Control Statements In Csharp
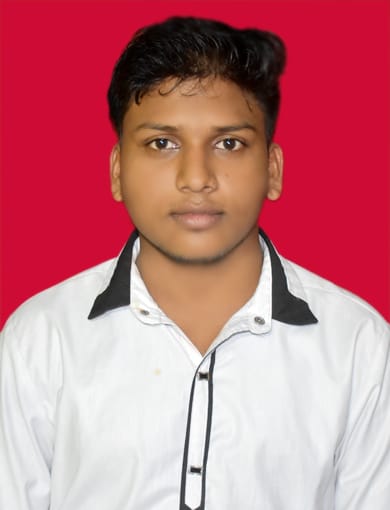
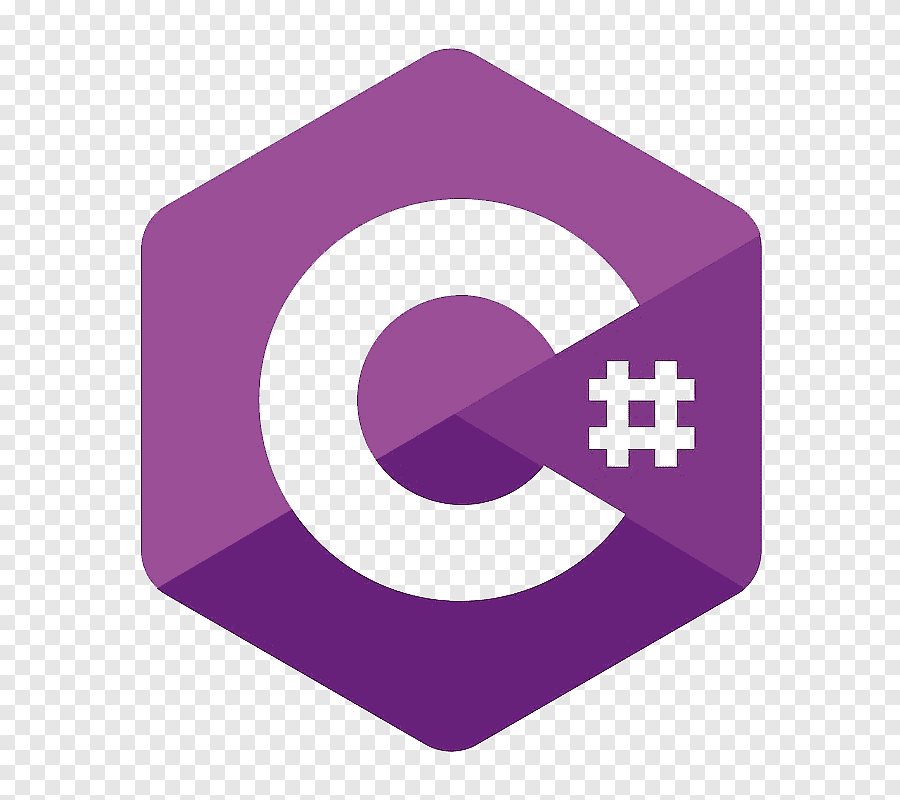
In this article, we will learn about the control statements in Csharp
Control statements are used to control the flow of execution of the program. There are different types of control statements present in C# and they are:-
If-else
switch
while
do-while
for
foreach
break
continue
goto
If-else:-
If-else condition is used when we want to execute a specific block of code, the code within the block will be executed if the condition given is true if the condition given is false then the code within the else block will be executed.
using ControlStatements;
using System;
namespace ControlStatements
{
internal class Program
{
static void Main(string[] args)
{
bool s = true;
if (s)
{
Console.WriteLine("If the condition is true Code within the if block executed.");
}
else
{
Console.WriteLine("If the condition is false Code within the else block will be executed.");
}
Console.ReadLine();
}
}
}
the nested if-else condition can also be used if we have more than one condition to check and execute.
using System;
namespace ControlStatements
{
internal class Program
{
static void Main(string[] args)
{
int s = 3;
if (s == 0)
{
Console.WriteLine("First block");
}
else if(s == 1)
{
Console.WriteLine("Second block");
}
else
{
Console.WriteLine("When the condition is not found.");
}
Console.ReadLine();
}
}
}
Switch:-
Switch case conditions are used when we have to execute a specific block of code out of multiple blocks of code.
using System;
namespace ControlStatements
{
internal class Program
{
static void Main(string[] args)
{
int s = 9;
switch (s)
{
case 0:
Console.WriteLine("This is the first block of code.");
break;
case 1:
Console.WriteLine("This is the second block of code.");
break;
case 2:
Console.WriteLine("This is the third block of code.");
break;
default:
Console.WriteLine("This is the default block of code.");
break;
}
Console.ReadLine();
}
}
}
While:-
While loop is used when we want to execute a certain block of code repeatedly until the condition is satisfied.
using System;
namespace ControlStatements
{
internal class Program
{
static void Main(string[] args)
{
int s = 0;
while(s <= 10)
{
Console.WriteLine(s);
s++;
}
Console.ReadLine();
}
}
}
Do-while:-
the do-while loop is also used to execute the same block of code until the satisfied condition but in a do-while loop, the code within its block will be executed at least once if the condition is false or satisfied.
using System;
namespace ControlStatements
{
internal class Program
{
static void Main(string[] args)
{
int s = 0;
do
{
Console.WriteLine(s);
s++;
} while (s > 10); // This loop will run at least once even if the given condition is false.
Console.ReadLine();
}
}
}
For:-
For loop is used when we know exactly how many times we need to execute the same block of code repeatedly.
using System;
namespace ControlStatements
{
internal class Program
{
static void Main(string[] args)
{
for(int i = 0; i < 4; i++)
{
Console.WriteLine(i);
}
Console.ReadLine();
}
}
}
Foreach:-
Foreach loop is used when we want to execute or print each statement inside an Array or Collection, In the foreach loop two variables are needed to run the loop the first variable with the data type before the in keyword represents the return type of the foreach loop and the variable after the in keyword represents the Array or Collection which will be traversed.
using System;
namespace ControlStatements
{
internal class Program
{
static void Main(string[] args)
{
string[] arr = { "S", "o", "u", "m", "y", "a" };
foreach (string k in arr)
{
Console.WriteLine(k);
}
Console.ReadLine();
}
}
}
Break:-
The break statement is used when we want to get out of any loop or switch case statements.
using System;
namespace ControlStatements
{
internal class Program
{
static void Main(string[] args)
{
for(int i = 0; i <=3 ; i++)
{
Console.WriteLine(i);
if (i == 0)
{
break; // It will get you out of the loop when the i = 0
}
}
Console.ReadLine();
}
}
}
Continue:-
The continue statement is used when we want to jump to the next iteration of the loop immediately.
using System;
namespace ControlStatements
{
internal class Program
{
static void Main(string[] args)
{
for(int i = 0; i <=3 ; i++)
{
if(i == 0)
{
continue;
}
Console.WriteLine(i);
}
Console.ReadLine();
}
}
}
Goto:-
A Goto statement is used when we want to jump directly from a loop or switch case to a specific block of code.
using System;
namespace ControlStatements
{
internal class Program
{
static void Main(string[] args)
{
for(int i = 0; i <=3 ; i++)
{
if(i == 0)
{
goto Show;
}
Console.WriteLine(i);
}
Show:
Console.WriteLine("It is to show the flow of execution of the goto statement.");
Console.ReadLine();
}
}
}
For now, we have learned the control statements in C#, If you have any doubts regarding the article feel free to ask me. This is it for now in the next article we will discuss some other topics till then keep learning and exploring.
Thank you for reading this article.
Subscribe to my newsletter
Read articles from Soumya Ranjan Pratap directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
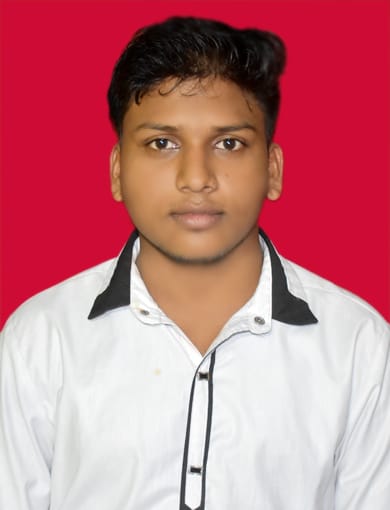
Soumya Ranjan Pratap
Soumya Ranjan Pratap
I am a MCA student learning about full stack web development and I'm also very curious to learn about new things.