.Map() in React.
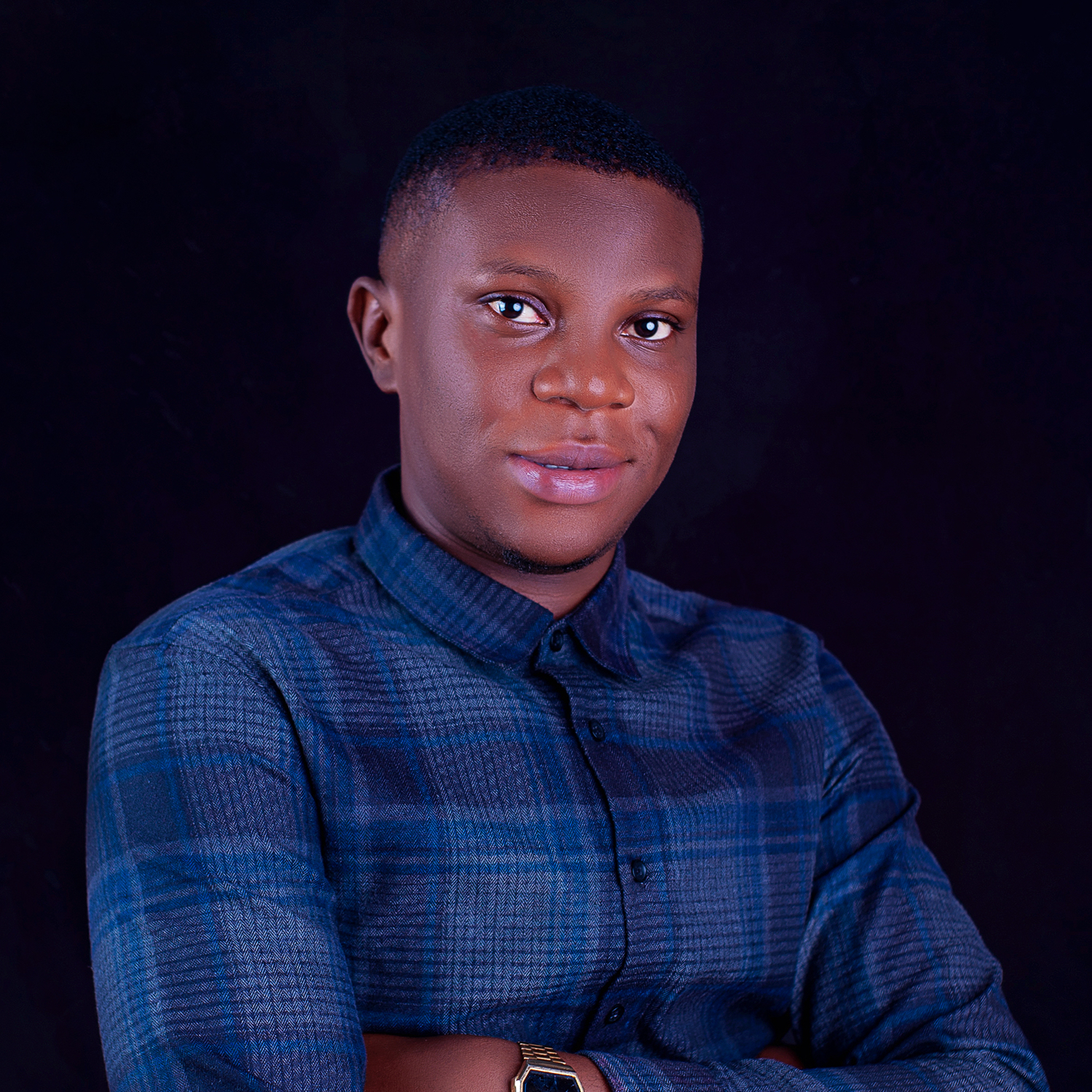
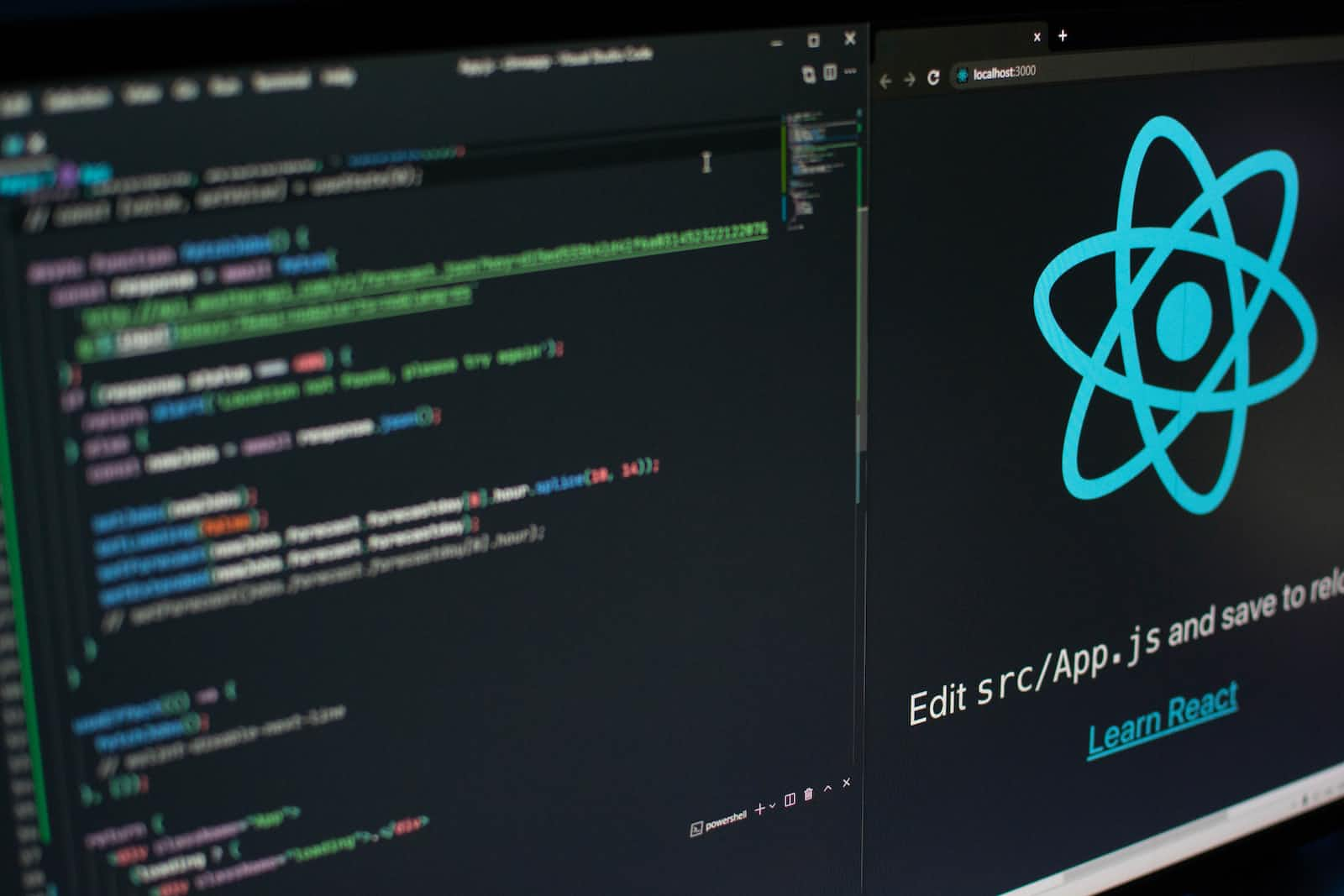
I had a hard time understanding JavaScript's mapping functions until I came across a project using React (a JavaScript framework). Through this project and some online resources, I learned more about mapping. This is one of the many reasons experienced developers tell junior developers to create more projects instead of watching thousands of programming tutorials.
In this article, I will explain how to use react mapping and when to use it. I know so many are like me, I will carefully explain so we can understand it
We will learn the following;
What's mapping?
How to use mapping in our React Project
When to use Mapping in React
WHAT'S MAPPING?
A map is a data collection type where data is stored in the form of pairs. It contains a unique key. The value stored in the map must be mapped to the key. We cannot store a duplicate pair in the
map()
. It is because of the uniqueness of each stored key. It is mainly used for fast searching and looking up data.
A .map()
is used when you have a collection of data and you want to do something to the data without picking the data one after the other. Let's assume you have 10 fruits in a basket and you want to slice all the fruits, you would pick the fruit one after the other to slice them. Now a .map()
is a magical knife you send to the basket to slice all the 10 fruits in the basket at once, it works like magic. That's .map() for you, let's look at a few examples below.
const Baskets = [
{Fruits:'Banana', id:1},
{Fruits:'Apple', id:2},
{Fruits:'Mango', id:3},
{Fruits:'Pawpaw', id:4},
];
console.log(Baskets[0]); // {Fruits:'Banana', key:1}
console.log(Baskets[1].Fruits); // 'Apple'
console.log(Baskets[2].id); // 3
Explanation:
1.) I declared a variable of arrays called Baskets
each element in the array is an object representing a fruit basket. Each fruit basket object has two properties: Fruits
and id
. And the fruits contain Banana, Apple, Mango, and Pawpaw. While, the key has numbers 1, 2, 3, 4.
2.) We can't list all the fruits in the basket at once, so to list all the fruits in the basket at once this where the magician .map()
comes in;
when writing .map()
function you need to keep in mind;
the Plural variable .map(the singular variable\=> (changes you to affect the elements in the variable ) e.g;
Baskets.map(basket => basket.Fruits);
const Baskets = [
{Fruits:'Banana', id:1},
{Fruits:'Apple', id:2},
{Fruits:'Mango', id:3},
{Fruits:'Pawpaw', id:4},
];
const fruitNames = Baskets.map(basket => basket.Fruits);
console.log(fruitNames);
//output: ['Banana', 'Apple', 'Mango', 'Pawpaw']
We list all the fruits in the basket out all at once using the;
const fruitNames = Baskets.map(basket => basket.Fruits); console.log(fruitNames);
How to use .Map()
in our React Project
.map()
in a react component is so cool and makes our code clean and also makes the project less-stress project. Look at the example below;In the lines of code below, the array of React JSX elements represents the list of fruits, the output would not be directly visible in the console. Instead, you would typically render the listFruits
array in a React component or a webpage to see the rendered list.
If you were to render the listFruits
array in a React component using JSX, it might look something like this:
import React from 'react';
const Baskets = [
{Fruits: 'Banana', id: 1},
{Fruits: 'Apple', id: 2},
{Fruits: 'Mango', id: 3},
{Fruits: 'Pawpaw', id: 4},
];
const FruitList = () => {
const listFruits = Baskets.map(Basket => (
<li key={Basket.id}>{Basket.Fruits}</li>
));
return (
<div>
<h1>List of Fruits</h1>
<ul>
{listFruits}
</ul>
</div>
);
};
export default FruitList;
import React from 'react';
import ReactDOM from 'react-dom';
import FruitList from './FruitList';
ReactDOM.render(
<FruitList />,
document.getElementById('root')
);
In this example, you noticed the <li>
has an attribute of key={Basket.id}
the key
attribute is used in React when rendering lists of elements to help React identify each individual item in the list and efficiently update the components when the list changes.
In the code example, I provided the key
attribute is set to the id
property of each fruit object in the Baskets
array.
The key
attribute helps React keep track of which items have changed, been added, or been removed from the list. It helps with the reconciliation process when React needs to update the UI efficiently.
When rendering lists in React, it is recommended to assign a unique key
to each item in the list. In this case, using the id
property as the key
is a common practice because it provides a unique identifier for each fruit object.
Having a unique key
for each list item helps React optimize the rendering process by efficiently updating only the necessary components when the list changes, rather than re-rendering the entire list. It also helps with performance and avoiding potential issues related to list item ordering or duplication.
THE OUTPUT OF THE RENDERED COMPONENT WOULD BE;
List of Fruits
- Banana
- Apple
- Mango
- Pawpaw
When to use .map()
in React
Mapping is commonly used in React when you have an array of data and you need to render components or elements dynamically based on that data. Here are a few scenarios where mapping is often used in React:
Rendering a list: When you have an array of items and you want to render a list of components or elements for each item in the array, you can use mapping. For example, rendering a list of blog posts, displaying a list of products, or showing a list of user profiles.
import React from 'react'; const BlogPostList = ({ posts }) => { return ( <ul> {posts.map((post) => ( <li key={post.id}>{post.title}</li> ))} </ul> ); }; // Usage: const posts = [ { id: 1, title: 'React Hooks Tutorial' }, { id: 2, title: 'Introduction to JSX' }, { id: 3, title: 'State Management in Redux' }, ]; ReactDOM.render(<BlogPostList posts={posts} />, document.getElementById('root'));
Creating dynamic components: If you have a variable number of components to render based on certain conditions or data, mapping can be useful. You can map over an array and dynamically render components based on the data.
import React from 'react'; const DynamicComponentList = ({ components }) => { return ( <div> {components.map((Component, index) => ( <Component key={index} /> ))} </div> ); }; // Usage: const components = [ComponentA, ComponentB, ComponentC]; ReactDOM.render(<DynamicComponentList components={components} />, document.getElementById('root'));
Generating options for a select input: If you have an array of options and you want to generate
<option>
elements for a<select>
input, you can use mapping to iterate over the array and create the options dynamically.import React from 'react'; const SelectOptions = ({ options }) => { return ( <select> {options.map((option) => ( <option key={option.value} value={option.value}> {option.label} </option> ))} </select> ); }; // Usage: const options = [ { value: 'apple', label: 'Apple' }, { value: 'banana', label: 'Banana' }, { value: 'orange', label: 'Orange' }, ]; ReactDOM.render(<SelectOptions options={options} />, document.getElementById('root'));
Creating table rows: When you have tabular data and want to render rows dynamically based on the data, mapping can be used. Each item in the array can represent a row, and you can map over the array to create table rows.
import React from 'react'; const TableRow = ({ data }) => { return ( <tr> <td>{data.name}</td> <td>{data.age}</td> <td>{data.location}</td> </tr> ); }; const Table = ({ rows }) => { return ( <table> <thead> <tr> <th>Name</th> <th>Age</th> <th>Location</th> </tr> </thead> <tbody> {rows.map((row) => ( <TableRow key={row.id} data={row} /> ))} </tbody> </table> ); }; // Usage: const rows = [ { id: 1, name: 'John Doe', age: 30, location: 'New York' }, { id: 2, name: 'Jane Smith', age: 25, location: 'London' }, { id: 3, name: 'Bob Johnson', age: 40, location: 'Sydney' }, ]; ReactDOM.render(<Table rows={rows} />, document.getElementById('root'));
Mapping allows you to iterate over an array, perform transformations or computations on each item, and return an array of components or elements that can be rendered by React. It helps make your React components more dynamic and flexible by rendering elements based on the provided data.
We have come to the end of this article, I hope this helps. If you get stuck you can hit me up on Twitter, Linkedin, and WhatsApp.
Subscribe to my newsletter
Read articles from Olusola Samuel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
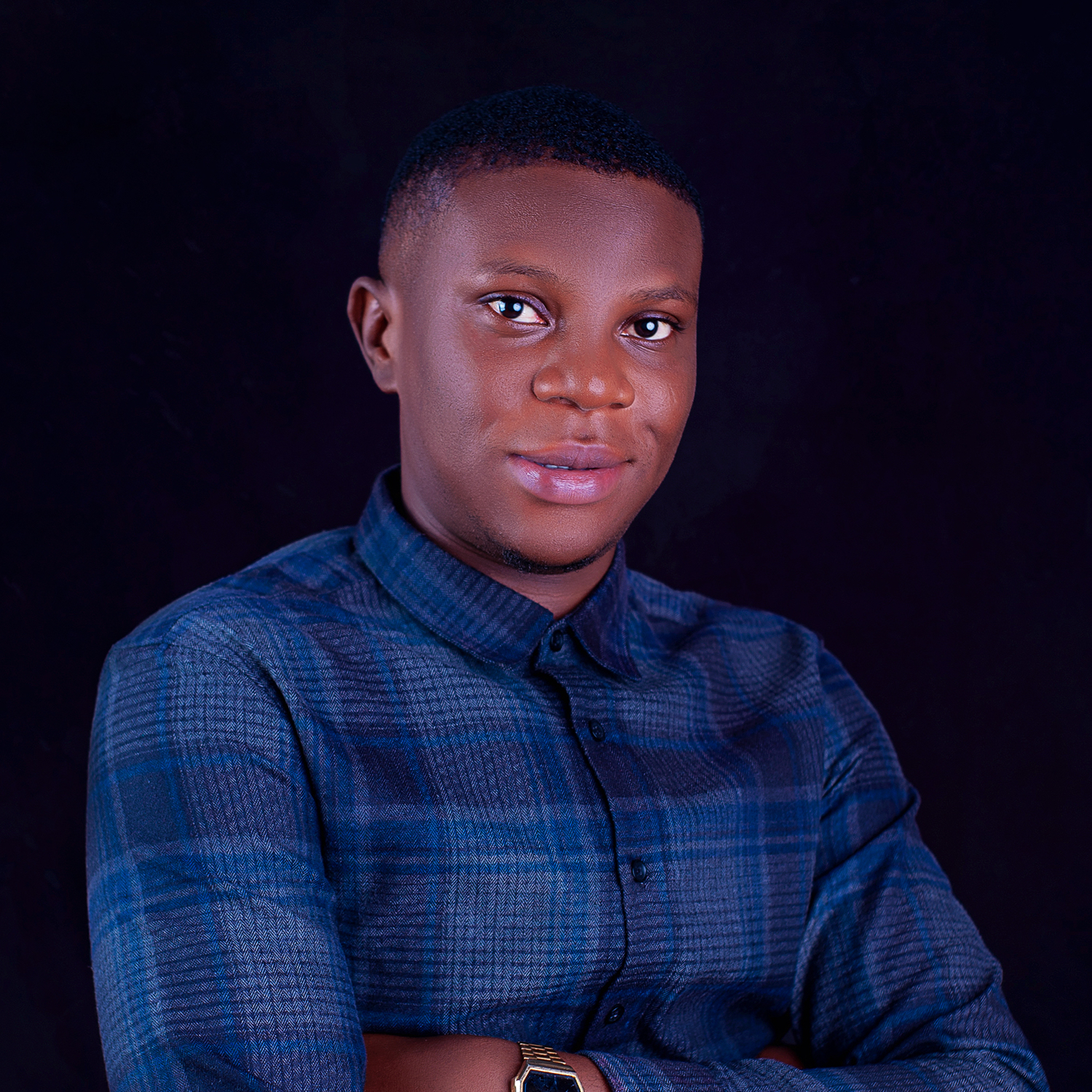