Introduction to Data Structures and Algorithms: Understanding Essential Concepts and Performance Analysis

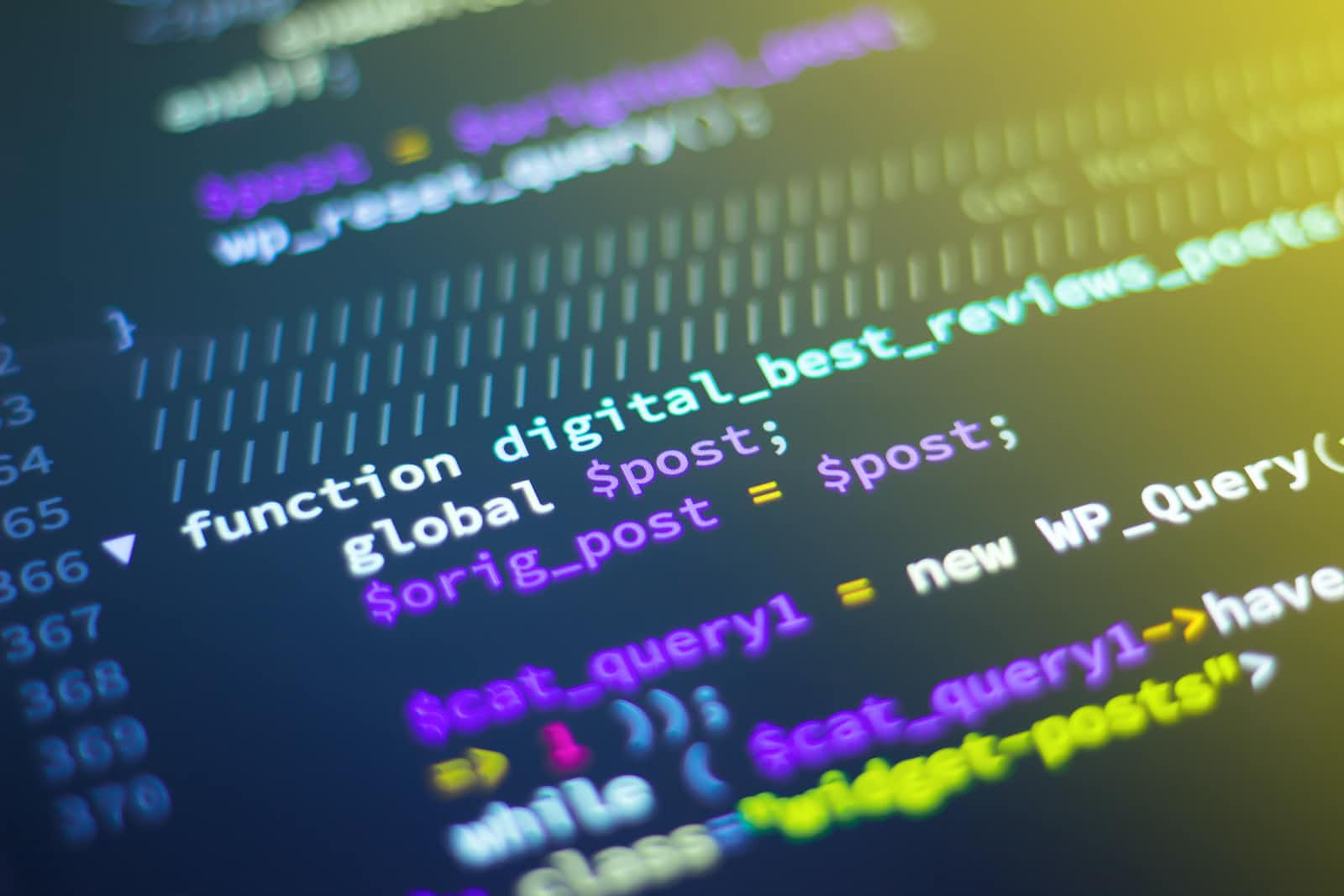
Introduction: Data structures and algorithms are the backbone of efficient and effective software development. They provide the foundation for organizing and manipulating data, enabling us to solve complex problems. In this article, we will explore essential data structures such as arrays, linked lists, and trees, along with fundamental algorithms like sorting and searching. Additionally, we will delve into the concept of time and space complexities to evaluate the efficiency of these data structures and algorithms.
Data Structures: 1.1 Arrays:
Definition and characteristics
Accessing elements
Insertion and deletion operations
Common use cases
Time and space complexities
1.2 Linked Lists:
Singly-linked lists vs. doubly-linked lists
Insertion and deletion operations
Traversing and accessing elements
Advantages and disadvantages
Time and space complexities
1.3 Trees:
Binary trees and their properties
Traversing techniques (pre-order, in-order, post-order)
Binary search trees and their operations
Balanced trees (e.g., AVL, Red-Black)
Time and space complexities
Algorithms: 2.1 Sorting Algorithms:
Bubble Sort
Selection Sort
Insertion Sort
Merge Sort
Quick Sort
Comparison of sorting algorithms based on time complexity
2.2 Searching Algorithms:
Linear Search
Binary Search
Hashing and Hash Tables
Comparison of searching algorithms based on time complexity
Time and Space Complexities:
Big O notation and its significance
Best-case, worst-case, and average-case complexities
Analyzing time and space complexities for various algorithms
Comparing different algorithms based on efficiency
Conclusion: Understanding data structures and algorithms is crucial for building efficient and optimized software solutions. By mastering the concepts of arrays, linked lists, trees, sorting, and searching algorithms, developers gain the ability to tackle complex problems and design performant applications. Moreover, comprehending time and space complexities enables the evaluation and selection of the most appropriate algorithms for specific tasks. As you continue your programming journey, delve deeper into these topics, and explore more advanced data structures and algorithms to enhance your problem-solving skills and become a proficient developer.
Remember, proficiency in data structures and algorithms is a continuous learning process. Regular practice and hands-on implementation of these concepts will sharpen your skills and contribute to your growth as a software engineer.
Subscribe to my newsletter
Read articles from Samraj directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Samraj
Samraj
I am Samraj, a motivated fresher with a strong background in Computer Science. I excel in Java programming and have a keen interest in open-source projects. With hands-on experience in Java technologies, I write clean and efficient code. I actively contribute to open-source projects, showcasing my commitment to collaboration and community. My passion for learning and expertise in Java make me an asset for organizations seeking innovative solutions in the open-source space.