Understanding and Implementing the useContext Hook in React

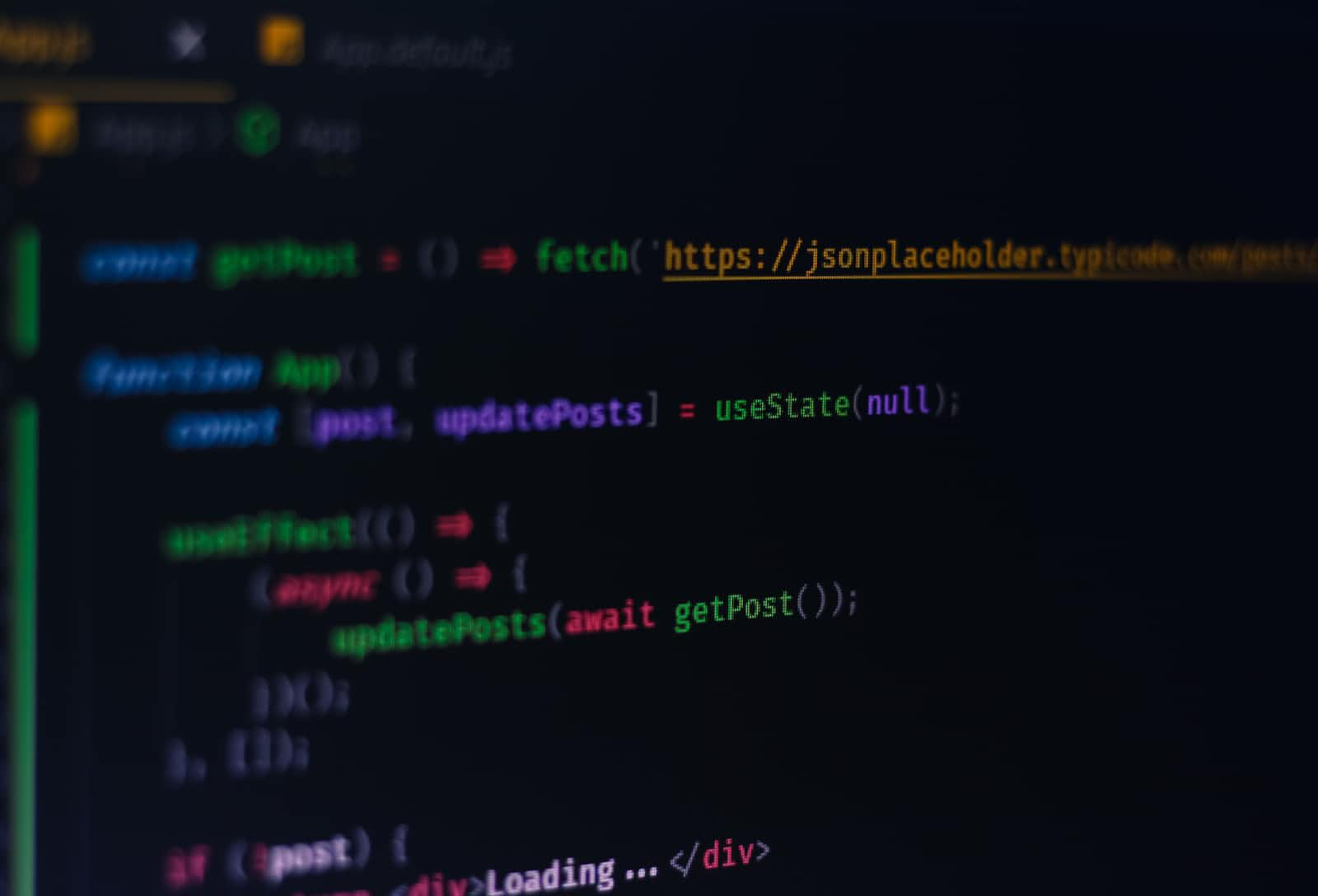
React's useContext hook is a powerful tool that allows components to consume context values without the need for prop drilling. It simplifies the sharing of data and state across components, making code more concise and maintainable. In this article, we will explore the useContext hook in depth and provide practical examples to demonstrate its implementation.
Table of Contents:
What is the useContext Hook?
Setting Up Context
Consuming Context Values
Example: Theme Context
Example: User Authentication Context
Benefits of Using useContext
Best Practices and Considerations
Conclusion
What is the useContext Hook?
The useContext hook is a built-in hook in React that allows components to consume values from a React context. React Context provides a way to share data across the component tree without manually passing props through every level. The useContext hook simplifies the process of accessing context values by providing a more concise and readable syntax.
The useContext hook takes a context object as its argument and returns the current value of that context. It allows functional components to access the context value directly, without the need for a wrapper component or prop drilling.
By using the useContext hook, components can access and utilize context values without having to explicitly pass them through each level of the component tree. This promotes cleaner and more modular code, as components can focus on consuming the values they need without being concerned about how the values are propagated.
The useContext hook is particularly useful when dealing with shared state, such as themes, user authentication, or language preferences. It enables components to subscribe to context changes and automatically update when the context value changes, ensuring that they stay in sync with the latest data.
- Example: Theme Context
To further illustrate the usage of the useContext hook, let's consider an example of a theme context. Assume we have an application where the user can switch between light and dark themes. We want to share the current theme across different components without passing it explicitly through props.
First, let's set up the theme context using createContext():
// ThemeContext.js
import { createContext } from 'react';
const ThemeContext = createContext();
export default ThemeContext;
Next, create a theme provider component that will wrap the components that need access to the theme context. The provider component will manage the theme state and provide it to the consuming components:
// ThemeProvider.js
import React, { useState } from 'react';
import ThemeContext from './ThemeContext';
const ThemeProvider = ({ children }) => {
const [theme, setTheme] = useState('light');
const toggleTheme = () => {
setTheme(theme === 'light' ? 'dark' : 'light');
};
return (
<ThemeContext.Provider value={{ theme, toggleTheme }}>
{children}
</ThemeContext.Provider>
);
};
export default ThemeProvider;
In the above example, we use the useState hook to manage the theme state. The toggleTheme function is responsible for toggling between the light and dark themes. We provide the theme value and the toggleTheme function through the context provider.
Now, let's consume the theme context in a component using the useContext hook:
// ThemeButton.js
import React, { useContext } from 'react';
import ThemeContext from './ThemeContext';
const ThemeButton = () => {
const { theme, toggleTheme } = useContext(ThemeContext);
return (
<button
style={{ backgroundColor: theme === 'dark' ? 'black' : 'white' }}
onClick={toggleTheme}
>
Toggle Theme
</button>
);
};
export default ThemeButton;
In the above component, we use the useContext hook to access the theme and toggleTheme function from the theme context. The button's background color is set based on the current theme value, and the onClick event triggers the toggleTheme function to toggle the theme.
By using the useContext hook, we can consume the context values directly within the component without the need for intermediate prop passing. This simplifies the code and improves its readability.
- Example: User Authentication Context
Let's consider another example of a user authentication context. Assume we have an application where the user can log in and log out, and we want to share the user's authentication status across different components.
First, set up the user authentication context using createContext():
// UserContext.js
import { createContext } from 'react';
const UserContext = createContext();
export default UserContext;
Next, create an authentication provider component that manages the user authentication state and provides it to the consuming components:
// UserProvider.js
import React, { useState } from 'react';
import UserContext from './UserContext';
const UserProvider = ({ children }) => {
const [user, setUser] = useState(null);
const loginUser = (userData) => {
setUser(userData);
};
const logoutUser = () => {
setUser(null);
};
return (
<UserContext.Provider value={{ user, loginUser, logoutUser }}>
{children}
</UserContext.Provider>
);
};
export default UserProvider;
In this example, we use the useState hook to manage the user state. The loginUser function is responsible for setting the user data upon successful login, and the logoutUser function clears the user data upon logout. We provide the user object and the login/logout functions through the
context provider.
Now, let's consume the user authentication context in a component using the useContext hook:
// UserProfile.js
import React, { useContext } from 'react';
import UserContext from './UserContext';
const UserProfile = () => {
const { user, logoutUser } = useContext(UserContext);
return (
<div>
<h1>Welcome, {user?.name}</h1>
<button onClick={logoutUser}>Logout</button>
</div>
);
};
export default UserProfile;
In the above component, we use the useContext hook to access the user object and the logoutUser function from the user authentication context. We display the user's name and provide a button that triggers the logoutUser function upon clicking.
By utilizing the useContext hook, we can easily consume the user authentication context values and manage the user's authentication status across multiple components in a convenient and efficient manner.
These examples demonstrate how the useContext hook allows us to consume context values without the need for prop drilling. It simplifies the sharing and management of data across components, making our code more concise and readable.
Benefits of Using useContext
Using the useContext hook in React Context offers several advantages:
Simplified access to context values: With the useContext hook, we can directly access context values in functional components, eliminating the need for intermediate prop passing or using higher-order components.
Avoiding prop drilling: Prop drilling can become complex and cumbersome when dealing with deeply nested components. By utilizing the useContext hook, we can consume context values without the need to pass them through intermediate components, reducing component coupling.
Enhanced code readability and maintainability: Context values can be accessed directly within components, making the code more readable and easier to understand. It also simplifies the maintenance of code, as changes to context values automatically trigger component updates.
Best Practices and Considerations
When using the useContext hook, it is important to keep the following best practices and considerations in mind:
Separating concerns and creating separate contexts for different data: If your application has multiple sets of related data, it's best to create separate contexts for each data set. This helps in keeping the code organized and improves the reusability of components.
Avoiding unnecessary context updates: Context updates can cause unnecessary re-renders of components. To optimize performance, ensure that context updates only occur when necessary. Consider using memoization techniques or memoized selectors to prevent unnecessary re-renders.
Conclusion
The useContext hook provides a convenient way to access and utilize context values in React applications, eliminating the need for prop drilling and improving code readability. By leveraging this powerful hook, you can create more modular, maintainable, and reusable components, enhancing the overall development experience.
By understanding and implementing the useContext hook effectively, you can take full advantage of React Context and create dynamic and interconnected applications with ease.
Subscribe to my newsletter
Read articles from Darshana Mallick directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Darshana Mallick
Darshana Mallick
I am a Web Developer and SEO Specialist.