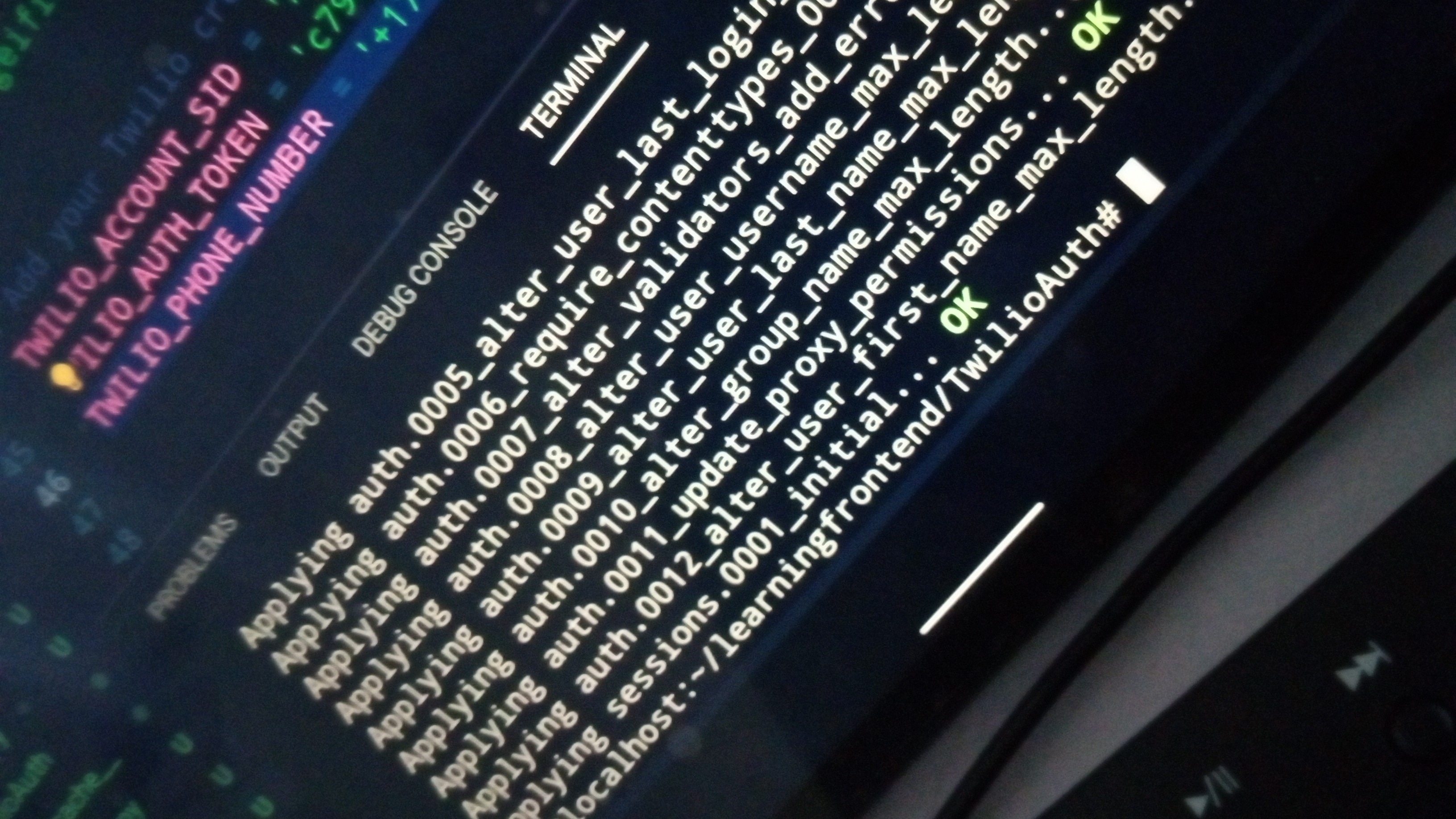