React Data Table: A Comprehensive Guide

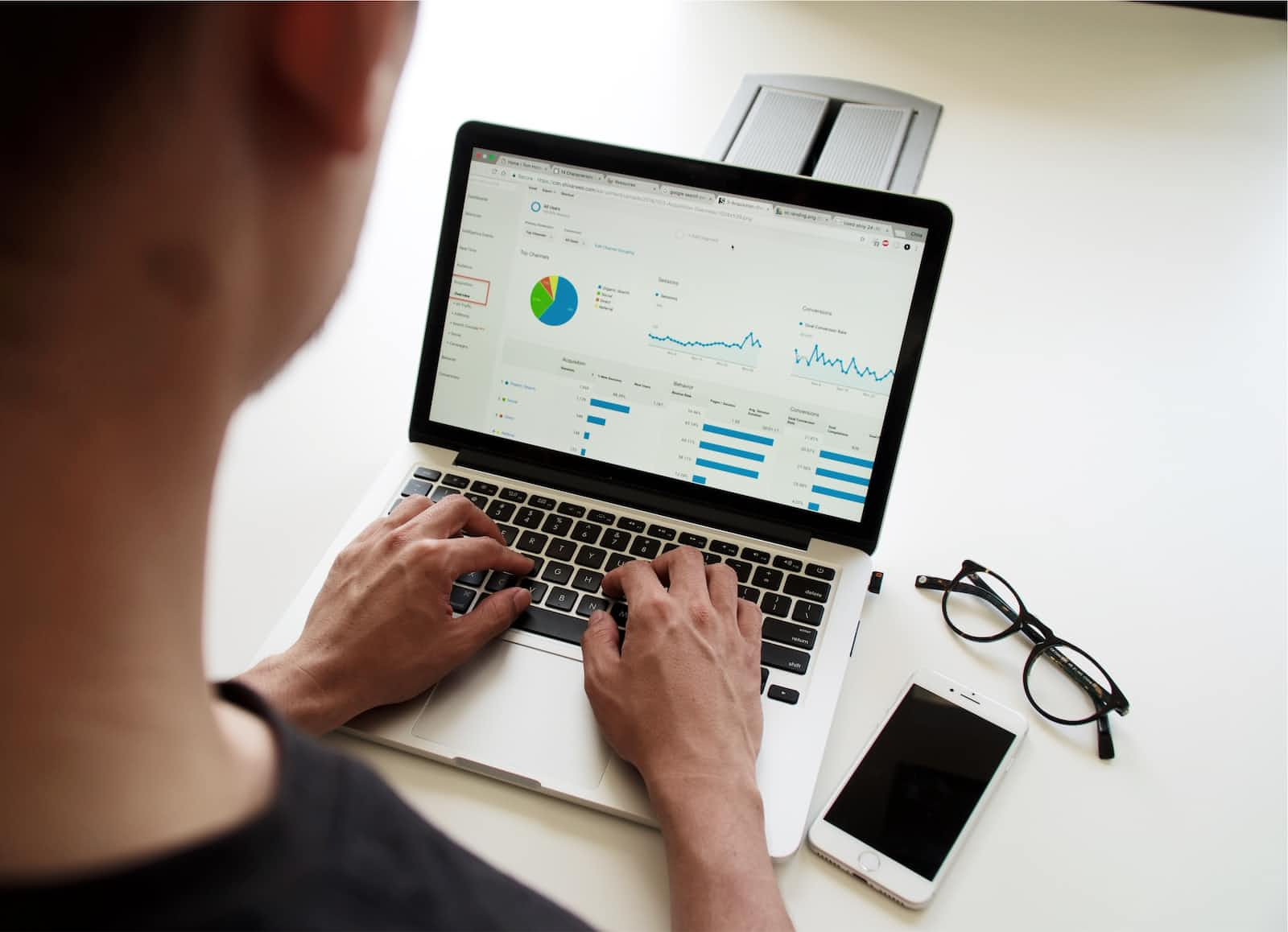
Table of contents:
What is a React Data Table?
Key Features of React Data Tables
Implementing React Data Tables
3.1 Installation and Setup
3.2 Building a Basic Data Table
3.3 Handling Sorting and Filtering
3.4 Customizing the Table AppearanceBest Practices for Using React Data Tables
4.1 Data Fetching and Pagination
4.2 Handling Large Datasets
4.3 Accessibility Considerations
4.4 Responsive Design and Mobile CompatibilityAdvanced Features and Customizations
5.1 Adding Pagination and Infinite Scrolling
5.2 Implementing Row Selection and Actions
5.3 Server-side Data Processing
5.4 Internationalization and LocalizationConclusion
What is a React Data Table?
A React Data Table is a component in the React JavaScript library that enables the creation of interactive and customizable tables for displaying and managing data. It provides a structured and organized way to present large datasets in a tabular format, allowing users to sort, filter, and interact with the data.
Key Features of React Data Tables:
Data Rendering: React Data Tables allow for the efficient rendering of large datasets by utilizing virtualization techniques. Only the visible rows are rendered, improving performance and reducing memory usage.
Sorting: Sorting functionality is an essential feature of data tables. React Data Tables enable users to sort columns in ascending or descending order, making it easier to organize and analyze data based on specific criteria.
Filtering: Filtering allows users to narrow down the dataset based on specific conditions. React Data Tables provide options for creating filter controls, such as input fields or dropdown menus, to dynamically filter the displayed data.
Pagination: When dealing with a large dataset, pagination allows for dividing the data into smaller, manageable chunks or pages. React Data Tables provide pagination controls to navigate through the different pages of the table, improving performance and user experience.
Customization: React Data Tables offer a high level of customization, allowing developers to tailor the table's appearance and behavior to meet specific requirements. Customizations include styling, column resizing, column reordering, and custom cell rendering.
Row Selection and Actions: React Data Tables enable users to select one or multiple rows, providing functionality for performing actions on the selected rows, such as deleting, editing, or exporting data.
Responsive Design: With the increasing use of mobile devices, responsive design is crucial for providing an optimal user experience. React Data Tables can adapt to different screen sizes and orientations, ensuring that the table remains readable and usable on various devices.
Accessibility: Accessibility is an important aspect of web development. React Data Tables can be designed and implemented to meet accessibility standards, ensuring that users with disabilities can navigate and interact with the table using assistive technologies.
Integration with Backend APIs: React Data Tables can seamlessly integrate with Backend APIs to fetch and update data. This enables real-time data synchronization and provides a more dynamic and interactive experience for users.
Extensibility: React Data Tables are highly extensible, allowing developers to add additional features and functionality as needed. This can include integration with charting libraries, exporting data in various formats, or implementing advanced data processing algorithms.
By leveraging these key features, React Data Tables provide a robust solution for presenting, managing, and interacting with data in a tabular format, making them a valuable tool for building powerful data-driven UIs in React applications.
Implementing React Data Tables
3.1 Installation and Setup:
To start implementing React Data Tables, you need to have a React project set up. Here are the steps to install and set up the necessary dependencies:
Step 1: Create a new React project or navigate to your existing project's directory.
Step 2: Open the command line or terminal and run the following command to install the required packages:
npm install react-table
Step 3: Wait for the installation to complete. Once done, you can import the necessary components and styles in your project to begin building the data table.
3.2 Building a Basic Data Table:
Once you have the dependencies installed and imported into your project, you can start building a basic data table. Here's an example of how to create a simple table:
Step 1: Import the required components from the react-table
package:
import ReactTable from 'react-table';
import 'react-table/react-table.css';
Step 2: Set up your data source, such as an array of objects containing the table rows and columns:
const data = [
{ id: 1, name: 'John', age: 25 },
{ id: 2, name: 'Jane', age: 30 },
{ id: 3, name: 'Bob', age: 35 },
// Add more data rows as needed
];
Step 3: Define the columns for your table:
const columns = [
{ Header: 'ID', accessor: 'id' },
{ Header: 'Name', accessor: 'name' },
{ Header: 'Age', accessor: 'age' },
];
Step 4: Render the table component with the data and columns:
const BasicTable = () => {
return <ReactTable data={data} columns={columns} />;
};
Step 5: Finally, render the BasicTable
component wherever you want to display the data table in your application.
This will create a basic data table with the provided data and columns.
3.3 Handling Sorting and Filtering:
React Data Tables provide built-in sorting and filtering functionality. To enable sorting and filtering, you need to make slight modifications to the table's columns.
Step 1: Update the column definitions to include the sortable
and filterable
properties:
const columns = [
{ Header: 'ID', accessor: 'id', sortable: true },
{ Header: 'Name', accessor: 'name', sortable: true },
{ Header: 'Age', accessor: 'age', sortable: true },
];
Step 2: Render the updated BasicTable
component:
const BasicTable = () => {
return <ReactTable data={data} columns={columns} defaultPageSize={10} />;
};
With these modifications, the table headers will have sorting icons, and you can click on the headers to sort the data accordingly. Additionally, the table will have a search input to filter the data based on the entered text.
3.4 Customizing the Table Appearance:
React Data Tables offer various customization options to match the table's appearance with your application's design. You can modify the table's styles, add custom CSS classes, and customize the cell rendering.
Step 1: Add custom CSS classes or inline styles to the table component:
const BasicTable = () => {
return (
<ReactTable
data={data}
columns={columns}
className="-striped -highlight" // Add custom classes
style={{ background: 'white' }} // Add inline styles
/>
);
};
Step 2: Customize the cell rendering using the Cell
property in the column definition:
const columns = [
{ Header: 'ID', accessor: 'id', Cell: (cell) => <div className="custom-cell">{cell.value}</div> },
// Define other columns
];
By specifying the Cell
property and providing a custom render function, you can apply custom styling or add additional elements to the cells.
These are just a few examples of how you can implement and customize React Data Tables. The react-table
package provides extensive documentation with more advanced features and customization options, such as pagination, row selection, and cell editing. By exploring the documentation and experimenting with the available features, you can create powerful and highly customized data tables in your React applications.
Best Practices for Using React Data Tables
4.1 Data Fetching and Pagination:
When working with large datasets, it's best to fetch data asynchronously instead of loading all the data at once. This can be achieved by implementing pagination, where data is fetched in smaller chunks as the user navigates through the table.
To implement pagination with React Data Tables, you can use the react-table
package's built-in pagination functionality. This allows you to specify the number of rows to be displayed per page and provides controls for navigating between pages.
4.2 Handling Large Datasets:
When dealing with large datasets, performance can become a concern. To ensure smooth rendering and interactions, consider implementing the following techniques:
Virtualization: Use libraries like
react-virtualized
orreact-window
to render only the visible rows, dynamically rendering additional rows as the user scrolls. This improves performance by reducing the number of DOM elements rendered.Server-Side Pagination and Filtering: If your dataset is too large to fetch all the data at once, consider implementing server-side pagination and filtering. This allows you to make API calls to fetch only the necessary data based on the current page and filtering criteria.
4.3 Accessibility Considerations:
To ensure your data table is accessible to all users, follow these accessibility best practices:
Use Semantic HTML: Use appropriate HTML elements (
table
,thead
,tbody
,th
,tr
,td
) to structure your data table. This helps screen readers and assistive technologies understand the table's structure.Provide Descriptive Labels: Use
aria-label
oraria-labelledby
attributes to provide descriptive labels for the table and its elements. This helps users with visual impairments understand the purpose and context of the table.Keyboard Navigation: Ensure that users can navigate through the table using keyboard shortcuts and tab navigation. Implement keyboard accessibility features, such as arrow key navigation, focus management, and support for assistive technologies.
4.4 Responsive Design and Mobile Compatibility:
With the increasing use of mobile devices, it's crucial to make your data tables responsive and mobile-friendly. Consider the following practices:
Responsive Layout: Design your table to adapt to different screen sizes. Use CSS media queries to adjust the table's layout and styling based on the viewport size.
Mobile Optimization: Simplify the table's design and prioritize important information for mobile users. Hide less critical columns or provide collapsible sections to enhance the user experience on smaller screens.
Touch-friendly Interactions: Optimize the table's interactions for touch devices. Consider using touch gestures, such as swipe actions for pagination or filtering, to improve usability on mobile devices.
By following these best practices, you can enhance the usability, performance, and accessibility of your React Data Tables. Remember to consider the specific requirements of your application and adapt these practices accordingly to provide the best user experience for your users.
Advanced Features and Customizations for React Data Tables
5.1 Adding Pagination and Infinite Scrolling:
Pagination allows users to navigate through large datasets by displaying a limited number of rows per page. It improves performance by reducing the amount of data loaded at once. React Data Tables often provide built-in pagination support, allowing you to specify the number of rows per page and implement controls for navigating between pages.
Infinite scrolling is an alternative to pagination where new data is loaded dynamically as the user scrolls. This provides a seamless scrolling experience without the need for explicit pagination controls. Implementing infinite scrolling requires handling scroll events and making asynchronous API calls to fetch additional data as needed.
5.2 Implementing Row Selection and Actions:
Row selection allows users to select one or multiple rows in the data table. This feature is useful when performing batch actions or applying specific operations to selected rows. To implement row selection, you can use checkboxes or other interactive elements within each row and maintain the selected state in your component's state.
Once row selection is implemented, you can provide actions such as delete, edit, or perform other operations on the selected rows. These actions can be triggered through buttons or contextual menus associated with the selected rows.
5.3 Server-side Data Processing:
In some cases, the dataset may reside on the server, and you need to perform data processing or filtering on the server side. Implementing server-side data processing involves sending requests to the server with filtering, sorting, and pagination parameters, and receiving the processed data as a response. This approach is particularly useful for handling large datasets and optimizing performance.
To implement server-side data processing, you need to define an API endpoint that accepts the necessary parameters and performs the required operations on the server side. Your React Data Table component then makes requests to this endpoint and updates the table based on the received data.
5.4 Internationalization and Localization:
If your application needs to support multiple languages or localize the content of the data table, implementing internationalization and localization is essential. This allows you to display table headings, labels, and other textual elements in different languages based on the user's locale.
To implement internationalization and localization, you can leverage localization libraries such as react-intl
or create your own localization system. This involves managing language files or translation dictionaries and dynamically rendering the table content based on the selected language.
By incorporating these advanced features and customizations into your React Data Tables, you can provide a more comprehensive and tailored user experience. Consider the specific requirements of your application and the needs of your users to determine which features and customizations are most relevant.
Conclusion:
React Data Tables offer a powerful and flexible solution for presenting, managing, and interacting with tabular data in web applications. By understanding the key features, implementation strategies, customization options, and best practices outlined in this article, developers can leverage the full potential of React Data Tables to create intuitive and efficient data-driven UIs. Whether you're building an e-commerce platform, financial analytics dashboard, or project management tool, mastering React Data Tables will elevate your web development skills and enhance the user experience of your applications.
Subscribe to my newsletter
Read articles from Darshana Mallick directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Darshana Mallick
Darshana Mallick
I am a Web Developer and SEO Specialist.