Deep Dive in Git & GitHub for DevOps Engineers

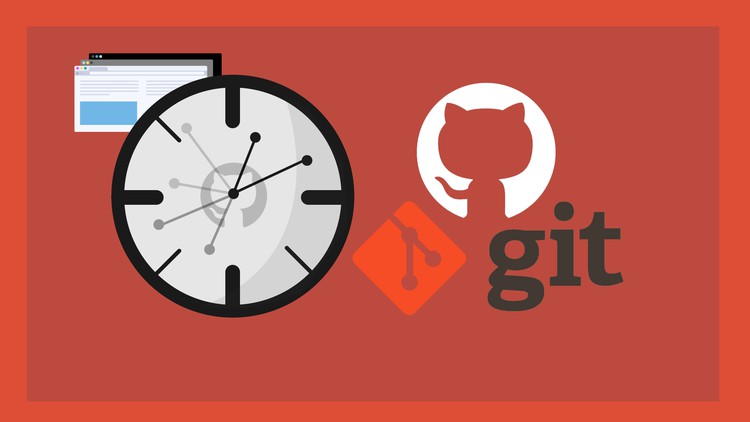
What is Git and why is it important?
Git is a version control system that allows developers to track changes to their code over time. It was created by Linus Torvalds in 2005 and has since become one of the most widely used tools in the software development industry.
Git is important for several reasons:
Collaboration: Git enables multiple developers to work on the same codebase simultaneously without interfering with each other's work. This is achieved through branching and merging, which allow developers to create separate copies of the code and then integrate their changes seamlessly.
Version control: Git provides a complete history of changes made to the codebase, which helps developers keep track of what changes were made, who made them, and when. This is especially useful when debugging issues or rolling back to previous versions of the code.
Backup: Git also serves as a backup mechanism for code. Since every change is tracked and stored, developers can easily recover lost or deleted code from the Git repository.
Open source: Git is an open-source tool, which means it's free to use and anyone can contribute to its development. This has led to a large and active community of developers who contribute to the tool's development and help improve its functionality and features.
Overall, Git is an essential tool for modern software development and has revolutionized the way developers collaborate and manage code.
What is the difference Between Main Branch and Master Branch?
In Git, "main" and "master" branches are both used as the default branch name in different contexts. The difference between them is mostly historical and political.
Traditionally, the default branch in Git was named "master". This name was used in the original Git documentation and in many popular Git workflows. However, some people have argued that the term "master" has negative connotations due to its association with slavery, and have therefore advocated for the use of "main" instead.
In response to these concerns, many Git hosting platforms (such as GitHub and GitLab) have started using "main" as the default branch name. This has led to a shift in the industry towards using "main" instead of "master".
However, it's important to note that the difference between "main" and "master" is mostly cosmetic. They both serve the same purpose as the default branch in Git, which is to serve as the starting point for new development and to be the branch that contains the most up-to-date version of the code.
Ultimately, whether you use "main" or "master" as the default branch in your Git repository is a matter of personal preference and your organization's policies.
Difference between Git and GitHub?
The following table enumerates the key differences between Git and GitHub:
Git | GitHub |
Git is software. | It is a service. |
Linux maintains Git. | Microsoft maintains GitHub. |
It is a command-line tool. | It is a graphical user interface. |
You can install it locally on the system. | It is hosted on the web. It is exclusively cloud-based. |
It is a VCS to manage source code history. | It is a hosting service for Git repositories. |
It focuses on code sharing and version control. | It focuses on centralized source code hosting. |
It lacks a user management feature. | It has a built-in user management feature. |
Git was launched in 2005. | GitHub was released in 2008. |
Git has minimum external tool configuration. | It has an active marketplace for tool integration. |
It is open-source licensed. | It has a free-tier and pay-for-use tier. |
Its desktop interface is named Git Gui. | Its desktop interface is named |
How do you create a new repository on GitHub?
To create a new repository on GitHub, you can follow these steps:
Log in to your GitHub account. If you don't have one, you can create a new account for free at https://github.com.
Once you're logged in, click on the "+" icon at the top right corner of the GitHub homepage and select "New repository" from the dropdown menu.
On the "Create a new repository" page, you'll need to provide some information about your repository:
Repository name: Choose a unique name for your repository. This name will be part of the repository's URL and should be descriptive.
Description (optional): You can add a brief description to explain what your repository is about.
Visibility: Choose whether you want your repository to be public (visible to everyone) or private (visible only to you and collaborators you invite).
Initialize this repository with a README: If you check this option, GitHub will create a README file for your repository. It's a good practice to have a README file that provides information about your project.
Add .gitignore: You can select a template to automatically generate a .gitignore file. This file specifies which files and directories should be ignored by Git version control.
Choose a license (optional): You can select a license if you want to specify how others can use your code. If you're unsure, you can skip this step and add a license later.
Once you've provided the necessary information, click on the "Create repository" button. GitHub will create the repository for you.
Congratulations! You have successfully created a new repository on GitHub. You can now add files, create branches, and collaborate with others on your project.
What is the difference between local & remote repositories? How to connect local to remote?
Local and remote repositories are different aspects of version control systems like Git. Here's an explanation of each and how to connect them:
Local Repository: A local repository resides on your computer. It contains the complete history and files of your project. It is where you make changes, create branches, commit your work, and manage your project using Git commands. The local repository is typically stored in a hidden folder named ".git" within your project's directory.
Remote Repository: A remote repository is hosted on a remote server, such as GitHub, GitLab, or Bitbucket. It serves as a centralized location where you can share and collaborate on your project with others. Remote repositories provide a way to store and synchronize your local repository's changes with others, enabling team collaboration and backup.
Connecting Local to Remote Repository: To connect your local repository to a remote repository, you typically follow these steps:
Create a remote repository: On platforms like GitHub, GitLab, or Bitbucket, create a new repository by following the platform-specific instructions. This will create a remote repository with a URL.
Add a remote URL: Open your local repository in a terminal or command prompt and use the following command to add the remote URL:
csharpCopy codegit remote add origin <remote_repository_url>
Replace
<remote_repository_url>
with the URL of your remote repository. "origin" is the conventional name for the main remote repository, but you can choose a different name if desired.Push to the remote repository: After adding the remote URL, you can push your local repository's changes to the remote repository using the following command:
perlCopy codegit push -u origin master
This command pushes your local branch named "master" (the default branch) to the remote repository.
Authenticate and provide credentials (if required): Depending on the remote repository platform and your settings, you may be prompted to enter your username and password or authenticate through other means to establish a connection between your local and remote repositories.
Once you've completed these steps, your local and remote repositories are connected. You can now push your changes to the remote repository using git push
and fetch changes from the remote repository using git pull
. This allows you to collaborate with others and keep your repositories in sync.
Tasks:-
01. Set your user name and email address, which will be associated with your commits
To set your user name and email address in Git, you can use the following commands:
Open a terminal or command prompt.
Navigate to the directory containing the Git repository.
Run the following command, replacing “your name” with your own name:
git config --global user.name "your name"
This sets your name as the author of all commits you to make in the future.
4. Run the following command, replacing “your email” with your own email address:
git config --global user.email "your email"
This sets your email address as the contact information associated with your commits.
By setting your user name and email address in Git, you can ensure that your contributions to a project are properly attributed to you. It also helps other developers and contributors to contact you if they have questions or comments about your code.
02. Create a repository named “DevOps” on GitHub
To connect your local Git repository to the repository on GitHub, you can follow these steps:
Open a terminal or command prompt.
Navigate to the directory of your local Git repository.
Use the following command to add the remote repository’s URL to your local Git repository:
git remote add origin <remote-repository-URL>
Replace
<remote-repository-URL>
with the URL of your GitHub repository. For example:git remote add origin https://github.com/yourusername/your-repository.git
4. Verify that the remote repository has been added to your local Git repository by running the following command:
git remote -v
5. This command will show you a list of all the remote repositories that your local repository is connected to. You should see the URL of your GitHub repository listed here.
6. Push your local Git repository changes to the remote repository on GitHub by running the following command:
git push -u origin master
This command will push your local
master
branch to the remotemaster
branch on GitHub. If you have a different branch name, replacemaster
with your branch name.The
-u
option tells Git to remember the remote repository and branch so that you can use the shortergit push
command in the future.You may be prompted to enter your GitHub username and password to authenticate your push.
Now your local Git repository is connected to the repository on GitHub, and you can push and pull changes between the two repositories as needed.
03. Create a new file in Devops/Git/Day-02.txt & add some content to it
To create a new file named Day-02.txt
in the Devops/Git
directory on GitHub and add some content to it, you can follow these steps:
Open your web browser and navigate to your repository on GitHub.
Click on the “Create new file” button.
In the “Name your file…” field, type
Devops/Git/Day-02.txt
.In the large text box, add the content you want to include in the file.
At the bottom of the page, you will see a section labeled “Commit new file”. Here you can add a commit message to describe the changes you’ve made. Type a message such as “Add Day-02.txt” in the “Commit new file” field.
Make sure the “Commit directly to the master branch” option is selected.
Click the “Commit new file” button to create the new file and commit your changes.
Your new file,
Day-02.txt
is now added to theDevops/Git
directory on GitHub and contains the content you added. You can now access this file from your local Git repository by pulling the changes from the remote repository on GitHub.
04. Push your local commits to the repository on GitHub
To push your local commits to the repository on GitHub, you can follow these steps:
Open a terminal or command prompt.
Navigate to the directory of your local Git repository.
Pull any changes from the remote repository on GitHub to make sure you have the latest version of the repository:
git pull origin master
This command will fetch and merge any changes from the remote
master
branch on GitHub to your localmaster
branch.4. Add and commit any changes you’ve made to your local Git repository:
git add . git commit -m "Your commit message"
Replace “Your commit message” with a brief description of the changes you’ve made.
05. Push your local Git repository changes to the remote repository on GitHub:
git push origin master
This command will push your local
master
branch to the remotemaster
branch on GitHub. If you have a different branch name, replacemaster
with your branch name.You may be prompted to enter your GitHub username and password to authenticate your push.
Now your local Git repository changes are pushed to the repository on GitHub, and others who have access to the repository can see and pull your changes.
Subscribe to my newsletter
Read articles from Mizan Firdausi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
