React interview preparation

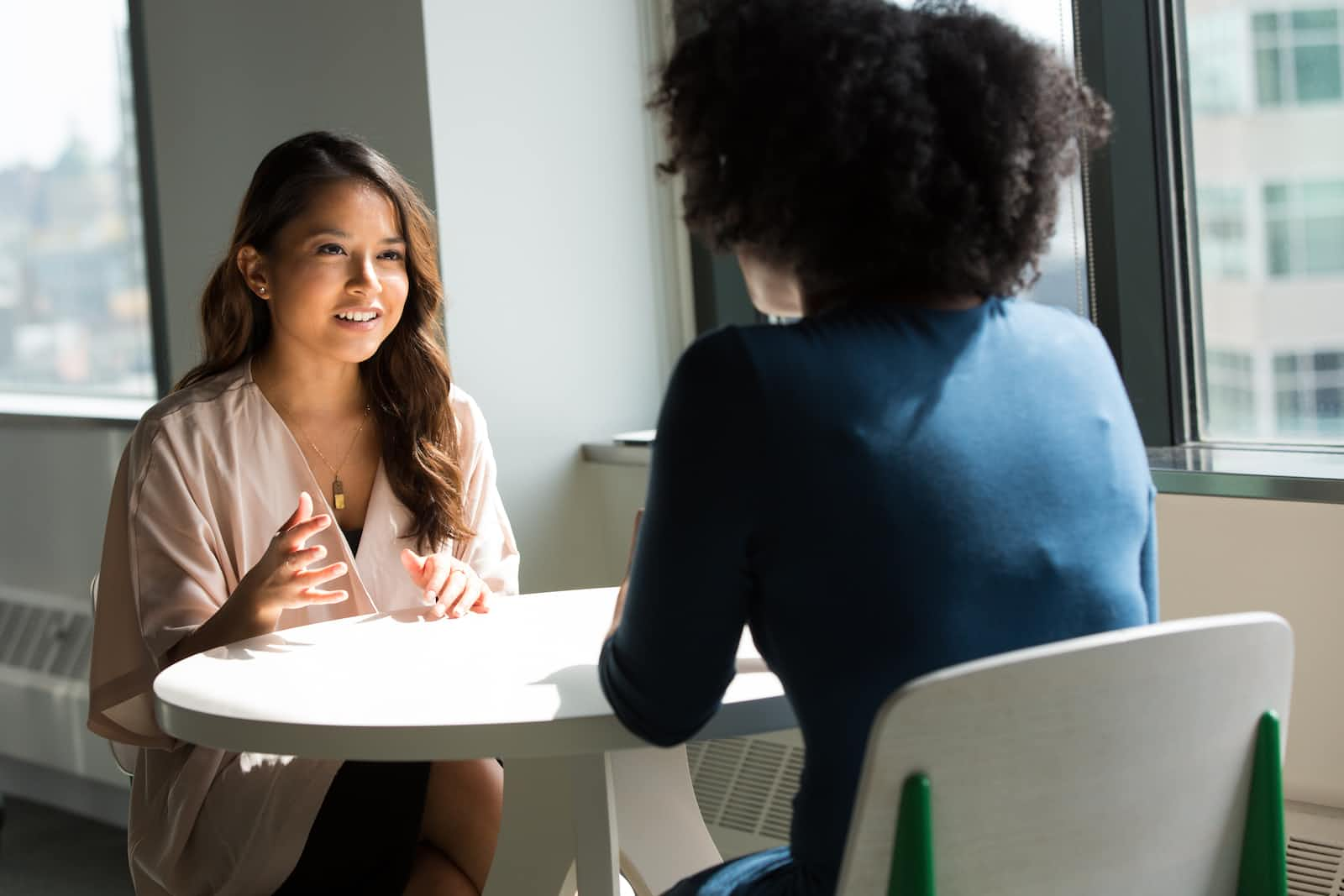
Arrow functions :
Arrow functions allow us to write shorter function syntax:
It gets shorter! If the function has only one statement, and the statement returns a value, you can remove the brackets and the return
keyword:
If you have parameters, you pass them inside the parentheses:
if you have only one parameter, you can skip the parentheses as well:
TypeOf operator :
You can use the typeof
operator to find the data type of a JavaScript variable.
Spread operator:
The JavaScript spread operator (...
) allows us to quickly copy all or part of an existing array or object into another array or object.
- Difference between var,const and let Keywords.
i. var variables can be reassigned while let and const variables can not.
ii. var variables are declared using the var keyword while let and const variables are declared using the let and const keywords respectively.
iii. const variables are immutable while let and var variables are not.
Hoisting :
Hoisting is JavaScript's default behavior when moving declarations to the top.
Assignment & Comparison operators :
Assignment Operator :
Comparison Operator :
Logical operators:
Arrays in js:
Data Structures in JavaScript:
Event-driven Programming :
An event-driven architecture uses events to trigger and communicate between decoupled services and is common in modern applications built with microservices. An event is a change in state, or an update, like an item being placed in a shopping cart on an e-commerce website.
Browser Storage(Local Storage,Session Storage and Cookies):
JSON.parse():
-> A common use of JSON is to exchange data to/from a web server.
-> When receiving data from a web server, the data is always a string.
-> Parse the data with JSON.parse()
, and the data becomes a JavaScript object.
.................. Before using JSON.parse ............
............................. After Using JSON.parse() ..........................
JSON.stringfy():
-> When sending data to a web server, the data has to be a string.
-> Convert a JavaScript object into a string with JSON.stringify()
.
.......................... Before using JSON.stringfy() ...............
........................... After using JSON.stringfy() ......................
CRUD operations:
CRUD stands for Create, Read, Update, and Delete, which make up these four fundamental operations. Because REST API calls invoke CRUD operations, understanding their relationship with one another is important for API developers and data engineers.
Distrubuted Systems Application:
A distributed system allows resource sharing, including software by systems connected to the network. Examples of distributed systems / applications of distributed computing : Intranets, Internet, WWW, email. Telecommunication networks: Telephone networks and Cellular networks.
Asynchronous JavaScript:
Asynchronous programming is an essential concept in JavaScript that allows your code to run in the background without blocking the execution of other code. Developers can create more efficient and responsive applications by using features like callbacks, async/await, and promises.
Beta version:
A pre-release of software that is given out to a large group of users to try under real conditions.
what is process.env in node js?
In Node. js, process. env is a global variable that is injected during runtime. It is a view of the state of the system environment variables. When we set an environment variable, it is loaded into process.
React fragment ?
React Fragments allow you to wrap or group multiple elements without adding an extra node to the DOM.
This can be useful when rendering multiple child elements/components in a single parent component
why we are using require in express and import in react?
One of the major differences between require() and import() is that require() can be called from anywhere inside the program whereas import() cannot be called conditionally, it always runs at the beginning of the file. To use the require() statement, a module must be saved with.
Socket.IOis a JavaScript library for realtime web applications. It enables realtime, bi-directional communication between web clients and servers. It has two parts: a client-side library that runs in the browser, and a server-side library for Node.js. Both components have a nearly identical API.
Body-parser ?
body-parser
is a middleware module for Node.js web applications, specifically those built on top of frameworks like Express.js. It is used to parse the incoming request bodies, particularly data sent in the HTTP POST request, and make it accessible in a convenient and usable format within your application.
When a client submits a POST request with data, such as form submissions or JSON data, the data is sent in the request body.body-parser
helps in extracting this data from the request body and converting it into a format that can be easily processed by your server-side code.
EJS ?
EJS, or Embedded JavaScript, is a template engine for JavaScript that enables you to generate dynamic HTML markup on the server-side. It's commonly used with Node.js and web frameworks like Express.js to create dynamic web pages and views.
With EJS, you can embed JavaScript code directly within your HTML templates. This allows you to generate HTML content dynamically based on data from your application. EJS templates are processed on the server-side before being sent to the client's browser, which can improve performance and allow for more advanced templating features compared to client-side rendering.
Async/await ?
why should we use axios but not fetch ?
Difference b/w stateful and stateless components?
Stateless Components: They don't have internal state and are purely based on the props they receive. They are simpler and easier to understand.
Stateful Components: They have their own internal state, which can change over time based on user interactions or other factors. They manage their own state and handle more complex logic.
useMemo()?
With useMemo , React can store the result of a function call and reuse it when the dependencies of that function haven't changed, rather than recalculating the value on every render
What is hoc?
A Higher-Order Component (HOC) is a pattern used in React to reuse component logic. It's a function that takes a component and returns a new enhanced component with additional features or behavior. HOCs help in separating concerns and making components more modular and reusable.
Pure function?
i) it takes two input parameters.
ii) It does not modify any variables outside its scope.
Event loop ?
The event loop continuously checks the call stack and the callback queue. If the call stack is empty, the event loop will take the first callback from the callback queue and push it onto the call stack for execution.
setTimeout and setInterval
setTimeout: It's a function in JavaScript that executes a callback function after a specified delay (in milliseconds). It triggers the function once after the delay.
setInterval: Also a function in JavaScript, it repeatedly calls a callback function at a specified interval (in milliseconds). It continues to trigger the function at regular intervals until it's cleared or stopped.
where we use rest operator?
The rest operator (
...
) in JavaScript is used to gather remaining parameters into an array. We typically use it in two main contexts:Function Parameters: To gather all remaining arguments into an array, especially useful when the number of arguments is not fixed.
Array Destructuring: To collect the remaining elements of an array into a new array variable.
what is meant by sallow copy and deep copy?
SALLOW: In Shallow copy, a copy of the original object is stored and only the reference address is finally copied.
DEEP: In Deep copy, the copy of the original object and the repetitive copies are both stored.
what are closure?
a closure gives you access to an outer function's scope from an inner function.
Diff b/w map and reduce?
Promises and callback?
. Callback: A callback is a function passed into another function as an argument. It's executed later when a particular event happens or when a task is completed. Callbacks are commonly used for handling asynchronous operations in JavaScript.
Promises: Promises are objects in JavaScript used for asynchronous operations. They represent the eventual completion (or failure) of an asynchronous operation, and allow chaining of operations using
.then()
and handling errors with.catch()
.useCallBack() ?
useCallback()
is a hook provided by React that memoizes a callback function. This means it returns a memoized version of the callback that only changes if one of the dependencies has changed. It's useful for optimizing performance by avoiding unnecessary re-renders of child components that rely on that callback.What is useEffect and when to use?
useEffect
is a hook provided by React that allows you to perform side effects in function components. Side effects include data fetching, subscriptions, or manually changing the DOM.When to Use
useEffect
:Fetching Data: Use
useEffect
to fetch data from an API or server when the component mounts.Subscriptions: If your component needs to subscribe to some external event (like WebSocket updates),
useEffect
can manage the subscription.DOM Manipulation:
useEffect
can perform imperative DOM operations after the component renders.Event Listeners: Attach or remove event listeners using
useEffect
.
What is useRef?
how to implement lazy loading in react-router?
how handle errors in react?
what is the error boundary in react?
Subscribe to my newsletter
Read articles from Mohan Krishna Madanapu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
