Java Threads & Concurrency API — Part I
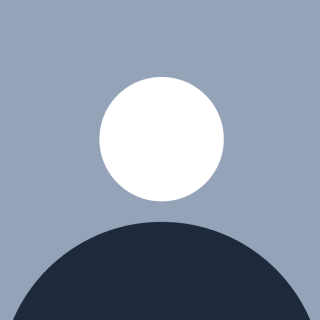
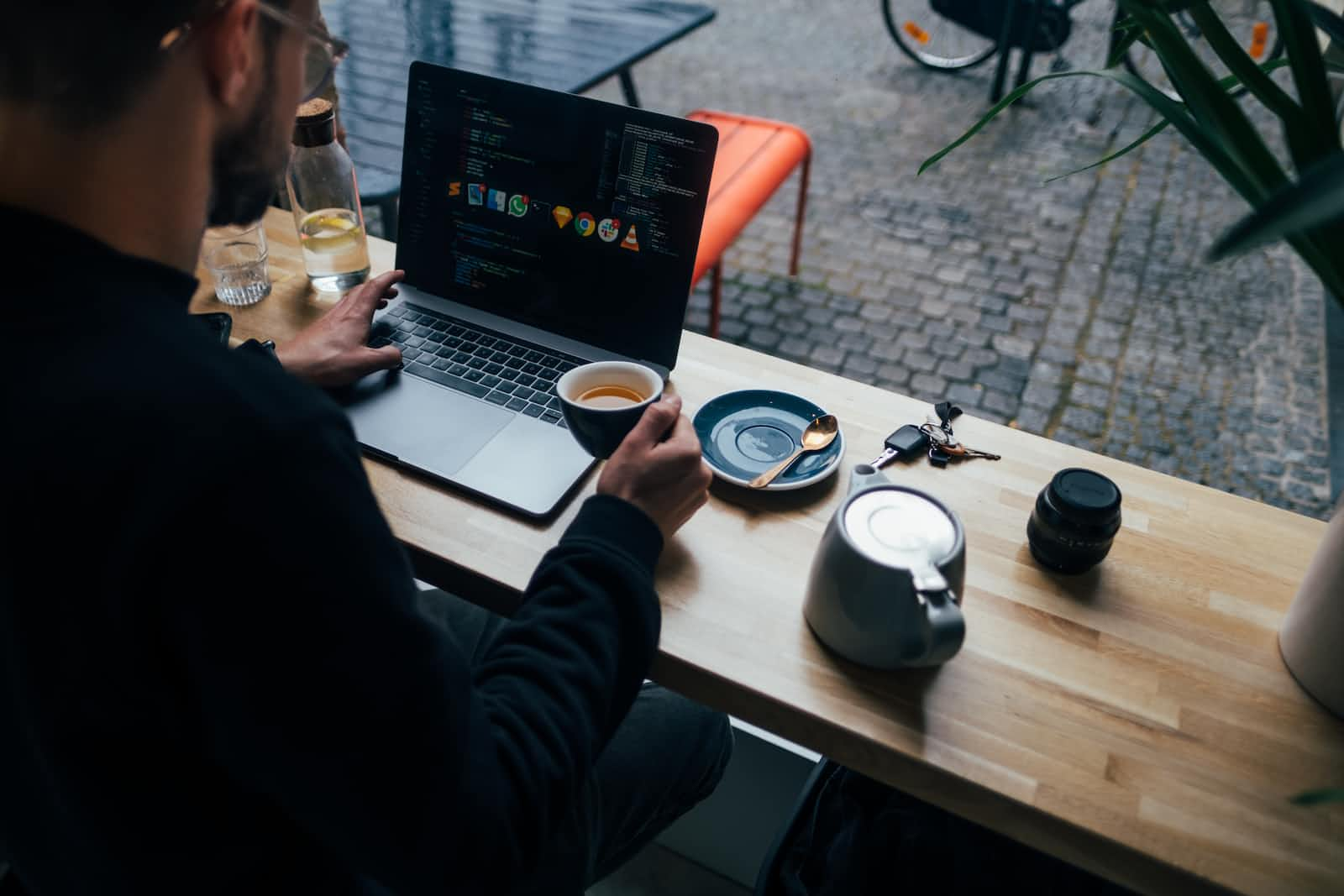
What is a Thread?
Thread is the smallest unit of execution that can be scheduled by the OS.
What is a Task?
A task is a single unit of work performed by a Thread.
But then how do we define this work (or create a task for a thread)?
- A common way is to use a Runnable Interface (we will implement it using Lambda expression)
Runnable runnable = () -> System.out.println("I am another Thread");
Thread anotherThread = new Thread(runnable);
anotherThread.start();
- Or you create a Java class that implements a Runnable interface and then pass the instance of this class while creating an instance of Thread.
Thread someThread =
new Thread(new AUserDefinedClassThatImplementsRunnable());
someThread.start();
- With a class implementing Callable Interface. Instead of overriding
run()
method, for this one we overridecall()
method, which unlikerun()
can throw an exception and can return a generic value (a String in the example below)
public class TestCallable implements Callable {
public static void main(String[] args) throws Exception {
TestCallable callable = new TestCallable();
String message = callable.call();
System.out.println(message);
}
@Override
public String call() throws Exception {
return "Task creation for thread using Callable";
}
}
Or by creating a class that extends the Thread class. Then create an instance of this class and invoke “
start()
” method on it. Thread class itself implements a Runnable interface. (Avoid this way of Thread creation)and also by creating Threads with the ExecutorService (discussed in detail in part 2 of this article)
What is the difference between the Runnable and Callable Interfaces?
Runnable interface has an abstract “
run()
” method whereas Callable has an abstract “call()
” method.Runnable interface’s “
run()
” method hasvoid
return type whereas Callable’s abstract “call()
” method can have a generic return type.run() method doesn’t throw an exception while
call()
can throw an exception
@FunctionalInterface
public interface Callable {
V call() throws Exception;
}
//-------------------------------------------------------------
//-------------------------@FunctionalInterface
public interface Runnable {
public abstract void run();
}
What is a Process?
Now, envision a group of related threads executing within the same shared environment. This is what a process resembles.
Types of Threads?
System-defined threads — by the JVM and run in the background of the application (like garbage collection daemon thread)
User-defined threads — created by the application developer to accomplish a certain task
What is Concurrency?
The property of executing multiple threads at the same time is referred to as concurrency.
It can be misleading when we say "at the same time," because in a single-core processor, only one task is executed at any given moment. However, the context switch occurs rapidly enough to create the illusion of multiple tasks being accomplished simultaneously.
(like listening to music while preparing a Word document)
Part II in progress…
Dear Readers,
I hope you are enjoying the content I provide on my blog. As a passionate writer and dedicated software developer, I strive to create valuable and informative articles that resonate with you. Today, I would like to extend an invitation to support my work and help me continue producing high-quality content.
I have set up a Buy Me a Coffee page, a platform that allows readers like you to show their appreciation by making a small donation. Your contribution, no matter how big or small, goes a long way in supporting my efforts and keeping the blog running. You can also sponsor using the links at the bottom of this page.
Subscribe to my newsletter
Read articles from Ish Mishra directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Ish Mishra
Ish Mishra
Welcome to Bits8Byte! I’m Ish, a seasoned Software Engineer with 11+ years of experience in software development, automation, and AI/ML. I have a deep passion for technology, problem-solving, and continuous learning, and I created this blog to share my insights, experiences, and discoveries in the ever-evolving world of software engineering. Throughout my career, I’ve worked extensively with Java (Spring Boot), Python (FastAPI), AI/ML, Cloud Computing (AWS), DevOps, Docker, Kubernetes, and Test Automation frameworks. My journey has led me to explore microservices architecture, API development, observability (OpenTelemetry, Prometheus), and AI-powered solutions. On this blog, you’ll find practical tutorials, in-depth technical discussions, and real-world problem-solving strategies. I’ll also share my experiences working on high-performance microservices, AI applications, cloud deployments, and automation frameworks, along with best practices to help fellow developers and engineers. I encourage you to join the conversation—leave comments, ask questions, and share your thoughts! Let’s learn, innovate, and grow together in this exciting journey of software development.