creating an HTTP server using Express.js in Node.js
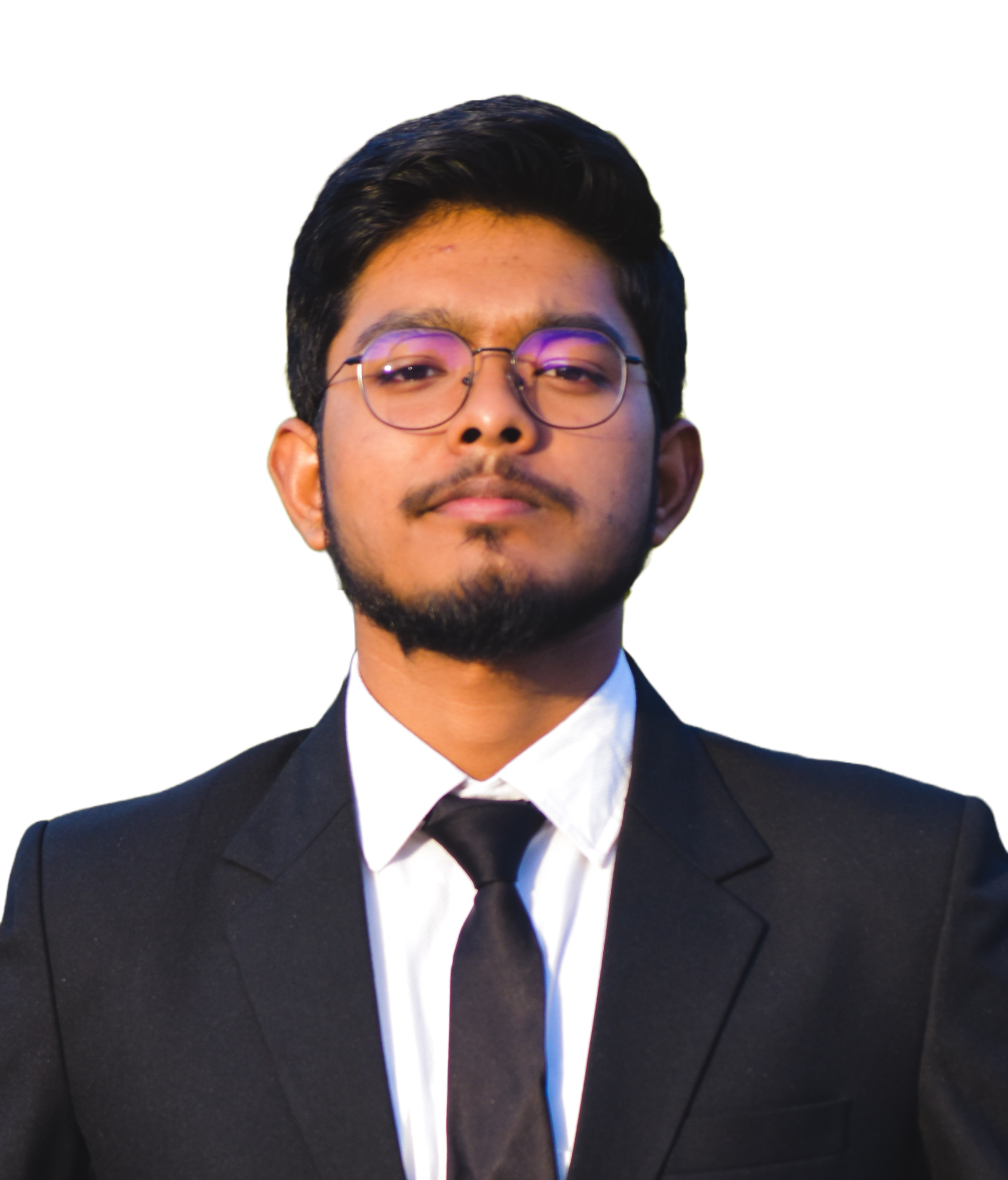
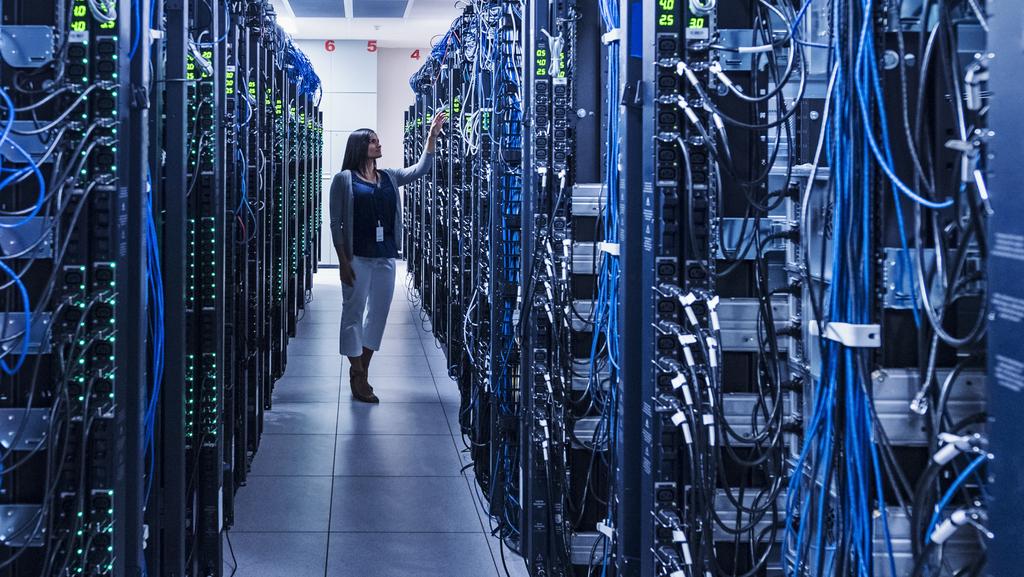
Start by creating a new directory for your project and navigate to it in your terminal.
Initialize a new Node.js project by running the following command:
npm init -y
- Install Express.js as a dependency by running the following command:
npm install express
Create a new file, for example
server.js
, and open it in a text editor.In
server.js
, import Express and create an instance of the Express application:
const express = require('express');
const app = express();
- Define a route that will handle incoming HTTP requests. For example, let's create a route that responds with "Hello, World!" when someone accesses the root URL ("/"):
app.get('/', (req, res) => {
res.send('Hello, World!');
});
- Set up the server to listen on a specific port. For instance, let's listen on port 8000:
const PORT = 8000;
app.listen(PORT, () => {
console.log(`Server is running on port ${PORT}`);
});
Save the file.
In your terminal, run the following command to start the server:
node server.js
You should see the message "Server is running on port 8000" in the console.
Now, you can open a web browser and navigate to
http://localhost:8000
to see the "Hello, World!" message.You can add more routes and functionality to your Express server as needed. This example provides a basic starting point for creating an HTTP server using Express.js.
Subscribe to my newsletter
Read articles from Arka Raha directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
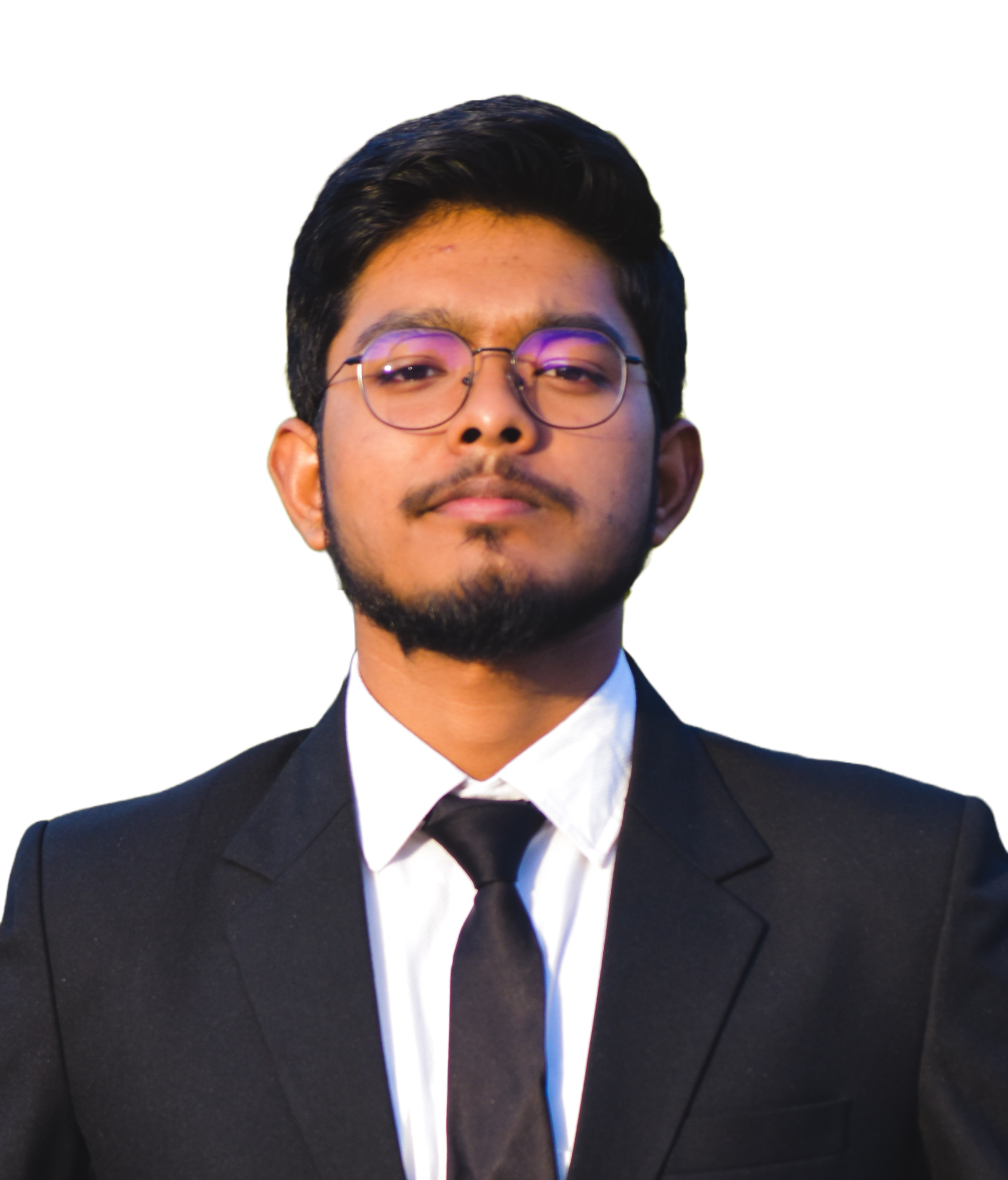
Arka Raha
Arka Raha
A results-oriented Software Engineer with entry-level experience, specializing in designing custom software solutions and driving new feature development. Learning and coordinating with on-shore and off-shore teams throughout the application development cycle. Adept at defining user requirements and driving scalability.