Node.js Testing: Best Practices and Frameworks

Table of contents
- Introduction
- Overview of Node.js Testing
- Importance of Testing in Node.js
- Unit Testing in Node.js
- Integration Testing in Node.js
- End-to-End Testing in Node.js
- Test-Driven Development (TDD) with Node.js
- Choosing the Right Testing Framework
- Popular Testing Frameworks for Node.js
- Conclusion
- FAQs (Frequently Asked Questions)
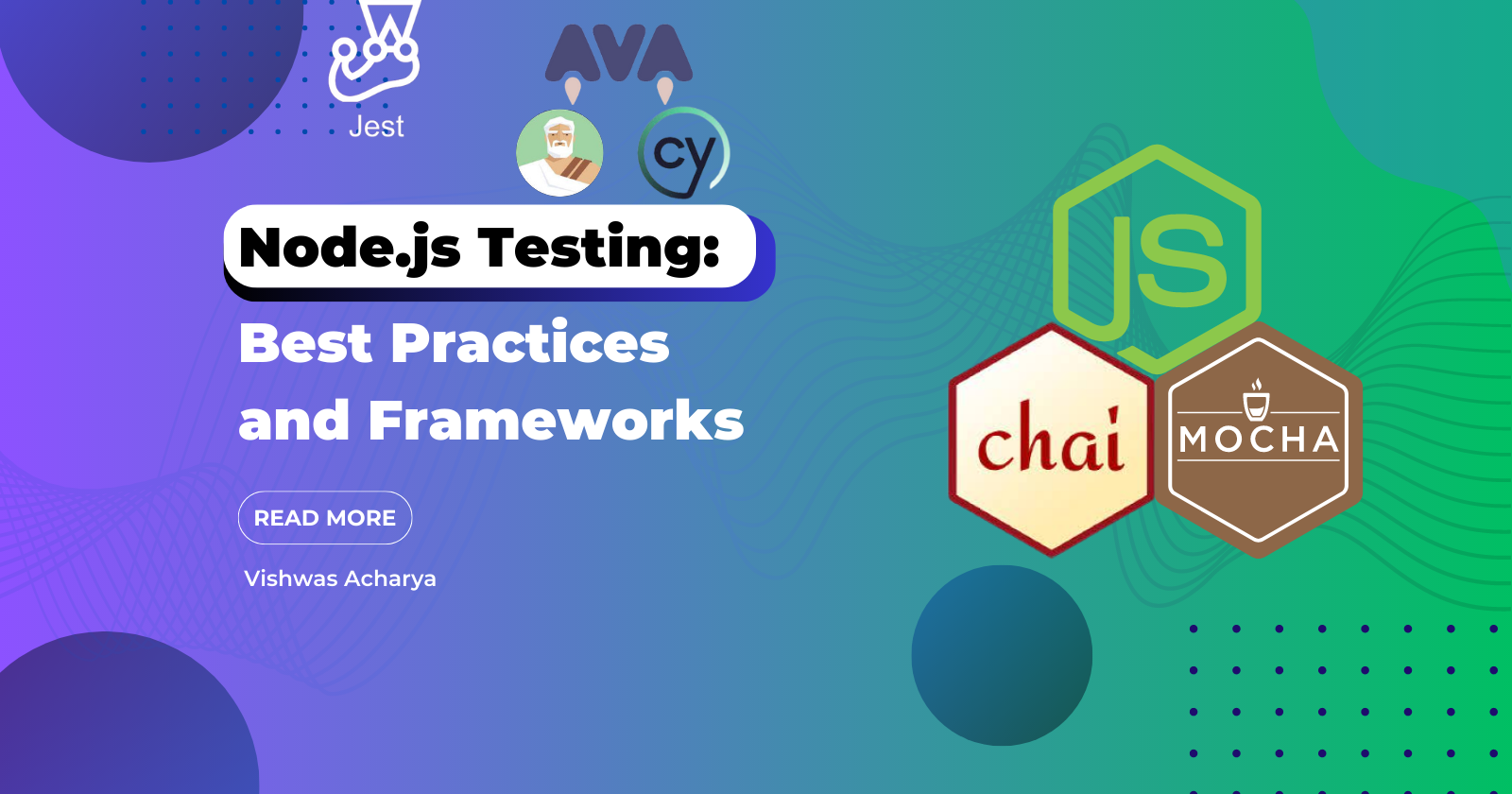
Introduction
Testing is an essential part of software development, ensuring the quality and reliability of the code. Node.js, a popular JavaScript runtime, requires robust testing practices to deliver high-performing and bug-free applications. In this article, we will explore the best practices and frameworks for testing Node.js applications.
Overview of Node.js Testing
Node.js testing involves the process of verifying the behavior and functionality of Node.js applications. It aims to identify bugs, ensure code correctness, and maintain the desired quality standards. Testing can be categorized into unit testing, integration testing, and end-to-end testing.
Importance of Testing in Node.js
Testing plays a crucial role in Node.js development for several reasons. Firstly, it helps in identifying and fixing issues early in the development cycle, reducing the chances of bugs reaching the production environment. Additionally, testing promotes code maintainability, as it allows developers to make changes confidently without breaking existing functionality. Moreover, it enhances code modularity and reusability, as well as improves the overall stability and performance of the application.
Unit Testing in Node.js
Unit testing focuses on testing individual components or units of code in isolation. It ensures that each unit performs as expected and meets the desired functionality. Node.js provides various frameworks and tools for unit testing, making it easier to write and execute tests.
Integration Testing in Node.js
Integration testing involves testing the interaction between different components or modules within a Node.js application. It helps in identifying issues that may arise due to the integration of multiple units. Integration tests ensure that the various components work together seamlessly and produce the expected results.
End-to-End Testing in Node.js
End-to-end testing verifies the entire application flow, simulating real-world scenarios. It tests the interaction between different components, including external dependencies such as databases and APIs. End-to-end tests validate the application's behavior from the user's perspective, ensuring that all functionalities work correctly.
Test-Driven Development (TDD) with Node.js
Test-Driven Development (TDD) is a development approach where tests are written before the actual code. It follows a red-green-refactor cycle, where developers first write a failing test, then implement the code to make the test pass, and finally refactor the code for better design and readability. TDD promotes a test-first mindset and helps in creating more reliable and maintainable code.
Choosing the Right Testing Framework
When it comes to selecting a testing framework for Node.js, several factors need to be considered. These include ease of use, community support, integration with other tools, and the ability to handle different types of tests. Choosing the right framework ensures efficient testing and smooth integration with the development workflow.
Popular Testing Frameworks for Node.js
Mocha
Mocha is a widely used testing framework for Node.js applications. It offers a flexible and feature-rich environment for writing tests. Mocha supports various testing styles, including BDD (Behavior-Driven Development) and TDD. It provides extensive assertion libraries and integrates well with other tools like Chai and Sinon.
Here's an example code snippet that demonstrates how to write tests using the Mocha testing framework in Node.js:
// Import the necessary modules and dependencies
const assert = require('assert');
// Describe a test suite using Mocha's "describe" function
describe('Math operations', () => {
// Define a test case using Mocha's "it" function
it('should add two numbers correctly', () => {
const result = 1 + 2;
assert.strictEqual(result, 3); // Assert the expected result
});
it('should multiply two numbers correctly', () => {
const result = 3 * 4;
assert.strictEqual(result, 12);
});
it('should handle division by zero', () => {
const divideByZero = () => {
const result = 10 / 0;
};
assert.throws(divideByZero, Error); // Assert that an error is thrown
});
});
In the above code, we import the assert
module to perform assertions and verify the expected outcomes of our tests. We then define a test suite using the describe
function, which groups related tests together. Inside the test suite, we define individual test cases using the it
function. Each test case verifies a specific behavior or functionality. In this example, we test addition, multiplication, and division by zero.
To run the Mocha tests, you need to have Mocha installed globally on your system. You can install it by running npm install -g mocha
. After installation, navigate to the directory containing your test file and execute the command mocha
. Mocha will automatically discover and execute the tests, displaying the results in the console.
Jest
Jest is a popular JavaScript testing framework maintained by Facebook. It is known for its simplicity and powerful features. Jest offers built-in code coverage, mocking capabilities, and parallel test execution. It is easy to set up and has excellent support for testing React applications.
Here's an example code snippet that demonstrates how to write tests using the Jest testing framework in Node.js:
// Import the necessary modules and dependencies
const { sum, subtract } = require('./math');
// Describe a test suite using Jest's "describe" function
describe('Math operations', () => {
// Define a test case using Jest's "test" function
test('should add two numbers correctly', () => {
const result = sum(1, 2);
expect(result).toBe(3); // Expect the result to be 3
});
test('should subtract two numbers correctly', () => {
const result = subtract(5, 3);
expect(result).toBe(2);
});
});
In the above code, we assume that there is a separate file called math.js
which exports the sum
and subtract
functions. The describe
function is used to define a test suite, and inside it, we define individual test cases using the test
function provided by Jest. Each test case verifies a specific behavior or functionality. In this example, we test addition and subtraction.
To run the Jest tests, you need to have Jest installed as a dev dependency in your project. You can install it by running npm install --save-dev jest
. After installation, you can execute the command npx jest
in the terminal from the root of your project directory. Jest will automatically discover and execute the tests, displaying the results in the console.
Ava
Ava is a minimalistic and highly performant testing framework for Node.js. It focuses on parallel test execution, providing faster feedback during development. Ava's test syntax is concise and easy to read, making it a great choice for developers who prefer simplicity and speed.
Here's an example code snippet that demonstrates how to write tests using the Ava testing framework in Node.js:
// Import the necessary modules and dependencies
const test = require('ava');
const { multiply, divide } = require('./math');
// Define a test case using Ava's "test" function
test('should multiply two numbers correctly', (t) => {
const result = multiply(3, 4);
t.is(result, 12); // Assert the result using Ava's "t.is" assertion
});
test('should handle division by zero', (t) => {
const divideByZero = () => {
const result = divide(10, 0);
};
t.throws(divideByZero, { instanceOf: Error }); // Assert that an error is thrown
});
In the above code, we assume that there is a separate file called math.js
which exports the multiply
and divide
functions. We import the test
function from the Ava module to define a test case. Inside each test case, we perform the necessary operations and use Ava's assertion methods to verify the expected outcomes.
To run the Ava tests, you need to have Ava installed as a dev dependency in your project. You can install it by running npm install --save-dev ava
. After installation, you can execute the command npx ava
in the terminal from the root of your project directory. Ava will automatically discover and execute the tests, displaying the results in the console.
Chai
Chai is an assertion library that can be used with various testing frameworks, including Mocha and Jasmine. It provides a rich set of assertion styles, allowing developers to write expressive and readable tests. Chai supports both BDD and TDD styles and offers plugins for extending its functionality.
Here's an example code snippet that demonstrates how to write tests using the Chai assertion library in Node.js:
// Import the necessary modules and dependencies
const chai = require('chai');
const { add, subtract } = require('./math');
// Use the Chai assertion style
const { expect } = chai;
// Describe a test suite
describe('Math operations', () => {
// Define a test case
it('should add two numbers correctly', () => {
const result = add(1, 2);
expect(result).to.equal(3); // Expect the result to be equal to 3
});
it('should subtract two numbers correctly', () => {
const result = subtract(5, 3);
expect(result).to.equal(2);
});
});
In the above code, we assume that there is a separate file called math.js
which exports the add
and subtract
functions. We import the chai
module and specifically use the expect
assertion style provided by Chai. Inside the test cases, we perform the necessary operations and use the expect
method to assert the expected outcomes.
To run the Chai tests, you don't need any specific test runner. You can simply execute the test file using a Node.js runner or the mocha
command. For example, if you have installed Mocha globally, you can run the command mocha path/to/test-file.js
in the terminal to execute the tests.
Sinon
Sinon is a powerful mocking and stubbing library often used in conjunction with testing frameworks like Mocha and Jasmine. It enables developers to create test doubles, such as spies, stubs, and mocks, facilitating better control and verification of code behavior during tests.
Here's an example code snippet that demonstrates how to write tests using the Sinon library for mocking and stubbing in Node.js:
// Import the necessary modules and dependencies
const sinon = require('sinon');
const { fetchData, processData } = require('./data');
// Describe a test suite
describe('Data processing', () => {
// Define a test case
it('should process fetched data correctly', () => {
// Create a fake data object
const fakeData = { id: 1, name: 'John Doe' };
// Create a spy for the fetchData function
const fetchDataSpy = sinon.spy(fetchData);
// Create a stub for the fetchData function
const fetchDataStub = sinon.stub().returns(fakeData);
// Replace the original fetchData function with the stub
sinon.replace(data, 'fetchData', fetchDataStub);
// Call the function that processes the data
const result = processData();
// Expect the result to be the processed data
expect(result).to.deep.equal({ id: 1, name: 'JOHN DOE' });
// Verify that the fetchData function was called once
expect(fetchDataSpy.calledOnce).to.be.true;
// Restore the original fetchData function
sinon.restore();
});
});
In the above code, we assume that there are two separate files: data.js
, which contains the fetchData
and processData
functions, and the test file where the tests are written. We import the sinon
module to create spies and stubs. Inside the test case, we create a spy for the fetchData
function to track its invocation and a stub to replace its behavior and return fake data. We then call the processData
function, asserting the expected result. Finally, we verify that the fetchData
function was called once and restore the original function using sinon.restore()
.
To run the tests using Sinon, you can use a test runner like Mocha or Jest. You need to have Sinon and the test runner installed as dependencies in your project. After installing the necessary dependencies, you can execute the test file using the test runner. For example, with Mocha, you can use the mocha
command followed by the path to the test file.
Cypress
Cypress is an end-to-end testing framework specifically designed for modern web applications. It provides an interactive test runner and comprehensive tooling for debugging and time-traveling during test execution. Cypress offers a user-friendly API and is well-suited for testing Node.js applications with frontend components.
Here's an example code snippet that demonstrates how to write Cypress tests for a Node.js web application:
// In your Cypress test file (e.g., cypress/integration/node-app.spec.js)
// Define a test suite
describe('Node.js Web App', () => {
// Define a test case
it('should display the homepage correctly', () => {
// Visit the homepage of your Node.js web application
cy.visit('http://localhost:3000');
// Assert the expected behavior of the homepage
cy.contains('Welcome to My Node.js Web App');
cy.get('button').should('have.length', 3);
});
// Define another test case
it('should navigate to the about page', () => {
// Visit the homepage
cy.visit('http://localhost:3000');
// Click on the link to the about page
cy.contains('About').click();
// Assert the expected behavior of the about page
cy.url().should('include', '/about');
cy.contains('About Page');
});
});
In the above code, we assume that you have a Node.js web application running on localhost
at port 3000
. You can customize the URLs and assertions based on your application's specific routes and content. Cypress provides a fluent and expressive API to interact with and assert against elements on your web application.
To run the Cypress tests, you need to have Cypress installed as a dev dependency in your project. You can install it by running npm install --save-dev cypress
. After installation, you can execute the command npx cypress open
in the terminal from the root of your project directory. Cypress will open its Test Runner, allowing you to select and run your tests interactively.
Conclusion
Testing is a critical aspect of Node.js development, ensuring the reliability and quality of applications. By following best practices and leveraging appropriate testing frameworks like Mocha, Jest, Ava, Chai, Sinon, and Cypress, developers can streamline the testing process and deliver robust and bug-free Node.js applications.
FAQs (Frequently Asked Questions)
Q: How important is testing in Node.js development?
A: Testing is crucial in Node.js development as it helps identify and fix issues early, ensures code correctness, and enhances application stability.
Q: What is the difference between unit testing and integration testing in Node.js?
A: Unit testing focuses on testing individual components in isolation, while integration testing verifies the interaction between multiple components.
Q: Which testing framework is best for Node.js?
A: The choice of testing framework depends on specific requirements. Popular options include Mocha, Jest, Ava, Chai, Sinon, and Cypress.
Q: What is Test-Driven Development (TDD) in Node.js?
A: Test-Driven Development (TDD) is an approach where tests are written before writing the actual code, promoting a test-first mindset.
Q: Can Cypress be used for testing Node.js applications with frontend components?
A: Yes, Cypress is a suitable testing framework for Node.js applications with frontend components, providing an interactive test runner and comprehensive tooling.
By Vishwas Acharya 😉
Checkout my other content as well:
YouTube:
Podcast:
Book Recommendations:
Subscribe to my newsletter
Read articles from Vishwas Acharya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Vishwas Acharya
Vishwas Acharya
Embark on a journey to turn dreams into digital reality with me, your trusted Full Stack Developer extraordinaire. With a passion for crafting innovative solutions, I specialize in transforming concepts into tangible, high-performing products that leave a lasting impact. Armed with a formidable arsenal of skills including JavaScript, React.js, Node.js, and more, I'm adept at breathing life into your visions. Whether it's designing sleek websites for businesses or engineering cutting-edge tech products, I bring a blend of creativity and technical prowess to every project. I thrive on overseeing every facet of development, ensuring excellence from inception to execution. My commitment to meticulous attention to detail leaves no room for mediocrity, guaranteeing scalable, performant, and intuitive outcomes every time. Let's collaborate and unleash the power of technology to create something truly extraordinary. Your dream, my expertise—let's make magic happen! Connect with me on LinkedIn/Twitter or explore my work on GitHub.