DevOps(Day-21) : Docker Interview Questions
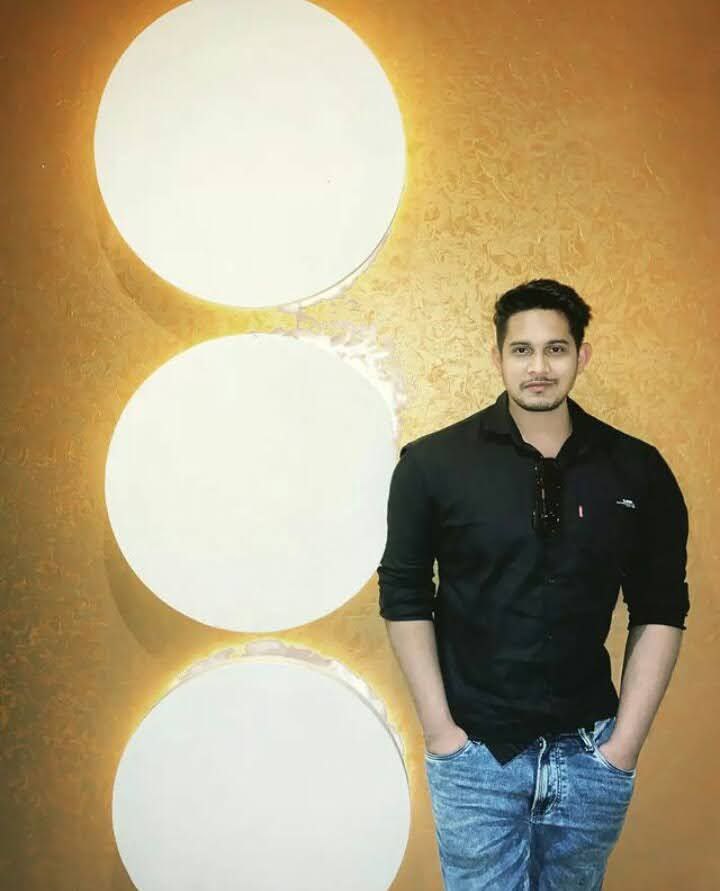
What is the Difference between an Image, Container and Engine?
Image: Docker image is a read-only package containing necessary dependencies, and commands that can be executed to run an application. It is the prerequisite for creating a container.
Container: It is the ability to package the application that has its own network, mounts and OS that can run anywhere, anytime and as many times as you want in any system.
Engine: It is an open-source containerization for building and containerization of your application. It enables you to separate your applications from infrastructure which helps in faster delivery of the software.
What is the Difference between the Docker command COPY vs ADD?
These both commands use to add a specific file from one location to another.
COPY: This command copies the source code of the application from its original location to the path specified.
ADD: It has the same functionality as COPY command but supports file compression and URL sources.
The more secure way is COPY command. Therefore it is widely used.
What is the Difference between the Docker command CMD vs RUN?
RUN: RUN executes when we build the image. That means the command passed to run executes on top of the current image in a new layer.
CMD: With the cmd instruction, we can specify a default command that executes when the container is starting.
How Will you reduce the size of the Docker image?
When building a Docker image, you may want to make sure to keep it light. Avoiding large images speed up the build and deployment of containers and hence, it is critical to reduce the image size of images to a minimum.
Here are some basic steps recommended to follow, which will help create smaller and more efficient Docker images.
Use a smaller base image.
Try to minimize the number of layers to install the packages in the Dockerfile.
Utilize the Multi-Stage Builds Feature in Docker.
Beware of Updates and Unnecessary Packages and Dependencies.
Why and when to use Docker?
Why? - Docker is used to containerising an application that can be run in any system with any configuration at any time.
When? - Suppose there are two developers designed a build and one of them want to transfer the new version of applications to their teammate but now it doesnot work. This situation came due to the difference in the dependencies of the their systems. The only way to overcome the situation is by using containers which can be achieved by Docker.
Explain the Docker components and how they interact with each other.
It is divided into three parts:-
Docker Client - Docker client uses commands and REST APIs to communicate with the Docker Daemon (Server). When a client runs any docker command on the docker client terminal, the client terminal sends these docker commands to the Docker daemon. Docker daemon receives these commands from the docker client in the form of command and REST API's request.
Docker Host - Docker Host is used to provide an environment to execute and run applications. It contains the docker daemon, images, containers, networks, and storage.
Docker Registry - Docker Registry manages and stores the Docker images.
There are two types of registries in the Docker -
Pubic Registry - Public Registry is also called as Docker hub.
Private Registry - It is used to share images within the enterprise.
Explain the terminology: Docker Compose, Docker File, Docker Image, Docker Container?
Docker Compose - Docker Compose is a tool that was developed to help define and share multi-container applications. With Compose, we can create a YAML file to define the services and with a single command, can spin everything up or tear it all down.
Docker File - It is a simple text file with a set of commands or instructions. These commands/instructions are executed successively to perform actions on the base image to create a new docker image.
Docker Image - Docker image is created when we run the Docker file. A Docker image is a file used to execute code in a Docker container. Docker images act as a set of instructions to build a Docker container, like a template. Docker images also act as the starting point when using Docker. An image is comparable to a snapshot in virtual machine (VM) environments.
Docker Container - Docker containers are the live, running instances of Docker images. While Docker images are read-only files and executable content. Users can interact with them, and administrators can adjust their settings and conditions using Docker commands.
In what real scenarios have you used Docker?
I have used Docker to containerise an nginx application. I have written a Docker file including the base image and its dependencies. Thus resulting in building a container. This helped to push the image to the remote repository i.e. DockerHub This image now can be pulled anytime from the repo to run on any system and this reduced the time of building the application everytime for the project.
Docker vs Hypervisor?
The software that helps in the creation of Virtual machines where a virtual platform is provided to the operating systems to manage and execute the virtual machines is called Hypervisor which is otherwise called Virtual Machine Monitor or Emulator or Virtualizer. One system can control various virtual machines and this helps to manage the working of virtual machines via the hypervisor.
Docker is a service for virtualization used in OS where software is delivered in containers with software, libraries, and configuration files. Written in the Go language and developed by Solomon Hykes, the applications are created and deployed using containers and are developed as packages inside the same.
What are the advantages and disadvantages of using docker?
Advantages
Docker containers are extremely portable and can operate on any platform that supports Docker.
Docker containers have a smaller footprint than typical virtual machines, allowing for faster deployment times and lower infrastructure costs.
Docker adds layers of security by separating apps and their dependencies into distinct containers. This can aid in the prevention of security breaches.
Docker delivers consistency by guaranteeing that programs execute on consistent environments across various servers and development workstations.
Docker makes it easier for developers to share their work environments and work together on projects, which increases productivity and guarantees that everyone in the team is using the same codebase and dependencies.
Disadvantages
Docker can increase complexity by requiring additional tools and skills for maintaining containers and coordinating containerized applications.
While Docker provides a solution for dependency management, it may also create extra issues for maintaining dependencies across multiple containers and environments.
Docker may cause compatibility difficulties with current programs since they may not be compatible with containerization or may require additional configuration to function in a containerized environment.
What is a Docker namespace?
Docker uses namespaces of various kinds to provide the isolation that containers need to remain portable and refrain from affecting the remainder of the host system. Each aspect of a container runs in a separate namespace and its access is limited to that namespace.
Namespace Types:
Process ID
Mount
IPC (Interprocess communication)
User (currently experimental support for)
Network
What is a Docker registry?
A Docker registry is a storage and distribution system for named Docker images. The same image might have multiple different versions, identified by their tags. A Docker registry is organized into Docker repositories, where a repository holds all the versions of a specific image.
What is an entry point?
In a Dockerfile, we use the entry point instruction to provide executables that will always execute when the container is launched.
How to implement CI/CD in Docker?
Dockers help developers to build their code and test their code in any environment to catch bugs early in the application development life cycle. Dockers help streamline the process, save time on builds, and allow developers to run tests in parallel.
Dockers can integrate with source control management tools like GitHub and Integration tools like Jenkins. Developers submit the code to GitHub and test the code that automatically triggers a build using Jenkins to create an image. This image can be added to the Docker registry to deal with inconsistencies between different environment types.
One method to fit the Docker in the CI process is to have the CI server build the Docker Image after it has built the application. The application goes inside the image, and the image is then pushed to the Docker hub. On another host, either QA/Dev/Production environment, pull the nearly completed build from the Docker Hub and run the Container which will run your application. In the CI server, you could even have your Compile and Testing done as part of the Image build.
Will data on the container be lost when the docker container exits?
The container data will be lost if the container is not tagged to the volume.
Docker volumes are a widely used and useful tool for ensuring data persistence while working in containers. Docker volumes are file systems mounted on Docker containers to preserve data generated by the running container.
The data doesn't persist when that container no longer exists, and it can be difficult to get the data out of the container if another process needs it.
A container's writable layer is tightly coupled to the host machine where the container is running. The data cannot be easily moveable somewhere else.
Writing into a container's writable layer requires a storage driver to manage the filesystem.
Docker has two options for containers to store files in the host machine so that the files are persisted even after the container stops:
Volumes are stored in a part of the host filesystem, which is managed by
Bind mounts may be stored anywhere on the host system.
What is a Docker swarm?
A Docker Swarm is a container orchestration tool running the Docker application. It has been configured to join together in a cluster. The activities of the cluster are controlled by a swarm manager, and machines that have joined the cluster are referred to as nodes.
What are the docker commands for the following:
view running containers
docker ps -a
command to run the container under a specific name
docker run --name 'container-name' 'image-name'
command to export a docker
docker export 'container-name' 'path-to-save-file>.tar'
command to import an already existing docker image
docker import 'path-to-docker-image' 'image-name'
commands to delete a container
docker stop <container-name>
docker rm <container-name>
command to remove all stopped containers, unused networks, build caches, and dangling images?
docker system prune
Thanks for reading my article. Have a nice day.
WRITTEN BY Biswaraj Sahoo --AWS Community Builder | DevOps Engineer | Docker | Linux | Jenkins | AWS | Git | Terraform | Docker | kubernetes
Empowering communities via open source and education. Connect with me over linktree: linktr.ee/biswaraj333
Subscribe to my newsletter
Read articles from Biswaraj Sahoo directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
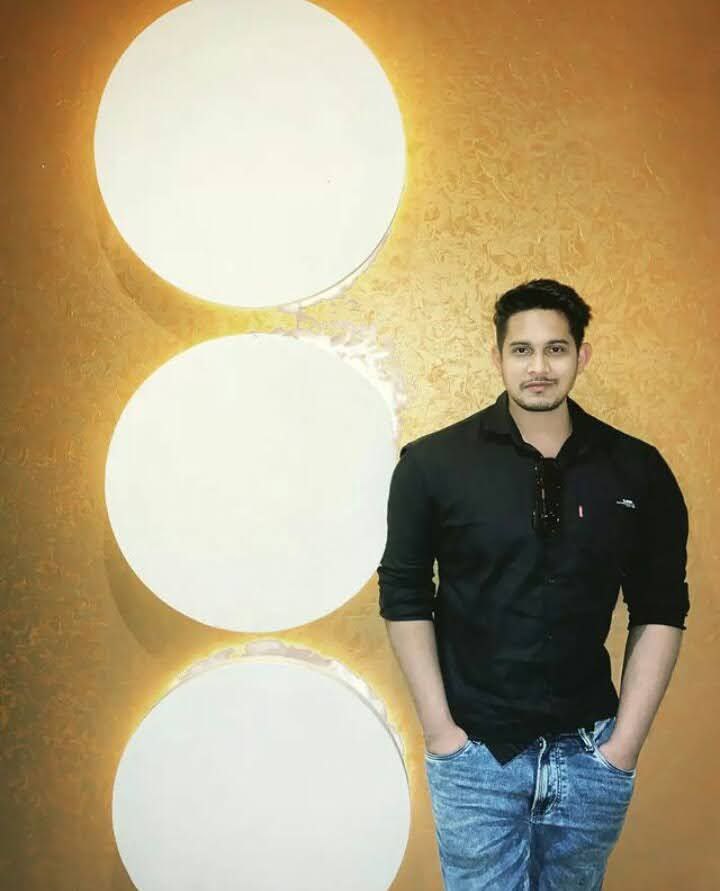
Biswaraj Sahoo
Biswaraj Sahoo
--AWS Community Builder | DevOps Engineer | Docker | Linux | Jenkins | AWS | Git | Terraform | Docker | kubernetes Empowering communities via open source and education.