Onchange vs Oninput
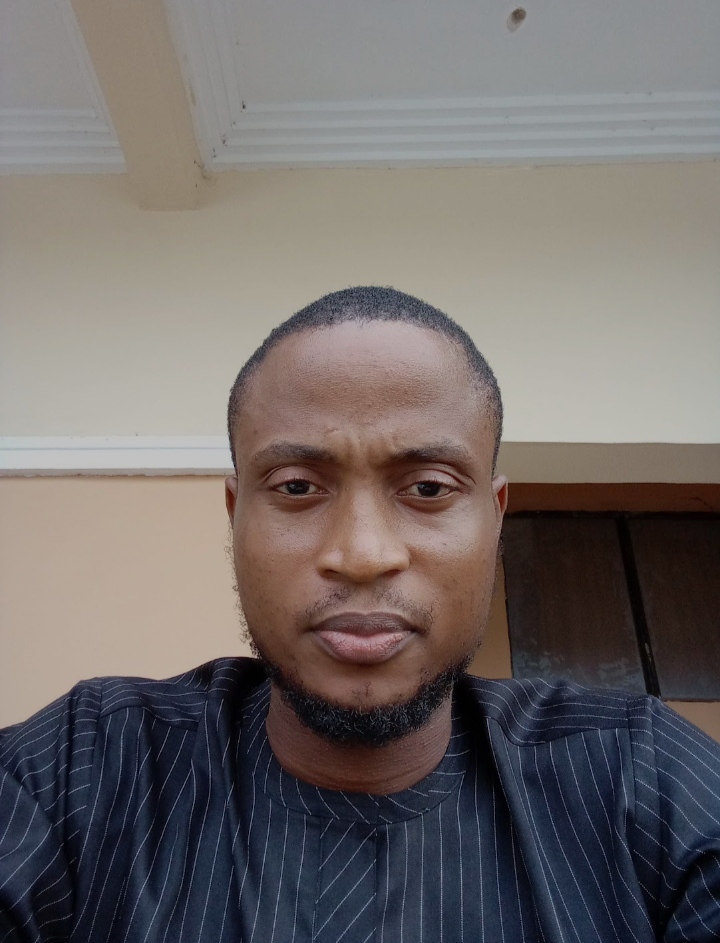
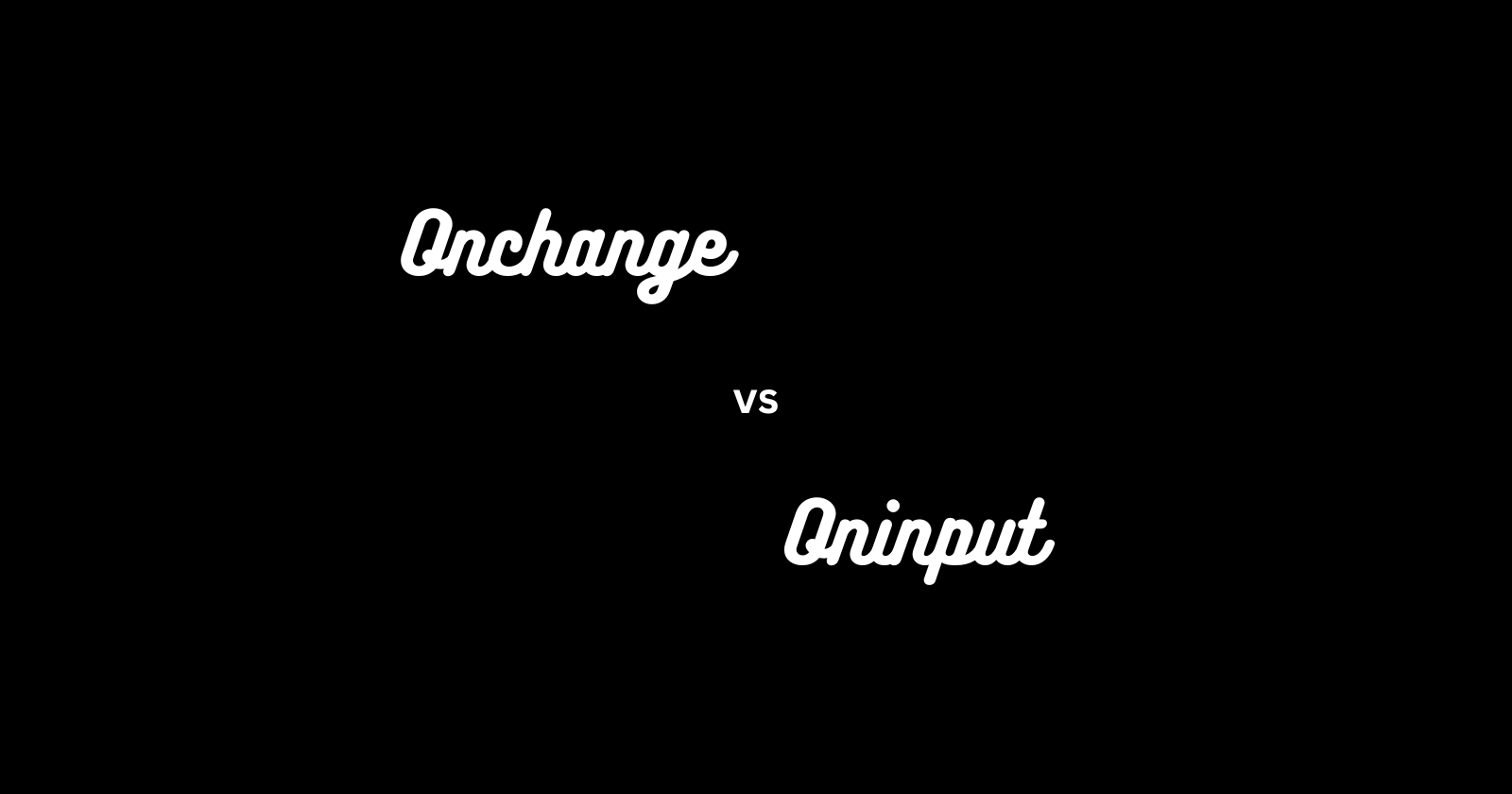
Onchange
and Oninput
are two HTML attributes that are often confused with each other. Both are used within HTML tags or programmatically in JavaScript to specify the event handler code that should run when a certain event is triggered. This article will briefly discuss the use cases for both attributes, as well as the similarities and differences between them.
Onchange
The onchange
attribute detects and responds to change events on DOM elements. These events are triggered when a user modifies the element's value or causes the element to lose focus by actions such as:
Exiting or tabbing out of an input field
Hitting the
enter
keySelecting an item from a list of
radio
buttons
To better understand how the onchange
attribute works, consider the code snippet below:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<link rel="stylesheet" type="text/css" href="sample.css"/>
</head>
<body>
<div class="style">
<h1>onchange Event Example</h1>
<h2>Enter a value below</h2>
<input type="text" id="input" onchange="testFunction()" >
<p id="grab"></p>
</div>
<script>
testFunction = () => {
let hold = document.getElementById("input").value;
document.getElementById("grab").innerHTML = "You just entered the value: " + hold;
}
</script>
</body>
</html>
The input
element in the code above has an onchange
attribute that triggers a JavaScript function named testFunction
when a change event occurs on the input element. This function displays the value entered into the input field in the browser window. Run the code on your device to observe that the onchange event fires only after pressing the enter
key or tabbing out of the input field.
figure 1: onchange attribute in action.
Oninput
The oninput
attribute tracks and responds to input events in an input field. Unlike onchange
, which triggers after a change occurs, oninput
is more dynamic and calls the associated JavaScript function with every change made to the input field, including key presses and pasting text. To better understand this concept, examine the code snippet below
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<link rel="stylesheet" type="text/css" href="untitled.css"/>
</head>
<body>
<div class="style">
<h1>oninput Event Example</h1>
<h3>Enter any value in the text area below:</h3>
<input type="text" id="input" placeholder="enter text here" oninput="testFunction()">
<p id="grab"></p>
<span>Notice how the values are printed in real time above.</span>
</div>
<script>
testFunction = () => {
let hold = document.getElementById("input").value;
document.getElementById("grab").innerHTML = "You entered: " + hold;
}
</script>
</body>
</html>
In the code above, the input
element has an oninput
attribute that calls the JavaScript function testFunction
in response to input events on the element. When called, testFunction
displays the values entered into the input field in the browser as they are typed or pasted.
figure 2: oninput attribute in action.
Use Cases
The choice between oninput
and onchange
depends on the nature of the event and the desired behavior. As demonstrated in the preceding sections, oninput
is used for dynamic reactions to user input in real-time, such as typing or pasting in an input field. In contrast, onchange
detects and responds to changes in DOM elements, such as when an element loses focus or when an item is selected from a list of options.
Similarities Between Onchange and Oninput
Both onchange
and oninput
are HTML attributes used to handle events in JavaScript. They work by triggering a predefined function in response to specific user actions within a browser window.
Differences Between Onchange and Oninput
The table below outlines the key differences between the onchange
and oninput
attributes:
Onchange | Oninput |
Listens for change events | Listens for input events |
Calls a JavaScript function after a change event occurs in a DOM element | Calls a JavaScript function with each key press or when text is pasted into an input field |
Comparing Onchange and Oninput Attributes
The table below compares how the onchange
and oninput
attributes respond under various conditions:
Onchange | Oninput | |
Condition | ||
1. User tabs out of a text area | Fires | Does not fire |
2. The text area gets input | Does not fire | Fires |
3. User hits the enter key | Fires | Does not fire |
4. User selects an option from a list of radio buttons | Fires | Does not fire |
5. User marks a checkbox | Fires | Does not fire |
Conclusion
This article explored the onchange
and oninput
attributes in HTML, highlighting their differences, similarities, and appropriate use cases. While there are other HTML attributes for handling browser events, this article focused on these two due to the common confusion they create for new developers. To deepen your understanding, consider experimenting with both onchange
and oninput
in your projects to see firsthand how they behave in different scenarios.
Subscribe to my newsletter
Read articles from Ken Udeh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
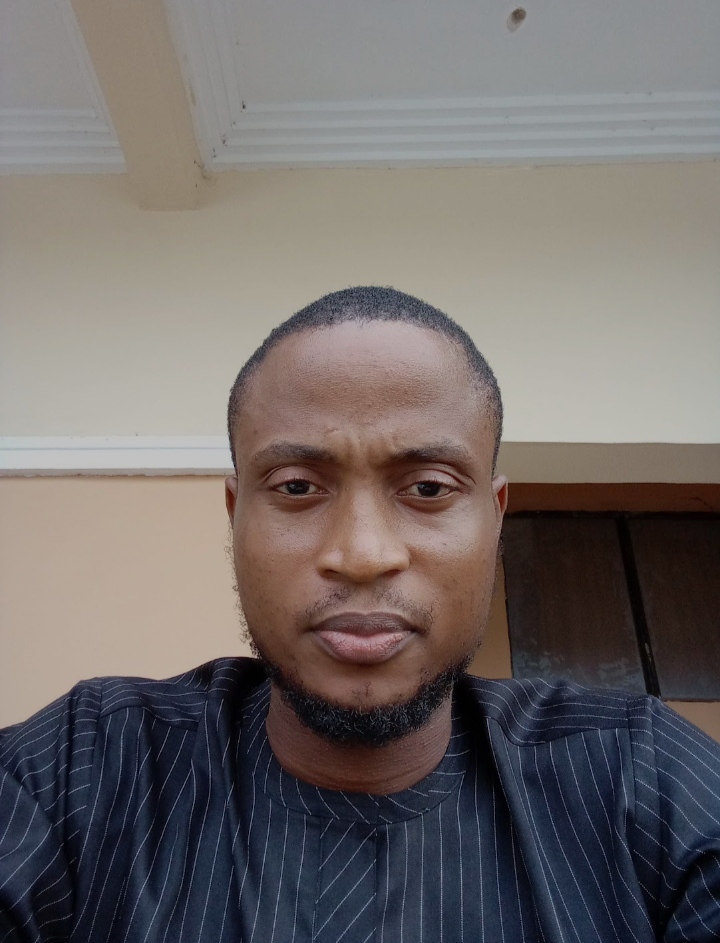
Ken Udeh
Ken Udeh
API technical writer.