Arrays in JavaScript (1/3)

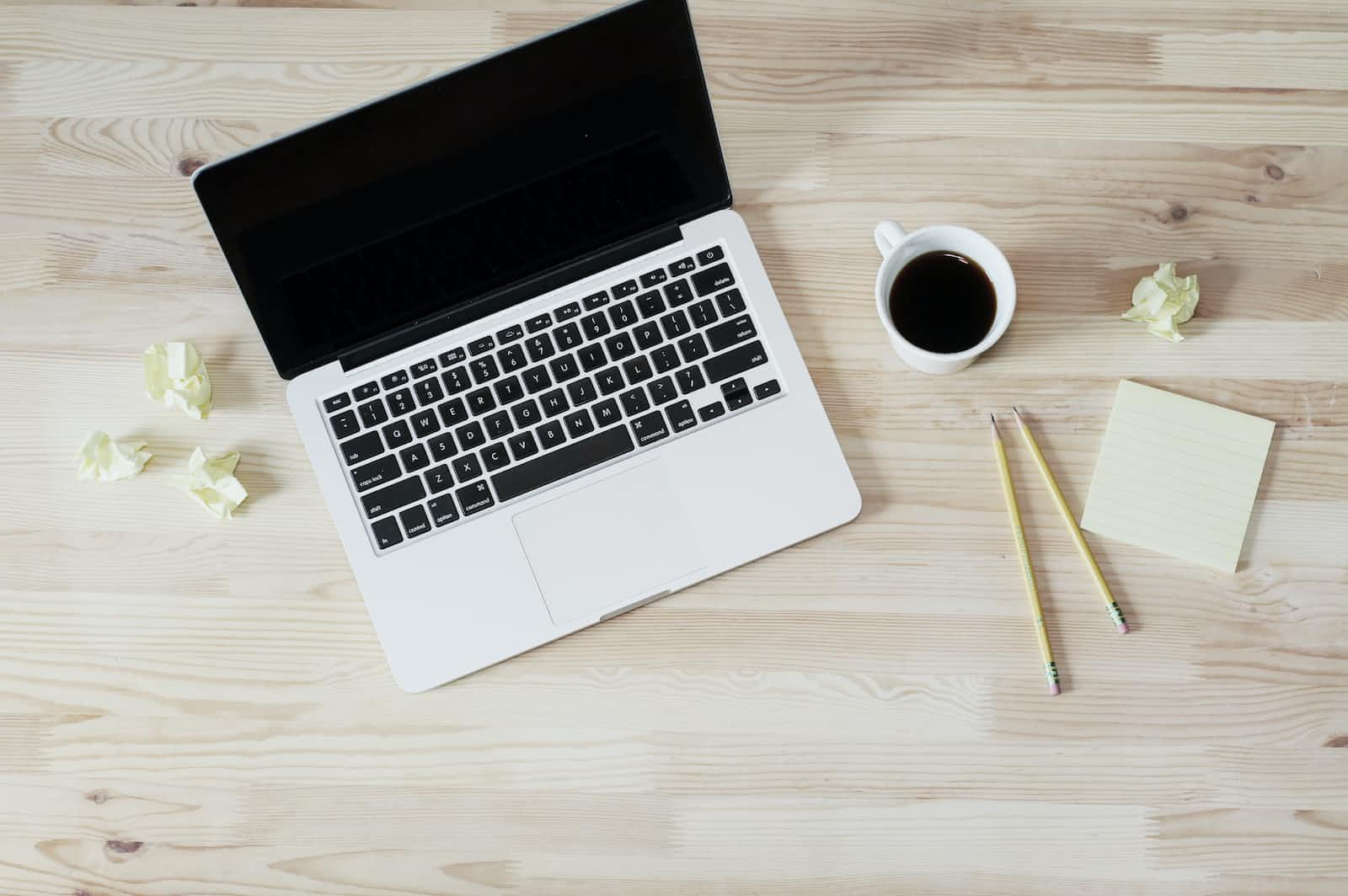
Introduction
Arrays are fundamental data structure in JavaScipt that enables you to store and manipulate a collection of values.
Arrays are a non-primitive type of data structure means they can store multiple values in a simple variable.
They provide a convenient way to organize related data elements and perform various operations on them.
In this article, we will delve into arrays in JavaScript, covering their creation, properties, common methods, and essential operations. By the end, you will have a solid understanding of arrays and can be ready to utilize them effectively in your JavaScript projects.
Fun Fact: Arrays in JS are flexible
Arrays in JS have the remarkable ability to store different types of values, such as strings, numbers, and even objects,...etc within a single variable.
The flexibility is due to JavaScript's dynamic typing and the absence of strict type enforcement. So, the next time you're working with arrays in JavaScript, remember that they can happily hold a diverse range of data types, making them incredibly versatile and adaptable.
Let's embrace the power of arrays and explore the exciting possibilities they offer in your coding adventures!
The Importance of Arrays in JavaScript: Organize, Access, and Manipulate Your Data
Introduction:
Arrays are fundamental components of JavaScript that offer numerous benefits when it comes to managing and manipulating the collection of data. By grouping related data elements, providing efficient storage, enabling easy access and modification, supporting iteration and manipulation, and accommodating dynamic size and diverse data types, arrays play a crucial role in JS programming. so, let's explore why arrays are essential in JS and how they enhance our abilities to organize, access and manipulate data effectively.
Grouping Related Data:
One of the primary reasons we need arrays in JavaScript is to group related data elements under a single variable. Instead of creating separate variables for each piece of data, an array allows us to organize them logically and intuitively within a structured data structure. Whether it's a list of names, a collection of numbers, or any other related data, arrays provide a convenient way to group and manage these values efficiently.
Efficient Data Storage:
Arrays excel at efficient data storage. Instead of cluttering our code with individual variables, arrays allow us to store multiple values sequentially within a single data structure. This promotes code simplicity and readability by eliminating the need for excessive variable declarations. With arrays, we can keep our code concise and organized, enhancing its maintainability and understandability.
Accessing and Modifying Elements:
Arrays in JavaScript offer easy access to specific elements through their indexes. Each element in an array is assigned a unique index, starting from 0. This enables efficient retrieval and modification of values based on their positions within the array. By using the index, we can directly access and update elements, making arrays a powerful tool for managing data.
Iteration and Manipulation:
Arrays facilitate iteration over their elements using loops or built-in methods. This allows us to perform operations on each element, such as calculations, transformations, or filtering based on specific conditions. Whether we need to sum all the numbers in an array or filter out specific values, arrays provide a convenient way to manipulate data effectively.
Ordered Collection:
One of the key features of arrays is their ability to maintain the order of elements. When elements are added to an array, their order is preserved. This characteristic is crucial when dealing with sequences or when maintaining a specific order of data is essential. By relying on the index, we can access elements based on their relative positions, ensuring data consistency and integrity.
Dynamic Size:
JavaScript arrays have a dynamic size, meaning we can add or remove elements as needed. This flexibility allows arrays to adapt to changing data requirements during runtime. We can dynamically resize arrays to accommodate new data or shrink them to optimize memory usage. This feature enhances the flexibility and versatility of arrays in JavaScript.
Storing Different Data Types:
Another advantage of arrays in JavaScript is their ability to store elements of different data types within a single array. This flexibility allows us to combine strings, numbers, booleans, objects, or even other arrays in a single data structure. Arrays empower us to work with diverse data types, providing versatility in handling various kinds of information.
Conclusion:
Arrays are a vital tool in JavaScript programming, offering significant advantages when it comes to organizing, accessing, and manipulating data. By grouping related data, efficiently storing multiple values, enabling easy access and modification, supporting iteration and manipulation, accommodating dynamic size, and allowing storage of different data types, arrays provide a solid foundation for efficient data management. Understanding and effectively utilizing arrays will empower you to write cleaner, more organized, and more powerful JavaScript code. Embrace the power of arrays and unlock the full potential of your data manipulation capabilities.
Creating an Array in JavaScript:
Simple Array
To create an array in JavaScript, you can follow a simple syntax using square brackets [] and separating the elements by commas. Here's an example:
let fruits = ['apple', 'banana', 'orange'];
In the above code snippet, we have created an array called fruits
that contains three elements: 'apple', 'banana', and 'orange'. Each element is enclosed in single quotes and separated by a comma. However, you can use double quotes or no quotes at all for strings as well.
Mixed Array
Arrays can also store values of different data types, including numbers, strings, booleans, objects, or even other arrays. Here's an example of an array with different data types:
let mixedArray = ['apple', 123, true, { name: 'John', age: 25 }];
In this case, a mixed array
contains a string, a number, a boolean, and an object as its elements. This illustrates the flexibility of arrays in JavaScript to store various types of data within a single data structure.
Empty Array
You can also create an empty array and populate it with elements later. Here's an example:
let emptyArray = [];
emptyArray[0] = 'first';
emptyArray[1] = 'second';
emptyArray[2] = 'third';
In this example, we initialized an empty array emptyArray
and assigned values to its elements using the index. The index starts from 0, so emptyArray[0]
represents the first element, emptyArray[1]
represents the second element, and so on.
By Constructor
Arrays can also be created using the Array
constructor. Here's an example:
let numbers = new Array(1, 2, 3, 4, 5);
In this case, we used the Array
constructor with a list of numbers as arguments to create the numbers
array.
Summary
In summary, creating an array in JavaScript involves using square brackets [ ] to enclose the elements, separated by commas. Arrays can store values of different data types and can be initialized with elements or created as empty arrays. Understanding how to create arrays is essential for effectively utilizing their capabilities in JavaScript programming.
Accessing an Array in JavaScript:
Once an array is created, accessing its elements is a common operation in JavaScript. You can retrieve specific elements from an array using their index value, which starts from 0 for the first element. Let's explore different ways to access array elements:
Using Index:
To access a specific element in an array, you can use the index within square brackets after the array name. Here's an example:
let fruits = ['apple', 'banana', 'orange'];
console.log(fruits[0]); // Output: 'apple'
console.log(fruits[1]); // Output: 'banana'
console.log(fruits[2]); // Output: 'orange'
In the above code snippet, we accessed the first element of the fruits
array using fruits[0]
, which returns apple
. Similarly, fruits[1] returns banana
, and fruits[2]
returns orange
.
Dynamic Access:
Array elements can also be accessed dynamically using variables or expressions as the index value. For example:
let index = 1;
console.log(fruits[index]); // Output: 'banana'
let expression = 2 + 1;
console.log(fruits[expression]); // Output: 'orange'
In this case, we used the variables index
and expression
to access elements dynamically. The value of index
represents the index position we want to access, and the expression holds an expression
that evaluates to the desired index.
Negative Indexing:
JavaScript arrays also support negative indexing, where -1 represents the last element, -2 represents the second-to-last element, and so on. Here's an example:
console.log(fruits[-1]); // Output: 'orange'
console.log(fruits[-2]); // Output: 'banana'
console.log(fruits[-3]); // Output: 'apple'
In this example, we accessed elements using negative indexes, retrieving the last, second-to-last, and third-to-last elements of the fruits
array.
Array Length and Bounds:
You can obtain the length of an array using the length
property. It returns the total number of elements in the array. However, it's important to note that array indexes should be within the bounds of the array, from 0 to length-1. Accessing an index outside these bounds will result in undefined
. Here's an example:
console.log(fruits.length); // Output: 3
console.log(fruits[3]); // Output: undefined
In the above code, fruits.length
returns 3, indicating that there are three elements in the fruits
array. Attempting to access fruits[3
] is outside the array bounds and returns undefined
.
Conclusion
By understanding these various techniques, you can easily access and retrieve specific elements from arrays in JavaScript. Arrays provide a flexible and efficient way to store and retrieve data, making them a powerful tool in your JavaScript coding arsenal.
Subscribe to my newsletter
Read articles from Kapil Chaudhary directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
