React Router A Comprehensive Guide

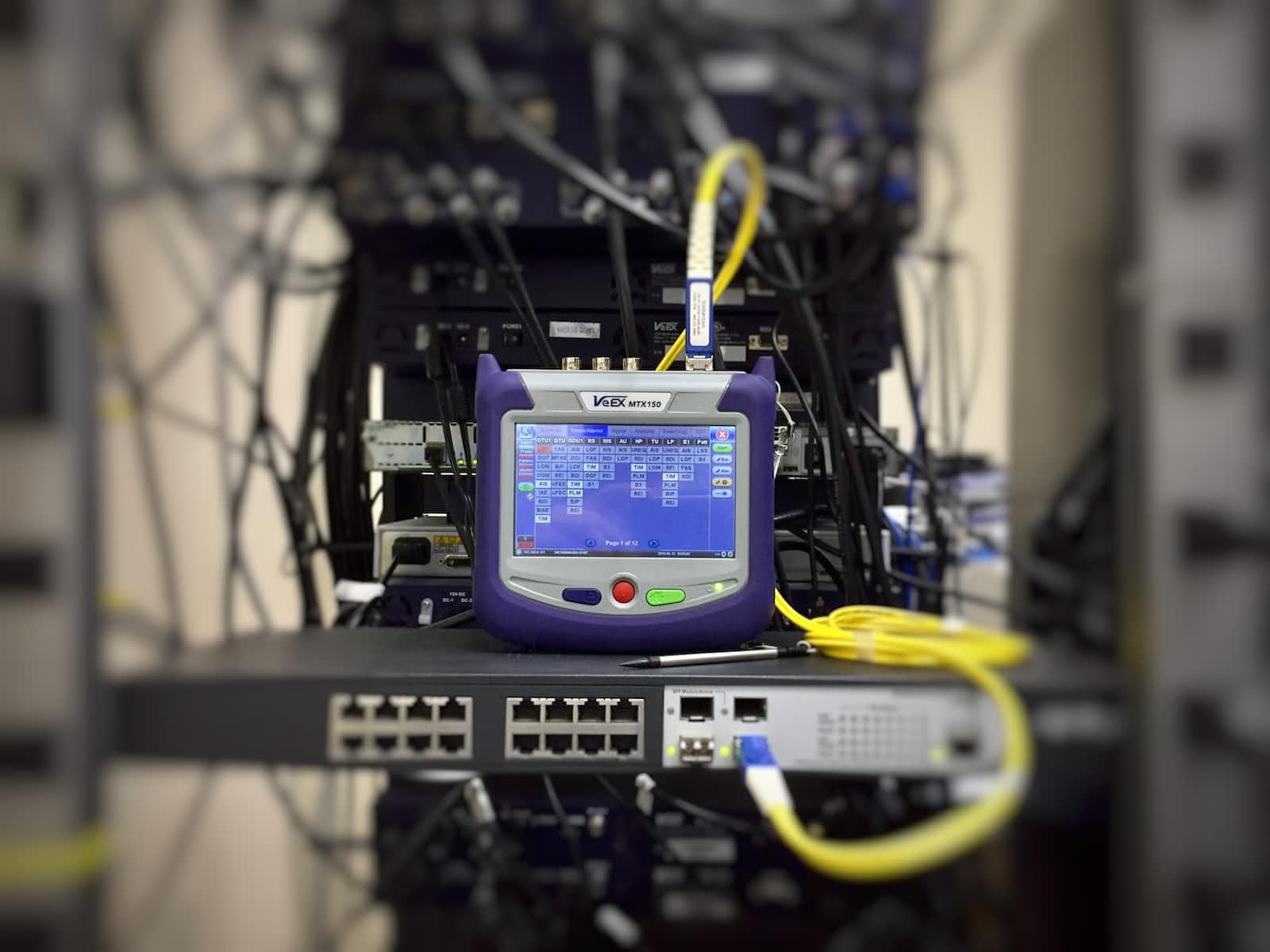
React Router is a powerful library that enables declarative routing in React applications. It allows you to navigate between different pages or views without the need for a full-page refresh. Whether you are building a simple single-page application or a complex multi-page application, React Router provides the tools and functionality you need to handle navigation and rendering of different views.
Why Is React Router Needed?
React Router is needed in React applications for several reasons:
Client-side Routing: React Router allows us to implement client-side routing in our React applications. With client-side routing, the browser doesn't perform a full-page refresh when navigating between different views or pages. Instead, React Router manipulates the URL and updates the corresponding components, resulting in a smoother and more seamless user experience.
Single-Page Applications (SPAs): React Router is particularly useful for building single-page applications (SPAs) where all the content is loaded once, and subsequent navigation happens within the same page. SPAs provide a more fluid user experience by eliminating the need for page reloads and providing faster navigation between different sections of the application.
Dynamic Routing: React Router allows us to define dynamic routes that can handle varying parameters or segments in the URL. This enables us to create routes that can accept parameters such as IDs, usernames, or any other dynamic value. Dynamic routing is essential for applications that need to display different content based on user input or data retrieval.
Nested Routing: React Router supports nested routing, allowing us to create complex application structures with multiple levels of nested routes. This is particularly useful for applications that have nested layouts or component hierarchies, such as dashboards or multi-level navigation menus.
URL Manipulation: React Router provides tools to manipulate the URL programmatically. It allows us to navigate to different routes, modify URL parameters, and even handle history management, such as going back or forward in the browser's history.
Code Organization: React Router promotes a structured and organized approach to managing routes in a React application. It allows us to define routes and their corresponding components in a centralized manner, making it easier to understand and maintain the application's navigation logic.
Now we will dive into the intricacies of React Router and explore how it can be used to create dynamic and interactive single-page applications.
To get started, let's install React Router in our project using npm or yarn. Once installed, we can import the necessary components from the React Router library. The key components we'll work with are <BrowserRouter>
, <Switch>
, and <Route>
.
The <BrowserRouter>
component is the root component that wraps our entire application. It provides the necessary routing functionality for our React application. Inside the <BrowserRouter>
, we can define different routes using the <Route>
component. Each <Route>
component specifies a path and the corresponding component to render when that path is matched.
For example, let's say we have a simple blog application with two pages: a home page and a blog post page. We can define our routes as follows:
import { BrowserRouter, Switch, Route } from 'react-router-dom';
import Home from './components/Home';
import BlogPost from './components/BlogPost';
function App() {
return (
<BrowserRouter>
<Switch>
<Route exact path="/" component={Home} />
<Route path="/blog/:id" component={BlogPost} />
</Switch>
</BrowserRouter>
);
}
In this example, the <Route>
component with exact path="/" component={Home}
specifies that the Home
component should be rendered when the path is exactly /
. The <Route>
component with path="/blog/:id" component={BlogPost}
specifies a dynamic route where the BlogPost
component will be rendered when the path matches /blog/:id
. The :id
parameter allows us to pass a unique identifier for each blog post, which can be accessed within the BlogPost
component.
React Router also provides various ways to navigate between routes within our application. We can use the <Link>
component to create links to different routes, or we can use the history
object provided by React Router to programmatically navigate.
For example, let's say we have a list of blog posts on our home page, and we want to link each post to its corresponding blog post page:
import { Link } from 'react-router-dom';
function Home() {
const blogPosts = [
{ id: 1, title: 'React Basics' },
{ id: 2, title: 'React Hooks' },
{ id: 3, title: 'React Context' },
];
return (
<div>
<h1>Blog Posts</h1>
<ul>
{blogPosts.map((post) => (
<li key={post.id}>
<Link to={`/blog/${post.id}`}>{post.title}</Link>
</li>
))}
</ul>
</div>
);
}
In this example, we use the <Link>
component from React Router to create a link for each blog post. The to
prop specifies the path for the link, which includes the dynamic :id
parameter for each post.
React Router also provides additional features, such as nested routes, route parameters, route guards, and more, to handle more complex routing scenarios. These features allow you to create sophisticated navigation systems and handle authentication or authorization in your application.
By leveraging the power of React Router, you can create seamless and intuitive navigation experiences within your React applications. Whether you're building a simple portfolio website or a complex web application, React Router simplifies the process of managing routes and enables you to create dynamic and interactive single-page applications.
What Is React Router DOM?
React Router DOM is a specific package within the React Router library that provides bindings for using React Router with web applications. It is specifically designed for routing in the browser environment.
React Router DOM includes components and utilities that allow you to define and manage routes, handle navigation, and control the rendering of different components based on the current URL. It works seamlessly with React to enable client-side routing and create single-page applications.
Some key components provided by React Router DOM include:
BrowserRouter: This component provides the foundation for client-side routing and manages the application's history. It uses the HTML5 history API to manipulate the browser's URL and handle navigation events.
Route: This component defines a route and specifies which component should render when the route's path matches the current URL. It can accept props such as
path
,exact
, andcomponent
to configure the route's behavior.Switch: The Switch component is used to render only the first matching Route or Redirect. It is useful when you want to render a single route exclusively, preventing multiple routes from being rendered simultaneously.
Link: This component allows you to create clickable links that navigate to different routes within your application. It generates an
<a>
tag with the appropriate URL, ensuring a seamless navigation experience.Redirect: The Redirect component is used to redirect the user to a different route. It can be used for cases such as authentication, where the user is redirected to a login page if they are not authenticated.
useHistory: This hook provides access to the browser history object, allowing you to programmatically navigate, go back or forward, or manipulate the browser's history stack.
React Router DOM provides an intuitive and declarative API for handling routing in web applications built with React. It simplifies the process of creating dynamic and navigable interfaces, enabling developers to build rich and interactive web experiences.
How to Use React Router DOM?
To use React Router DOM in your React application, you need to follow these steps:
Install the package: Start by installing the React Router DOM package using a package manager like npm or Yarn. Open your terminal and run the following command:
npm install react-router-dom
or
yarn add react-router-dom
Import the necessary components: In the file where you want to set up your routing, import the required components from the
react-router-dom
package. Commonly used components includeBrowserRouter
,Route
,Switch
,Link
, andRedirect
. For example:import { BrowserRouter, Route, Switch, Link, Redirect } from 'react-router-dom';
Wrap your app with the
BrowserRouter
: Wrap your entire application with theBrowserRouter
component to enable routing. This component provides the necessary context for managing the application's history and handling navigation. Place it around your root component in the entry file of your application. For example:import React from 'react'; import ReactDOM from 'react-dom'; import { BrowserRouter } from 'react-router-dom'; import App from './App'; ReactDOM.render( <BrowserRouter> <App /> </BrowserRouter>, document.getElementById('root') );
Define routes: Inside your application, define routes using the
Route
component. TheRoute
component maps a URL path to a specific component to render when the path matches the current URL. Specify the path using thepath
prop and the component to render using thecomponent
prop. For example:import React from 'react'; import { Route } from 'react-router-dom'; import Home from './Home'; import About from './About'; function App() { return ( <div> <Route path="/" exact component={Home} /> <Route path="/about" component={About} /> </div> ); }
Use navigation components: React Router DOM provides components like
Link
andNavLink
to create navigation links within your application. Use these components to create clickable links that navigate to different routes. For example:import React from 'react'; import { Link } from 'react-router-dom'; function Navigation() { return ( <nav> <ul> <li> <Link to="/">Home</Link> </li> <li> <Link to="/about">About</Link> </li> </ul> </nav> ); }
Handle route rendering: Finally, use the
Switch
component to control the rendering of routes. TheSwitch
component renders only the firstRoute
orRedirect
that matches the current URL, ensuring that only one route is rendered at a time. Place your routes inside theSwitch
component. For example:import React from 'react'; import { Route, Switch } from 'react-router-dom'; import Home from './Home'; import About from './About'; import NotFound from './NotFound'; function App() { return ( <div> <Switch> <Route path="/" exact component={Home} /> <Route path="/about" component={About} /> <Route component={NotFound} /> </Switch> </div> ); }
That's it! You have now set up React Router DOM in your React application. You can define routes, create navigation links, and handle different components rendering based on the current URL. Explore the React Router DOM documentation for more advanced features and options to customize your routing implementation.
React Router vs. React Router DOM: the Difference
React Router and React Router DOM are two related packages that serve different purposes within the React Router ecosystem.
React Router:
React Router is the core package that provides the foundation for routing in React applications. It defines the fundamental routing components and functionality that allow you to navigate between different views in your application. It is designed to be platform-agnostic and can be used with various rendering targets, including React DOM for web applications, React Native for mobile applications, and React Native for Web for hybrid applications.
React Router DOM:
React Router DOM is a specific implementation of React Router designed for web applications using React DOM. It builds on top of the React Router core package and provides additional features specific to web routing, such as handling browser history and rendering components for different routes. React Router DOM is widely used in web development with React and is the most commonly used package within the React Router ecosystem.
Conclusion:
React Router is an essential tool in the React ecosystem, providing a comprehensive solution for handling navigation and routing in your applications. By leveraging its features and adopting best practices, you can create user-friendly and efficient applications that allow seamless transitions between different views and pages.
Subscribe to my newsletter
Read articles from Darshana Mallick directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Darshana Mallick
Darshana Mallick
I am a Web Developer and SEO Specialist.