Understanding extension methods in flutter,

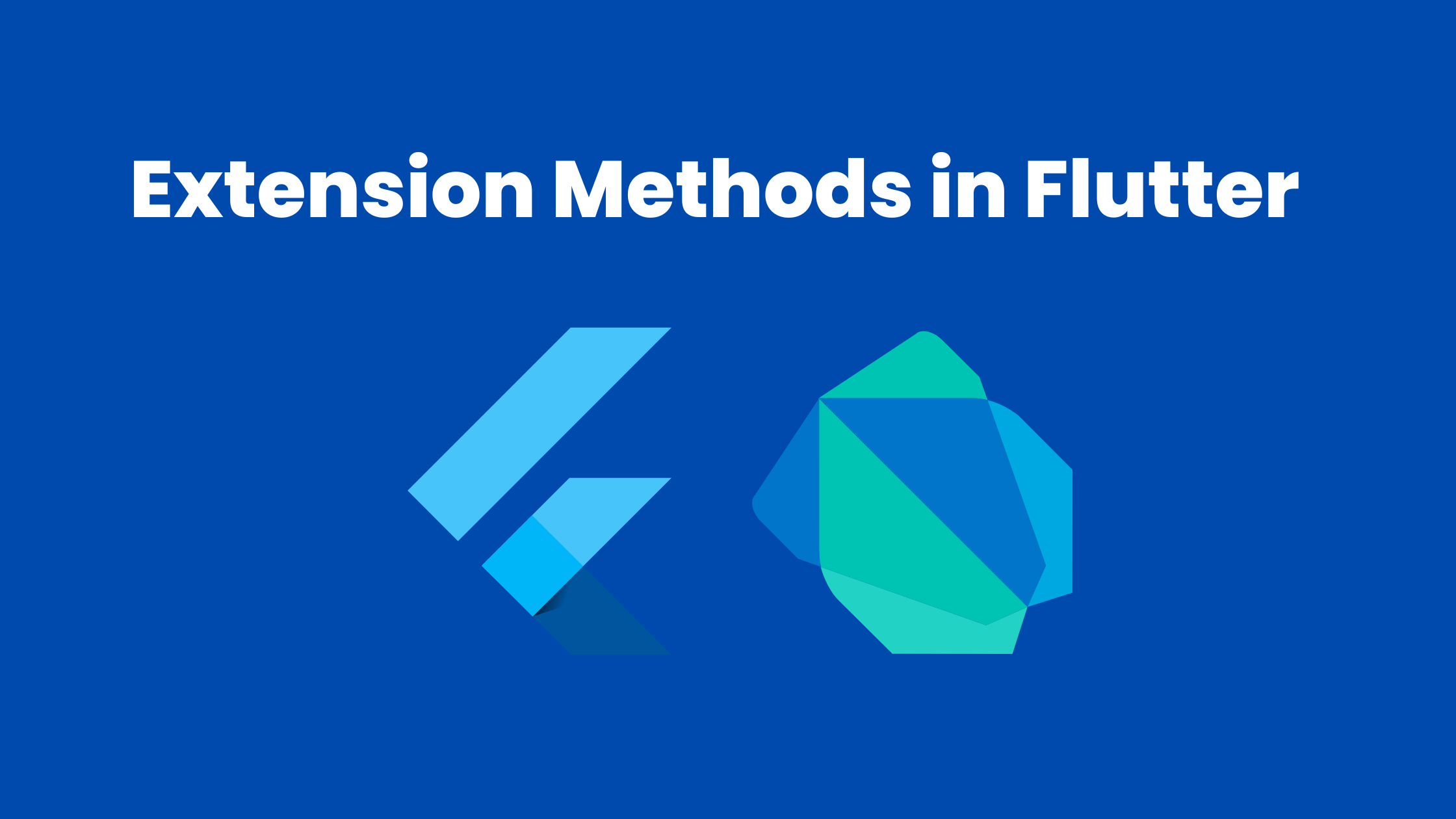
Have you ever thought of capitalizing the first letter of a word? Well, you might have thought that. Most beginner developers, don't know how to make the implementation more efficient using extension methods in Dart.
What's the hustle without extension methods?
Well, let's assume, there are 4 different screens in the App, for which there are 4 different dart files. You managed to capitalize the first letter, in the first file. However, as the project files and projects get large, it's difficult to manage the functions, or we will end up repeating ourselves, which leads to the violation of the DRY ( Do not Repeat Yourself) Principle.
How do extension methods help to write more efficient code?
Introduction,
Flutter, a popular cross-platform development framework, offers a powerful feature called "extensions." Extensions allow developers to add new functionality to existing classes or types without modifying their original implementation. Similar to extension methods in other programming languages, Flutter's extensions provide a way to extend the behavior of a class, enhancing code organization and reusability.
In Flutter, extension methods can be used to add new functionality or modify existing behavior of classes or types. Here are some common things that can be achieved using extension methods in Flutter:
Formatting and Manipulating Strings,
Date and Time Operations,
Validating and Parsing Inputs,
Converting Data Types,
List and Collection Manipulation,
Utility Functions,
Widget Enhancements,
Creating extension methods,
To create an extension in Flutter, follow these steps:
Start by defining a new extension using the
extension
keyword, followed by the extension's nameon
<datatype you want your extension to operate on >
. For example:extension StringExtensions on String { //extension 1 //extension 2 }
Within the extension, you can define various extension methods. These methods will be available on any instance of the extended type. For instance:
extension StringExtensions on String { String capitalize() { return this.substring(0, 1).toUpperCase() + this.substring(1); } }
In the above example, an extension method named capitalize()
is added to the String
class. This method capitalizes the first letter of a string.
Using the extension methods,
After defining the extension, you can easily use it within your Flutter application. Here's how:
Import the file where the extension is defined into the file where you want to use it. For instance:
import 'extensions.dart'; // import the path of your extension file void main() { String name = 'john'; String capitalized = name.capitalize(); print(capitalized); // Output: John }
Once imported, you can invoke the extension method on an instance of the extended type. In this case,
capitalize()
can be called on anyString
object.
Conclusion,
Extensions in Flutter provide developers with a powerful tool to extend the functionality of existing classes without modifying their original implementation. By adding new methods to classes, developers can enhance code organization, and reusability, and maintain the principle of separation of concerns. Whether it's adding utility methods or modifying behavior, Flutter's extensions offer a flexible and efficient way to customize and extend existing classes.
Remember, when utilizing extensions in your Flutter projects, it's essential to maintain clarity, readability, and adherence to best coding practices. With this knowledge in hand, you can leverage extensions to streamline your development process and create more robust, maintainable Flutter applications.
More Examples,
extension DateTimeExtensions on DateTime {
String format(String format) {
final formatter = DateFormat(format);
return formatter.format(this);
}
int get differenceInDays {
final now = DateTime.now();
return this.difference(now).inDays;
}
// Other date and time operations...
}
extension StringExtensions on String {
bool isValidEmail() {
final emailRegex = RegExp(r'^[\w-]+(\.[\w-]+)*@([\w-]+\.)+[a-zA-Z]{2,7}$');
return emailRegex.hasMatch(this);
}
int toInt() {
return int.tryParse(this) ?? 0;
}
// Other validation and parsing methods...
}
extension WidgetExtensions on Widget {
Widget withPadding(EdgeInsets padding) {
return Padding(padding: padding, child: this);
}
Widget withBackground(Color color) {
return Container(color: color, child: this);
}
// Other widget enhancement methods...
}
Thank you for your valuable time. See you in the next article. Peace!
Subscribe to my newsletter
Read articles from Rabin Acharya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Rabin Acharya
Rabin Acharya
Building Apps🛠️🔥 with Flutter🚀