Zend Framework 2 Doctrine 2 one-to-many checkbox hydration
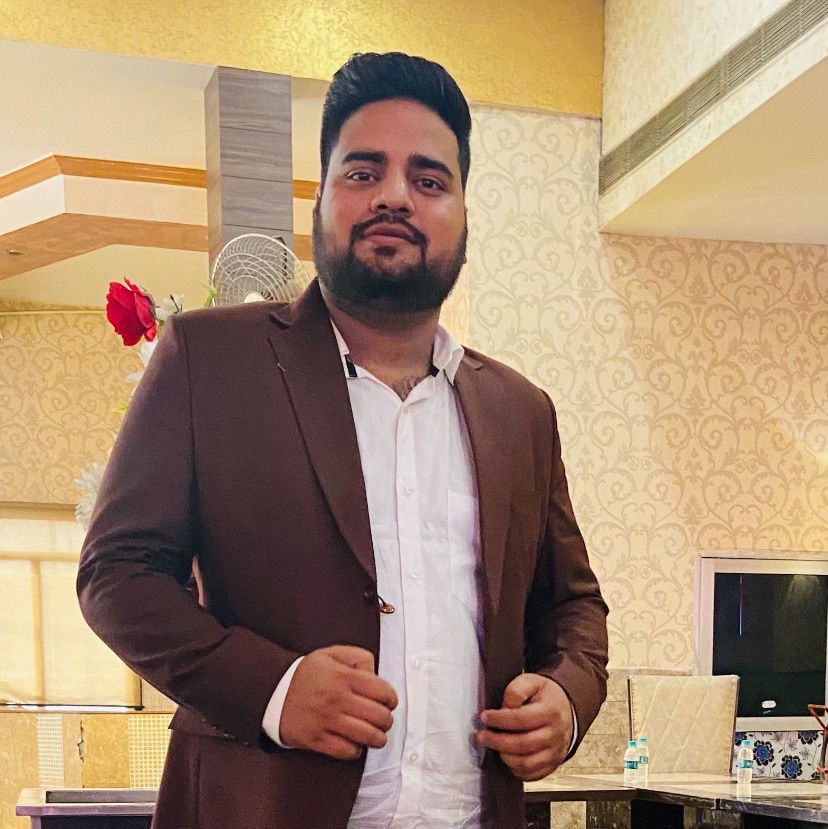
When using Zend Framework 2 and Doctrine 2 with a one-to-many relationship and checkboxes for hydration, you can follow these steps:
- Define Entities: Create two entities, let's say
User
andRole
. TheUser
entity will have a one-to-many relationship with theRole
entity.
namespace Application\Entity;
use Doctrine\ORM\Mapping as ORM;
use Doctrine\Common\Collections\ArrayCollection;
/**
* @ORM\Entity
* @ORM\Table(name="users")
*/
class User
{
// ... entity definitions ...
/**
* @ORM\OneToMany(targetEntity="Role", mappedBy="user", cascade={"persist", "remove"})
*/
protected $roles;
public function __construct()
{
$this->roles = new ArrayCollection();
}
// ... getters and setters ...
}
/**
* @ORM\Entity
* @ORM\Table(name="roles")
*/
class Role
{
// ... entity definitions ...
/**
* @ORM\ManyToOne(targetEntity="User", inversedBy="roles")
* @ORM\JoinColumn(name="user_id", referencedColumnName="id")
*/
protected $user;
// ... getters and setters ...
}
- Create Form: Create a form for the
User
entity that includes checkboxes for selecting roles.
namespace Application\Form;
use Zend\Form\Form;
use Zend\Form\Element;
use DoctrineModule\Form\Element\ObjectMultiCheckbox;
use DoctrineModule\Persistence\ObjectManagerAwareInterface;
use Doctrine\Common\Persistence\ObjectManager;
class UserForm extends Form implements ObjectManagerAwareInterface
{
protected $objectManager;
public function __construct(ObjectManager $objectManager)
{
parent::__construct('user-form');
$this->setObjectManager($objectManager);
// ... add other form elements ...
$rolesField = new ObjectMultiCheckbox('roles');
$rolesField->setOptions([
'object_manager' => $objectManager,
'target_class' => 'Application\Entity\Role',
'property' => 'id',
]);
$this->add($rolesField);
}
public function setObjectManager(ObjectManager $objectManager)
{
$this->objectManager = $objectManager;
}
public function getObjectManager()
{
return $this->objectManager;
}
}
- Controller Actions: In your controller actions, handle the form submission and populate the related entities.
namespace Application\Controller;
use Zend\Mvc\Controller\AbstractActionController;
use Application\Form\UserForm;
use Application\Entity\User;
class UserController extends AbstractActionController
{
protected $objectManager;
public function indexAction()
{
$form = new UserForm($this->getObjectManager());
if ($this->getRequest()->isPost()) {
$user = new User();
$form->bind($user);
$form->setData($this->getRequest()->getPost());
if ($form->isValid()) {
$this->getObjectManager()->persist($user);
$this->getObjectManager()->flush();
// handle successful form submission
// redirect or display success message
}
}
return ['form' => $form];
}
public function setObjectManager(ObjectManager $objectManager)
{
$this->objectManager = $objectManager;
}
public function getObjectManager()
{
return $this->objectManager;
}
}
Make sure to configure your Doctrine 2 and Zend Framework 2 environments properly, including database connectivity, entity mapping, and dependency injection for the entity manager.
With these steps, you should be able to create a form with checkboxes for a one-to-many relationship using Zend Framework 2 and Doctrine 2.
Subscribe to my newsletter
Read articles from Gaurav Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
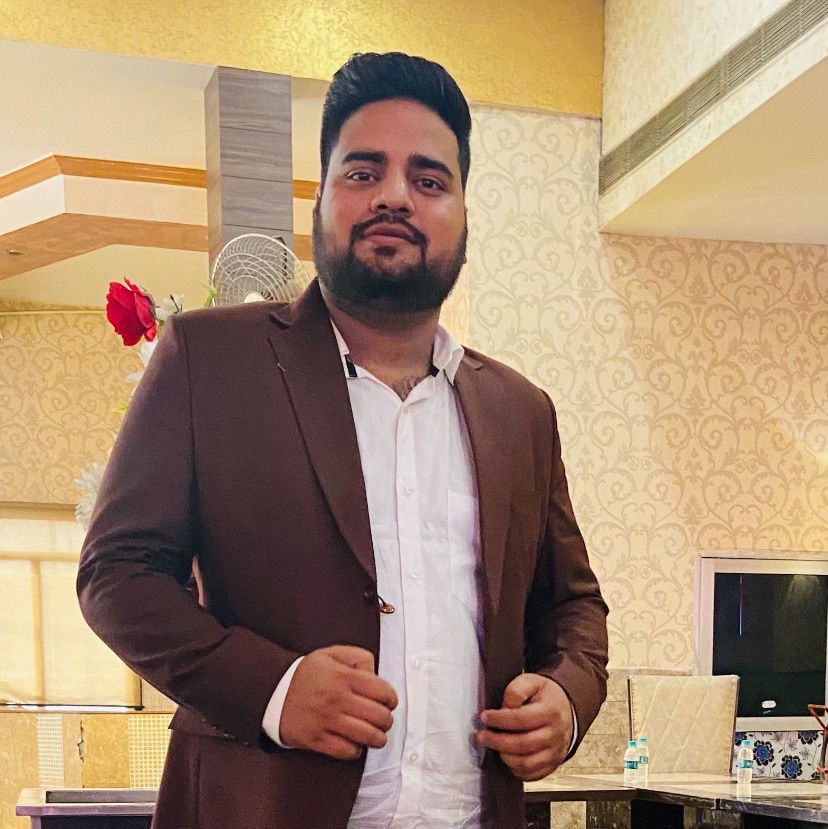
Gaurav Kumar
Gaurav Kumar
Hello, I'm Gaurav Kumar PHP Framework Expert with 8+ Years of Experience I am an experienced PHP framework expert with over 8 years of dedicated experience. Specializing in frameworks like Laravel, Symfony, CodeIgniter, and Zend. With a deep understanding of web development intricacies, I excel in developing custom web applications, e-commerce platforms, content management systems, and API integrations. I am well-versed in modern development practices, including OOP, MVC architecture, RESTful APIs, and version control systems. If you are in need of any help on PHP frameworks, You can contact me. I will love to help you! ๐