How to use elastic search in nodejs: the ultimate guide

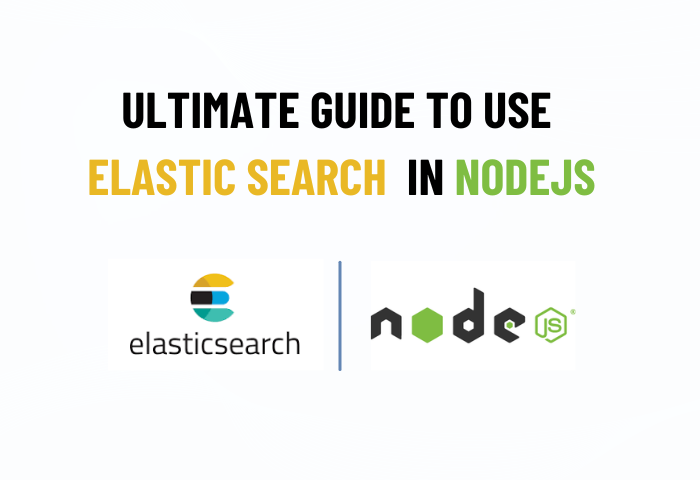
Nodejs is one of the most popular run-time environments to create backends of web applications. it has the fastest performance when it comes to handling user requests. But that's not it, you can take your website to the next level by integrating elastic search in your nodejs application.
Introduction to elastic search
ElasticSearch is a scalable search and analytics engine that can store, search, and analyze large volumes of data. As an open-source solution, it offers a RESTful API for querying data, making it a great fit for Node.js applications. ElasticSearch supports real-time search and analytics and is versatile enough to be used for various use cases, including e-commerce search, log analysis, and business intelligence.
it is advisable to hire node js experts if you are looking to harness the power of elastic search to scale up your web application built with nodejs.
Setting up ElasticSearch
To set up ElasticSearch, the first step is to download and install the software.
ElasticSearch can be downloaded from the official website, and installation instructions are available for different operating systems. Once ElasticSearch is installed, the next step is to set up an ElasticSearch cluster, which is a group of nodes that work together to provide search and analytics capabilities. To index data into ElasticSearch, you need to create an index and define its mapping, which specifies the data types and fields that will be indexed. Once the index is created, you can start indexing data using the ElasticSearch API or a client library. With the right setup and configuration, ElasticSearch can provide fast and reliable search and analytics capabilities for your Node.js application.
Connecting to ElasticSearch from Node.js
To connect to an ElasticSearch cluster from a Node.js application, you need to install the ElasticSearch client for Node.js. The official ElasticSearch client for Node.js is called "elasticsearch.js", and it can be installed using npm. Once installed, you can use the client to connect to an ElasticSearch cluster by specifying the host and port of the cluster. Here's an example code snippet that shows how to connect to an ElasticSearch cluster using the elasticsearch.js client:
const { Client } = require('@elastic/elasticsearch');
// create a new client instance
const client = new Client({ node: 'http://localhost:9200' });
// ping the cluster to check if it's running
client.ping((err) => {
if (err) {
console.error('Elasticsearch cluster is down!');
} else {
console.log('Connected to Elasticsearch cluster');
}
});
In this example, we create a new client instance by passing the URL of the ElasticSearch cluster to the Client constructor. We then call the ping method to check if the cluster is running. If there is an error, we log a message indicating that the cluster is down. If there is no error, we log a message indicating that we are connected to the cluster.
Indexing and querying data
The indexing and querying data is a complex process. and it is often advised to hire nodejs developers with good experience who have worked on plenty of projects.
In Node.js, the elasticsearch.js client's index
method can be used to index data in ElasticSearch. This method takes an index name, a document ID, and the document data, and returns a promise that resolves to the ElasticSearch response. Here's an example code snippet:elasticsearch.js client:
javascript
Copy
const { Client } = require('@elastic/elasticsearch');
// create a new client instance
const client = new Client({ node: 'http://localhost:9200' });
// index a document
client.index({
index: 'myindex',
id: '1',
body: {
title: 'My Document Title',
content: 'Lorem ipsum dolor sit amet, consectetur adipiscing elit.',
tags: ['tag1', 'tag2']
}
}).then((response) => {
console.log(response);
}).catch((error) => {
console.error(error);
});
To query data using the ElasticSearch query DSL, you can use the search method of the client. The search method takes a search query object, which can include criteria such as search terms, filters, aggregations, and sorting options. Here's an example code snippet that shows how to search for documents using the elasticsearch.js client:
const { Client } = require('@elastic/elasticsearch');
// create a new client instance
const client = new Client({ node: 'http://localhost:9200' });
// search for documents
client.search({
index: 'myindex',
body: {
query: {
match: {
content: 'consectetur adipiscing'
}
}
}
}).then((response) => {
console.log(response.hits.hits);
}).catch((error) => {
console.error(error);
});
In this example, we search for documents that contain the phrase "consectetur adipiscing" in the content field of the myindex index. The results are returned as an array of hits, which contain information about the matched documents.
Advanced features
Aggregations
ElasticSearch enables data analysis and insights extraction from search results in Node.js apps with a powerful aggregation framework. Aggregations perform statistical calculations, grouping, filtering, and more on data, and can be implemented with the elasticsearch.js client.
Search filters and sorting
ElasticSearch offers search filters and sorting options to refine search results in Node.js apps. Filters narrow down results based on specific criteria, like date ranges, geolocation, or keyword filters. Sorting orders results based on specific fields or criteria.
Pagination and scroll
ElasticSearch supports pagination and scroll to handle large result sets efficiently in Node.js apps. Pagination and scroll allow retrieval and processing of large result sets with better performance and efficiency, using the elasticsearch.js client.
Best practices
Optimize ElasticSearch performance
To optimize performance in Node.js applications, it's important to use appropriate hardware, monitor cluster health, and use best practices such as shard allocation, indexing, and querying for efficient data handling.
Handle errors and exceptions
When working with ElasticSearch in Node.js applications, it's important to handle errors and exceptions effectively. This includes using the appropriate error handling techniques, logging errors for debugging, and handling network connectivity issues.
Real-world use cases
ElasticSearch is used in a variety of real-world applications such as e-commerce search, log analysis, and business intelligence. Real-world use cases can provide valuable insights into how ElasticSearch can be used in Node.js applications to solve business problems and improve search and analytics capabilities.
Conclusion:
When you hire dedicated nodejs developers you not only make extremely fast and scalable websites but can also take them to the next level by integrating elastic search in it as ElasticSearch offers powerful search and analytics capabilities that can help improve data handling and insights extraction in Node.js applications.
Subscribe to my newsletter
Read articles from Aaric Evans directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
