Mastering Variadic Functions in C
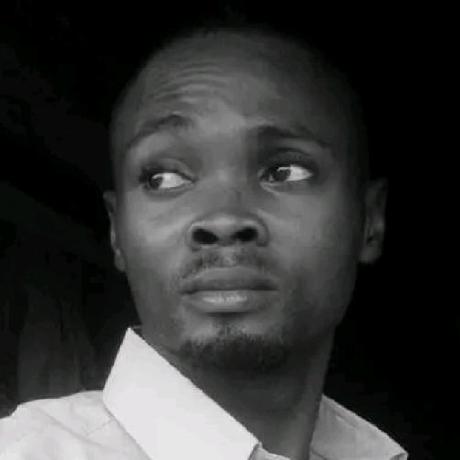
Introduction
In the world of C programming, variadic functions offer a powerful and flexible tool that allows developers to create functions that can accept a variable number of arguments. By leveraging variadic functions, programmers can design versatile and reusable code that can adapt to different scenarios. In this article, we will delve into the intricacies of variadic functions, explore their syntax, and usage, and provide practical examples to demonstrate their capabilities.
By mastering variadic functions, programmers can elevate their C programming skills to new heights, enabling them to tackle complex programming challenges while maintaining code elegance and efficiency
Remember, with great power comes great responsibility, so it's crucial to handle variadic functions with care, ensuring proper argument handling and adherence to best practices for robust and reliable code.
References:
C Programming Language (2nd Edition) by Brian W. Kernighan and Dennis M. Ritchie.
"Variadic functions" - cppreference.com
"C Variadic Functions" - GeeksforGeeks
(Note: This article assumes a basic understanding of the C programming language and its syntax.)
Understanding Variadic Functions:
The concept of variadic functions:
Variadic functions are functions in C that can accept a variable number of arguments. Unlike regular functions, which have a fixed number of arguments defined in their parameter list, variadic functions allow for flexibility in the number and type of arguments that can be passed. This feature makes them extremely useful when dealing with situations where the number of arguments may vary based on specific conditions or requirements.
Benefits and use cases of variadic functions:
Variadic functions offer several benefits and find application in various scenarios:
Flexibility: Variadic functions provide a way to handle a varying number of arguments, allowing developers to create more versatile and adaptable code. This flexibility can be particularly useful when designing functions that need to accommodate different use cases or deal with varying input parameters.
Code reusability: By utilizing variadic functions, programmers can create generic functions that work with different types and numbers of arguments. This promotes code reuse and reduces the need for writing multiple specialized functions for different scenarios.
Logging and debugging: Variadic functions are often used to implement logging systems, where the function can accept different log messages with varying levels of information. This allows for customizable and extensible logging mechanisms.
Mathematical operations: Variadic functions can be employed to implement mathematical operations that can handle different numbers of operands. For example, a variadic sum function can accept any number of integers and return their sum, regardless of the count.
Generic utility functions: Variadic functions are instrumental in creating generic utility functions that can handle different data types and argument counts. This can be beneficial when developing libraries or frameworks that need to work with diverse input.
Limitations and precautions when using variadic functions:
While variadic functions provide flexibility, it is essential to be aware of their limitations and take precautions:
Type safety: Variadic functions can be prone to type-related issues since the compiler cannot perform its usual type-checking on the variable arguments. Developers must exercise caution to ensure proper type handling and avoid runtime errors.
Argument count determination: Since the number of arguments can vary, it is crucial to have a mechanism to determine the count of the arguments passed to a variadic function. Often, an additional argument is needed to specify the count explicitly.
Limited type information: The variadic nature of the function makes it difficult to determine the types of the arguments passed without additional mechanisms, such as format specifiers in functions like
printf
.Portable va_list usage: The usage of the
<stdarg.h>
header and related macros involve some platform-specific considerations. Developers should ensure portability across different compilers and systems.
Understanding these concepts and considerations will enable developers to effectively harness the power of variadic functions while mitigating potential pitfalls and ensuring reliable and efficient code.
Working with Variadic Functions:
Syntax and declaration of variadic functions:
Variadic functions in C are declared using the ellipsis (...
) notation in the function parameter list. The general syntax is as follows:
return_type function_name(type1 arg1, type2 arg2, ...)
{
// Function body
}
Here, return_type
represents the type of value the function returns, and arg1
, arg2
, etc., are the explicitly declared arguments before the ellipsis.
The <stdarg.h>
header and related macros:
To work with variadic functions, the <stdarg.h>
header must be included. This header provides macros and functions to access the variable arguments. The essential macros used with variadic functions are:
va_list
: It is a type representing the variable argument list. Typically, ava_list
object is declared within the variadic function using the identifierap
(for example:va_list ap;
).va_start
: This macro initializes theva_list
object. It takes two arguments: theva_list
object (often namedap
) and the last named parameter before the ellipsis (...
). It prepares theva_list
object for argument retrieval.va_arg
: This macro retrieves the next argument from theva_list
object. It takes two arguments: theva_list
object and the type of the argument to be retrieved. The macro returns the argument of the specified type and advances theva_list
object to the next argument.va_end
: This macro ends the processing of the variable argument list. It takes one argument: theva_list
object. It performs the necessary cleanup associated with theva_list
object.
Example usage of these macros is as follows:
#include <stdarg.h>
int sum(int count, ...)
{
int total = 0;
va_list ap;
va_start(ap, count);
for (int i = 0; i < count; i++) {
int num = va_arg(ap, int);
total += num;
}
va_end(ap);
return total;
}
In this example, the function sum
takes an integer count
as the first argument, followed by any number of integer arguments. Within the function, we initialize the va_list
object ap
using va_start
, retrieve each integer argument using va_arg
, and perform the necessary cleanup with va_end
. The total sum is returned as the result.
It is important to note that the order, types, and number of arguments must be known by the programmer to correctly handle variadic functions. Incorrect usage can lead to undefined behavior and runtime errors.
Understanding the syntax and utilizing the <stdarg.h>
Macros allow programmers to effectively implement variadic functions, making their code more flexible and adaptable to different argument requirements.
Implementing printf
Let us implement our printf function. This is only for educational purposes. We name it my_printf(). It has one string argument (str) and the rest are variable arguments. Variable arguments are managed by macros like va_start, va_arg and va_end. A temporary buffer (buff) is there to construct the output buffer. A while loop is needed to scan each character in the input string. Now we iterate character by character in the loop and copy each character to the output string. Same time we check for "%". "%" is not copied to the output string. Once we find it, we check the next character.
This is the formatting character. The formatting character says how to format the argument to a visible output string. Printf supports varieties of formatting. C is for character, d for decimal integer, f for floating point, x for hexadecimal and s for strings. We match the formatting and pick the argument variable using va_arg().
The argument variable is then converted to a string format and appended to the output string. the character can be copied as it is and Itoa() function is used for integer-to-string conversion.
The C itoa() function has been used to convert the argument integer to string. Integer to String conversion is a process of taking each digit and converting those to ASCII format.
This process of copying characters and conversion of arguments repeats until the string is terminated to the last NULL character. For simplicity, we have implemented only c, d, and x formatting cases. Now at the end, we have the output string ready. This is now passed to fwrite() to stdout. Thus the output string prints in the actual console. Printf then returns the number of characters which is printed in the console and exits the function.
/*This is a simple implementation of the printf function*/
/*for educational purposes only*/
#include <stdio.h>
#include <stdlib.h>
#include <stdarg.h>
#include <string.h>
char *_strrev (char *str)
{
int i;
int len = 0;
char c;
if (!str)
return NULL;
while(str[len] != '\0'){
len++;
}
for(i = 0; i < (len/2); i++)
{
c = str[i];
str [i] = str[len - i - 1];
str[len - i - 1] = c;
}
return str;
}
char * _itoa(int i, char *strout, int base)
{
char *str = strout;
int digit, sign = 0;
if (i < 0) {
sign = 1;
i *= -1;
}
while(i) {
digit = i % base;
*str = (digit > 9) ? ('A' + digit - 10) : '0' + digit;
i = i / base;
str ++;
}
if(sign) {
*str++ = '-';
}
*str = '\0';
_strrev(strout);
return strout;
}
int myprintf (char * str, ...)
{
va_list vl;
int i = 0, j=0;
char buff[100]={0}, tmp[20];
char * str_arg;
va_start( vl, str );
while (str && str[i])
{
if(str[i] == '%'){
i++;
switch (str[i]) {
/* Convert char */
case 'c': {
buff[j] = (char)va_arg( vl, int );
j++;
break;
}
/* Convert decimal */
case 'd': {
_itoa(va_arg( vl, int ), tmp, 10);
strcpy(&buff[j], tmp);
j += strlen(tmp);
break;
}
/* Convert hex */
case 'x': {
_itoa(va_arg( vl, int ), tmp, 16);
strcpy(&buff[j], tmp);
j += strlen(tmp);
break;
}
/* Convert octal */
case 'o': {
_itoa(va_arg( vl, int ), tmp, 8);
strcpy(&buff[j], tmp);
j += strlen(tmp);
break;
}
/* copy string */
case 's': {
str_arg = va_arg( vl, char* );
strcpy(&buff[j], str_arg);
j += strlen(str_arg);
break;
}
}
} else {
buff[j] =str[i];
j++;
}
i++;
}
fwrite(buff, j, 1, stdout);
va_end(vl);
return j;
}
int main (int argc, char *argv[])
{
int ret;
ret = my_printf("%c %d %o %x %s\n", 'A', 10, 100, 1000, "Hello from printf!");
printf("printf returns %d bytes\n", ret);
return 0;
}
Output
A 10 144 3E8 Hello from printf!
printf return 32
By utilizing variadic functions and macros, you can create a versatile printf function that handles different data types and a variable number of arguments. The macro-based approach enhances type safety, ensuring that the arguments match the format specifiers in the format string.
Conclusion:
Variadic functions in C provide a powerful mechanism for creating functions that can handle a variable number of arguments. By leveraging the <stdarg.h>
header and related macros, developers can build flexible and adaptable functions that cater to different argument requirements. From logging systems to mathematical operations and generic utility functions, variadic functions open up possibilities for writing reusable code.
Subscribe to my newsletter
Read articles from Davies Aniefiok directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
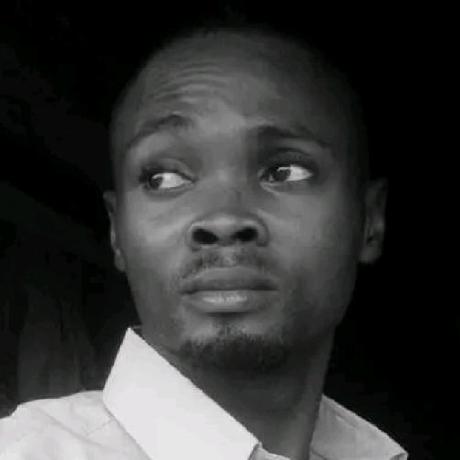