Smooth Data Modeling with 'Freezed'
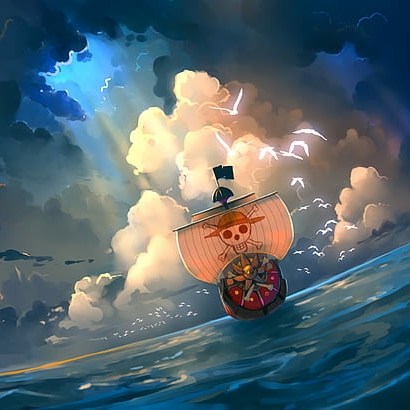
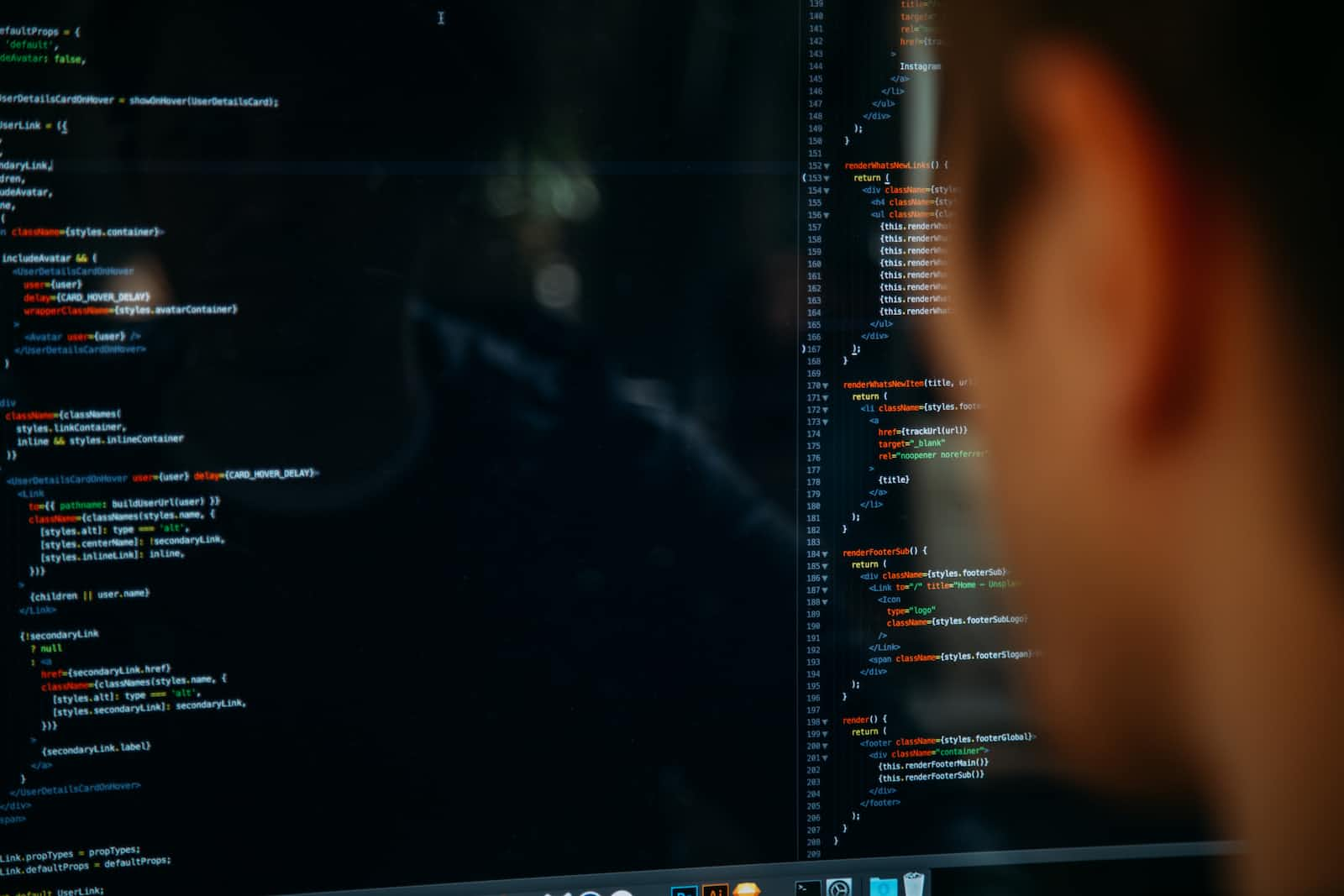
Introduction
Freezed, is the ultimate solution for painless data class creation and seamless state management in Flutter.
With Freezed, you can effortlessly generate concise data classes, eliminating repetitive code and enhancing stability. Plus, Freezed empowers you to handle UI updates effortlessly, simplifying state management as your app grows.
Say goodbye to tedious tasks and hello to streamlined Flutter development with Freezed.
Installation :
You can power up your project with Freezed using the commands mentioned below. (I recommend using this method to get the latest versions of dependencies)
flutter pub add freezed_annotation
flutter pub add --dev build_runner
flutter pub add --dev freezed
If you want to use toJson (toMap) & fromJson (fromMap) methods you have to add commands mentioned below also
flutter pub add json_annotation
flutter pub add --dev json_serializable
You can go to the Basics sections now.
Alternate Installation :
You can edit pubspec.yaml file to get dependencies like below
(You have to check for the latest or your preferred versions)
dependencies:
## Add The Following lines
freezed_annotation: ^2.2.0
json_annotation: ^4.8.1
dev_dependencies:
build_runner: ^2.4.4
freezed: ^2.3.5
json_serializable: ^6.7.0
Basics :
Now that Freezed is installed, get ready to experience the incredible ease and efficiency it brings to data class creation in Flutter
If you're new to code generation-based packages, it may initially seem confusing. But trust me, once you start using Freezed, you'll have a new level of productivity with minimal hassle.
It saves you from repetitive code and by reducing the chances of errors.
Creating a simple data class :
part 'person.freezed.dart';
// Required if you want to generate toMap and fromMap methods
part 'person.g.dart';
@freezed
class Person with _$Person {
const factory Person({
required String firstName,
required String lastName,
required int age,
}) = _Person;
// Required if you want to generate toMap and fromMap methods
factory Person.fromJson(Map<String, Object?> json)
=> _$PersonFromJson(json);
}
This code will throw compile time errors but don't be confused it will go away once you generate the required code
To generate code for a freezed class we have already added 'build_runner' as a dev dependency.
dart run build_runner build
Run the above command inside the terminal to generate the required code.
Done, Now you have created a Person data class with all the required methods with so much less code.
More Information about written code
Some Commonly used Annotations on properties :
@Default()
You can provide a default value to a property using this.
class Example with _$Example { const factory Example({ @Default('value') required String property, }) = _Example; }
@JsonKey()
Very common to use while working with APIs.
You can change behaviour of fromMap and toMap functionalities using this annotation on a property of the data class.
@freezed class Example with _$Example { factory Example(@JsonKey(name: 'my_property') String myProperty) = _Example; factory Example.fromJson(Map<String, dynamic> json) => _$ExampleFromJson(json); }
This will map 'my_property' value from Json Response from API to 'myProperty' field of your generated class.
@JsonKey also contains many useful properties for commonly used functionalities while working with JSON response.
Bonus Tips:
- You can generate freezed code on save of your class code file if you use :
dart run build_runner watch --delete-conflicting-outputs
instead of the above build command
- If you are using Visual Studio Code and want to hide the generated files you can create a file in your project such as :
- <projectRoot>/.vscode/settings.json
{
"files.exclude": {
"**/*.freezed.dart": true,
"**/*.g.dart": true
},
}
Conclusion:
I have worked with model classes a lot in my previous projects, It was very tedious to change all the methods if I want to change a property in a class and it also increases the chances of silly mistakes.
Once I started to do this using freezed it is very easy and efficient that now I have forgotten the feeling of maintaining a data class.
So I just wanted to share it with the community and especially beginners that don't know about this type of thing.
Subscribe to my newsletter
Read articles from Jemish Mavani directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
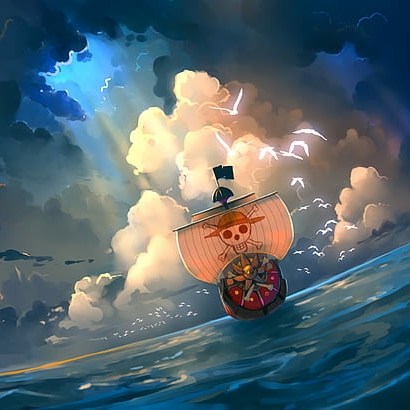