LeetCode Challenge #1 - Pascal's Triangle
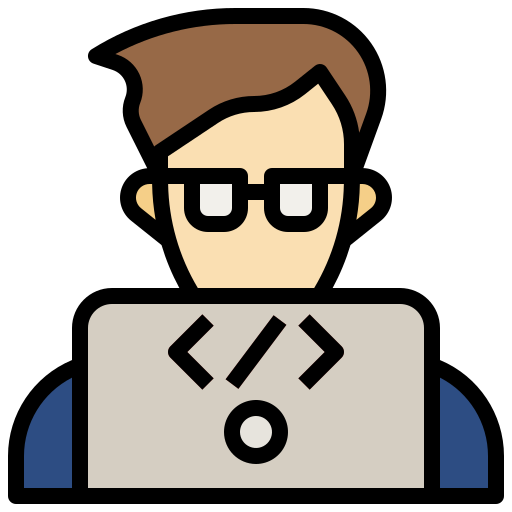
Table of contents
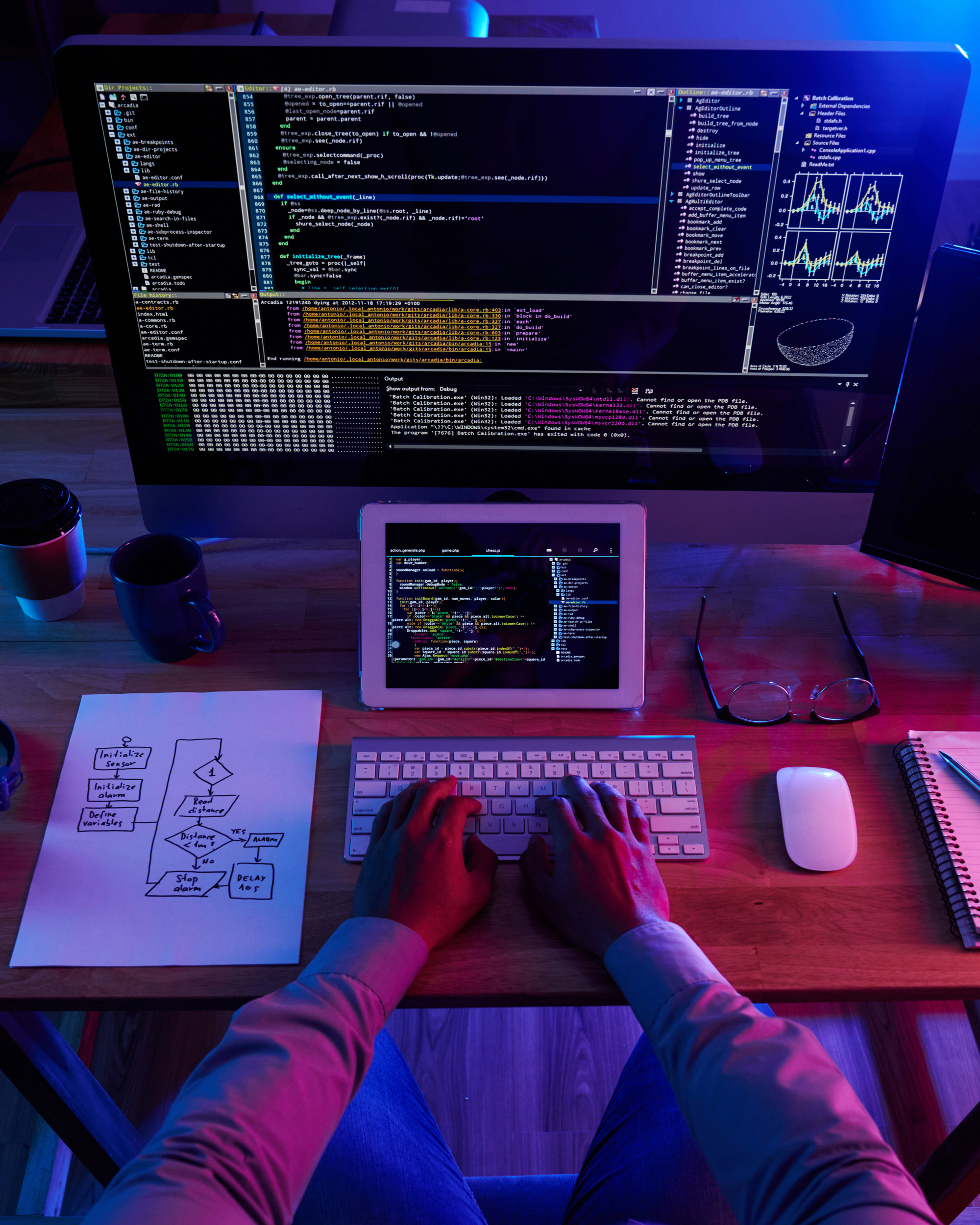
Introduction
In this Leetcode challenge, i'll take you through my approach and analysis in solving the infamous Pascal's triangle problem.
Question
Given an integer numRows
, return the first numRows of Pascal's triangle.
In Pascal's triangle, each number is the sum of the two numbers directly above it as shown:
Example 1:
Input: numRows = 5 Output: [[1],[1,1],[1,2,1],[1,3,3,1],[1,4,6,4,1]]
Example 2:
Input: numRows = 1 Output: [[1]]
Constraints: 1 <= numRows <= 30
Solution
Let's now delve into the steps to solve this challenge. Firstly the return type of the program will be a List of Integer Arraylists based on the input number of rows provided.
- Let's initialize this List of Integer Arraylists as a return variable output.
List<List<Integer>> output=new ArrayList<>();
- Now we'll provide a check for a scenario when the input number of Rows is Zero. Then we'll return the empty arraylist initialized.
if(numRows==0) {
return output;
}
- Now let's add the first element of the Pascal's triangle (1) as shown below.
List<Integer> row=new ArrayList<>();
row.add(1);
output.add(row);
- Next, we'll add a for loop to create the subsequent rows of the triangle and initialize the first element of the row. As we know in Pascal's triangle, except the first element and last element, the elements are calculated by adding the previous row consecutive 2 elements in subsequent order, we'll also initialize a for loop to perform this operation. We'll next add the last element of the row which is 1. Then add the current row to the Output List and then assign the current Row as the Previous row for the next iteration.
List<Integer> prevRow=row;
for(int i=1;i<numRows;i++){
List<Integer> currentRow=new ArrayList<>();
currentRow.add(1);
for(int j=1;j<i;j++){
currentRow.add(prevRow.get(j)+prevRow.get(j-1));
}
currentRow.add(1);
output.add(currentRow);
prevRow=currentRow;
}
- Now we'll return the final output list of rows list as below.
return result;
Time Complexity
The time complexity for this approach will be O(n^2). There are 2 loops which are running based on the number of rows (n) and number of elements (n) in each row.
Conclusion
I hope you have learned a lot from this article and your suggestion/approaches are also highly welcomed as I believe in learning in Public and consistent practice to improve logic building.
Thank you for taking the time to read this blog and will meet next time with another challenge..✌️
Subscribe to my newsletter
Read articles from Akshay Dev S V directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
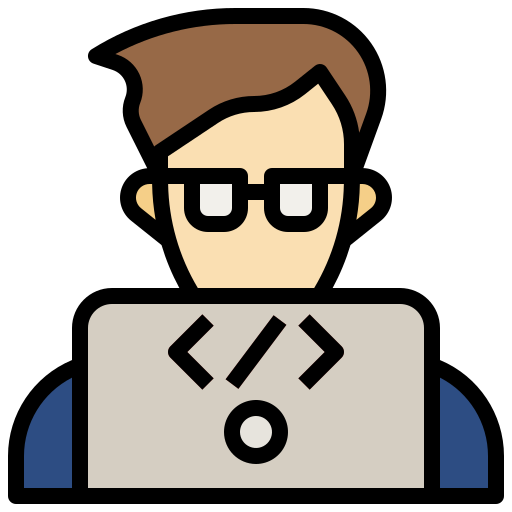
Akshay Dev S V
Akshay Dev S V
I am a Software Engineer with 3+ years of Experience in the Indian IT Sector. Skilled in IBM Maximo EAM, i also have practical knowledge on Java, C++, Python and SQL.