What is Asset fingerprinting or ( cache busting ) ? And How it Works in ReactJs
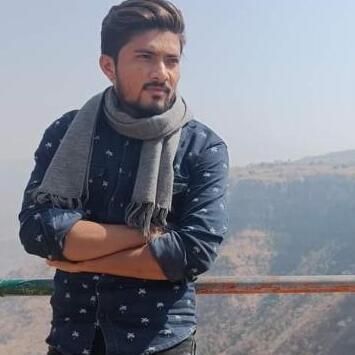
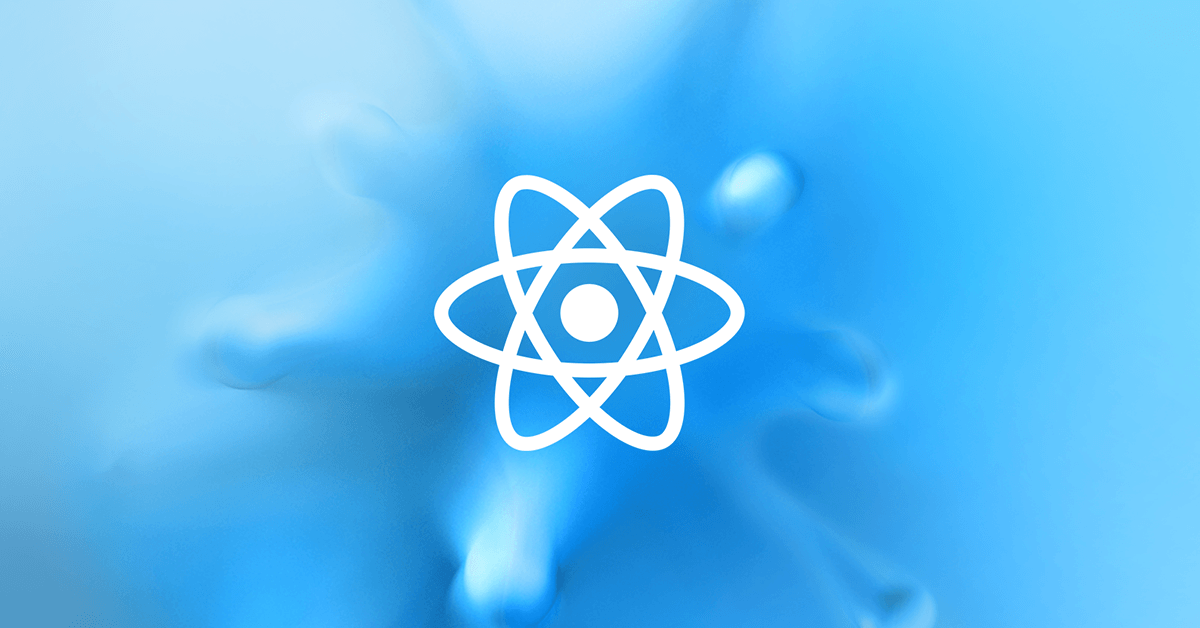
Asset fingerprinting in React (or in web development in general) is a technique used to manage cache and ensure that the latest version of an asset is served to users when changes are made.
It involves appending a unique identifier (often a hash) to the filename of each asset, typically CSS, JavaScript, or image files.
This unique identifier changes whenever the content of the asset changes, which prompts the browser to request the updated version.
Let's dive deeper into asset fingerprinting and how it works in React:
Purpose of Asset Fingerprinting:
Prevent Caching Issues: By changing the filename when the asset content changes, asset fingerprinting ensures that the browser doesn't rely on cached versions of assets that might be outdated.
- Cache Optimization: It allows the browser to cache assets more effectively by treating each version as a separate resource, improving performance and reducing bandwidth usage.
Implementation in React:
Build Tools: Asset fingerprinting is typically handled by build tools like Webpack or Create React App during the production build process.
Unique Identifiers: A unique identifier (e.g., hash) is generated based on the content of each asset file.
Appending Identifiers: The generated identifier is appended to the filename of the asset, creating a new fingerprinted filename.
Process Flow:
Development Environment: During development, you work with the original filenames of the assets.
Production Build: When you create a production build of your React app, the build tool applies asset fingerprinting.
Unique Identifiers: The build tool calculates unique identifiers for each asset based on its content.
Fingerprinted Filenames: The build tool renames the assets, appending the unique identifiers to their filenames.
Updated References: Any references to these assets in your code (e.g., HTML, CSS, or JavaScript files) are also updated with the new fingerprinted filenames.
Cache Management and Updates:
Initial Request: When a user accesses your React app, the browser fetches the assets using the fingerprinted filenames.
Caching: The browser caches the assets based on the unique fingerprinted filenames.
Subsequent Requests: When changes are made to the assets and a new build is deployed, the fingerprinted filenames change.
Cache Invalidation: The browser recognizes the new filenames as separate resources and fetches the updated assets, bypassing the cache.
Consistent Updates: With each subsequent build, the fingerprinted filenames change again, ensuring consistent updates for users.
By employing asset fingerprinting, you can effectively manage caching and ensure that users receive the latest versions of your assets. This technique improves the reliability and consistency of your React app, especially when updates are made to CSS, JavaScript, or image files.
Here are a few examples to illustrate asset fingerprinting in React:
Example CSS Asset:
Original Filename:
styles.css
Unique Identifier (Hash):
abcd1234
Fingerprinted Filename:
styles.abcd1234.css
Example JavaScript Asset:
Original Filename:
app.js
Unique Identifier (Hash):
efgh5678
Fingerprinted Filename:
app.efgh5678.js
Example Image Asset:
Original Filename:
logo.png
Unique Identifier (Hash):
ijkl9012
Fingerprinted Filename:
logo.ijkl9012.png
When these assets undergo changes and a new production build is created, the unique identifiers (hashes) will change, resulting in new fingerprinted filenames. For example, after a modification:
Updated CSS Asset:
Original Filename:
styles.css
Unique Identifier (Hash):
wxyz7890
Fingerprinted Filename:
styles.wxyz7890.css
Updated JavaScript Asset:
Original Filename:
app.js
Unique Identifier (Hash):
mnop3456
Fingerprinted Filename:
app.mnop3456.js
Updated Image Asset:
Original Filename:
logo.png
Unique Identifier (Hash):
qrst5678
Fingerprinted Filename:
logo.qrst5678.png
By changing the filenames with each build, the browser recognizes the assets as new resources and retrieves the updated versions, avoiding any caching issues that might occur if the filenames remained the same.
Subscribe to my newsletter
Read articles from Mayur Patil directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
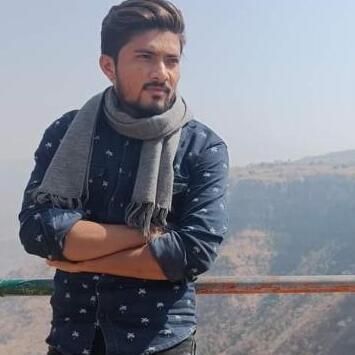
Mayur Patil
Mayur Patil
Frontend Developer || UI Developer || JavaScript || React